Change the status bar background and icon colors
By default, it's white on black. Now it's black on white
First, look at the renderings:
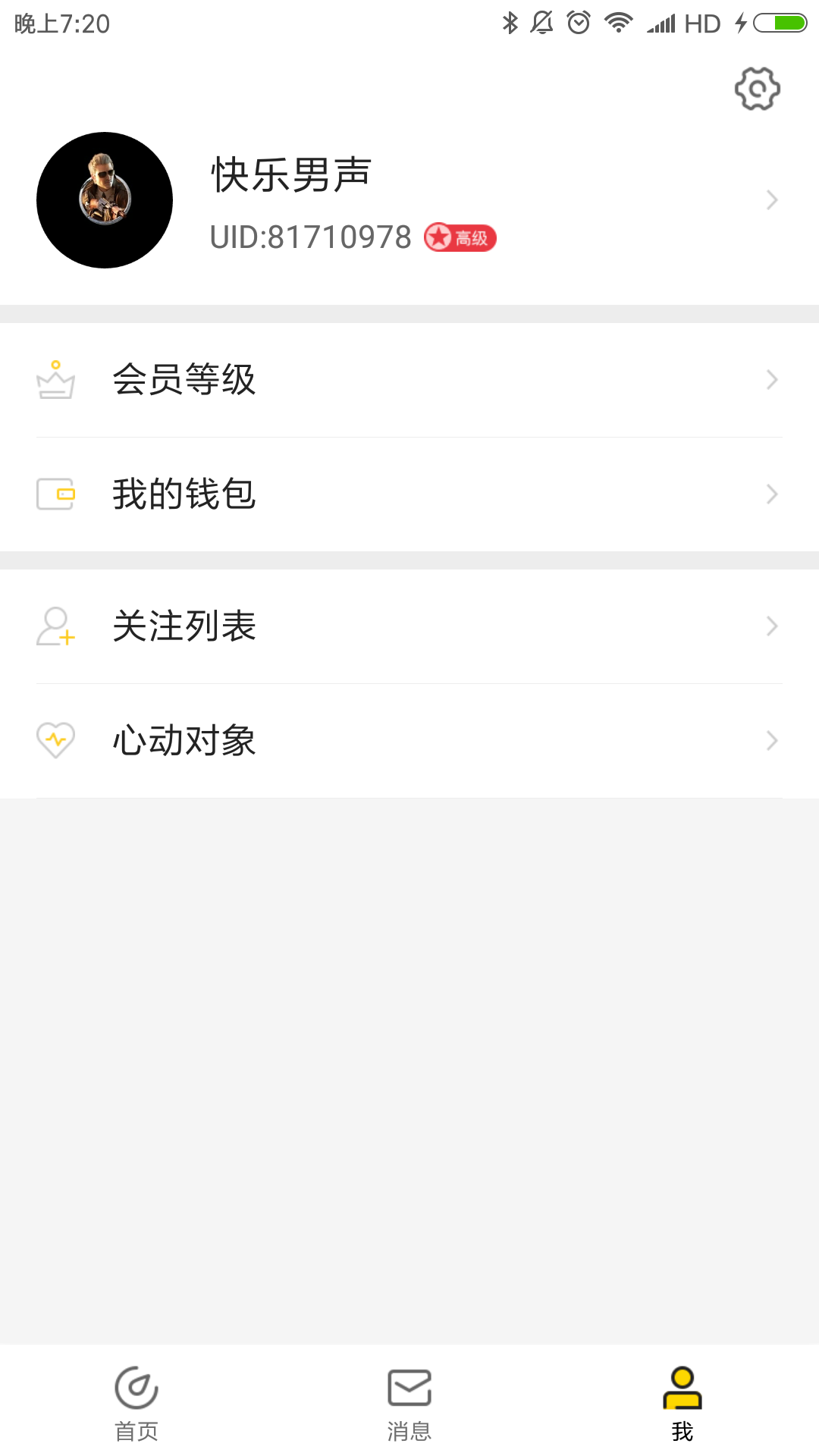
1. Status bar background is white: set in style
<item name="colorPrimaryDark">@color/white</item>
2. Write to modify the color of the status bar icon (only black and white are known for the time being)
public class StatusBarUtil { /** * Change the status bar to fully transparent * @param activity */ @TargetApi(19) public static void transparencyBar(Activity activity){ if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) { Window window = activity.getWindow(); window.clearFlags(WindowManager.LayoutParams.FLAG_TRANSLUCENT_STATUS); window.getDecorView().setSystemUiVisibility(View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN | View.SYSTEM_UI_FLAG_LAYOUT_STABLE); window.addFlags(WindowManager.LayoutParams.FLAG_DRAWS_SYSTEM_BAR_BACKGROUNDS); window.setStatusBarColor(Color.TRANSPARENT); } else if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) { Window window =activity.getWindow(); window.setFlags(WindowManager.LayoutParams.FLAG_TRANSLUCENT_STATUS, WindowManager.LayoutParams.FLAG_TRANSLUCENT_STATUS); } } /** * Modify the color of the status bar to support the version above 4.4 * @param activity * @param colorId */ public static void setStatusBarColor(Activity activity,int colorId) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) { Window window = activity.getWindow(); //window.addFlags(WindowManager.LayoutParams.FLAG_DRAWS_SYSTEM_BAR_BACKGROUNDS); //window.getDecorView().setSystemUiVisibility( View.SYSTEM_UI_FLAG_LIGHT_STATUS_BAR); window.setStatusBarColor(activity.getResources().getColor(colorId)); } else if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) { //To use the SystemBarTint library to change the color of the status bar in version 4.4, you need to set the status bar to transparent first transparencyBar(activity); SystemBarTintManager tintManager = new SystemBarTintManager(activity); tintManager.setStatusBarTintEnabled(true); tintManager.setStatusBarTintResource(colorId); } } /** *Status bar light color mode, setting status bar black text, icon, * Adapts to MIUIV, Flyme and other Android versions above 4.4 and 6.0 * @param activity * @return 1:MIUUI 2:Flyme 3:android6.0 */ public static int statusBarLightMode(Activity activity){ int result=0; if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) { if(MIUISetStatusBarLightMode(activity, true)){ result=1; }else if(FlymeSetStatusBarLightMode(activity.getWindow(), true)){ result=2; }else { activity.getWindow().getDecorView().setSystemUiVisibility( View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN|View.SYSTEM_UI_FLAG_LIGHT_STATUS_BAR); result=3; } } return result; } /** * Set status bar icons to dark and Meizu specific text styles * It can be used to determine whether it is a Flyme user * @param window Windows to set * @param dark Set the status bar text and icon color to dark * @return boolean Successful execution returns true * */ public static boolean FlymeSetStatusBarLightMode(Window window, boolean dark) { boolean result = false; if (window != null) { try { WindowManager.LayoutParams lp = window.getAttributes(); Field darkFlag = WindowManager.LayoutParams.class .getDeclaredField("MEIZU_FLAG_DARK_STATUS_BAR_ICON"); Field meizuFlags = WindowManager.LayoutParams.class .getDeclaredField("meizuFlags"); darkFlag.setAccessible(true); meizuFlags.setAccessible(true); int bit = darkFlag.getInt(null); int value = meizuFlags.getInt(lp); if (dark) { value |= bit; } else { value &= ~bit; } meizuFlags.setInt(lp, value); window.setAttributes(lp); result = true; } catch (Exception e) { } } return result; } /** * Need MIUIV6 or above * @param activity * @param dark Whether to set the status bar text and icon color to dark * @return boolean Successful execution returns true * */ public static boolean MIUISetStatusBarLightMode(Activity activity, boolean dark) { boolean result = false; Window window=activity.getWindow(); if (window != null) { Class clazz = window.getClass(); try { int darkModeFlag = 0; Class layoutParams = Class.forName("android.view.MiuiWindowManager$LayoutParams"); Field field = layoutParams.getField("EXTRA_FLAG_STATUS_BAR_DARK_MODE"); darkModeFlag = field.getInt(layoutParams); Method extraFlagField = clazz.getMethod("setExtraFlags", int.class, int.class); if(dark){ extraFlagField.invoke(window,darkModeFlag,darkModeFlag);//Status bar transparent and black font }else{ extraFlagField.invoke(window, 0, darkModeFlag);//Clear black font } result=true; if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { //Development version 7.7.13 and later uses system API, the old method is invalid but will not report errors, so both methods should be added if(dark){ activity.getWindow().getDecorView().setSystemUiVisibility( View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN| View.SYSTEM_UI_FLAG_LIGHT_STATUS_BAR); }else { activity.getWindow().getDecorView().setSystemUiVisibility(View.SYSTEM_UI_FLAG_VISIBLE); } } }catch (Exception e){ } } return result; }}
The code above is https://www.jianshu.com/p/7f5a9969be53 Go to find it. You can go to see it
3. Specific reference column
In BaseActivity
@Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); ActivityUtils.add(this, getClass()); mContext = this; StatusBarUtil.statusBarLightMode(this); }
4. The normal status bar has changed
The status bar has changed, but you will see that the entire activity layout will move up and fill the screen
Solution 1: add AppTheme in style
<item name="android:fitsSystemWindows">true</item>
If the code layout above is added and moved down, nothing else will be affected. Then you don't have to look down
android:fitsSystemWindows is very poor. There are many problems with the style of pop-up boxes
Solution 2: add paddingTop for each layout
LibUtils:
/** * Get status bar height * @return */ public static int getStatusBarHeight(Context context) { int result = 0; int resourceId = context.getResources().getIdentifier("status_bar_height", "dimen", "android"); if (resourceId > 0) { result = context.getResources().getDimensionPixelSize(resourceId); } return result; } //Set layout distance from status bar height public static void setLayoutPadding(Activity activity, View contentLayout) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) { contentLayout .setPadding(contentLayout.getPaddingLeft(), getStatusBarHeight(activity) + contentLayout.getPaddingTop(), contentLayout.getPaddingRight(), contentLayout.getPaddingBottom()); } }
Reference:
protected void onCreate(@NonNull Bundle savedInstanceState, int resId, int titleId) { super.onCreate(savedInstanceState); mContext = this; setContentView(R.layout.activity_base); StatusBarUtil.statusBarLightMode(this); LibUtils.setLayoutPadding(this,((ViewGroup)findViewById(android.R.id.content)).getChildAt(0));}
Note: after setContentView is called for LibUtils.setLayoutPadding, android.R.id.content is to obtain the root layout of each layout, and Baidu does not understand itself
We also need to consider the android version. In general, the system under 5.0 still uses the default
If you have any questions, please add QQ:893151960, or leave a message