Copyright Statement: This is an original article created by a blogger and may not be reproduced without the permission of the blogger.
When downloading videos, we often face the choice of storage path. Choosing a good storage path can optimize the memory in the Android system.The main app download path analysis and common path selection schemes are summarized as follows:
The video storage path scheme as an APP is provided below.The program flow chart of the specific scheme is as follows:
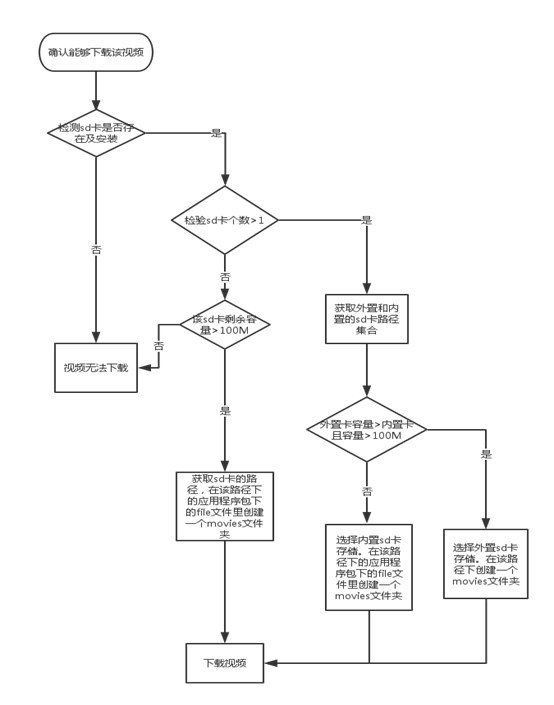
An overview of data storage paths in Android systems:
There are two main parts: internal storage and external storage
Internal storage is not memory.Internal storage is a special location in the system. If you want to store files in internal storage, files are accessible only by default to your application, and all files created by an application are in the same directory as the application package name.When an application is uninstalled, these files in the internal storage are also deleted.Technically, if you set the file properties to be readable when creating internal storage files, other apps can access the data of their own applications, provided they know the package name of the application. If the properties of a file are private, they will not be accessible even if they know the package name of other applications.Internal storage space is very limited and therefore valuable. It is also the main data storage location for the system itself and system applications. Once the internal storage space is exhausted, the mobile phone will not be available.So we try to avoid using internal storage space.Shared Preferences and SQLite databases are stored in internal storage space.
Storage Path: Files typically under data/data/package name/
External storage, it is easy to think that the built-in storage of the fuselage is internal storage, while the extended T card is external storage.For example, our task 16GB version of Nexus 4 has 16GB of internal storage, which the average consumer understands, but not in Android programming, which is still external storage.Android's early devices, when the internal storage of the device was really fixed, and the external storage was really movable like a U disk.However, in later devices, many high-end and mid-end machines extended their body storage to more than 8G. They conceptually divided the storage into "internal" and "external" parts, but they are actually inside the phone.
Storage Path: / sd Card Root directory / Android / data/<package name>/ file, specific path varies from model to model
2. Detailed analysis of the above APP flowchart
(1) Detect the existence and installation of sd card
/** * Does an sd card exist for a path * */ public static boolean isSdcardExist(Context context, String path) { boolean isSdcardExist = false; List<String> pathList = getExternalSdPath(context); if(!TextUtils.isEmpty(path) && path.equals(SDCARD_PATH)) { isSdcardExist = true; } else if(pathList != null && pathList.size() > 0) { for(int i = 0; i < pathList.size(); i++) { if(pathList.get(i).equals(path)) { isSdcardExist = true; break; } } } return isSdcardExist; }
/** * sd Whether the card is installed */ public static boolean isSDCardMounted() { return Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED); }
When operating on external storage, determine whether an sd card exists and is installed (mounted) and add the following permissions:
<!--Permission to write data to sdcard-->
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"></uses-permission>
<!--Permissions to create/delete files in sdcard-->
<uses-permission android:name="android.permission.MOUNT_UNMOUNT_FILESYSTEMS"></uses-permission>
(2) Detect the number of installed sd cards
The external storage of Android is an SD card. Generally, there is a built-in SD card inside the mobile phone. For example, a mobile phone has 16G memory. By default, the 16G memory of the body is set to the built-in SD card, which is the built-in SD card of the mobile phone.Some mobile phones also support external SD card insertion, so the location of the built-in memory card is obtained by the Environment.getExternal Storage Directory () method, and we need to write a special method to get the path of the external memory card.However, fewer and fewer mobile phones are equipped with a micro SD memory card slot in today's market. Even Samsung, which has been sticking to this design, this year's S6 and S6 Edge abandoned this feature and used built-in storage.There are also very few mobile phones that have multiple SD cards (not considered here).
/** * Get the number of sd cards in your mobile phone * */ private static int getSdcardCount(Context context) { int sdCount = 0; List<String> pathList = SDCardUtils.getExternalSdPath(context); if(SDCardUtils.isSDCardMounted()) { sdCount = 1; if(pathList != null && pathList.size() > 0) { sdCount = pathList.size() + 1; } } else { if(pathList != null && pathList.size() > 0) { sdCount = pathList.size(); } } return sdCount; }
(3) Get the path and remaining capacity of the built-in sd card
* sd Card Path */ public final static String SDCARD_PATH = Environment.getExternalStorageDirectory().getAbsolutePath();
/** * Gets the remaining space size of the sd card in M * */ public static double getSdAvailStorage(Context context, String sdPath) { double availStorage = 0; if(isSdcardExist(context, sdPath)) { StatFs sf = new StatFs(sdPath); long blockSize = sf.getBlockSize(); long availCount = sf.getAvailableBlocks(); availStorage = availCount*blockSize/1024/1024; } return availStorage; }
(3) Get the path of the external sd card
/** * Get External SD Card Path * */ public List getExtSDCardPath() { List lResult = new ArrayList(); try { Runtime rt = Runtime.getRuntime(); Process proc = rt.exec("mount"); InputStream is = proc.getInputStream(); InputStreamReader isr = new InputStreamReader(is); BufferedReader br = new BufferedReader(isr); String line; while ((line = br.readLine()) != null) { if (line.contains("extSdCard")) { String [] arr = line.split(" "); String path = arr[1]; File file = new File(path); if (file.isDirectory()) { lResult.add(path); } } } isr.close(); } catch (Exception e) { } return lResult; }
(3) Problems related to the storage of external sd cards
After android 4.4, when you switch the cache path to an external sd card, the video cannot be downloaded.The reason is that since android 4.4, google has restricted third-party applications from reading and writing access to external sd cards, making android more standardized.If you want to read and write an external sd card, you can only read and write from the Android/data/directory in the sd card root directory.Therefore, it is generally not possible and recommended to store external sd cards.
(4) Create a new video folder for video storage
/** * android4.4 After that, the SD card downloads the system directory */ public static String getSysDownLoadFolder(Context context, String path) { StringBuilder sb = new StringBuilder(); sb.append("Android/data/").append(context.getPackageName()).append(path).toString(); return sb.toString(); } } /* * Create a directory on the SD card; */ public File createDIR(String dirpath) { File dir=new File(SDPATH+dirpath); dir.mkdir(); return dir; }