First, reference
Two. Example
1. Common spinner usage and data loading in string array. This UI is in MD style. In fact, there are different UI displays in different themes. You can also choose dropdown mode: dropdown or dialog, which is dropdown by default
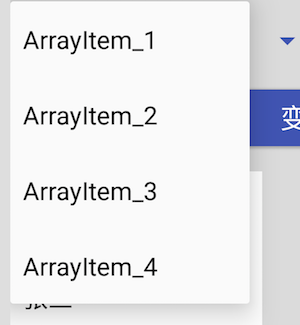
1-2.png
Layout:
<!--Simplest load drop-down array: entries,Content defined in string-array in--> <Spinner android:id="@+id/spinner_simple" android:layout_marginTop="10dp" android:layout_width="200dp" android:layout_height="50dp" android:entries="@array/study_view_spinner_values" />
2. Next change the following styles: 1. Data change 2. After selection, the filled text turns red in the middle 3. The pull-down text turns green in the middle 4. Various width and height controls, etc
Note: the custom layout loaded in ArrayAdapter must be textview, otherwise an exception will be reported: ArrayAdapter requires the resource ID to be a TextView

1-3.png
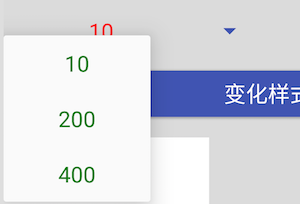
1-4.png
Code:
/** * Spinner custom style * 1,Spinner TextView style in: item [Select] * 2,Spinner TextView style of each item in the drop-down: item [drop] * 3,Spinner Dropdown styles, property settings * */ public void ChangeSpinner(View v){ mSpinnerSimple.setDropDownWidth(400); //Pull-down width mSpinnerSimple.setDropDownHorizontalOffset(100); //Pull down lateral offset mSpinnerSimple.setDropDownVerticalOffset(100); //Drop down vertical offset //Mspinnersimple. Setbackgroundcolor (apputil. Getcolor (instance, r.color. Wx? BG? Gray)); / / drop down background color //spinner mode : dropdown or dialog , just edit in layout xml //mSpinnerSimple.setPrompt("Spinner Title"); / / pop up box title, valid under dialog String[] spinnerItems = {"10","200","400"}; //Customize the font style after filling //Only textview style is allowed, otherwise an error is reported: ArrayAdapter requires the resource ID to be a TextView ArrayAdapter<String> spinnerAdapter = new ArrayAdapter<>(instance, R.layout.item_select, spinnerItems); //Custom drop-down font style spinnerAdapter.setDropDownViewResource(R.layout.item_drop); //Under different themes, the display effect is different //spinnerAdapter.setDropDownViewTheme(Theme.LIGHT); mSpinnerSimple.setAdapter(spinnerAdapter); }
Layout: item [select.xml
<?xml version="1.0" encoding="utf-8"?> <TextView xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:textColor="@color/red" android:textSize="@dimen/normal_text_size"/>
Layout: item'drop.xml
<?xml version="1.0" encoding="utf-8"?> <TextView xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:gravity="center" android:textColor="@color/green" android:textSize="@dimen/normal_text_size"/>
3. Set various properties in the spinner layout to achieve the effect, and increase the listening. The listening note is that the AdapterView.OnItemSelectedListener is this. The meaning of the callback return value in the listening is in the code comment. By default, there are two simple loading layouts: android.r.layout.simple ﹐ spinner ﹐ item ---- - android.r.layout.simple ﹐ spinner ﹐ dropdown ﹐ item
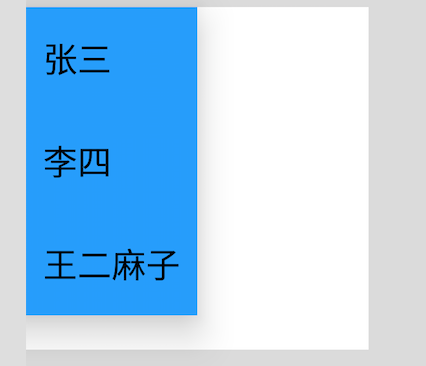
2-2.png
Layout:
<!-- //Code loading drop-down array, various properties, and listening 1,Drop down or pop-up spinnerMode="dropdown" spinnerMode="dialog" 2,Drop down width: default width and spinner Almost. Remove the width of the pull-down button on the left dropDownWidth="xxdp" 3,Drop down background color popupBackground="resColor/resMipmap" 4,Background color: hides the drop-down button on the right by default background="resColor/resMipmap" --> <Spinner android:id="@+id/spinner_1" android:layout_marginTop="10dp" android:layout_width="160dp" android:layout_height="160dp" android:gravity="center" android:spinnerMode="dropdown" android:dropDownWidth="80dp" android:popupBackground="@color/wx_blue" android:background="@color/white" />
Code: there will be selective listening
/** * Test: load data column, monitor and select * */ private void testSpinner1(){ //Original string array final String[] spinnerItems = {"Zhang San","Li Si","Wang two pock marks"}; //Simple string array adapter: style res, array ArrayAdapter<String> spinnerAdapter = new ArrayAdapter<>(instance, android.R.layout.simple_spinner_item, spinnerItems); //Drop down style res spinnerAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); //Bind Adapter to control mSpinner1.setAdapter(spinnerAdapter); //Selective monitoring mSpinner1.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() { //Parent is the parent control spinner //view is the textview filled in the spinner, id = @ Android: ID / text1 //Position is the position of the array of values //id is the position of the row where the value is located, generally consistent with position @Override public void onItemSelected(AdapterView<?> parent, View view, int pos, long id) { LogUtil.i("onItemSelected : parent.id="+parent.getId()+ ",isSpinnerId="+(parent.getId() == R.id.spinner_1)+ ",viewid="+view.getId()+ ",pos="+pos+",id="+id); ToastUtil.showShort(instance,"Chose["+spinnerItems[pos]+"]"); //Center the fill text in the spinner //((TextView)view).setGravity(Gravity.CENTER); } @Override public void onNothingSelected(AdapterView<?> parent) { // Another interface callback } }); }
4. Fully customized spinner: basically, it's all code implementation. The custom adapter binds the list < T > and dropdown layout files, and then the spinner loads the adapter

3-1.png
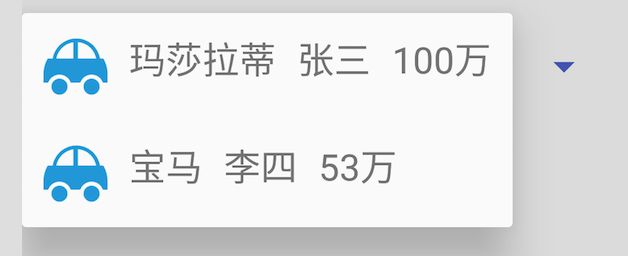
3-2.png
Code:
//CarBean won't post it /** * Spinner Fully custom UI and binding data * */ private void testSpinnerSelf(){ ArrayList<CarBean> cars = new ArrayList<>(); CarBean car = new CarBean(); car.setBrand("Maserati"); car.setOwner("Zhang San"); car.setCost("100 ten thousand"); cars.add(car); car = new CarBean(); car.setBrand("BMW"); car.setOwner("Li Si"); car.setCost("53 ten thousand"); cars.add(car); final CarAdapter adapter = new CarAdapter(instance,cars); mSpinnerSelf.setAdapter(adapter); mSpinnerSelf.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() { @Override public void onItemSelected(AdapterView<?> parent, View view, int pos, long id) { ToastUtil.showShort(instance,((CarBean)adapter.getItem(pos)).toString()); } @Override public void onNothingSelected(AdapterView<?> parent) { } }); }
Code: CarAdapter
public class CarAdapter extends BaseAdapter { private Context ctx; private LayoutInflater li; private ArrayList<CarBean> dataList; public CarAdapter(Context ctx,ArrayList<CarBean> dataList) { this.ctx = ctx; this.li = LayoutInflater.from(ctx); this.dataList = dataList; } @Override public int getCount() { return dataList.size(); } @Override public CarBean getItem(int position) { return dataList.get(position); } @Override public long getItemId(int position) { return position; } @Override public View getView(final int position, View convertView, ViewGroup parent) { if (convertView == null) { convertView = View.inflate(ctx, R.layout.item_car, null); new ViewHolder(convertView); } ViewHolder holder = (ViewHolder) convertView.getTag();// get convertView's holder holder.car_brand.setText(getItem(position).getBrand()); holder.car_owner.setText(getItem(position).getOwner()); holder.car_cost.setText(getItem(position).getCost()); return convertView; } class ViewHolder { TextView car_brand; TextView car_owner; TextView car_cost; public ViewHolder(View convertView){ car_brand = (TextView) convertView.findViewById(R.id.tv_car_brand); car_owner = (TextView) convertView.findViewById(R.id.tv_car_owner); car_cost = (TextView) convertView.findViewById(R.id.tv_car_cost); convertView.setTag(this);//set a viewholder } } }
Layout file: item_car.xml, you will find that this layout is used on the spinner selected result fill control and dropdown control
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:background="?android:attr/selectableItemBackground" android:padding="10dp"> <ImageView android:id="@+id/iv_car" android:layout_width="30dp" android:layout_height="30dp" android:src="@mipmap/car"/> <TextView android:id="@+id/tv_car_brand" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="10dp" android:textSize="@dimen/normal_text_size"/> <TextView android:id="@+id/tv_car_owner" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="10dp" android:textSize="@dimen/normal_text_size"/> <TextView android:id="@+id/tv_car_cost" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="10dp" android:textSize="@dimen/normal_text_size"/> </LinearLayout>
The first one in 2018, it's easy to write. I hope you like it.