Experiment Task 1
assume cs:code, ds:data data segment x db 1, 9, 3 len1 equ $ - x ; y dw 1, 9, 3 len2 equ $ - y ; data ends code segment start: mov ax, data mov ds, ax mov si, offset x ; mov cx, len1 ; mov ah, 2 s1:mov dl, [si] or dl, 30h int 21h mov dl, ' ' int 21h ; inc si loop s1 mov ah, 2 mov dl, 0ah int 21h ; mov si, offset y ; mov cx, len2/2 ; mov ah, 2 s2:mov dx, [si] or dl, 30h int 21h mov dl, ' ' int 21h ; add si, 2 loop s2 mov ah, 4ch int 21h code ends end start
Run result: Output two lines 19 3
(1) line27, the assembly instruction loop s1 jumps according to the amount of displacement. Through debug disassembly, look at its machine code and analyze how much displacement it jumps? (Displacement values are answered in decimal numbers) From the perspective of the CPU, how to calculate the offset address of the instruction following the jump label s1.
The displacement is: 14 (Loop instruction end address: 001B, s1 instruction start address: 000D, 001B-000D=14)
Analysis: or dl, 30h command takes up three bytes, inc command takes up one byte, other commands take up two bytes, totaling 14 bytes.
(2) line44, the assembly instruction loop s2 jumps according to the amount of displacement. Through debug disassembly, look at its machine code and analyze how much displacement it jumps? (Displacement values are answered in decimal numbers) From the perspective of the CPU, how to calculate the offset address of the instruction following the jump label s2.
The displacement is: 16 (Loop instruction end address: 0039, s1 instruction start address: 0029, 001B-000D=16)
Analysis: or dl, the 30h command takes up three bytes, and the other commands take up two bytes, totaling 16 bytes.
Experiment Task 2
assume cs:code, ds:data data segment dw 200h, 0h, 230h, 0h data ends stack segment db 16 dup(0) stack ends code segment start: mov ax, data mov ds, ax mov word ptr ds:[0], offset s1 mov word ptr ds:[2], offset s2 mov ds:[4], cs mov ax, stack mov ss, ax mov sp, 16 call word ptr ds:[0] s1: pop ax call dword ptr ds:[2] s2: pop bx pop cx mov ah, 4ch int 21h code ends end start
Question 1
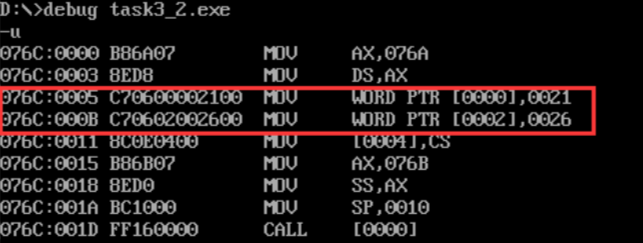
Experiment Task 3
assume cs:code, ds:data data segment x db 99, 72, 85, 63, 89, 97, 55 len equ $ - x data ends code segment start: mov ax,data mov ds,ax mov byte ptr ds:[len],10 mov cx,7 mov bx,0 s: mov al,ds:[bx] mov ah,0 inc bx call printNumber call printSpace loop s mov ah,4ch int 21h printNumber: div byte ptr ds:[len] mov dx,ax mov ah,2 or dl,30h int 21h mov ah,2 mov dl,dh or dl,30h int 21h ret printSpace: mov dl,' ' mov ah,2 int 21h ret code ends end start
Run Test Screenshot
Experiment Task 4
assume cs:code,ds:data data segment str db 'try' len equ $ - str data ends code segment start: mov ax, data mov ds, ax mov bl, 2 mov bh, 0 call printStr mov bl, 4 mov bh, 24 call printStr mov ax, 4c00h int 21h printStr: mov ax, 0b800h mov es, ax mov si, offset str mov cx, len mov al,0A0h mul bh mov di, ax s: mov al,[si] mov es:[di], al mov es:[di+1], bl inc si add di, 2 loop s ret code ends end start
Run Test Screenshot:
Experiment Task 5
assume ds:data, cs:code data segment stu_no db '201983300980' len = $ - stu_no data ends code segment start: mov ax, data mov ds, ax mov cx, 4000 mov si, offset stu_no mov ax, 0b800h mov es, ax mov di, 0 mov ah,17h s: mov al, 0 mov es:[di], al mov es:[di+1], ah inc si add di, 2 loop s mov di, 3840 mov si, offset stu_no mov cx, 74 mov ah, 17h s1: call printgang add di, 2 loop s1 mov di, 3908 mov si, offset stu_no mov cx, len mov ah, 17h s2: call printStu_no inc si add di, 2 loop s2 mov di, 3932 mov si, offset stu_no mov cx, 74 mov ah, 17h s3: call printgang add di, 2 loop s3 mov ax, 4c00h int 21h printStu_no:mov al, [si] mov es:[di], al mov es:[di+1], ah ret printgang:mov al, 45 mov es:[di], al mov es:[di + 1], ah ret code ends end start
Run result screenshot: