This demo calls back to Hell:
Simulate the movie website to determine whether the user is the VIP user of the website (the maximum permission is vip)
If the vpi shows the vip movie, click on the corresponding movie to show the details of the movie.
------------------------------------------------------------------
Let's first look at the finished drawings.
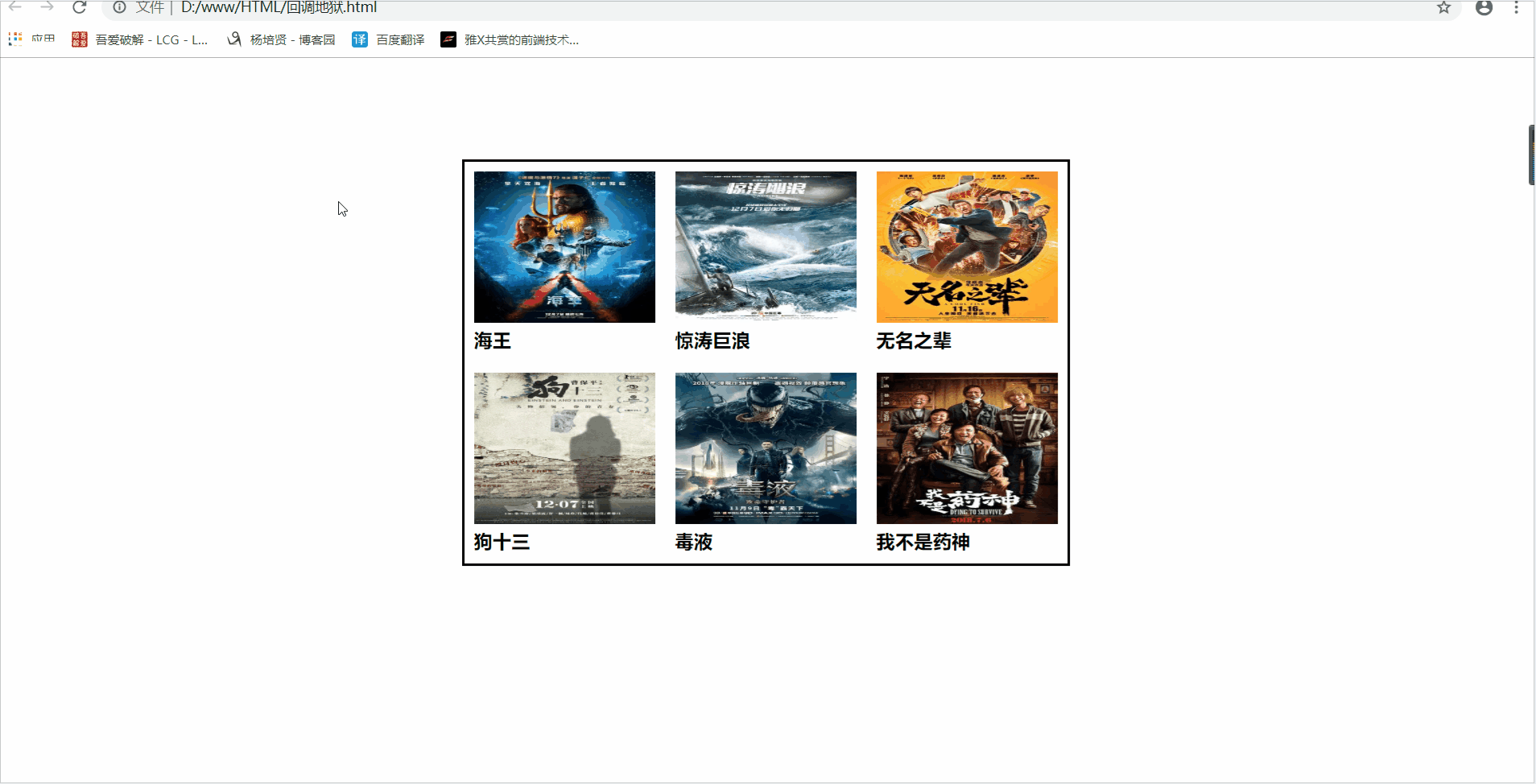
Firstly, the HTML+CSS structure is built. The code is as follows:
1 <style> 2 *{ 3 padding: 0px; 4 margin:0px; 5 } 6 .tpl{ 7 display: none; 8 } 9 .wrapper{ 10 overflow: hidden; 11 border:2px solid black; 12 width:600px; 13 margin:100px auto 0px; 14 } 15 .movieSection{ 16 float:left; 17 width:180px; 18 height:180px; 19 padding:10px; 20 } 21 .movieSection img{ 22 width:100%; 23 height:150px; 24 cursor:pointer; 25 } 26 .movieSection h3{ 27 height:30px; 28 } 29 </style> 30 </head> 31 <body> 32 <div class="wrapper"> 33 <div class="tpl"> 34 <img src=""> 35 <h3 class="movieName"></h3> 36 </div> 37 </div>
The first time a network request is sent to obtain data, verifying that it is a vip user.
root is the highest permission, expressed as vip user.
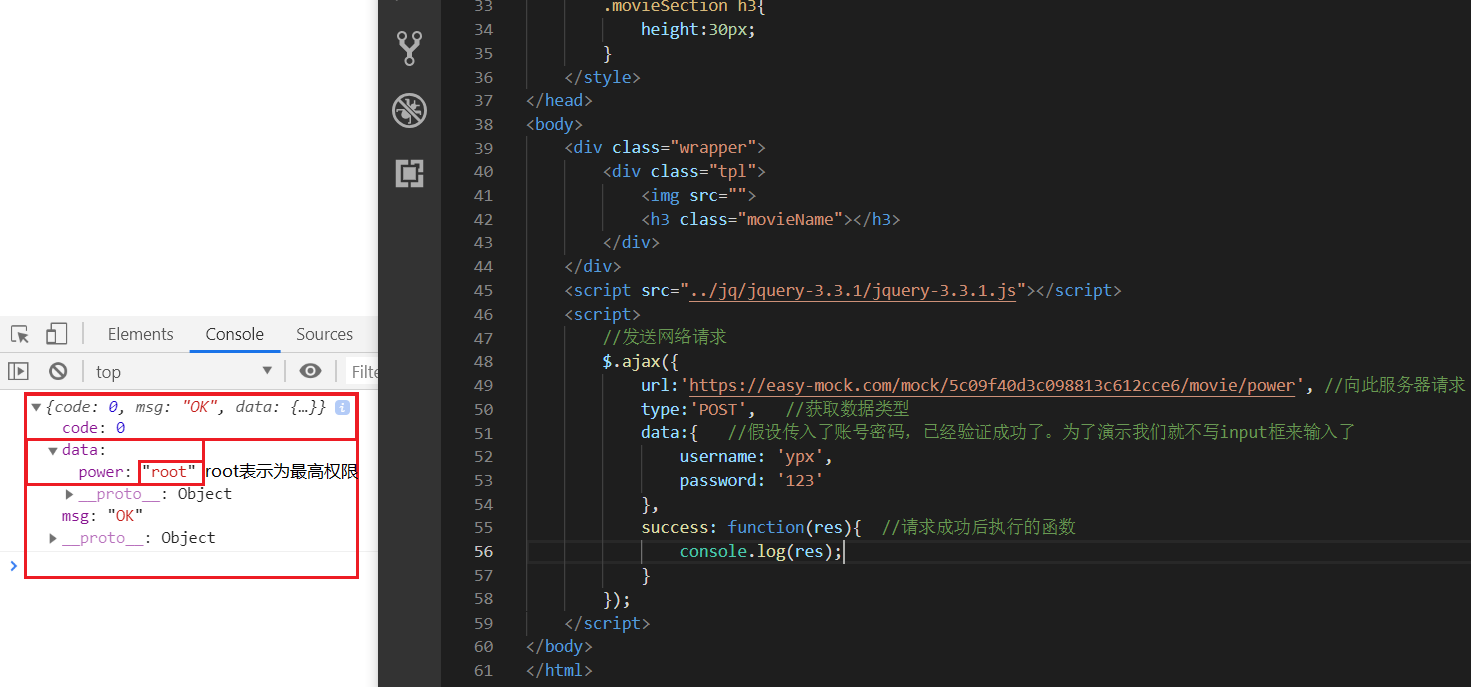
If it is a vip user, it returns the vip movie resource
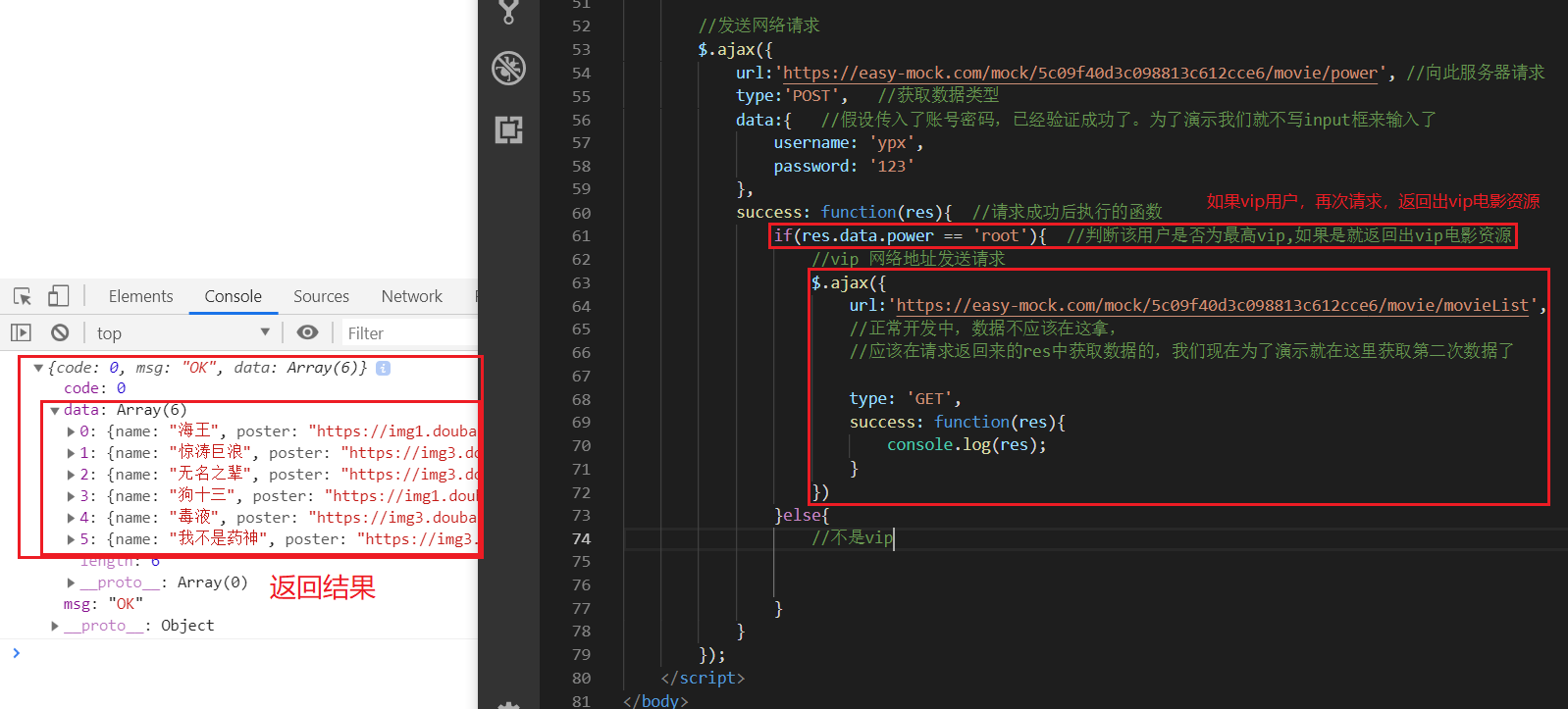
Now that we've got the data, let's visualize it.
Send a network request to get data, the request succeeds, and then render the page
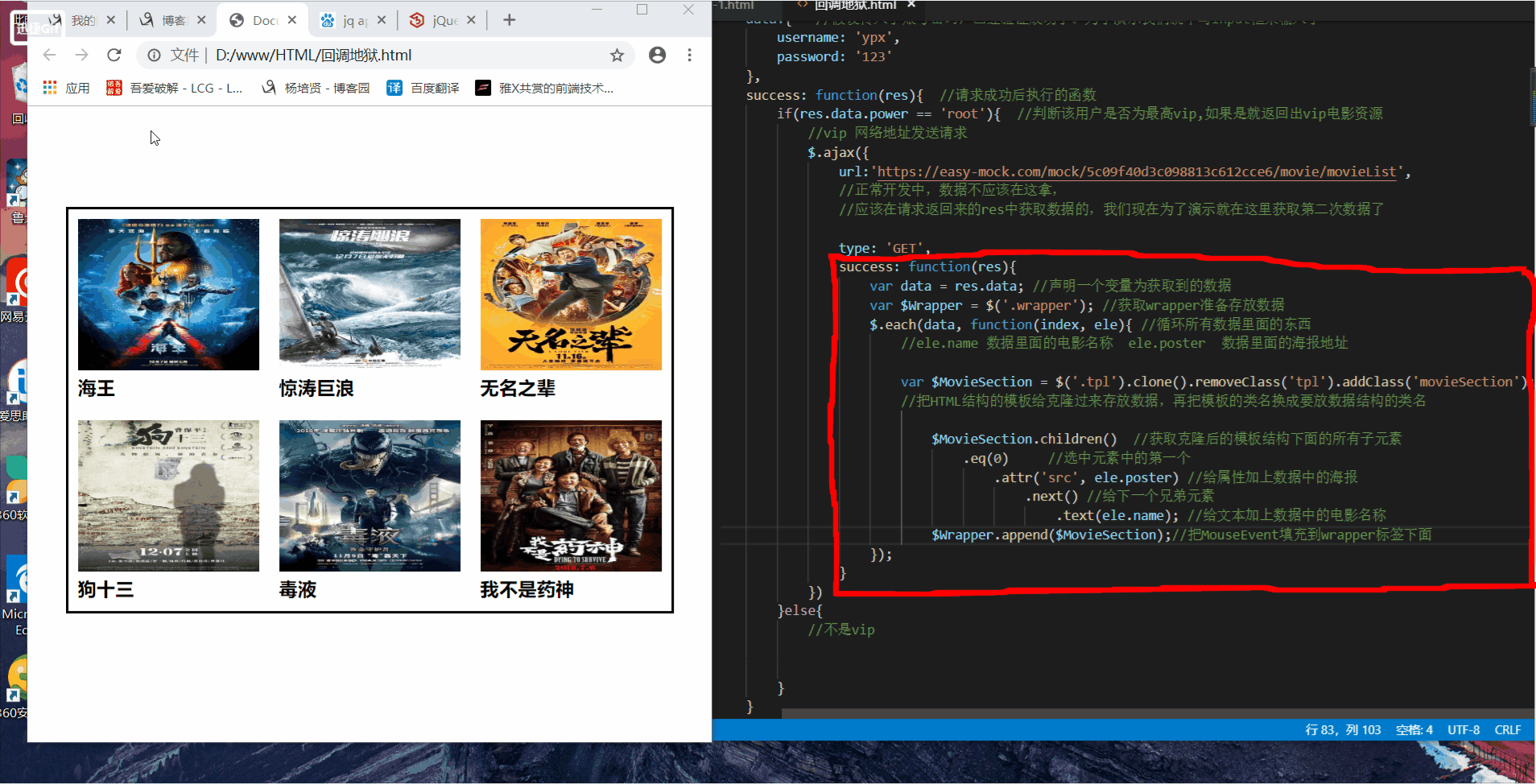
Although we have successfully obtained the data now, we have not let the server know which movie we need, so we need to send the ID of the clicked movie to the server, because the ID is only one.
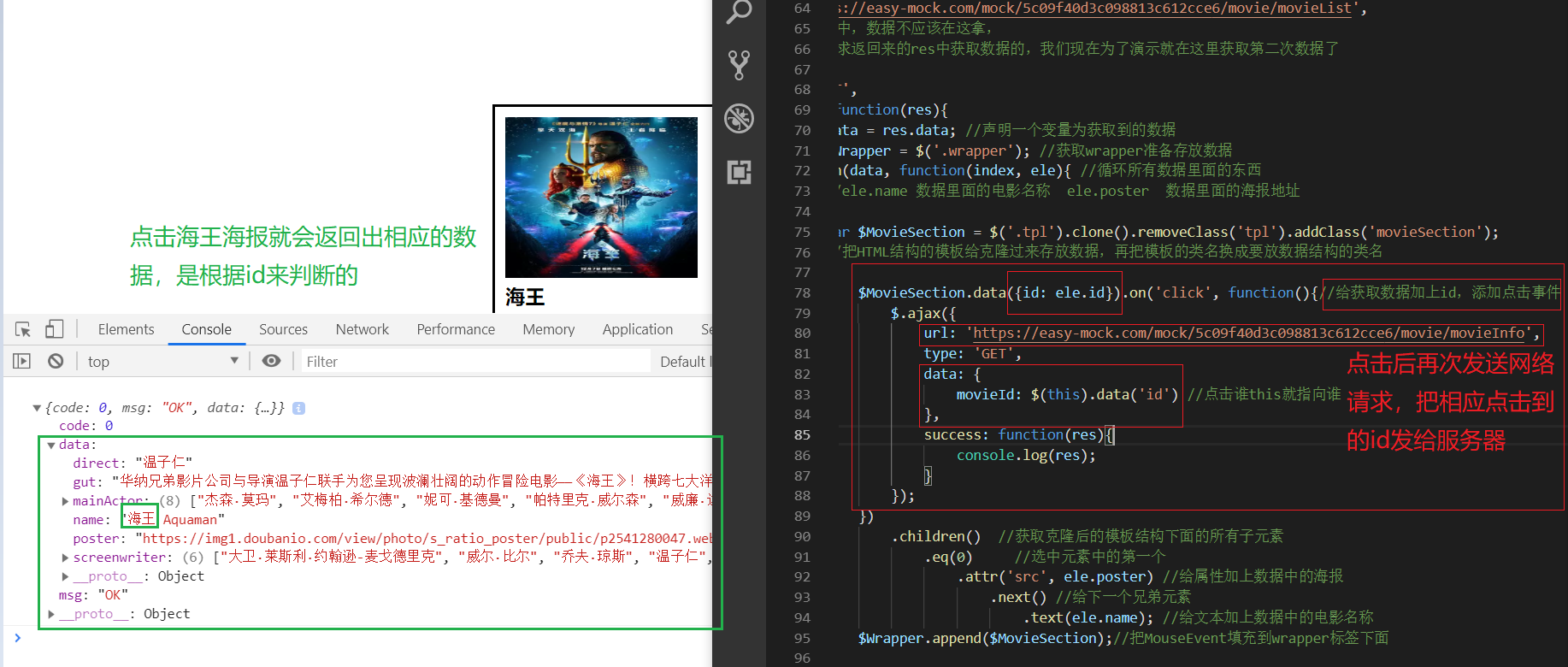
Next, let's do a click to show the details of the movie.
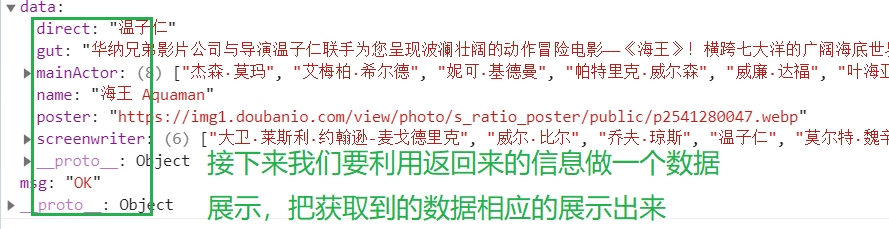
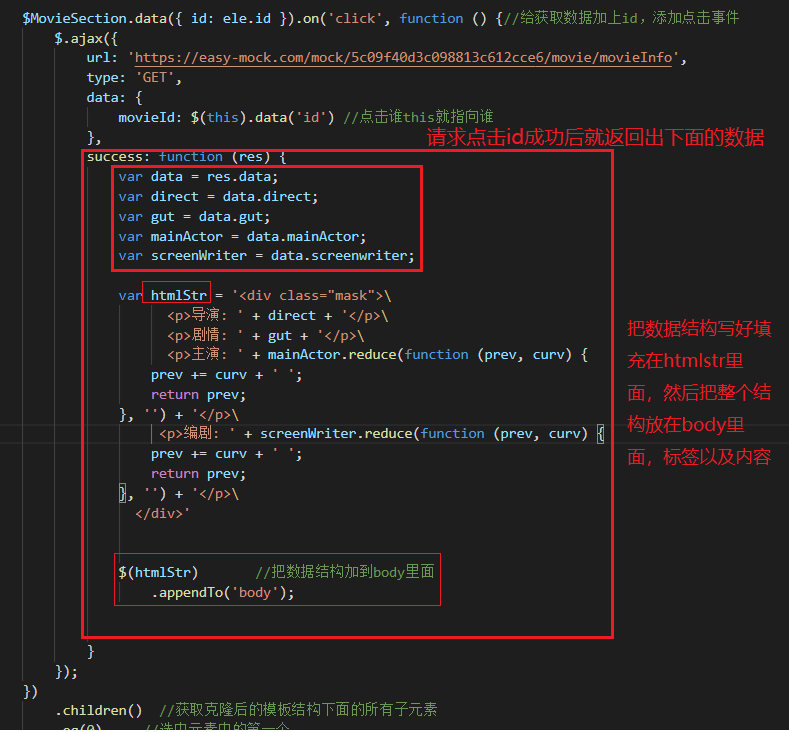
Then visualize the acquired data.
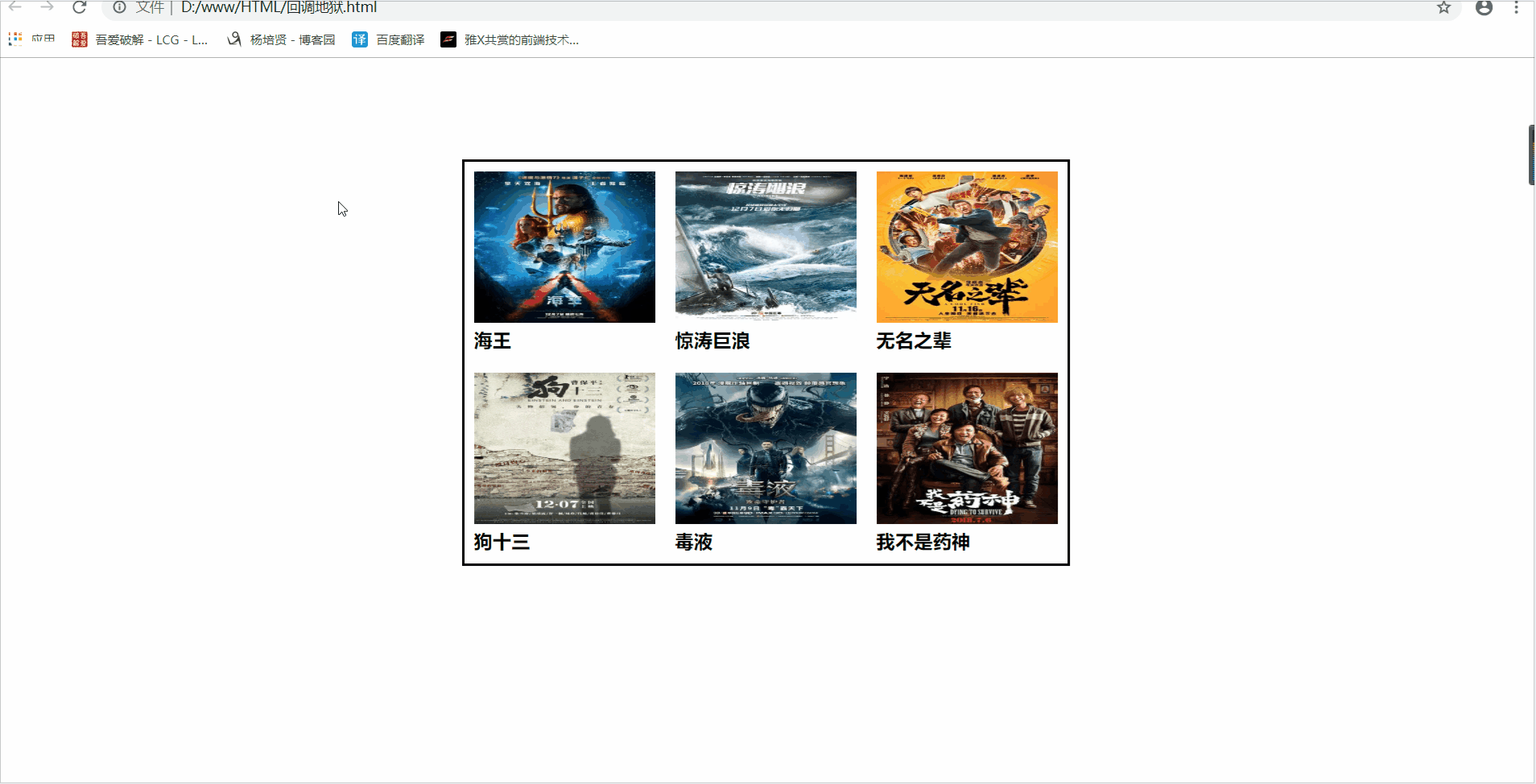
Finally, complete code is attached:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <style> * { padding: 0px; margin: 0px; } .tpl { display: none; } .wrapper { overflow: hidden; border: 2px solid black; width: 600px; margin: 100px auto 0px; } .movieSection { float: left; width: 180px; height: 180px; padding: 10px; } .movieSection img { width: 100%; height: 150px; cursor: pointer; } .movieSection h3 { height: 30px; } </style> </head> <body> <div class="wrapper"> <div class="tpl"> <img></img> <h3 class="movieName"></h3> </div> </div> <script src="../jq/jquery-3.3.1/jquery-3.3.1.js"></script> <script> //Simulate the movie website to determine whether the user is the website vip user(The maximum permission is vip) //If vpi Show off vip Movie, click on the corresponding movie to show the details of the film. //Sending network requests $.ajax({ url: 'https://easy-mock.com/mock/5c09f40d3c098813c612cce6/movie/power', //Request from this server type: 'POST', //Get the data type data: { //Assuming that an account password has been passed in, it has been verified successfully. We won't write it for demonstration. input Box to enter username: 'ypx', password: '123' }, success: function (res) { //Functions executed after successful request if (res.data.power == 'root') { //Determine whether the user is the highest vip,If so, go back. vip Film resources //vip Network Address Sending Request $.ajax({ url: 'https://easy-mock.com/mock/5c09f40d3c098813c612cce6/movie/movieList', //In normal development, data should not be taken here. //Should be returned on request res In order to demonstrate that we are here to get the second data. type: 'GET', success: function (res) { var data = res.data; //Declare a variable as the acquired data var $Wrapper = $('.wrapper'); //Obtain wrapper Prepare to store data $.each(data, function (index, ele) { //Loop everything in the data. //ele.name Film Names in Data ele.poster Poster address in data var $MovieSection = $('.tpl').clone().removeClass('tpl').addClass('movieSection'); //hold HTML The template of the structure is cloned to store the data, and the class name of the template is replaced by the class name of the data structure. $MovieSection.data({ id: ele.id }).on('click', function () {//Add data to the acquisition id,Add Click Events $.ajax({ url: 'https://easy-mock.com/mock/5c09f40d3c098813c612cce6/movie/movieInfo', type: 'GET', data: { movieId: $(this).data('id') //Click who this Pointing to who }, success: function (res) { var data = res.data; var direct = data.direct; var gut = data.gut; var mainActor = data.mainActor; var screenWriter = data.screenwriter; var htmlStr = '<div class="mask">\ <p>director: ' + direct + '</p>\ <p>Plot: ' + gut + '</p>\ <p>To star: ' + mainActor.reduce(function (prev, curv) { prev += curv + ' '; return prev; }, '') + '</p>\ <p>Screenwriter: ' + screenWriter.reduce(function (prev, curv) { prev += curv + ' '; return prev; }, '') + '</p>\ </div>' $(htmlStr) //Add data structures to body inside .appendTo('body'); } }); }) .children() //Get all the sub-elements under the cloned template structure .eq(0) //The first of the selected elements .attr('src', ele.poster) //Posters that add data to attributes .next() //Give the next sibling element .text(ele.name); //Add the name of the movie in the data to the text $Wrapper.append($MovieSection);//hold MouseEvent Fill to wrapper Under the label }); } }) } else { //No vip } } }); </script> </body> </html>
Callback to Hell: Not reusable. Poor reading also means poor maintainability. The scalability is also poor. To solve this problem, please move on to the next article, the tool method for jQuery notes - Ajax $.Deferrnd
Callback to Hell: Not reusable. Poor reading also means poor maintainability. The scalability is also poor. To solve this problem, please move on to the next article, the tool method for jQuery notes - Ajax $.Deferrnd
Callback to Hell: Not reusable. Poor reading also means poor maintainability. The scalability is also poor. To solve this problem, please move on to the next article, the tool method for jQuery notes - Ajax $.Deferrnd