IV. EXPERIMENTAL CONCLUSIONS
Experiment Task 1
Task3_ Source for 1
assume cs:code, ds:data data segment x db 1, 9, 3 len1 equ $ - x y dw 1, 9, 3 len2 equ $ - y data ends code segment start: mov ax, data mov ds, ax mov si, offset x mov cx, len1 mov ah, 2 s1:mov dl, [si] or dl, 30h int 21h mov dl, ' ' int 21h inc si loop s1 mov ah, 2 mov dl, 0ah int 21h mov si, offset y mov cx, len2/2 mov ah, 2 s2:mov dx, [si] or dl, 30h int 21h mov dl, ' ' int 21h add si, 2 loop s2 mov ah, 4ch int 21h code ends end start
Run result: Output two lines 19 3
Question 1
The machine code of loop1 is E2F2. The jump displacement is 001B-000D=E That is 001B+(-E)=000D, displacement is -14
From the CPU point of view, E2F2, where the displacement is F2, F stands for forward transfer, 001B+00F2=010D, take the last two bits
Question 2
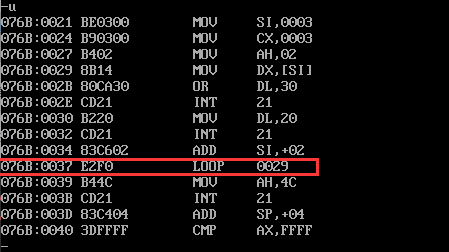
loops2's machine instruction is E2F0, jump offset is 0039-0029=0010 jump forward 16 bytes
From the CPU point of view, E2F0, where the displacement is F0, F stands for forward transfer, 0039+00F0=0129, take the last two bits
2. Experimental Task 2
Tsak3_ Source for 2
assume cs:code, ds:data data segment dw 200h, 0h, 230h, 0h data ends stack segment db 16 dup(0) stack ends code segment start: mov ax, data mov ds, ax mov word ptr ds:[0], offset s1 mov word ptr ds:[2], offset s2 mov ds:[4], cs mov ax, stack mov ss, ax mov sp, 16 call word ptr ds:[0] s1: pop ax call dword ptr ds:[2] s2: pop bx pop cx mov ah, 4ch int 21h code ends end start
Question 1
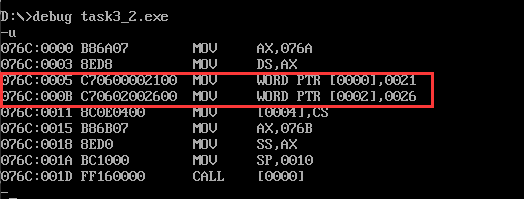
3. Experimental Task 3
data segment x db 99, 72, 85, 63, 89, 97, 55 len equ $- x data ends
assume cs:code, ds:data data segment x db 99,72,85,63,89,97,55 len equ $ - x data ends code segment start: mov ax,data mov ds,ax mov si,offset x mov cx,len mov byte ptr ds:[10],10 s1: mov ah,0 mov al,ds:[si] div byte ptr ds:[10] call printNumber call printSpace inc si loop s1 mov ah,4ch int 21h printNumber: mov dl,al mov dh,ah or dl,30h mov ah,2 int 21h mov dl,dh or dl,30h int 21h ret printSpace: mov ah,2 mov dl,' ' int 21h ret code ends end start
4. Experimental Task 4
Write 8086 assembly source program task3_4.asm, on the screen to specify the color, row, on the screen output string.
data segment str db 'try' len equ $ - str data ends
assume cs:code, ds:data data segment str db 'try' len equ $ - str data ends code segment start: mov ax,data mov ds,ax mov si,0 mov bh,0;Line Number mov bl,4;colour mov cx,len call printStr mov si,0 mov bh,24 mov bl,2 mov cx,len call printStr mov ah,4ch int 21h printStr: mov ax,0b800h;Video memory address mov es,ax mov ax,0 mov al,bh mov dx,160;160 characters per line mul dx mov di,ax s: mov al,ds:[si] mov ah,bl;colour mov es:[di],ax inc si add di,2 loop s ret code ends end start
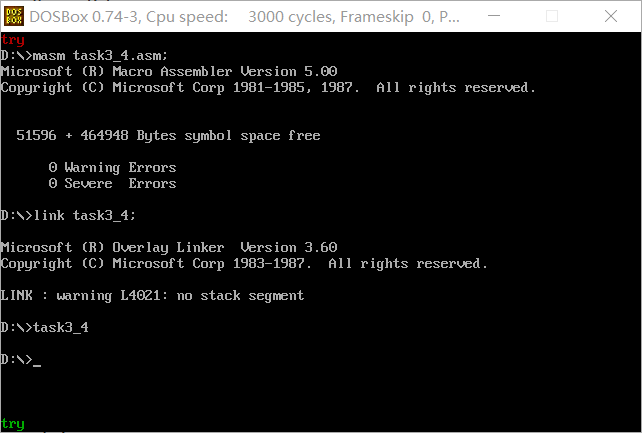
5. Experimental Task 5
assume cs:code, ds:data data segment stu_no db '201983290029' len = $ - stu_no l2 = 40 - len/2 data ends code segment start: mov ax,data mov ds,ax mov si,0 mov bl,00010001B call printOr mov bl,00010111B call printNo mov ah,4ch int 21h printOr: mov ax,0b800h;Video memory address mov es,ax mov ax,0 mov al,24 mov dx,160; mul dx mov cx,ax s0: mov byte ptr es:[di],' ' mov es:[di+1],bl add di,2 loop s ret printNo: mov ax,0b800h;Video memory address mov es,ax mov ax,0 mov al,24 mov dx,160;160 bytes per line mul dx mov di,ax mov cx,l2;Print- s: mov byte ptr es:[di],'-' mov es:[di+1],bl add di,2 loop s mov si,0 mov cx,len;Print school number s1: mov al,ds:[si] mov ah,bl;colour mov es:[di],ax add di,2 inc si loop s1 mov cx,l2;Print- s2: mov byte ptr es:[di],'-' mov es:[di+1],bl add di,2 loop s2 ret code ends end start
V. EXPERIMENTAL SUMMARY
A character displayed on the screen with foreground and background colors. Format of attribute bytes
7 6 5 4 3 2 1 0
BL R G B I R G B
Twinkle background Highlight background