Previously, we have released ten articles of Numpy series for you. Here we come to an end for the time being. Now we provide you with 100 Numpy exercises as a leak detection and filling!
Previously, I summarized the common functions in Numpy for you, but there are no examples to explain. Well, today's 100 questions are a good exercise.
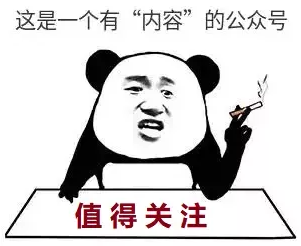
Source: https://github.com/rougier/numpy-100
Numpy is one of the basic libraries that Python must master for data analysis. The following topic is an open source project on github, which is mainly to test your numpy ability and supplement your learning. Huang spent 2 hours sorting it out for everyone. I hope it will be helpful to you.
1. Import numpy library and take the alias np (★☆☆)
(prompt: import... as...)
import numpy as np
2. Print out numpy version and configuration information (★☆☆)
(prompt: np.version, np.show_config)
print (np.__version__) print(np.show_config()
3. Create an empty vector with length of 10 (★☆☆)
(tip: np.zeros)
Z = np.zeros(10) print(Z)
4. How to find the memory size of any array? (★☆☆)
(prompt: size, itemsize)
Z = np.zeros((10,10)) print("%d bytes" % (Z.size * Z.itemsize))
5. How to get the description document of the add function in numpy from the command line? (★☆☆)
(tip: np.info)
import numpy numpy.info(numpy.add)
6. Create an empty vector with a length of 10 and a value of 1 except the fifth one (★☆)
(prompt: array[4])
Z = np.zeros(10) Z[4] = 1 print(Z)
7. Create a vector with a value range from 10 to 49 (★☆☆)
(tip: NP. Orange)
Z = np.arange(10,50) print(Z)
8. Invert a vector (the first element becomes the last) (★☆)
(hint: array[::-1])
Z = np.arange(50) Z = Z[::-1] print(Z)
9. Create a 3x3 matrix with values from 0 to 8 (★☆☆)
(prompt: reshape)
Z = np.arange(9).reshape(3,3) print(Z)
10. Find the location index of non-0 elements in the array [1,2,0,0,4,0] (★☆)
(tip: np.nonzero)
nz = np.nonzero([1,2,0,0,4,0]) print(nz)
11. Create a 3x3 identity matrix (★☆☆)
(tip: np.eye)
Z = np.eye(3) print(Z)
12. Create a 3x3x3 random array (★☆)
(tip: np.random.random)
Z = np.random.random((3,3,3)) print(Z)
13. Create a 10x10 random array and find its maximum and minimum values (★☆)
(prompt: min, max)
Z = np.random.random((10,10)) Zmin, Zmax = Z.min(), Z.max() print(Zmin, Zmax)
14. Create a random vector with a length of 30 and find its average value (★☆)
(prompt: mean)
Z = np.random.random(30) m = Z.mean() print(m)
15. Create a two-dimensional array, where the boundary value is 1 and the other values are 0 (★☆)
(prompt: array[1:-1, 1:-1])
Z = np.ones((10,10)) Z[1:-1,1:-1] = 0 print(Z)
16. For an existing array, how to add a boundary filled with 0? (★☆☆)
(tip: np.pad)
Z = np.ones((5,5)) Z = np.pad(Z, pad_width=1, mode='constant', constant_values=0) print(Z)
17. What is the result of the following expression? (★☆☆)
(hint: NaN = not a number, inf = infinity) (hint: NaN: not a number, inf: infinite)
# expression # result 0 * np.nan nan np.nan == np.nan False np.inf > np.nan False np.nan - np.nan nan 0.3 == 3 * 0.1 False
18. Create a 5x5 matrix and set the values 1, 2, 3 and 4 to fall below its diagonal (★☆☆)
(tip: np.diag)
Z = np.diag(1+np.arange(4),k=-1) print(Z)
19. Create an 8x8 matrix and set it to chessboard style (★☆)
(hint: array[::2])
Z = np.zeros((8,8),dtype=int) Z[1::2,::2] = 1 Z[::2,1::2] = 1 print(Z)
20. Consider an array of (6,7,8) shapes. What is the index (x,y,z) of the 100th element?
(tip: np.unravel_index)
print(np.unravel_index(100,(6,7,8)))
21. Use tile function to create an 8x8 chessboard style matrix (★☆)
(tip: np.tile)
Z = np.tile( np.array([[0,1],[1,0]]), (4,4)) print(Z)
22. Normalize a 5x5 random matrix (★☆☆)
(prompt: (x - min) / (max - min))
Z = np.random.random((5,5)) Zmax, Zmin = Z.max(), Z.min() Z = (Z - Zmin)/(Zmax - Zmin) print(Z)
23. Create a custom dtype that describes the color as (RGBA) four unsigned bytes? (★☆☆)
(tip: np.dtype)
color = np.dtype([("r", np.ubyte, 1), ("g", np.ubyte, 1), ("b", np.ubyte, 1), ("a", np.ubyte, 1)]) color
24. When a 5x3 matrix is multiplied by a 3x2 matrix, what is the real matrix product? (★☆☆)
(prompt: np.dot | @)
Z = np.dot(np.ones((5,3)), np.ones((3,2))) print(Z)
25. Given a one-dimensional array, negate all elements between 3 and 8 (★☆)
(prompt: >, < =)
Z = np.arange(11) Z[(3 < Z) & (Z <= 8)] *= -1 print(Z)
26. What is the result of running the following script? (★☆☆)
(tip: np.sum)
# Author: Jake VanderPlas # result print(sum(range(5),-1)) 9 from numpy import * print(sum(range(5),-1)) 10 #numpy.sum(a, axis=None)
27. Considering an integer vector Z, which of the following expressions is valid? (★☆☆)
(hint: there is also "bitwise operator")
Z**Z True 2 << Z >> 2 False Z <- Z True 1j*Z True #complex Z/1/1 True Z<Z>Z False
28. What are the results of the following expressions? (★☆☆)
np.array(0) / np.array(0) nan np.array(0) // np.array(0) 0 np.array([np.nan]).astype(int).astype(float) -2.14748365e+09
29. How do I round a floating-point array from zero? (★☆☆)
(Tips: np.uniform, np.copysign, np.ceil, np.abs)
# Author: Charles R Harris Z = np.random.uniform(-10,+10,10) print (np.copysign(np.ceil(np.abs(Z)), Z))
30. How to find the elements common to two arrays? (★☆☆)
(tip: np.intersect1d)
Z1 = np.random.randint(0, 10, 10) Z2 = np.random.randint(0, 10, 10) print (np.intersect1d(Z1, Z2))
31. How do I ignore all numpy warnings (although not recommended)? (★☆☆)
(prompt: np.seterr, np.errstate)
# Suicide mode on defaults = np.seterr(all="ignore") Z = np.ones(1) / 0 # Back to sanity _ = np.seterr(**defaults) # Another equivalent way is to use the context manager with np.errstate(divide='ignore'): Z = np.ones(1) / 0
32. Is the following expression true? (★☆☆)
(hint: imaginary number)
np.sqrt(-1) == np.emath.sqrt(-1) Faslse
33. How do I get the dates of yesterday, today and tomorrow? (★☆☆)
(prompt: np.datetime64, np.timedelta64)
yesterday = np.datetime64('today', 'D') - np.timedelta64(1, 'D') today = np.datetime64('today', 'D') tomorrow = np.datetime64('today', 'D') + np.timedelta64(1, 'D')
34. How do I get all the dates related to July 2016? (★★☆)
(prompt: NP. Orange (dtype = datetime64 ['D '])
Z = np.arange('2016-07', '2016-08', dtype='datetime64[D]') print (Z)
35. How to calculate ((A+B)*(-A/2)) (without intermediate variables)? (★★☆)
(Tips: np.add(out=), np.negative(out=), np.multiply(out=), np.divide(out =))
A = np.ones(3) * 1 B = np.ones(3) * 1 C = np.ones(3) * 1 np.add(A, B, out=B) np.divide(A, 2, out=A) np.negative(A, out=A) np.multiply(A, B, out=A)
36. Five different methods are used to extract the integer part of the random array (★★☆)
(prompt:%, np.floor, np.ceil, astype, np.trunc)
Z = np.random.uniform(0, 10, 10) print (Z - Z % 1) print (np.floor(Z)) print (np.cell(Z)-1) print (Z.astype(int)) print (np.trunc(Z))
37. Create a 5x5 matrix, and the value range of each row is from 0 to 4 (★★☆)
(tip: NP. Orange)
Z = np.zeros((5, 5)) Z += np.arange(5) print (Z)
38. How to use a function to generate 10 integers to build an array (★☆)
(tip: np.fromiter)
def generate(): for x in range(10): yield x Z = np.fromiter(generate(), dtype=float, count=-1) print (Z)
39. Create a vector with a size of 10, with a value range of 0 to 1, excluding 0 and 1 (★★☆)
(tip: np.linspace)
Z = np.linspace(0, 1, 12, endpoint=True)[1: -1] print (Z)
40. Create a random vector of size 10 and sort it (★★☆)
(prompt: sort)
Z = np.random.random(10) Z.sort() print (Z)
41. Is there any way to sum a small array faster than np.sum? (★★☆)
(prompt: np.add.reduce)
# Author: Evgeni Burovski Z = np.arange(10) np.add.reduce(Z) # np.add.reduce is a ufunc(universal function) function in numpy.add module, which is implemented in C language
42. How to judge whether two arrays are equal to random arrays (★★☆)
(prompt: np.allclose, np.array_equal)
A = np.random.randint(0, 2, 5) B = np.random.randint(0, 2, 5) # Assume that the shape of the array is the same and an error tolerance equal = np.allclose(A,B) print(equal) # Check the shape and element values, and there is no error tolerance (the values must be exactly equal) equal = np.array_equal(A,B) print(equal)
43. Change the array to read-only (★★☆)
(prompt: flags.writeable)
Z = np.zeros(5) Z.flags.writeable = False Z[0] = 1
44. Convert a 10x2 Cartesian coordinate matrix into polar coordinates (★★☆)
(tip: np.sqrt, np.arctan2)
Z = np.random.random((10, 2)) X, Y = Z[:, 0], Z[:, 1] R = np.sqrt(X**2 + Y**2) T = np.arctan2(Y, X) print (R) print (T)
45. Create a random vector of size 10 and replace the largest value in the vector with 0 (★★☆)
(prompt: argmax)
Z = np.random.random(10) Z[Z.argmax()] = 0 print (Z)
46. Create a structured array where the X and y coordinates cover the [0,1] x [1,0] area (★★☆)
(tip: np.meshgrid)
Z = np.zeros((5, 5), [('x', float), ('y', float)]) Z['x'], Z['y'] = np.meshgrid(np.linspace(0, 1, 5), np.linspace(0, 1, 5)) print (Z)
47. Given two arrays X and Y, construct Cauchy matrix C(
C_{ij}=\frac{1}{x_i-y_j}) (★★☆)
(prompt: np.subtract.outer)
# Author: Evgeni Burovski X = np.arange(8) Y = X + 0.5 C = 1.0 / np.subtract.outer(X, Y) print (C) print(np.linalg.det(C)) # Computational determinant
48. Print the minimum and maximum representable values of each numpy type (★★☆)
(Tips: np.iinfo, np.finfo, eps)
for dtype in [np.int8, np.int32, np.int64]: print(np.iinfo(dtype).min) print(np.iinfo(dtype).max) for dtype in [np.float32, np.float64]: print(np.finfo(dtype).min) print(np.finfo(dtype).max) print(np.finfo(dtype).eps)
49. How to print all the values in the array? (★★☆)
(tip: np.set_printoptions)
np.set_printoptions(threshold=np.nan) Z = np.zeros((16,16)) print(Z)
50. How to find the value close to the given scalar in the array? (★★☆)
(prompt: argmin)
Z = np.arange(100) v = np.random.uniform(0, 100) index = (np.abs(Z-v)).argmin() print(Z[index])
51. Create a structured array (★★☆) representing position (x, y) and color (r, g, b, a)
(prompt: dtype)
Z = np.zeros(10, [('position', [('x', float, 1), ('y', float, 1)]), ('color', [('r', float, 1), ('g', float, 1), ('b', float, 1)])]) print (Z)
52. Consider the random vector with the shape of (100, 2) and calculate the distance between points (★★☆)
(tip: np.atleast_2d, T, np.sqrt)
Z = np.random.random((100, 2)) X, Y = np.atleast_2d(Z[:, 0], Z[:, 1]) D = np.sqrt((X-X.T)**2 + (Y-Y.T)**2) print (D) # Using the scipy library can be faster import scipy.spatial Z = np.random.random((100,2)) D = scipy.spatial.distance.cdist(Z,Z) print(D)
53. How to convert an array type of float(32-bit) to integer(32-bit)? (★★☆)
(prompt: asttype (copy = false))
Z = np.arange(10, dtype=np.int32) Z = Z.astype(np.float32, copy=False) print(Z)
54. How to read the following files? (★★☆)
(tip: NP. Genfromtext)
1, 2, 3, 4, 5 6, , , 7, 8 , , 9,10,11 # First save the above to the file example.txt # StringIO is not used here because there is a compatibility problem between Python 2 and python 3 Z = np.genfromtxt("example.txt", delimiter=",") print(Z)
55. Equivalent operation of numpy array enumeration? (★★☆)
(prompt: np.ndenumerate, np.ndindex)
Z = np.arange(9).reshape(3,3) for index, value in np.ndenumerate(Z): print(index, value) for index in np.ndindex(Z.shape): print(index, Z[index])
56. Construct a two-dimensional Gaussian matrix (★★☆)
(tip: np.meshgrid, np.exp)
X, Y = np.meshgrid(np.linspace(-1, 1, 10), np.linspace(-1, 1, 10)) D = np.sqrt(X**2 + Y**2) sigma, mu = 1.0, 0.0 G = np.exp(-( (D-mu)**2 / (2.0*sigma**2) )) print (G)
57. How to place p elements at random positions in a two-dimensional array? (★★☆)
(prompt: np.put, np.random.choice)
# Author: Divakar n = 10 p = 3 Z = np.zeros((n,n)) np.put(Z, np.random.choice(range(n*n), p, replace=False),1) print(Z)
58. Subtract the average value of each row of the matrix (★★☆)
(prompt: mean(axis=,keepdims =)
# Author: Warren Weckesser X = np.random.rand(5, 10) # new Y = X - X.mean(axis=1, keepdims=True) # used Y = X - X.mean(axis=1).reshape(-1, 1) print(Y)
59. How to sort an array by column n? (★★☆)
(prompt: argsort)
# Author: Steve Tjoa Z = np.random.randint(0,10,(3,3)) print(Z) print(Z[ Z[:,1].argsort() ])
60. How to judge that there are empty columns in a given two-dimensional array? (★★☆)
(prompt: any, ~)
# Author: Warren Weckesser Z = np.random.randint(0,3,(3,10)) print((~Z.any(axis=0)).any())
61. Find the value closest to the given value from the array (★★☆)
(prompt: np.abs, argmin, flat)
Z = np.random.uniform(0,1,10) z = 0.5 m = Z.flat[np.abs(Z - z).argmin()] print(m)
62. Think about two array shapes with shapes (1,3) and (3,1). How to use iterators to calculate their sum? (★★☆)
(tip: np.nditer)
A = np.arange(3).reshape(3, 1) B = np.arange(3).reshape(1, 3) it = np.nditer([A, B, None]) for x, y, z in it: z[...] = x + y print (it.operands[2])
63. Create an array class with name attribute (★★☆)
(prompt: class method)
class NameArray(np.ndarray): def __new__(cls, array, name="no name"): obj = np.asarray(array).view(cls) obj.name = name return obj def __array_finalize__(self, obj): if obj is None: return self.info = getattr(obj, 'name', "no name") Z = NamedArray(np.arange(10), "range_10") print (Z.name)
64. Given a vector, how to add 1 to each element of the second vector index (note the repeated index)? (★★★)
(prompt: np.bincount | np.add.at)
# Author: Brett Olsen Z = np.ones(10) I = np.random.randint(0,len(Z),20) Z += np.bincount(I, minlength=len(Z)) print(Z) # Another solution # Author: Bartosz Telenczuk np.add.at(Z, I, 1) print(Z)
65. How to accumulate the elements of vector X to array F according to index list I? (★★★)
(prompt: np.bincount)
# Author: Alan G Isaac X = [1,2,3,4,5,6] I = [1,3,9,3,4,1] F = np.bincount(I,X) print(F)
66. Think about the (w, h, 3) image of (dtype = ubyte) and calculate the value of unique color (★★★)
(tip: np.unique)
# Author: Nadav Horesh w,h = 16,16 I = np.random.randint(0,2,(h,w,3)).astype(np.ubyte) F = I[...,0]*256*256 + I[...,1]*256 +I[...,2] n = len(np.unique(F)) print(np.unique(I))
67. Think about how to find the data sum of the last two axes of a four-dimensional array (★★★)
(prompt: sum(axis=(-2,-1)))
A = np.random.randint(0,10,(3,4,3,4)) # Pass a tuple (numpy 1.7.0) sum = A.sum(axis=(-2,-1)) print(sum) # Compress the last two dimensions into one # (for functions that do not accept axis tuple parameters) sum = A.reshape(A.shape[:-2] + (-1,)).sum(axis=-1) print(sum)
68. Considering one-dimensional vector D, how to use vector S of the same size to calculate the mean of the subset of D and describe the subset index? (★★★)
(prompt: np.bincount)
# Author: Jaime Fernández del Río D = np.random.uniform(0,1,100) S = np.random.randint(0,10,100) D_sums = np.bincount(S, weights=D) D_counts = np.bincount(S) D_means = D_sums / D_counts print(D_means) # Pandas solution as a reference due to more intuitive code import pandas as pd print(pd.Series(D).groupby(S).mean())
69. How to get the diagonal of dot product? (★★★)
(tip: np.diag)
# Author: Mathieu Blondel A = np.random.uniform(0,1,(5,5)) B = np.random.uniform(0,1,(5,5)) # Slow version np.diag(np.dot(A, B)) # Fast version np.sum(A * B.T, axis=1) # Faster version np.einsum("ij,ji->i", A, B)
70. Considering the vector [1,2,3,4,5], how to establish a new vector with three consecutive zeros interleaved between each value? (★★★)
(hint: array[::4])
# Author: Warren Weckesser Z = np.array([1,2,3,4,5]) nz = 3 Z0 = np.zeros(len(Z) + (len(Z)-1)*(nz)) Z0[::nz+1] = Z print(Z0)
71. Consider an array of dimensions (5,5,3). How to multiply it by an array of dimensions (5,5)? (★★★)
(prompt: array [:,:, none])
A = np.ones((5,5,3)) B = 2*np.ones((5,5)) print(A * B[:,:,None])
72. How to exchange any two rows in an array? (★★★)
(prompt: array[[]] = array [[]])
# Author: Eelco Hoogendoorn A = np.arange(25).reshape(5,5) A[[0,1]] = A[[1,0]] print(A)
73. Think about a set of 10 triads describing 10 triangles (shared vertices) and find the unique set of line segments that make up all triangles (★★★)
(Tips: repeat, np.roll, np.sort, view, np.unique)
# Author: Nicolas P. Rougier faces = np.random.randint(0,100,(10,3)) F = np.roll(faces.repeat(2,axis=1),-1,axis=1) F = F.reshape(len(F)*3,2) F = np.sort(F,axis=1) G = F.view( dtype=[('p0',F.dtype),('p1',F.dtype)] ) G = np.unique(G) print(G)
74. Given A binary array C, how to generate an array A that satisfies np.bincount(A)==C? (★★★)
(tip: np.repeat)
# Author: Jaime Fernández del Río C = np.bincount([1,1,2,3,4,4,6]) A = np.repeat(np.arange(len(C)), C) print(A)
75. How to calculate the average of an array through a sliding window? (★★★)
(tip: np.cumsum)
# Author: Jaime Fernández del Río def moving_average(a, n=3) : ret = np.cumsum(a, dtype=float) ret[n:] = ret[n:] - ret[:-n] return ret[n - 1:] / n Z = np.arange(20) print(moving_average(Z, n=3))
76. Think about array Z and build a two-dimensional array. The first line is (Z[0],Z[1],Z[2]), then each line moves one bit, and the last line is (Z[-3],Z[-2],Z[-1]) (★★★★)
(prompt: from numpy.lib import stripe_tricks)
# Author: Joe Kington / Erik Rigtorp from numpy.lib import stride_tricks def rolling(a, window): shape = (a.size - window + 1, window) strides = (a.itemsize, a.itemsize) return stride_tricks.as_strided(a, shape=shape, strides=strides) Z = rolling(np.arange(10), 3) print(Z)
77. How to negate a Boolean value or change the sign of a floating-point number? (★★★)
(prompt: np.logical_not, np.negative)
# Author: Nathaniel J. Smith Z = np.random.randint(0,2,100) np.logical_not(Z, out=Z) Z = np.random.uniform(-1.0,1.0,100) np.negative(Z, out=Z)
78. Consider two sets of point sets P0 and P1 to describe a set of lines (two-dimensional) and a point p. how to calculate the distance from point p to each line i (P0[i],P1[i])? (★★★)
def distance(P0, P1, p): T = P1 - P0 L = (T**2).sum(axis=1) U = -((P0[:,0]-p[...,0])*T[:,0] + (P0[:,1]-p[...,1])*T[:,1]) / L U = U.reshape(len(U),1) D = P0 + U*T - p return np.sqrt((D**2).sum(axis=1)) P0 = np.random.uniform(-10,10,(10,2)) P1 = np.random.uniform(-10,10,(10,2)) p = np.random.uniform(-10,10,( 1,2)) print(distance(P0, P1, p))
79. Considering two sets of point sets P0 and P1 to describe a set of lines (two-dimensional) and a set of point sets P, how to calculate the distance from each point j(P[j]) to each line i (P0[i],P1[i])? (★★★)
# Author: Italmassov Kuanysh # based on distance function from previous question P0 = np.random.uniform(-10, 10, (10,2)) P1 = np.random.uniform(-10,10,(10,2)) p = np.random.uniform(-10, 10, (10,2)) print(np.array([distance(P0,P1,p_i) for p_i in p]))
80. Think about an arbitrary array and write a function that extracts a sub part with a fixed shape and centers on a given element (fill the value in this part) (★★★★)
(prompt: minimum, maximum)
# Author: Nicolas Rougier Z = np.random.randint(0,10,(10,10)) shape = (5,5) fill = 0 position = (1,1) R = np.ones(shape, dtype=Z.dtype)*fill P = np.array(list(position)).astype(int) Rs = np.array(list(R.shape)).astype(int) Zs = np.array(list(Z.shape)).astype(int) R_start = np.zeros((len(shape),)).astype(int) R_stop = np.array(list(shape)).astype(int) Z_start = (P-Rs//2) Z_stop = (P+Rs//2)+Rs%2 R_start = (R_start - np.minimum(Z_start,0)).tolist() Z_start = (np.maximum(Z_start,0)).tolist() R_stop = np.maximum(R_start, (R_stop - np.maximum(Z_stop-Zs,0))).tolist() Z_stop = (np.minimum(Z_stop,Zs)).tolist() r = [slice(start,stop) for start,stop in zip(R_start,R_stop)] z = [slice(start,stop) for start,stop in zip(Z_start,Z_stop)] R[r] = Z[z] print(Z) print(R)
81. Consider an array Z = [1,2,3,4,5,6,7,8,9,10,11,12,13,14], how to generate an array R = [[1,2,3,4], [2,3,4,5], [3,4,5,6],..., [11,12,13,14]]? (★★★)
(prompt: stripe_tricks. As_striped)
# Author: Stefan van der Walt Z = np.arange(1,15,dtype=np.uint32) R = stride_tricks.as_strided(Z,(11,4),(4,4)) print(R)
82. Calculate the rank of the matrix (★★★)
(tip: np.linalg.svd)
# Author: Stefan van der Walt Z = np.random.uniform(0,1,(10,10)) U, S, V = np.linalg.svd(Z) # Singular Value Decomposition rank = np.sum(S > 1e-10) print(rank)
83. How to find the most frequent value in the array? (★★★)
(prompt: np.bincount, argmax)
Z = np.random.randint(0,10,50) print(np.bincount(Z).argmax())
84. Extract continuous 3x3 blocks (★★★) from a 10x10 matrix
(prompt: stripe_tricks. As_striped)
# Author: Chris Barker Z = np.random.randint(0,5,(10,10)) n = 3 i = 1 + (Z.shape[0]-3) j = 1 + (Z.shape[1]-3) C = stride_tricks.as_strided(Z, shape=(i, j, n, n), strides=Z.strides + Z.strides) print(C)
85. Create a two-dimensional array subclass that satisfies Z[i,j] == Z[j,i] (★★★)
(prompt: class method)
# Author: Eric O. Lebigot # Note: only works for 2d array and value setting using indices class Symetric(np.ndarray): def __setitem__(self, index, value): i,j = index super(Symetric, self).__setitem__((i,j), value) super(Symetric, self).__setitem__((j,i), value) def symetric(Z): return np.asarray(Z + Z.T - np.diag(Z.diagonal())).view(Symetric) S = symetric(np.random.randint(0,10,(5,5))) S[2,3] = 42 print(S)
86. Considering p nxn matrices and a set of vectors with shape (n,1), how to directly calculate the product (n,1) of p matrices? (★★★)
(tip: np.tensordot)
# Author: Stefan van der Walt p, n = 10, 20 M = np.ones((p,n,n)) V = np.ones((p,n,1)) S = np.tensordot(M, V, axes=[[0, 2], [0, 1]]) print(S) # It works, because: # M is (p,n,n) # V is (p,n,1) # Thus, summing over the paired axes 0 and 0 (of M and V independently), # and 2 and 1, to remain with a (n,1) vector.
87. For a 16x16 array, how to get the sum of a region (the region size is 4x4)? (★★★)
(prompt: np.add.reduceat)
# Author: Robert Kern Z = np.ones((16,16)) k = 4 S = np.add.reduceat(np.add.reduceat(Z, np.arange(0, Z.shape[0], k), axis=0), np.arange(0, Z.shape[1], k), axis=1) print(S)
88. How to use numpy array to realize Game of Life? (★★★)
(tip: game of life, what graphics does game of life have?)
# Author: Nicolas Rougier def iterate(Z): # Count neighbours N = (Z[0:-2,0:-2] + Z[0:-2,1:-1] + Z[0:-2,2:] + Z[1:-1,0:-2] + Z[1:-1,2:] + Z[2: ,0:-2] + Z[2: ,1:-1] + Z[2: ,2:]) # Apply rules birth = (N==3) & (Z[1:-1,1:-1]==0) survive = ((N==2) | (N==3)) & (Z[1:-1,1:-1]==1) Z[...] = 0 Z[1:-1,1:-1][birth | survive] = 1 return Z Z = np.random.randint(0,2,(50,50)) for i in range(100): Z = iterate(Z) print(Z)
89. How to find the nth maximum value of an array? (★★★)
(prompt: np.argsort | np.argpartition)
Z = np.arange(10000) np.random.shuffle(Z) n = 5 # Slow print (Z[np.argsort(Z)[-n:]]) # Fast print (Z[np.argpartition(-Z,n)[:n]])
90. Given any number vector, create Cartesian product (each combination of each element) (★★★)
(tip: np.indices)
# Author: Stefan Van der Walt def cartesian(arrays): arrays = [np.asarray(a) for a in arrays] shape = (len(x) for x in arrays) ix = np.indices(shape, dtype=int) ix = ix.reshape(len(arrays), -1).T for n, arr in enumerate(arrays): ix[:, n] = arrays[n][ix[:, n]] return ix print (cartesian(([1, 2, 3], [4, 5], [6, 7])))
91. How to create a record array from a regular array? (★★★)
(tip: np.core.records.fromarrays)
Z = np.array([("Hello", 2.5, 3), ("World", 3.6, 2)]) R = np.core.records.fromarrays(Z.T, names='col1, col2, col3', formats = 'S8, f8, i8') print(R)
92. Think about a large vector Z and calculate its cube in three different ways (★★★)
(Tips: np.power, *, np.einsum)
# Author: Ryan G. x = np.random.rand(5e7) %timeit np.power(x,3) %timeit x*x*x %timeit np.einsum('i,i,i->i',x,x,x)
93. Consider two arrays A and B with shapes (8,3) and (2,2) respectively. How to find A row in array A that satisfies the elements in B? (regardless of the order of elements in each line in B)? (★★★)
(tip: np.where)
# Author: Gabe Schwartz A = np.random.randint(0,5,(8,3)) B = np.random.randint(0,5,(2,2)) C = (A[..., np.newaxis, np.newaxis] == B) rows = np.where(C.any((3,1)).all(1))[0] print(rows)
94. Think about a 10x3 matrix and how to decompose rows with different values (such as [2,2,3]) (★★★★)
# Author: Robert Kern Z = np.random.randint(0,5,(10,3)) print(Z) # solution for arrays of all dtypes (including string arrays and record arrays) E = np.all(Z[:,1:] == Z[:,:-1], axis=1) U = Z[~E] print(U) # soluiton for numerical arrays only, will work for any number of columns in Z U = Z[Z.max(axis=1) != Z.min(axis=1),:] print(U)
95. Convert an integer vector to a binary matrix (★★★)
(tip: np.unpackbits)
# Author: Warren Weckesser I = np.array([0, 1, 2, 3, 15, 16, 32, 64, 128]) B = ((I.reshape(-1,1) & (2**np.arange(8))) != 0).astype(int) print(B[:,::-1]) # Author: Daniel T. McDonald I = np.array([0, 1, 2, 3, 15, 16, 32, 64, 128], dtype=np.uint8) print(np.unpackbits(I[:, np.newaxis], axis=1))
96. Given a two-dimensional array, how to extract unique rows? (★★★)
(tip: np.ascontiguousarray)
# Author: Jaime Fernández del Río Z = np.random.randint(0,2,(6,3)) T = np.ascontiguousarray(Z).view(np.dtype((np.void, Z.dtype.itemsize * Z.shape[1]))) _, idx = np.unique(T, return_index=True) uZ = Z[idx] print(uZ)
97. Consider two vectors A and B and write the inner, outer, sum and mul functions corresponding to the einsum equation (★★★★)
(tip: np.einsum)
# Author: Alex Riley # Make sure to read: http://ajcr.net/Basic-guide-to-einsum/ A = np.random.uniform(0,1,10) B = np.random.uniform(0,1,10) np.einsum('i->', A) # np.sum(A) np.einsum('i,i->i', A, B) # A * B np.einsum('i,i', A, B) # np.inner(A, B) np.einsum('i,j->ij', A, B) # np.outer(A, B)
98. Considering a path (X,Y) described by two vectors, how to sample it with equidistance samples (★★★)?
(prompt: np.cumsum, np.interp)
# Author: Bas Swinckels phi = np.arange(0, 10*np.pi, 0.1) a = 1 x = a*phi*np.cos(phi) y = a*phi*np.sin(phi) dr = (np.diff(x)**2 + np.diff(y)**2)**.5 # segment lengths r = np.zeros_like(x) r[1:] = np.cumsum(dr) # integrate path r_int = np.linspace(0, r.max(), 200) # regular spaced path x_int = np.interp(r_int, r, x) # integrate path y_int = np.interp(r_int, r, y)
99. Given an integer n and a two-dimensional array x, select multiple distributed rows that can be interpreted as multiple n degrees from X, that is, these rows only contain the sum of integer pairs n. (★★★)
(prompt: np.logical_and.reduce, np.mod)
# Author: Evgeni Burovski X = np.asarray([[1.0, 0.0, 3.0, 8.0], [2.0, 0.0, 1.0, 1.0], [1.5, 2.5, 1.0, 0.0]]) n = 4 M = np.logical_and.reduce(np.mod(X, 1) == 0, axis=-1) M &= (X.sum(axis=-1) == n) print(X[M])
100. For a one-dimensional array X, calculate the average value of the 95% confidence interval after it is bootstrapped. (★★★)
(tip: np.percentile)
# Author: Jessica B. Hamrick X = np.random.randn(100) # random 1D array N = 1000 # number of bootstrap samples idx = np.random.randint(0, X.size, (N, X.size)) means = X[idx].mean(axis=1) confint = np.percentile(means, [2.5, 97.5]) print(confint)