Here I'll share with you 8 useful js tips I've learned recently. No more nonsense. Let's write the code
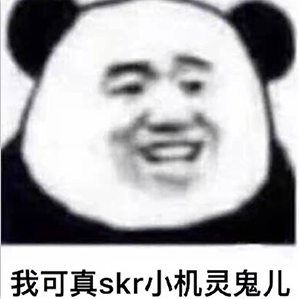
1. Ensure the array value
When using grid, you need to recreate the original data, and the column lengths of each row may not match. To ensure that the lengths of mismatched rows are equal, you can use the Array.fill method
let array = Array(5).fill('');
console.log(array); // outputs (5) ["", "", "", "", ""]
2. Get the unique value of the array
ES6 provides two very concise ways to extract unique values from arrays. Unfortunately, they don't handle arrays of non basic types very well. In this article, we mainly focus on basic data types.
const cars = [
'Mazda',
'Ford',
'Renault',
'Opel',
'Mazda'
]
const uniqueWithArrayFrom = Array.from(new Set(cars));
console.log(uniqueWithArrayFrom); // outputs ["Mazda", "Ford", "Renault", "Opel"]
const uniqueWithSpreadOperator = [...new Set(cars)];
console.log(uniqueWithSpreadOperator);// outputs ["Mazda", "Ford", "Renault", "Opel"]
3. Use the expand operator to merge objects and object arrays
Object merging is very common. We can use the new ES6 feature to handle the merging process better and more concisely.
// merging objects
const product = { name: 'Milk', packaging: 'Plastic', price: '5$' }
const manufacturer = { name: 'Company Name', address: 'The Company Address' }
const productManufacturer = { ...product, ...manufacturer };
console.log(productManufacturer);
// outputs { name: "Company Name", packaging: "Plastic", price: "5$", address: "The Company Address" }
// merging an array of objects into one
const cities = [
{ name: 'Paris', visited: 'no' },
{ name: 'Lyon', visited: 'no' },
{ name: 'Marseille', visited: 'yes' },
{ name: 'Rome', visited: 'yes' },
{ name: 'Milan', visited: 'no' },
{ name: 'Palermo', visited: 'yes' },
{ name: 'Genoa', visited: 'yes' },
{ name: 'Berlin', visited: 'no' },
{ name: 'Hamburg', visited: 'yes' },
{ name: 'New York', visited: 'yes' }
];
const result = cities.reduce((accumulator, item) => {
return {
...accumulator,
[item.name]: item.visited
}
}, {});
console.log(result);
/* outputs
Berlin: "no"
Genoa: "yes"
Hamburg: "yes"
Lyon: "no"
Marseille: "yes"
Milan: "no"
New York: "yes"
Palermo: "yes"
Paris: "no"
Rome: "yes"
*/
4. Array map method (without Array.Map)
Another way to implement array map is not to use Array.map.
Array.from can also accept the second parameter, which is similar to the map method of array. It is used to process each element and put the processed value into the returned array. As follows:
const cities = [
{ name: 'Paris', visited: 'no' },
{ name: 'Lyon', visited: 'no' },
{ name: 'Marseille', visited: 'yes' },
{ name: 'Rome', visited: 'yes' },
{ name: 'Milan', visited: 'no' },
{ name: 'Palermo', visited: 'yes' },
{ name: 'Genoa', visited: 'yes' },
{ name: 'Berlin', visited: 'no' },
{ name: 'Hamburg', visited: 'yes' },
{ name: 'New York', visited: 'yes' }
];
const cityNames = Array.from(cities, ({ name}) => name);
console.log(cityNames);
// outputs ["Paris", "Lyon", "Marseille", "Rome", "Milan", "Palermo", "Genoa", "Berlin", "Hamburg", "New York"]
5. Conditional object attributes
Instead of creating two different objects based on one condition, you can use the expand operation symbol to process them.
nst getUser = (emailIncluded) => {
return {
name: 'John',
surname: 'Doe',
...emailIncluded && { email : 'john@doe.com' }
}
}
const user = getUser(true);
console.log(user); // outputs { name: "John", surname: "Doe", email: "john@doe.com" }
const userWithoutEmail = getUser(false);
console.log(userWithoutEmail); // outputs { name: "John", surname: "Doe" }
6. Deconstruct the original data
Sometimes an object contains many attributes, and we only need a few of them. Here we can use deconstruction to extract the attributes we need. For example, the contents of a user object are as follows:
const rawUser = {
name: 'John',
surname: 'Doe',
email: 'john@doe.com',
displayName: 'SuperCoolJohn',
joined: '2016-05-05',
image: 'path-to-the-image',
followers: 45
...
}
We need to extract two parts, namely user and user information, which can be done as follows:
let user = {}, userDetails = {};
({ name: user.name, surname: user.surname, ...userDetails } = rawUser);
console.log(user); // outputs { name: "John", surname: "Doe" }
console.log(userDetails); // outputs { email: "john@doe.com", displayName: "SuperCoolJohn", joined: "2016-05-05", image: "path-to-the-image", followers: 45 }
7. Dynamic attribute name
In the early days, if the property name needs to be dynamic, we must first declare an object and then assign a property. These days have passed, and with the ES6 feature, we can do this.
const dynamic = 'email';
let user = {
name: 'John',
[dynamic]: 'john@doe.com'
}
console.log(user); // outputs { name: "John", email: "john@doe.com" }
8. String interpolation
In the use case, if you are building a template based helper component, this will be very prominent, which makes it much easier to connect dynamic templates.
const user = {
name: 'John',
surname: 'Doe',
details: {
email: 'john@doe.com',
displayName: 'SuperCoolJohn',
joined: '2016-05-05',
image: 'path-to-the-image',
followers: 45
}
}
const printUserInfo = (user) => {
const text = The user is ${user.name} ${user.surname}. Email: ${user.details.email}. Display Name: ${user.details.displayName}. ${user.name} has ${user.details.followers} followers.
console.log(text);
}
printUserInfo(user);
// outputs 'The user is John Doe. Email: john@doe.com. Display Name: SuperCoolJohn. John has 45 followers.'
If it helps you, you are welcome to pay attention. I will update the technical documents regularly to discuss, learn and make progress together. Finally, Xiaobian will share some front-end gift packs to help you learn better! [Jiajun sheep: 581286372]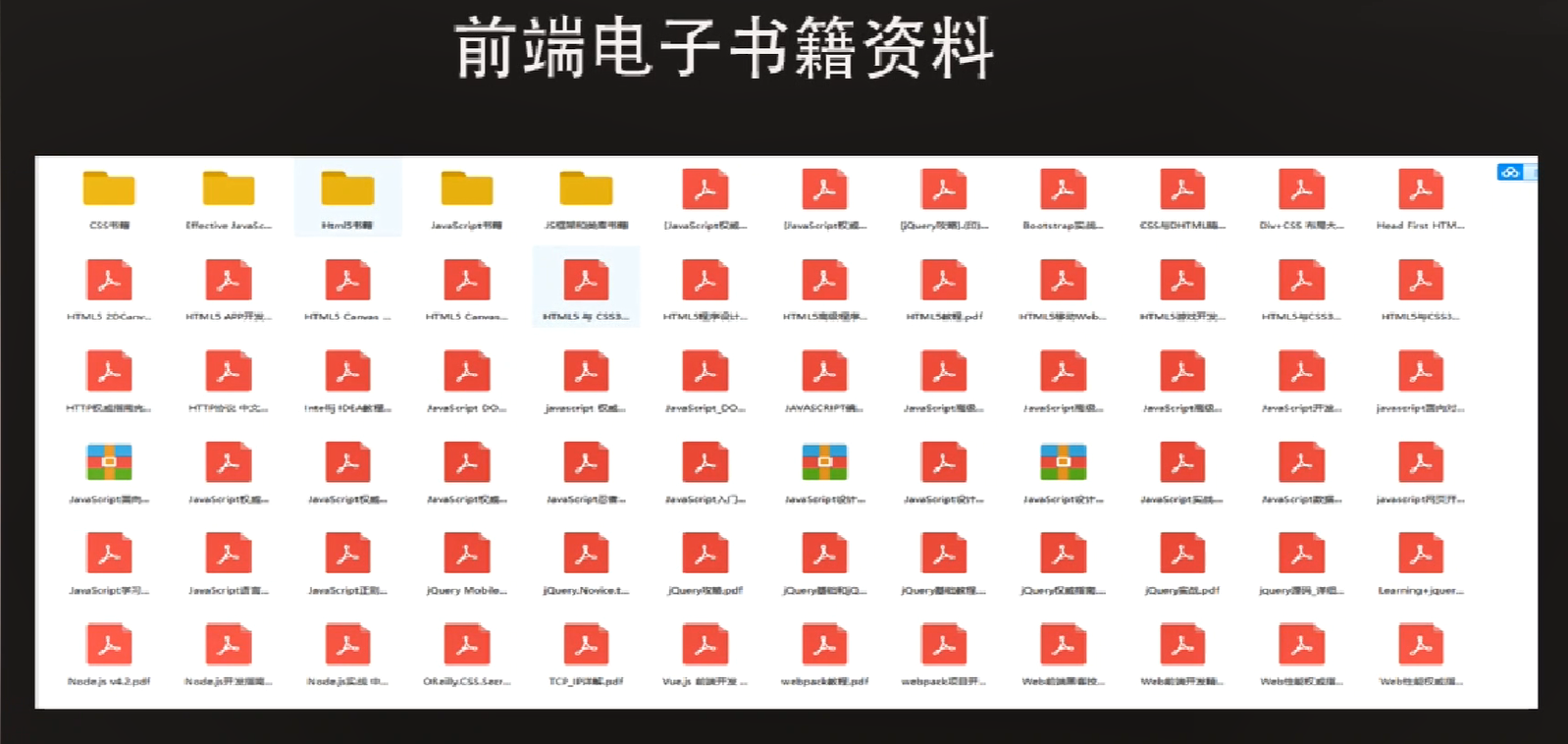