First, the first application of this module
SSH client
Here is a simple code to understand
import paramiko # Instantiate an ssh client object ssh = paramiko.SSHClient() # Automatically add the machine you want to connect to in know_host, ignore this verification, and pay attention to the passed parameter paramiko.AutoAddPolicy() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) # Connection information ssh.connect(hostname='hostadresss', port=22, username='root', password='password') # Transfer command. There are three return values. The first is the standard input, that is, the command you input. The second is the standard output, that is, the command return value # The third standard error will be returned to this value when an error is reported. At the same time, the return value is not fixed, but also an error when it changes stdin, stdout, stderr = ssh.exec_command('pwd') # Read output result = stdout.read() # Printout print(result) # Close client ssh.close()
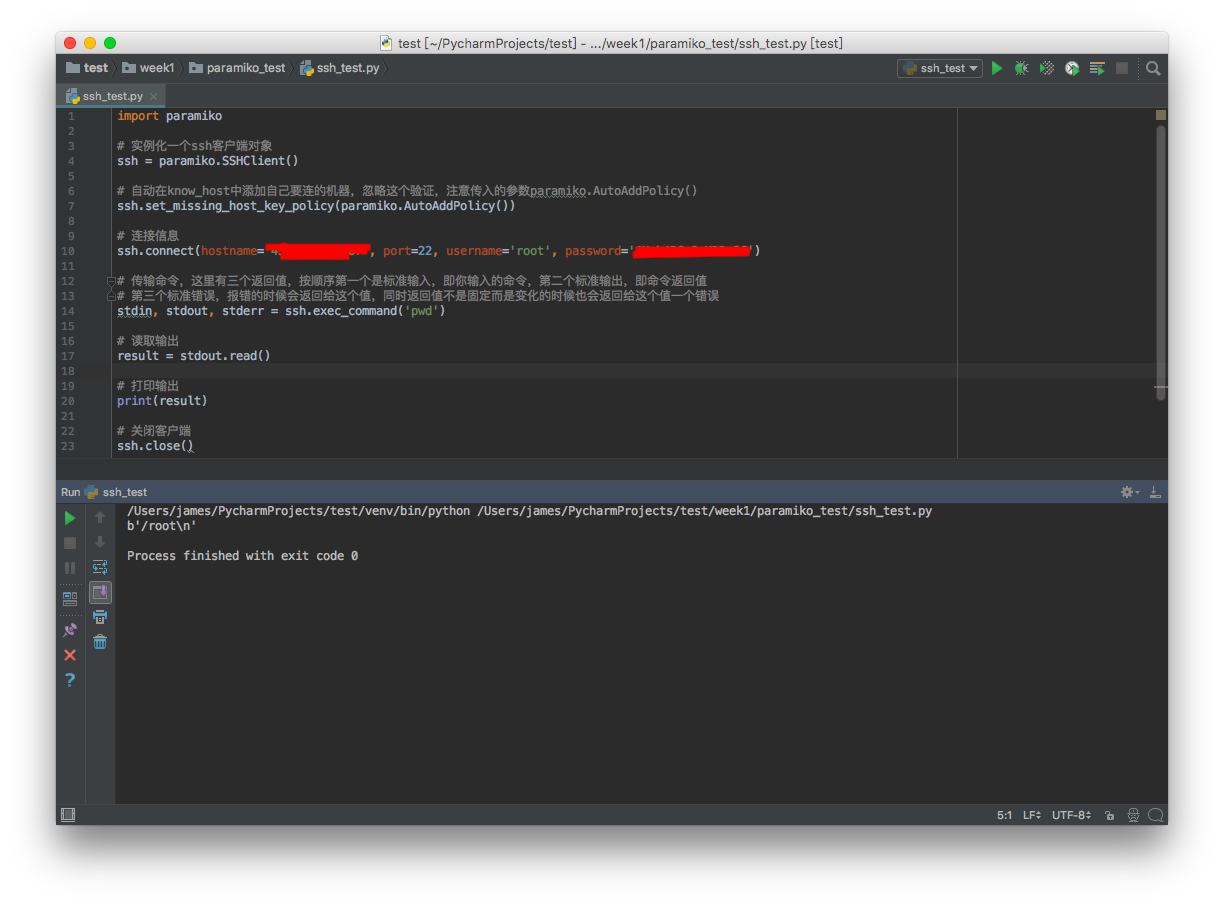
Note that there are three return values when connecting and sending commands
The second version uses the private key to log in (multiple functions)
import paramiko # Declare private key file path private_key = paramiko.RSAKey.from_private_key_file('id_rsa') # Generate ssh client instance ssh = paramiko.SSHClient() # Authentication by public means (does not need to exist in the known hosts file) ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) # Connection server declaration private key ssh.connect(hostname='45.32.131.167', port=22, username='root', pkey=private_key) # Get return value stdin, stdout, stderr = ssh.exec_command('pwd') # read result = stdout.read() # Printing print(result) # Closing instance ssh.close()
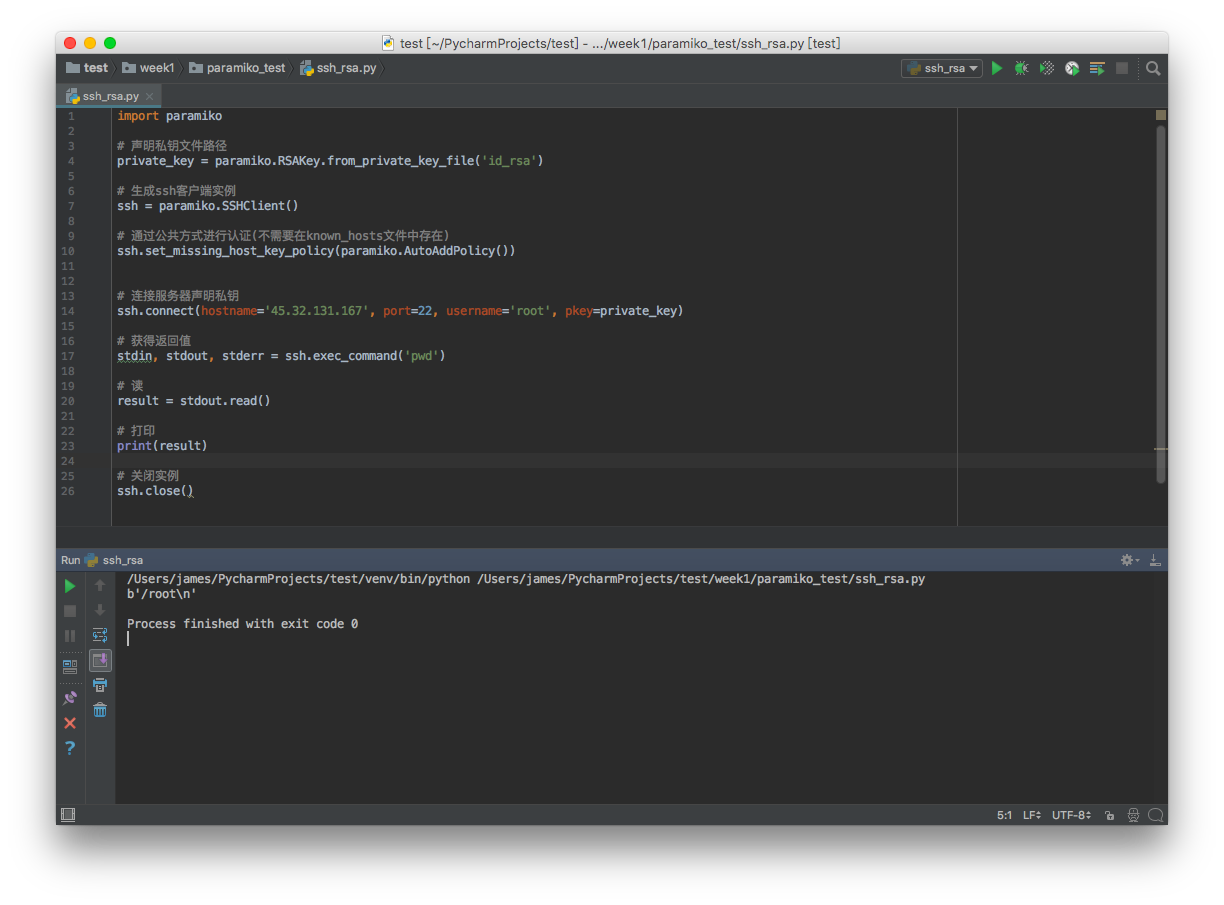
Do not forget to configure the ssh on the server side before use, and allow the key to log in
And then there's the second application
SFTP Client
import paramiko # Generate Transport instance incoming ip and port transport = paramiko.Transport(('hostname', 22)) # Incoming username password connection transport.connect(username='root', password='123') # Generate the SFTPClient instance and pass it to the above connected instance sftp = paramiko.SFTPClient.from_transport(transport) # Upload files sftp.put('put_file_path', 'server_path') # Download File sftp.get('download_file_path', 'local_path') # Closing instance transport.close()
Note that the SFTP instance is not created in one step
Reprint please indicate the source
python self study technology mutual help buckle group: 670402334