1, Experimental purpose
1. Understand and master the jump principle of transfer instruction
2. Master the method of using call and ret instructions to realize subroutines, and understand and master the parameter transfer mode
3. Understand and master 80 × 25 color character mode display principle
4. Integrate addressing mode and assembly instructions to complete simple application programming
2, Experimental preparation
Review chapters 9-10 of the textbook:
Jump principle of transfer instruction
Usage of assembly instructions jmp, loop, jcxz, call, ret, retf
3, Experimental content
1. Experimental task 1
task1.asm source code:
assume cs:code, ds:data data segment x db 1, 9, 3 len1 equ $ - x ; symbolic constants , $Refers to the offset address of the next data item. In this example, it is 3 y dw 1, 9, 3 len2 equ $ - y ; symbolic constants , $Refers to the offset address of the next data item. In this example, it is 9 data ends code segment start: mov ax, data mov ds, ax mov si, offset x ; Take symbol x Corresponding offset address 0 -> si mov cx, len1 ; From symbol x Number of consecutive byte data items at the beginning -> cx mov ah, 2 s1:mov dl, [si] or dl, 30h int 21h mov dl, ' ' int 21h ; Output space inc si loop s1 mov ah, 2 mov dl, 0ah int 21h ; Line feed mov si, offset y ; Take symbol y Corresponding offset address 3 -> si mov cx, len2/2 ; From symbol y Number of consecutive word data items started -> cx mov ah, 2 s2:mov dx, [si] or dl, 30h int 21h mov dl, ' ' int 21h ; Output space add si, 2 loop s2 mov ah, 4ch int 21h code ends end start
Operation screenshot:

Commissioning screenshot:
① line27, when the assembly instruction loop s1 jumps, it jumps according to the displacement. Check the machine through debug disassembly
What is the jump displacement of the code? (the displacement value is answered in decimal) from the perspective of CPU, it is explained
How to calculate the offset address of the instruction after the jump label s1.
Jump from 001B to 000D, the displacement is - 14, and the CPU storage content F2 is the complement of - 14
② line44. When the assembly instruction loop s2 jumps, it jumps according to the displacement. Check the machine through debug disassembly
What is the jump displacement of the code? (the displacement value is answered in decimal) from the perspective of CPU, it is explained
How to calculate the offset address of the instruction after the jump label s2.
Jump from 0039 to 0029, the displacement is - 16, and the CPU storage content F0 is the complement of - 16
2. Experimental task 2
task2.asm source code:
assume cs:code, ds:data data segment dw 200h, 0h, 230h, 0h data ends stack segment db 16 dup(0) stack ends code segment start: mov ax, data mov ds, ax mov word ptr ds:[0], offset s1 mov word ptr ds:[2], offset s2 mov ds:[4], cs mov ax, stack mov ss, ax mov sp, 16 call word ptr ds:[0] s1: pop ax call dword ptr ds:[2] s2: pop bx pop cx mov ah, 4ch int 21h code ends end start
① According to the jump principle of call instruction, it is analyzed theoretically that the register (ax) before the program execution to exit (line31)=
0021 register (bx) = 0026 register (cx) = 076C
② Assemble and link the source program to get the executable program task2.exe. Use debug to debug, observe and verify debugging
Whether the results are consistent with the theoretical analysis results.
The debugging screenshot shows that the results are consistent.
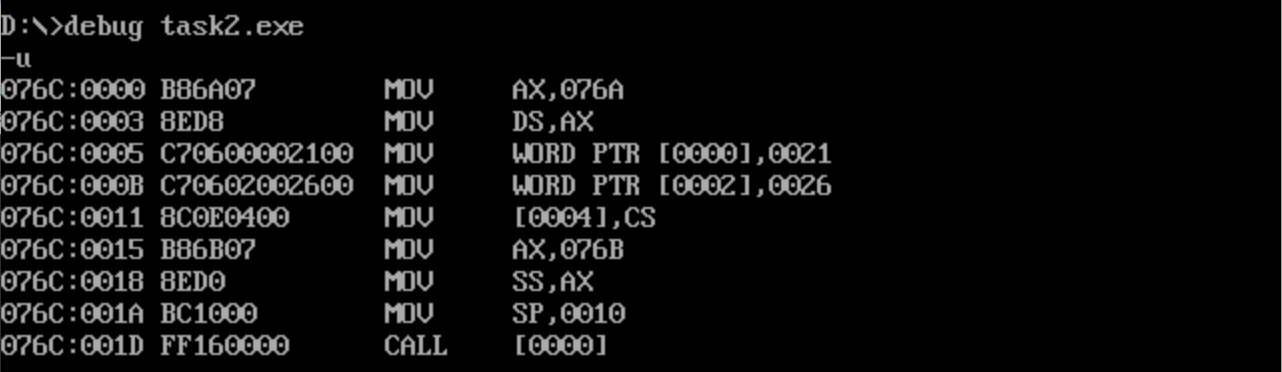
3. Experimental task 3
task3.asm source code:
assume cs:code, ds:data data segment x db 99, 72, 85, 63, 89, 97, 55 len equ $- x data ends code segment start: mov ax, data mov ds, ax mov cx, len mov si, 0 s: mov ah, 0 mov al, ds:[si] call printNumber call printSpace inc si loop s mov ah, 4ch int 21h printNumber: mov bl, 10 div bl mov dl, al mov dh, ah or dl, 30h or dh, 30h mov ah, 2 int 21h mov dl, dh int 21h ret printSpace: mov ah, 2 mov dl, ' ' int 21h ret code ends end start
Screenshot of running test:

4. Experimental task 4
task4.asm source code:
assume cs:code, ds:data data segment str db 'try' len equ $ - str data ends code segment start: mov ax, data mov ds, ax mov ax,0b800h mov es,ax mov si,offset printStr mov ah,2 mov bx,0 call si mov si,offset printStr mov ah,4 mov bx,0F00H call si mov ah, 4ch int 21h printStr: mov cx,len mov si,0 s: mov al,[si] mov es:[bx+si],ax inc si add bx,1 loop s ret code ends end start
Screenshot of running test:
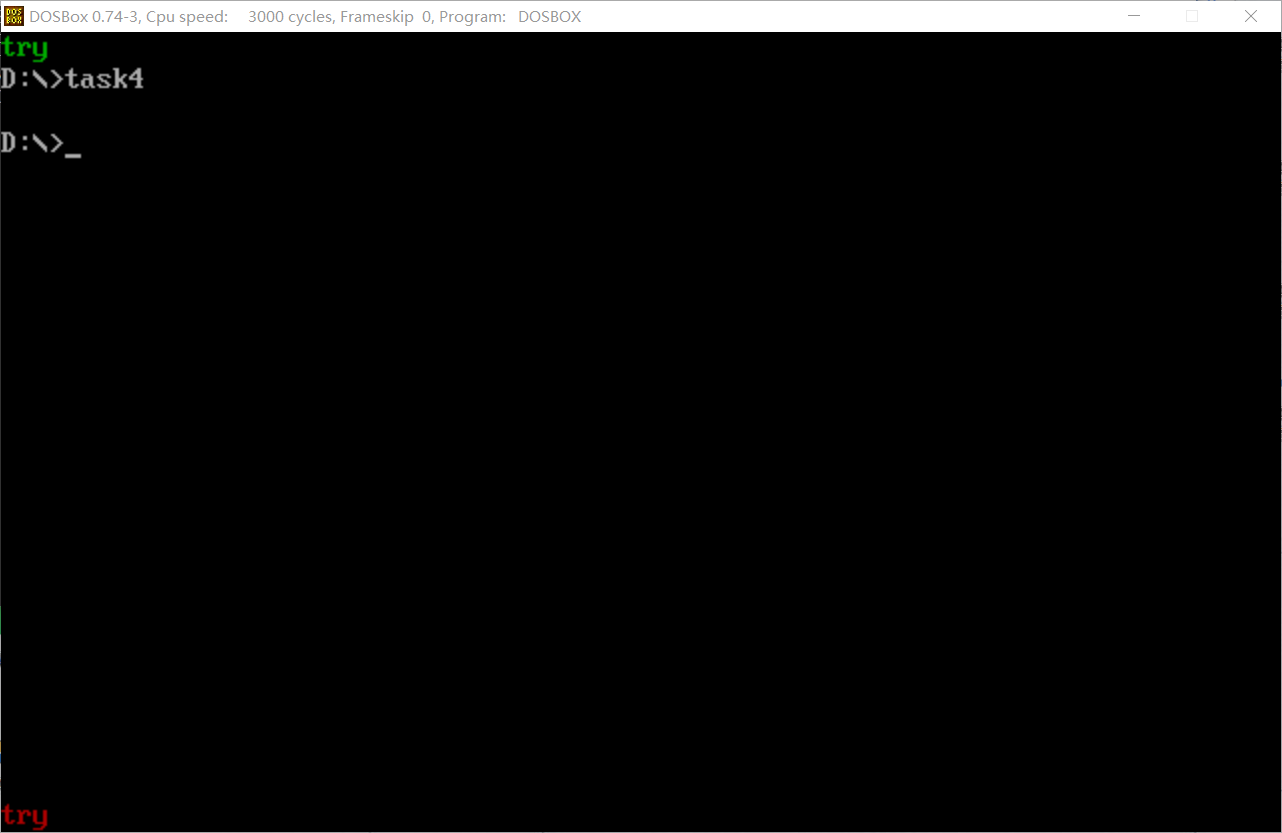
5. Experimental task 5
task5.asm source code:assume cs:code,ds:data data segment str db '201983290329' len equ $ - str data ends code segment start: mov ax,data mov ds,ax mov ax,0b800h mov es,ax mov cx,0F9Fh mov bx,0 s1: mov ah,17h mov al,' ' mov es:[bx],ax add bx,2 loop s1 mov bx,0F00h mov cx,34 s2: mov al,'-' mov es:[bx],ax add bx,2 loop s2 mov cx,len mov si,0 s3: mov al,[si] inc si mov es:[bx],ax add bx,2 loop s3 mov cx,34 s4: mov al,'-' mov es:[bx],ax add bx,2 loop s4 mov ax,4ch int 21h code ends end
Screenshot of running test:
4, Experimental summary
Master the method of using instructions to realize subroutines, feel the transfer process of parameters, and be familiar with the usage of assembly instructions such as jmp, loop, jcxz, call, ret, retf, etc