Now many vue projects are developed based on ES6, and most of my learning vue is based on the API of vue official website, which is based on ES5, so it is a challenge for me to change to the modular writing of the project. Therefore, I intend to make a vue demo based on ES5 first, and then write this demo based on ES6, which is a transition bar! This demo has some code borrowed from the keepfool God "Vue.js - 60 Minutes Quick Start for vue-router" Explain this first, respect the originality! It is suggested that the knowledge of vue-rouer can be learned by linking.
I. Project Effect Map

2. Coding process
1. Function I
Is there any problem with this code? Crap<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Demo</title> <link rel="stylesheet" href="bootstrap.min.css"> </head> <body> <div id="app"> <div class="container"> <div class="jumbotron"> <h1>A Demo!</h1> <p>Let's play a demo</p> <p><a class="btn btn-primary btn-lg" href="#" role="button" v-on:click="show">play</a></p> </div> </div> <div class="container" v-show="isShow"><!--isShow by true It shows that, in fact, it is control. display Value--> <div class="row"> <div class="col-md-2 col-md-offset-2"> <div class="list-group"> <a class="list-group-item" v-link="{path:'/home'}">Home</a><!--stay a Use of elements v-link Instruction jump to specified path--> <a class="list-group-item" v-link="{path:'/about'}">About</a> </div> </div> <div class="col-md-6"> <div class="panel"> <div class="panel-body"> <router-view></router-view><!--Render matching components--> </div> </div> </div> </div> </div> </div> <template id="home"> <div> <h1>Home</h1> <p>{{msg1}}</p> </div> </template> <template id="about"> <div> <h1>About</h1> <p>{{msg2}}</p> </div> </template> </body> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script src="bootstrap.min.js"></script> <script src="vue.min.js"></script><!--Version 2.4.0--> <script src="vue-router.js"></script><!--Version 2.7.0--> <script type="text/javascript"> var vm=new Vue({ el:'#app', data:{ isShow: false }, methods:{ show:function(){ this.isShow=true; } } }) var Home=Vue.extend({ //Create Components template:'#home', data:function(){ return{ msg1:'This is Home page!' } } }) var About=Vue.extend({ template:'#about', data:function(){ return{ msg2:'This is About page!' } } }) var router=new VueRouter(); //Establish router router.map({ //Mapping Routing '/home':{ component:Home }, '/about':{ component:About } }) var App=Vue.extend({}) //Start Routing router.start(App,'#app') </script> </html>

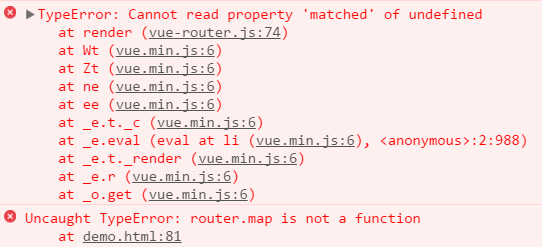
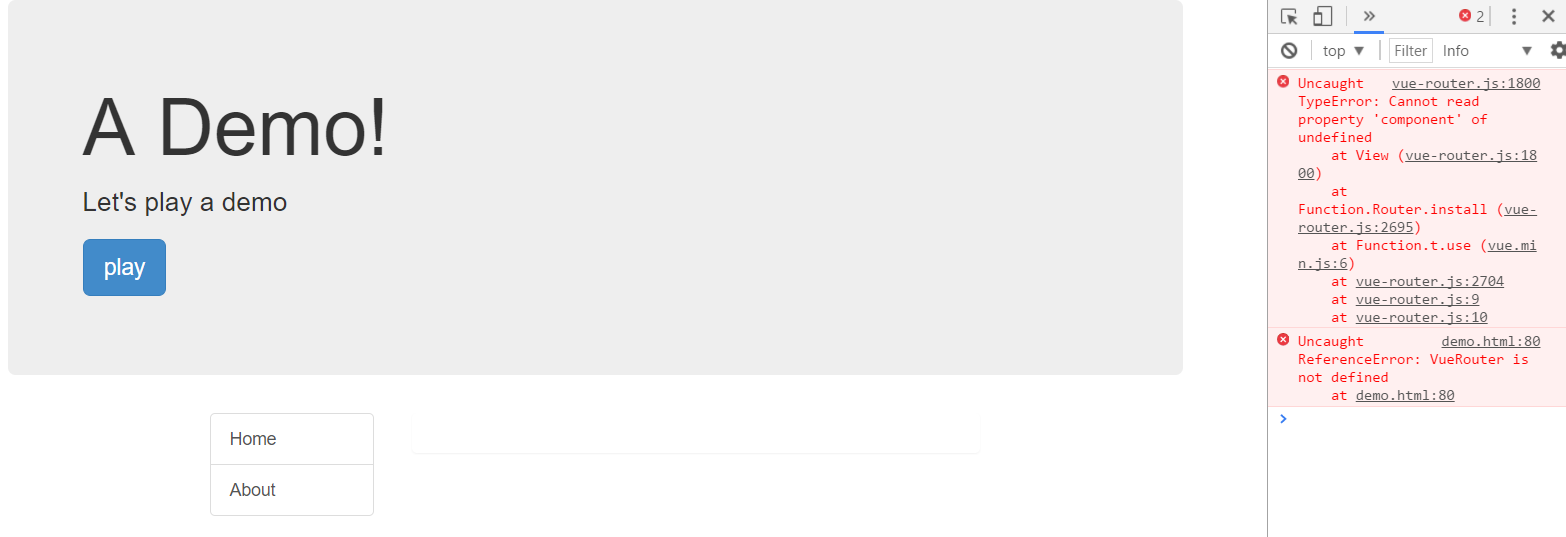


2. Full Routing Implementation Function 1
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Demo</title> <link rel="stylesheet" href="bootstrap.min.css"> </head> <body> <div id="app"> <div class="container"> <div class="jumbotron"> <h1>A Demo!</h1> <p>Let's play a demo</p> <p><a class="btn btn-primary btn-lg" href="#" role="button" v-link="{path:'/play'}">play</a></p> </div> </div> <router-view></router-view> </div> <template id="play"> <div class="container"> <div class="row"> <div class="col-md-2 col-md-offset-2"> <div class="list-group"> <a class="list-group-item" v-link="{path:'/play/home'}">Home</a> <a class="list-group-item" v-link="{path:'/play/about'}">About</a> </div> </div> <div class="col-md-6"> <div class="panel"> <div class="panel-body"> <router-view></router-view> </div> </div> </div> </div> </div> </template> <template id="home"> <div> <h1>Home</h1> <p>{{msg1}}</p> </div> </template> <template id="about"> <div> <h1>About</h1> <p>{{msg2}}</p> </div> </template> </body> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script src="bootstrap.min.js"></script> <script src="vue.min.js"></script> <script src="vue-router.js"></script> <script type="text/javascript"> var Play=Vue.extend({ template:'#play' }) var Home=Vue.extend({ template:'#home', data:function(){ return{ msg1:'This is Home page!' } } }) var About=Vue.extend({ template:'#about', data:function(){ return{ msg2:'This is About page!' } } }) var router=new VueRouter(); router.map({ '/play':{ component:Play, subRoutes: {//Define subrouting '/home':{ component:Home }, '/about':{ component:About } } } }) var App=Vue.extend({}) router.start(App,'#app') </script> </html>
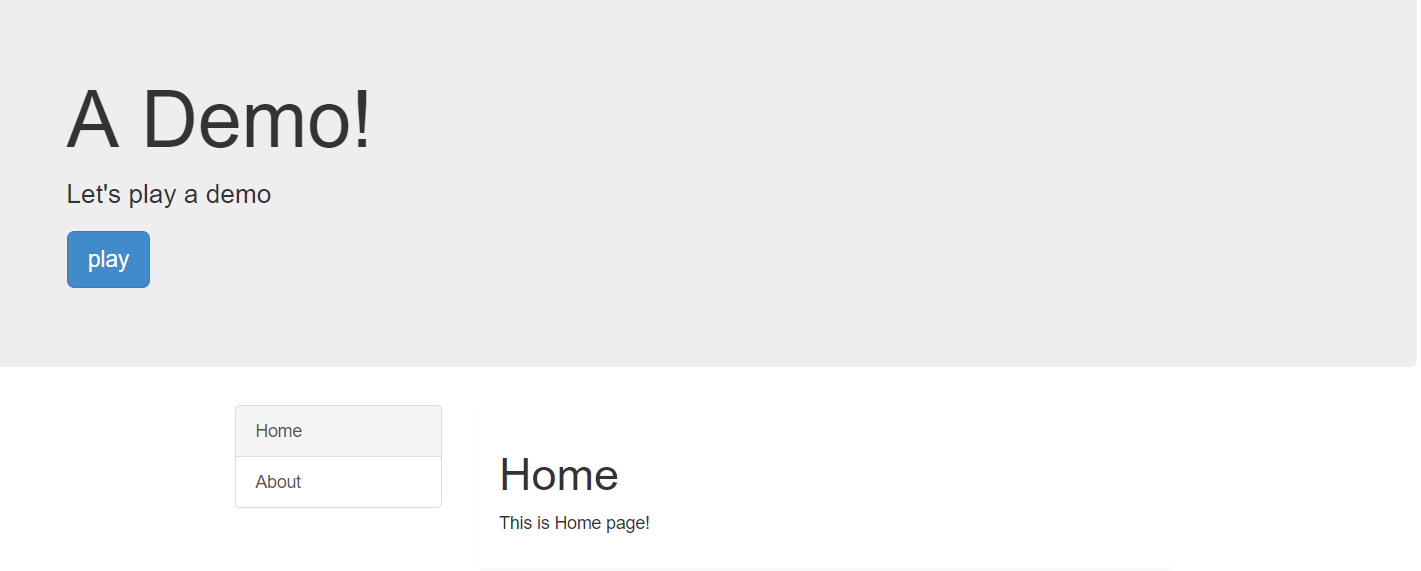
3. Functional Advancement
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Demo</title> <link rel="stylesheet" href="bootstrap.min.css"> </head> <body> <div id="app"> <div class="container"> <div class="jumbotron"> <h1>A Demo!</h1> <p>Let's play a demo</p> <p><a class="btn btn-primary btn-lg" href="#" role="button" v-link="{path:'/play'}">play</a></p> </div> </div> <router-view></router-view> </div> <template id="play"> <div class="container"> <div class="row"> <div class="col-md-2 col-md-offset-2"> <div class="list-group"> <a class="list-group-item" v-link="{path:'/play/home'}">Home</a> <a class="list-group-item" v-link="{path:'/play/about'}">About</a> </div> </div> <div class="col-md-6"> <div class="panel"> <div class="panel-body"> <router-view></router-view> </div> </div> </div> </div> </div> </template> <template id="home"> <div> <h1>Home</h1> <p>{{msg1}}<a class="btn" v-link="{path:'/play/home/time'}">Get the current date</a></p> </div> <router-view></router-view> </template> <template id="time"> <table class="table table-striped"> <tr> <td>Particular year</td> <td>Month</td> <td>day</td> </tr> <tr v-for="t in dates"> <td>{{t.year}}</td> <td>{{t.month}}</td> <td>{{t.day}}</td> </tr> </table> </template> <template id="about"> <div> <h1>About</h1> <p>{{msg2}}</p> </div> </template> </body> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script src="bootstrap.min.js"></script> <script src="vue.min.js"></script> <script src="vue-router.js"></script> <script type="text/javascript"> var Play=Vue.extend({ template:'#play' }) var Home=Vue.extend({ template:'#home', data:function(){ return{ msg1:'This is Home page!' } } }) var Time=Vue.extend({ template:'#time', data:function(){ var D = new Date(); return{ dates:[{ year:D.getFullYear(), month:D.getMonth()+1, day:D.getDate() }] } } }) var About=Vue.extend({ template:'#about', data:function(){ return{ msg2:'This is About page!' } } }) var router=new VueRouter(); router.map({ '/play':{ component:Play, subRoutes: { '/home':{ component:Home, subRoutes:{ '/time':{ component:Time } } }, '/about':{ component:About } } } }) var App=Vue.extend({}) router.start(App,'#app') </script> </html>
