1. Description: upload the attachment in a web project.
The jar package used in demo is commons-fileupload-1.3.3.jar commons-httpclient-3.1.jar commons-io-2.0.1.jar.
It can be obtained in the source code. Download address: http://download.csdn.net/download/shenju 2011/10215828
2. Code interpretation
(1) The jsp interface is processed with a form. Set the enctype = "multipart / form data" method="post" of the form;
The input type of the attachment loading input is file, another input type=submit is used to submit, and then a < textarea > is used to store the return results uploaded by the background attachment. The code is as follows:
(2) , add configuration in web.xml<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>Insert title here</title> </head> <body> <% String retJson = request.getAttribute("retJson")==null ? "" :request.getAttribute("retJson").toString(); %> <form method="post" action="/tcFact/TestServlet" ENCTYPE="multipart/form-data" id="form1"> <div> <br /> <br /> <input type="file" name= "url" id="url" size ="38"> <br /> <br /> <input type="submit" name="BtnSend" value="upload" id="BtnSend" /> <br /> <br /> Interface return <br /> <textarea name="retJson" id="retJson" style="width:100%;height:300px;"><%=retJson%></textarea> </div> </form> </body> </html>
(3) , java background. Here use filePath = "" + this.getClass().getResource(""); to get the address of the current environment.<servlet> <display-name> TestServlet</display-name> <servlet-name>TestServlet</servlet-name> <servlet-class> com.tcFact.action.TestServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>TestServlet</servlet-name> <url-pattern>/TestServlet</url-pattern> </servlet-mapping>
Note: the class file of the packed program. war will be in the.. / WEB-INF / directory, so the "WEB-INF" will be intercepted here. Then the current location is the root directory of the project. Here we explain that the project is published on tomcat or webSphere, which is different from the address obtained directly by this.getClass().getResource("") in eclipse with main Run.
Note: the obtained address must be filePath = URLDecoder.decode(filePath,"UTF-8"); Deal with it. Otherwise, if there is a space in your address, hum... If you try, you will fail.
(4) . upload implementationpackage com.tcFact.action; import java.io.IOException; import java.net.URLDecoder; import java.util.ArrayList; import java.util.Iterator; import java.util.List; import javax.servlet.RequestDispatcher; import javax.servlet.Servlet; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.apache.commons.fileupload.FileItem; import org.apache.commons.fileupload.FileUploadException; import org.apache.commons.fileupload.disk.DiskFileItemFactory; import org.apache.commons.fileupload.servlet.ServletFileUpload; /** * File copy * @author sj-homePc * */ public class TestServlet extends HttpServlet implements Servlet{ public TestServlet() { super(); } protected void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException { // TODO Auto-generated method stub doPost(req,res); } protected void doPost(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException { req.setCharacterEncoding("utf-8"); DiskFileItemFactory factory = new DiskFileItemFactory(); ServletFileUpload fileUpload = new ServletFileUpload(factory); //Set the upper limit of upload file size, - 1 means no upper limit fileUpload.setSizeMax(-1); List items = new ArrayList(); //Upload files, parse file fields and common fields contained in the form try { items = fileUpload.parseRequest(req); } catch (FileUploadException e) { // TODO Auto-generated catch block e.printStackTrace(); } //Traverse fields for processing Iterator iterator = items.iterator(); while(iterator.hasNext()){ FileItem fileItem =(FileItem)iterator.next(); if(!fileItem.isFormField()){//Not a normal field (file) if("url".equals(fileItem.getFieldName())){ //Upload; String filePath=null; String filename = fileItem.getName(); filePath = "" + this.getClass().getResource(""); int strPos = filePath.indexOf("WEB-INF"); filePath = filePath.substring(5,strPos); filePath = filePath.replace("/", "//") ; filePath = filePath + "" + filename; filePath = URLDecoder.decode(filePath,"UTF-8"); //create new object Upload upload = new Upload(); upload.doCover(fileItem,filePath); req.setAttribute("retJson", "Operation succeeded, please check"+filePath+"Directory exists"+filename+"If the file exists, it will be copied successfully!"); RequestDispatcher requestDispatcher=req.getRequestDispatcher("index.jsp"); requestDispatcher.forward(req,res); } } } } }
(4) Test interfacepackage com.tcFact.action; import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import org.apache.commons.fileupload.FileItem; public class Upload { public void doCover(FileItem fileItem, String out) { OutputStream outputStream = null; InputStream inputStream = null; try { outputStream = new FileOutputStream(out); inputStream = fileItem.getInputStream(); byte[] bytes = new byte[1024]; int num = 0; while ((num = inputStream.read(bytes)) != -1) { outputStream.write(bytes, 0, num); outputStream.flush(); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally { try { outputStream.close(); inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } }
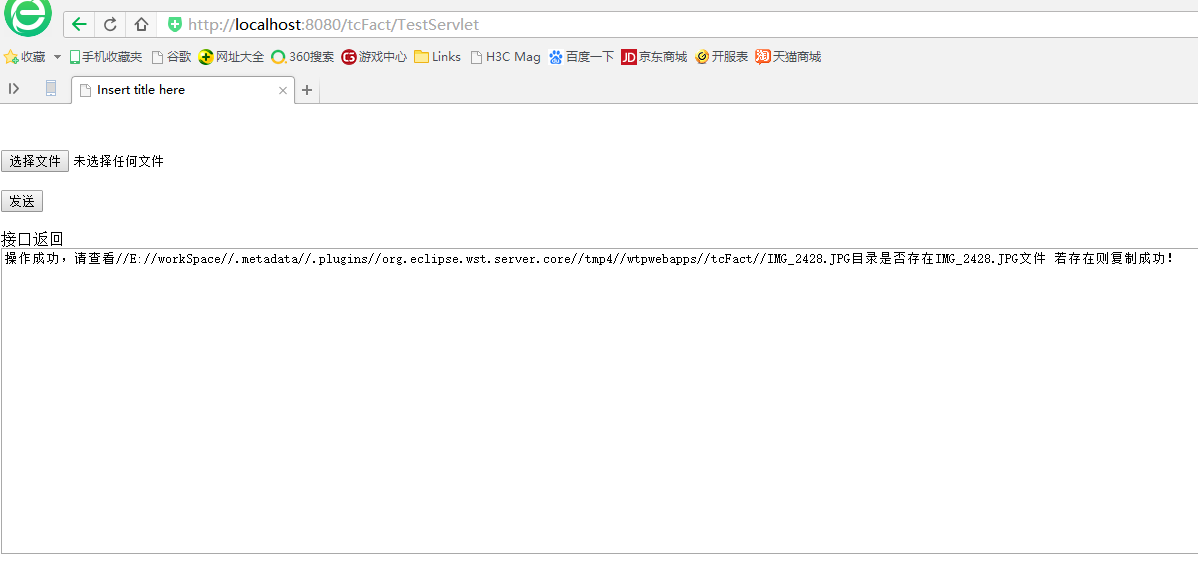
Finally, I hope I can help you...