1. Experimental task 1
Contents of this part:
Give the program task1.asm source code, and run screenshots
assume cs:code, ds:data data segment x db 1, 9, 3 len1 equ $ - x y dw 1, 9, 3 len2 equ $ - y data ends code segment start: mov ax, data mov ds, ax mov si, offset x mov cx, len1 mov ah, 2 s1:mov dl, [si] or dl, 30h int 21h mov dl, ' ' int 21h inc si loop s1 mov ah, 2 mov dl, 0ah int 21h mov si, offset y mov cx, len2/2 mov ah, 2 s2:mov dx, [si] or dl, 30h int 21h mov dl, ' ' int 21h add si, 2 loop s2 mov ah, 4ch int 21h code ends end start
Answer question ①
① line27, when the assembly instruction loop s1 jumps, it jumps according to the displacement. Check the machine code through debug disassembly and analyze the jump displacement? (the displacement value is answered in decimal) from the perspective of the CPU, explain how to calculate the offset address of the instruction after the jump label s1.
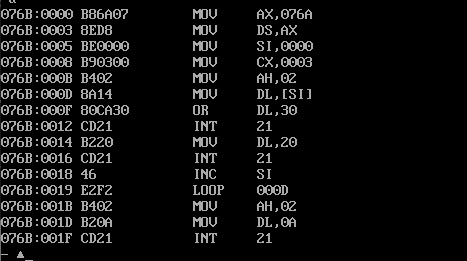
It can be seen from the figure that the machine code of loop s1 is E2F2. It is known that the loop jump instruction is a short-range jump with a jump range of - 128 ~ 127, so it corresponds to the two-bit machine code F2. Due to the jump to the low address, the jump displacement is the complement of F2, i.e. decimal 14 bytes.
Answer question ②
② line44. When the assembly instruction loop s2 jumps, it jumps according to the displacement. Check the machine code through debug disassembly and analyze the jump displacement? (the displacement value is answered in decimal) from the perspective of the CPU, explain how to calculate the offset address of the instruction after the jump label s2.
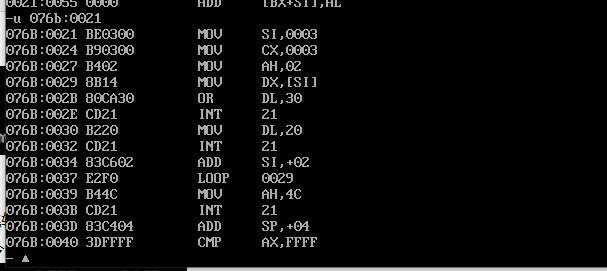
It can be seen from the figure that the machine code is E2F0, and F0 is the complement of the jump displacement. After the complement, 10010000 is obtained, and the decimal number corresponds to - 16, so the jump displacement is - 16.
From the perspective of CPU, when cx is not 0, (IP) = (IP) + 8-bit displacement, and the IP address is updated to the address 0039 of the next instruction of the Loop instruction. Therefore, when the displacement is - 16, jump from 0039 to 0029.
Question ③
③ Attach the disassembly screenshot of debugging observation in debug during the above analysis
1. 2 placed
2. Experimental task 2
Contents of this part:
The program task2.asm source code is given
assume cs:code, ds:data data segment dw 200h, 0h, 230h, 0h data ends stack segment db 16 dup(0) stack ends code segment start: mov ax, data mov ds, ax mov word ptr ds:[0], offset s1 mov word ptr ds:[2], offset s2 mov ds:[4], cs mov ax, stack mov ss, ax mov sp, 16 call word ptr ds:[0] s1: pop ax call dword ptr ds:[2] s2: pop bx pop cx mov ah, 4ch int 21h code ends end start
After analysis, debugging and verification, register (ax) =? (bx) = (cx) =? Attach the screenshot of the debugging result interface.
① According to the jump principle of call instruction, it is analyzed theoretically that before the program executes to exit (line31), register (ax) =? Register (bx) =? Register (cx) =?
Before the program exits, the data stored in ax is offset s1, the data stored in bx is offset s2, and the data stored in cx is cs
② Assemble and link the source program to get the executable program task2.exe. Use debug to observe and verify whether the debugging results are consistent with the theoretical analysis results.
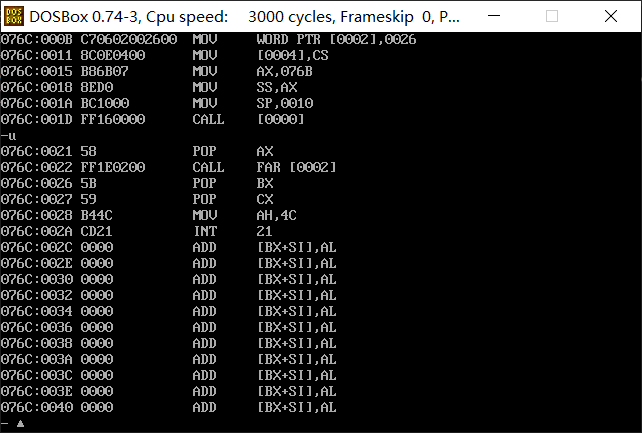
ax=0021 bx=0026 cx=076C, the conclusion is correct
3. Experimental task 3
Contents of this part:
assume cs:code, ds:data data segment x db 1, 9, 3 len1 equ $ - x y dw 1, 9, 3 len2 equ $ - y data ends code segment start: mov ax, data mov ds, ax mov si, offset x mov cx, len1 mov ah, 2 s1:mov dl, [si] or dl, 30h int 21h mov dl, ' ' int 21h inc si loop s1 mov ah, 2 mov dl, 0ah int 21h mov si, offset y mov cx, len2/2 mov ah, 2 s2:mov dx, [si] or dl, 30h int 21h mov dl, ' ' int 21h add si, 2 loop s2 mov ah, 4ch int 21h code ends end start
The program source code task3.asm running test screenshot is given
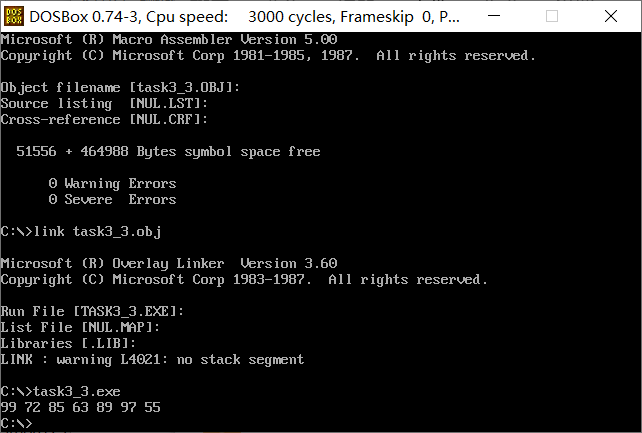
4. Experimental task 4
Contents of this part:
assume cs:code, ds:data data segment str db 'try' len = $-str data ends stack segment db 16 dup(0) stack ends code segment start: mov ax,data mov ds,ax mov ax,stack mov ss,ax mov sp,16 mov si,offset str mov cx,len mov bl,2;green mov bh,0 call printStr mov si,offset str mov cx,len mov bl,4 mov bh,24 call printStr mov ah,4ch int 21h printStr: mov dx,0b800h mov es,dx mov ah,0 mov al,bh mov di,160 mul di mov di,ax s: mov al,ds:[si] mov es:[di],al inc di mov es:[di],bl inc di inc si loop s ret code ends end start
The program source code task4.asm running test screenshot is given
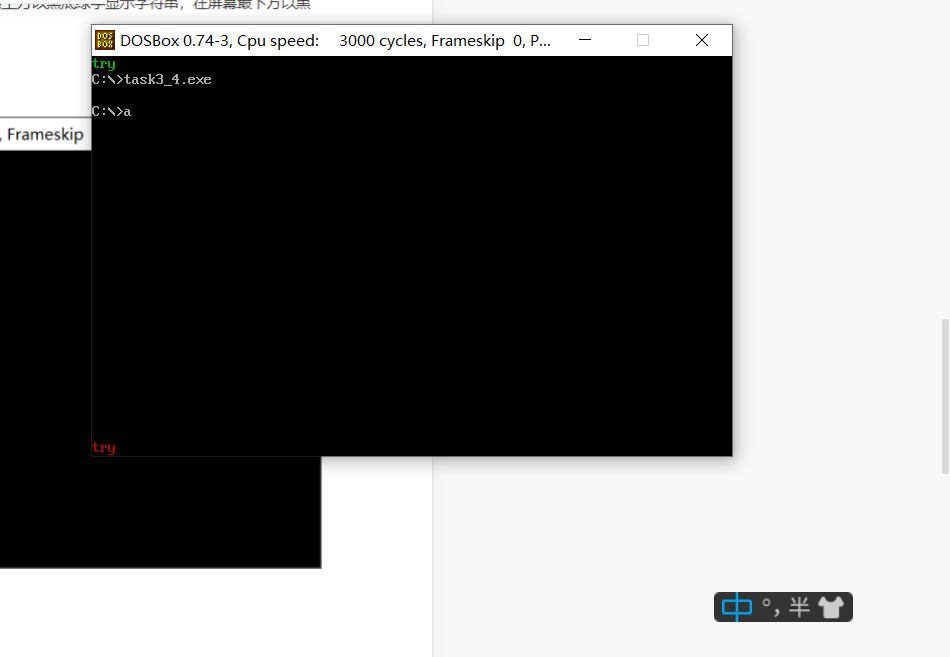
5. Experimental task 5
Contents of this part:
assume ds:data, cs:code data segment stu_no db '201983300980' len = $ - stu_no data ends code segment start: mov ax, data mov ds, ax mov cx, 4000 mov si, offset stu_no mov ax, 0b800h mov es, ax mov di, 0 mov ah,17h s: mov al, 0 mov es:[di], al mov es:[di+1], ah inc si add di, 2 loop s mov di, 3840 mov si, offset stu_no mov cx, 74 mov ah, 17h s1: call printgang add di, 2 loop s1 mov di, 3908 mov si, offset stu_no mov cx, len mov ah, 17h s2: call printStu_no inc si add di, 2 loop s2 mov di, 3932 mov si, offset stu_no mov cx, 74 mov ah, 17h s3: call printgang add di, 2 loop s3 mov ax, 4c00h int 21h printStu_no:mov al, [si] mov es:[di], al mov es:[di+1], ah ret printgang:mov al, 45 mov es:[di], al mov es:[di + 1], ah ret code ends end start
The program source code task5.asm running test screenshot is given
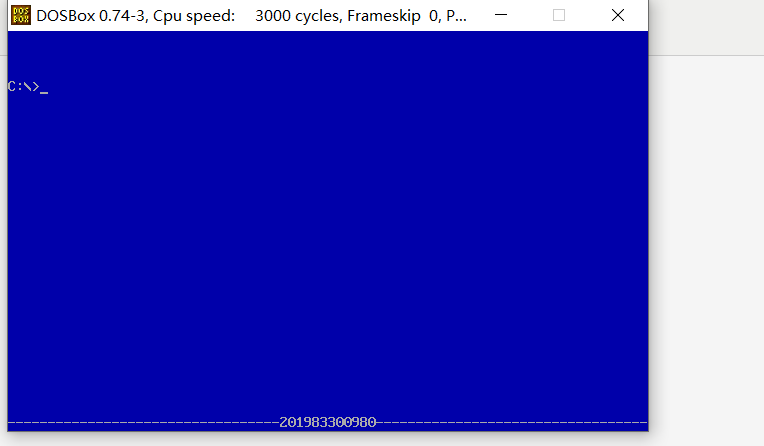