introduction
There are two types of loops in Python syntax
- while loop
- for loop
It also introduces the functions of the keywords continue and break in the loop.
Basic structure of program
In program development, there are three basic structures:
- Sequence - execute code sequentially from top to bottom
- Branch / select -- determine the branch to execute the code according to the condition judgment
- Loop -- let specific code execute repeatedly
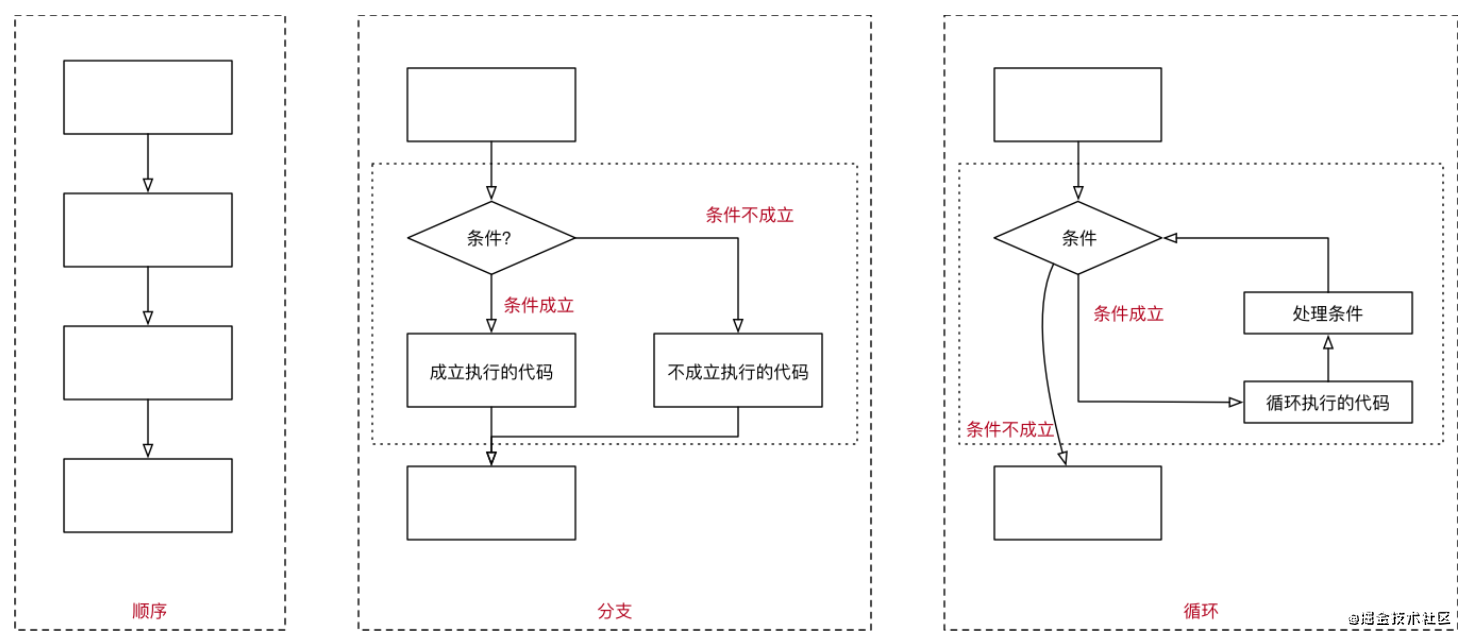
Basic use of while loop
- The function of the loop is to make the specified code execute repeatedly
- The most common application scenario of while loop is to make the executed code execute repeatedly for a specified number of times
Basic syntax of while statement
while Judgment conditions: Loop body statement
Note: the while statement and the indented part are a complete block of code
while loop flowchart
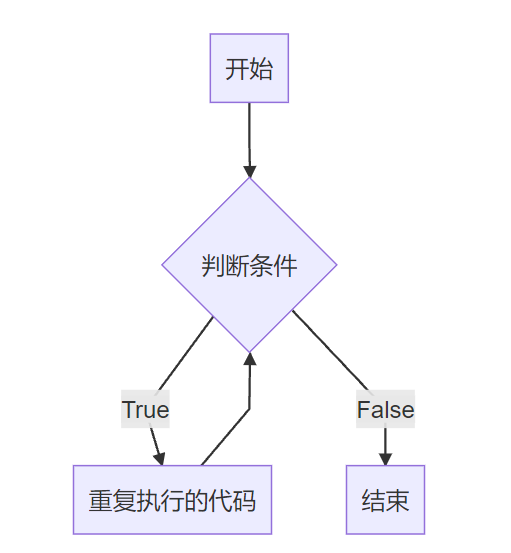
while loop case
Print 5 times Hello Python
In [22]: In [23]: i = 1 # Define repetition counter In [24]: while i <= 5: ...: print('Hello Python') ...: ...: # Processing counter i ...: i = i + 1 ...: Hello Python Hello Python Hello Python Hello Python Hello Python
Print little stars
demand
- Five consecutive lines * are output on the console, and the number of asterisks in each line increases in turn
* ** *** **** *****
- Print using string *
#!/usr/bin/python3 # -*- coding:utf-8 -*- # It is convenient to define a counter variable, starting with the number 1 row = 1 while row <= 5: print("*" * row) row += 1
Counting methods in Python
There are two common counting methods, which can be called:
- Natural counting (starting from 1) - more in line with human habits
- Program counting method (starting from 0) - almost all program languages choose to count from 0
As programmers, we try to form a habit when writing programs: unless the requirements are special, the cycle count starts from 0
while loop nesting
While nesting means that there are still while in while
Basic grammar
while Condition 1: Outer circulatory body ... while Condition 2: Inner circulatory body ... Outer circulatory body ...
Suppose Python does not provide a * operation for string splicing
demand
- Five consecutive lines * are output on the console, and the number of asterisks in each line increases in turn
* ** *** **** *****
Development steps
- 1) Complete a simple output of 5 lines
- 2) How to deal with the * inside each line?
- The number of stars displayed in each row is consistent with the current number of rows
- A small loop is nested to deal with the star display of columns in each row
#!/usr/bin/python3 # -*- coding:utf-8 -*- row = 1 while row <= 5: # Suppose python does not provide a string * operation # Inside the loop, another loop is added to realize the star printing of each line col = 1 while col <= row: print("*", end="") col += 1 # After the asterisk of each line is output, add a new line print() row += 1
print() function
- By default, after the print function outputs the content, a new line is automatically added at the end of the content
- If you don't want to add a newline at the end, you can add it after the output of the print function, end = ""
- Among them, you can specify the content you want to display after the print function outputs the content
- The syntax format is as follows:
# After the output to the console is completed, there is no newline print("*", end="") print("*", end=" ") # Add two spaces at the end without line breaks print("*", end="\t") # Add a tab at the end without line breaks # Simple line feed print()
end = "" in the print() function means that the output to the console will not wrap
Dead cycle
Due to the programmer's reason, he forgot to modify the judgment conditions of the loop inside the loop, resulting in the continuous execution of the loop and the program cannot be terminated!
# Always output hello i = 0 while i <= 10: print('hello') # i = i + 1
In the console, you can press Ctrl + C to forcibly exit the loop.
Basic use of for loop
In Python, the for loop can traverse all iteratable objects, such as a list, string, etc.
Iteratable objects, in [advanced Python] The column has a detailed introduction. If you want to know more about it, you can move to Python iterator see
Basic syntax of for statement
for variable in Iteratable object: Circulatory body
for loop flow chart
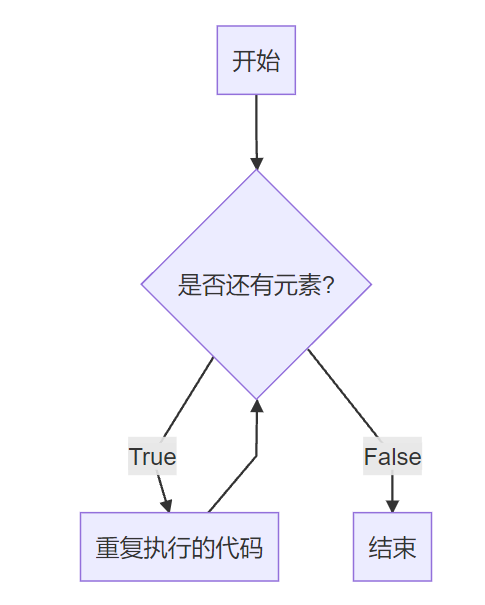
for loop instance
Traversing the list of programming languages
In [1]: languages = ['C', 'Python', 'Java', 'C++', 'Php'] In [2]: for lang in languages: ...: print(lang) ...: C Python Java C++ Php In [3]:
Traversal string
In [3]: message = 'Life is short, I use it Python' In [4]: for msg in message: ...: print(msg) ...: people living bitter short , I use P y t h o n In [5]:
Traverse the sequence of numbers generated by range()
range() syntax
range(start, stop[, step])
Parameter Description:
- Start: count starts from start. The default is to start from 0. For example, range(3) is equivalent to range(0, 3)
- Stop: count to the end of stop, but do not include stop. For example, range(0, 5) is [0, 1, 2, 3, 4] without 5
- Step: step size; the default value is 1. For example, range(0, 3) is equivalent to range(0, 3, 1)
IPython test
In [10]: # Specify start only In [11]: list(range(6)) Out[11]: [0, 1, 2, 3, 4, 5] In [12]: # Both start and stop are specified In [13]: list(range(3, 10)) Out[13]: [3, 4, 5, 6, 7, 8, 9] In [14]: # start, stop and step are all specified In [15]: list(range(0, 10, 2)) Out[15]: [0, 2, 4, 6, 8]
In this way, you need to use list to display its internal elements.
for loop traversal range()
In [16]: # for loop traversal range() In [17]: for i in range(6): ...: print(i) ...: 0 1 2 3 4 5 In [18]: for i in range(3, 9): ...: print(i) ...: 3 4 5 6 7 8 In [19]: for i in range(0, 10, 2): ...: print(i) ...: 0 2 4 6 8 In [20]:
for loop nesting
For nesting is: there is for inside for
Basic grammar
for variable in Iteratable object: Outer circulatory body ... for variable in Iteratable object: Inner circulatory body ... Outer circulatory body ...
Case: seek 1+ 2! + 3! + 4! + 5!, Sum of factorial accumulation between [1,5].
- Factorial of 2!, It's 1 * 2
- Factorial of 3!, It's 1 * 2 * 3
- . . .
The program design is as follows
#!/usr/bin/python3 # -*- coding:utf-8 -*- total = 0 for i in range(1, 6): # Calculate the factorial of i temp = 1 for j in range(1, i+1): temp = temp * j # Each factorial is accumulated total = total + temp print(total) # The result is 153
break and continue
break and continue are special keywords used in loops to interrupt loops.
- break, exit the loop of this layer and do not execute subsequent code
- continue, terminate this cycle, do not execute subsequent codes, and judge the cycle conditions again
break and continue are only valid for the current loop
break
- During a loop, if you no longer want the loop to continue after a certain condition is met, you can use break to exit the loop
i = 0 while i < 10: # When a certain condition is met, exit the loop and do not execute subsequent repeated code # i == 3 if i == 3: break print(i) i += 1 print("over")
break is only valid for the current loop
continue
- During the loop, if you do not want to execute the loop code but do not want to exit the loop after a certain condition is met, you can use continue
- That is, in the whole loop, only some conditions do not need to execute the loop code, while other conditions need to be executed
i = 0 while i < 10: # When i == 7, you do not want to execute code that needs to be executed repeatedly if i == 7: # Before using continue, you should also modify the counter # Otherwise, an endless cycle will occur i += 1 continue # Repeatedly executed code print(i) i += 1
- Note: when you use continue, you need to pay special attention to the code in the condition processing part. If you are not careful, an endless loop will appear
continue is only valid for the current cycle
else syntax for Python loops
Python loop statements for and while may have an else branch. When a for loop is normally executed, or when a while loop is normally executed (the loop condition becomes False), it will be triggered for execution. However, if the loop is abnormally terminated by the break statement, the else branch will not be executed.
while ... else ...
while Cycle condition: Circulatory body ... else: The cycle ends normally
for ... else ...
for variable in Iteratable object: Circulatory body else: The cycle ends normally
IPython test
# for loop In [1]: for i in range(5): ...: print(i) ...: else: ...: print('for The cycle ends normally') ...: print(i) ...: 0 1 2 3 4 for The cycle ends normally 4 # while loop In [2]: num = 1 ...: ...: while num <= 5: ...: print(num) ...: num = num + 1 ...: else: ...: print('while The cycle ends normally') ...: print(num) ...: 1 2 3 4 5 while The cycle ends normally 6 # break interrupt In [3]: for i in range(10): ...: print(i) ...: if i == 5: ...: break ...: else: ...: print('for The cycle ends normally') ...: print(i) ...: 0 1 2 3 4 5
Application scenario
For example, the example of finding prime numbers in Python official documents - printing prime numbers within 10
for n in range(2, 10): for x in range(2, n): if n % x == 0: print(n, 'equals', x, '*', n//x) break else: # loop fell through without finding a factor print(n, 'is a prime number')
The operation results are as follows:
2 is a prime number 3 is a prime number 4 equals 2 * 2 5 is a prime number 6 equals 2 * 3 7 is a prime number 8 equals 2 * 4 9 equals 3 * 3
Small case of circular actual combat
Cumulative summation results of all even numbers between [0, 100]
# 0. Used to count the final results result = 0 # 1. Counter i = 0 # 2. Start cycle while i <= 100: # Judge even number if i % 2 == 0: result += i # Accumulate i += 1 print(result) # Result: 2550
Print isosceles triangles
demand
- Print isosceles triangles of n layers
- Print using string *
- The number of * in each layer increases in the order of 1, 3, 5, 7 and 9, and forms an isosceles triangle
For example, the isosceles triangle of 5 layers is shown as follows:
* *** ***** ******* *********
The program design is as follows
#!/usr/bin/python3 # -*- coding: utf-8 -*- while True: level = input('Please enter the number of layers to print isosceles triangles(input q sign out): ') if level == 'q': break # Convert to int integer level = int(level) row = 1 # Level counter while row <= level: # Calculate the number of spaces per layer space_count = level - row print(' ' * space_count, end='') # Print spaces without wrapping # Calculate the number of * per layer char_count = row * 2 - 1 print('*' * char_count) # Print * for each layer and wrap # Level count plus 1 row = row + 1
The operation results are as follows:
Please enter the number of layers to print isosceles triangles(input q sign out): 3 * *** ***** Please enter the number of layers to print isosceles triangles(input q sign out): 5 * *** ***** ******* ********* Please enter the number of layers to print isosceles triangles(input q sign out): 7 * *** ***** ******* ********* *********** ************* Please enter the number of layers to print isosceles triangles(input q sign out): q Process finished with exit code 0
Print 99 multiplication formula table
for i in range(1, 10): for j in range(1, i + 1): # print(f'{j} * {i} = {j * i}', end='\t') print('%d * %d = %d' % (j, i, j * i), end='\t') # Each row is separated by tab s print()
This uses the formatted output of the string, where
- f'{j} * {i} = {j * i}', add f before the string, which is the template string. In the string, {xxx} can be directly used to reference variables or perform corresponding operations.
- '% d *% d =% d'% (J, I, J * I) ', is the format string,% d represents the format integer, and the data after% d will fill the placeholder of% d in turn.
Here is only a preliminary introduction, which will be introduced in detail in the subsequent string explanation.
The operation results are as follows:
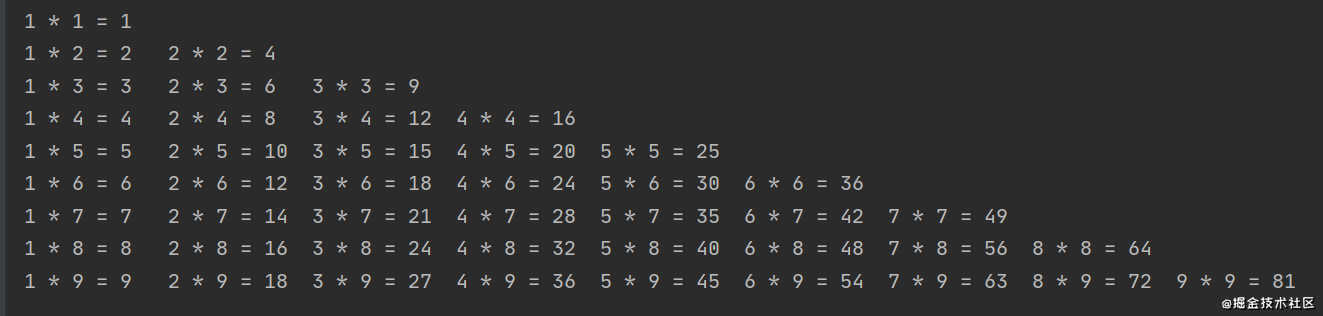