assume cs:code, ds:data data segment x db 1, 9, 3 len1 equ $ - x ; symbolic constants, $The offset address for the next data item, in this example, is 3 y dw 1, 9, 3 len2 equ $ - y ; symbolic constants, $The offset address for the next data item, in this example, is 9 data ends code segment start: mov ax, data mov ds, ax mov si, offset x ; Take Symbols x Corresponding offset address 0 -> si mov cx, len1 ; From Symbol x Number of consecutive byte data items to start with -> cx mov ah, 2 s1:mov dl, [si] or dl, 30h int 21h mov dl, ' ' int 21h ; Output space inc si loop s1 mov ah, 2 mov dl, 0ah int 21h ; Line Break mov si, offset y ; Take Symbols y Corresponding offset address 3 -> si mov cx, len2/2 ; From Symbol y Number of consecutive word data items to start with -> cx mov ah, 2 s2:mov dx, [si] or dl, 30h int 21h mov dl, ' ' int 21h ; Output space add si, 2 loop s2 mov ah, 4ch int 21h code ends end start
Source Code Run Results:
①
The machine code of the line27 loop command is E2F2, the octet binary form of F2 is 1110010, the complement is 10001110, the decimal form is -14, that is, the displacement is 14
From a CPU perspective, cs:ip points to the memory unit of line27, reading loop s1 into the instruction buffer
First modify the value of IP by reading the length of the instruction, ip=ip+instruction length
loop s1 is then executed, jumping to s1, where the displacement is equal to the offset address at S1 minus the offset address to which the current ip points
②
The machine code of the line44 loop command is E2F0, the octal binary form of F0 is 1110000, the complement is 10010000, the decimal form is -16, that is, the displacement is 16
From a CPU perspective, cs:ip points to the memory unit of line44, reading loop s2 into the instruction buffer
First modify the value of IP by reading the length of the instruction, ip=ip+instruction length
loop s2 is then executed, jumping to s2, where the displacement is equal to the offset address at S2 minus the offset address to which the current ip points
assume cs:code, ds:data data segment dw 200h, 0h, 230h, 0h data ends stack segment db 16 dup(0) stack ends code segment start: mov ax, data mov ds, ax mov word ptr ds:[0], offset s1 mov word ptr ds:[2], offset s2 mov ds:[4], cs mov ax, stack mov ss, ax mov sp, 16 call word ptr ds:[0] s1: pop ax call dword ptr ds:[2] s2: pop bx pop cx mov ah, 4ch int 21h code ends end start
Analysis: call word ptr ds:[0] short transfer, push the next instruction offset address (ip) onto the stack and transfer to ds:[0] address, which is s1, pop ax will stack this content to ax afterwards;
call dword ptr ds:[2] For inter-segment transfer, the next instruction base address and offset addresses (cs and ip) are pushed onto the stack and transferred to ds:[2] address, which is s2. After that, pop bx takes IP out of the stack to BX and pop CX takes CS out of the stack to cx.
So AX=0021 BX=0026 CX=076C
Verify that AX, BX, CX are correct after debug
data segment x db 99, 72, 85, 63, 89, 97, 55 len equ $- x data ends
assume cs:code, ds:data data segment x db 99, 72, 85, 63, 89, 97, 55 len equ $- x data ends code segment start: mov ax, data mov ds, ax mov cx, len mov si, 0 print: mov al, [si] mov ah, 0 call printNumber call printSpace inc si loop print mov ah, 4ch int 21h printNumber: mov bl, 10 div bl mov bx, ax mov ah, 2 mov dl, bl ; Printer or dl, 30h int 21h mov dl, bh ; Print Remainder or dl, 30h int 21h ret printSpace: mov ah, 2 mov dl, ' ' int 21h ret code ends end start

data segment str db 'try' len equ $ - str data ends
assume cs:code data segment str db 'try' len equ $ - str data ends stack segment dw 2 dup(?) stack ends code segment start: mov ax, data mov ds, ax mov ax, stack mov ss, ax mov sp, 2 mov cx, len ;String Length mov ax, 0 mov si, ax mov bl, 0Ah ;Green Character mov bh, 0 ;Line Number call printStr mov bl, 0Ch ;gules mov bh, 24 call printStr mov ah, 4ch int 21h printStr: mov al, bh mov dl, 0A0h ;160 bytes per line mul dl mov di, ax ;Line Start Address mov ax, 0b800h ;Display Start Address mov es, ax push si push cx startPrint: mov al, ds:[si] mov es:[di], al ;Place Characters mov es:[di+1], bl ;Place Color inc si inc di inc di loop startPrint pop cx pop si ret code ends end start
The output is as follows:
data segment stu_no db '201983290032' len = $ - stu_no data ends
assume cs:code, ds:data data segment stu_no db '201983290032' len = $ - stu_no data ends code segment start: mov ax, data mov ds, ax mov di, 0 call printStuNum mov ah, 4ch int 21h printStuNum: mov ax, 0b800h mov es, ax mov si, 1 mov al, 24 mov dl, 80 mul dl mov cx, ax printBlue: mov al, 17h ;00010111 mov es:[si], al ;fill color add si, 2 loop printBlue sub si, 1 mov ax, 80 sub ax, len mov dl, 2 div dl mov dx, ax ;Preservation-Length mov cx, dx call printheng mov cx, len printStu: ;Output number mov al, ds:[di] mov ah, 17h mov word ptr es:[si], ax inc di add si, 2 loop printStu mov cx, dx call printheng ret printheng: mov al, '-' mov ah, 17h mov word ptr es:[si], ax add si, 2 loop printheng ret code ends end start
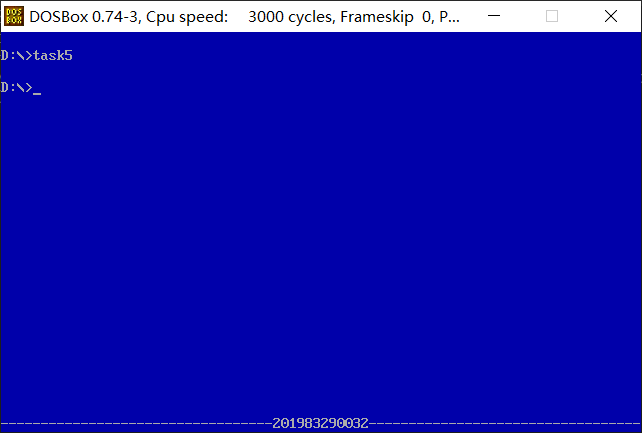