With the help of the authentication service, cloud function, SMS service and other services of AppGallery Connect (hereinafter referred to as AGC), when the user registers successfully, he can receive an application welcome SMS or welcome email in the registered mobile phone number or email address. So that developers can integrate into the application faster and know the hot content of the application at the first time.
Implementation process
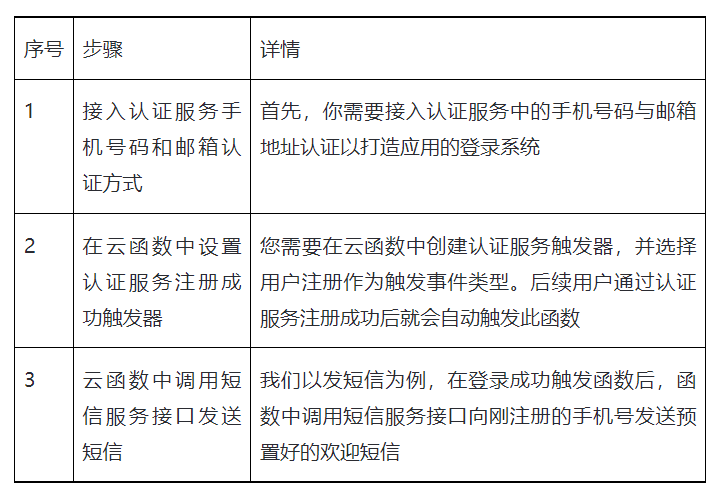
Access authentication service mobile phone number and email authentication method
First of all, we need to build an application account system by accessing authentication services.
Enable authentication service
1. Login AppGallery Connect Website, click "my project".
2. Click your item in the item list. Select build > authentication service,
3. Enter the authentication service page and complete the following operations:
a. Open authentication service
b. Enable mobile number and email address authentication
Develop mobile phone number authentication method
Since the development process of email address authentication is highly similar to that of mobile phone number authentication, we will take mobile phone number authentication as an example.
1. First, we need to call the sendVerifyCode method to obtain the verification code for registration:
public void sendPhoneVerify(String accountNumber) { String countryCode = "86"; VerifyCodeSettings settings = VerifyCodeSettings.newBuilder() .action(VerifyCodeSettings.ACTION_REGISTER_LOGIN) .sendInterval(30) .locale(Locale.SIMPLIFIED_CHINESE) .build(); if (notEmptyString(countryCode) && notEmptyString(accountNumber)) { Task<VerifyCodeResult> task = PhoneAuthProvider.requestVerifyCode(countryCode, accountNumber, settings); task.addOnSuccessListener(TaskExecutors.uiThread(), verifyCodeResult -> { mAuthReCallBack.onSendVerify(verifyCodeResult); }).addOnFailureListener(TaskExecutors.uiThread(), e -> { Log.e(TAG, "requestVerifyCode fail:" + e.getMessage()); mAuthReCallBack.onFailed(e.getMessage()); }); } else { Log.w(TAG, "info empty"); } }
2. Then we call the createUser method to register the user
public void registerPhoneUser(String accountNumber, String verifyCode, String password) { String countryCode = "86"; PhoneUser phoneUser = new PhoneUser.Builder() .setCountryCode(countryCode) .setPhoneNumber(accountNumber) .setVerifyCode(verifyCode) .setPassword(password) .build(); AGConnectAuth.getInstance().createUser(phoneUser) .addOnSuccessListener(signInResult -> { mAuthReCallBack.onAuthSuccess(signInResult, 11); }).addOnFailureListener(e -> { mAuthReCallBack.onFailed(e.getMessage()); }); }
3. For registered users, we can call the sign method to log in
public void phoneLogin(String phoneAccount, String photoPassword) { String countryCode = "86"; AGConnectAuthCredential credential = PhoneAuthProvider.credentialWithVerifyCode( countryCode, phoneAccount, photoPassword, null); AGConnectAuth.getInstance().signIn(credential).addOnSuccessListener(signInResult -> { Log.i(TAG, "phoneLogin success"); mAuthLoginCallBack.onAuthSuccess(signInResult, 11); signInResult.getUser().getToken(true).addOnSuccessListener(tokenResult -> { String token = tokenResult.getToken(); Log.i(TAG, "getToken success: " + token); mAuthLoginCallBack.onAuthToken(token); }); }).addOnFailureListener(e -> { Log.e(TAG, "Login failed: " + e.getMessage()); mAuthLoginCallBack.onAuthFailed(e.getMessage()); }); }
Set the authentication service registration success trigger in the cloud function
After the above operations are completed, you need to configure the authentication service trigger in the cloud function.
1. Login AppGallery Connect Website, click "my project".
2. Click your item in the item list. Select build > cloud function, enter the cloud function page, and complete the following operations:
a. Enable cloud function service.
b. Create a function to send a welcome message (described in detail in the next chapter)
c. Upload the function that sends the welcome message to the cloud function.
d. Create authentication service trigger : select "user registration" for the event name.
Calling SMS service interface to send SMS in cloud function
After the user is registered successfully, a welcome message needs to be sent to the user. Here, we use the message provided by AGC SMS service send out.
Open SMS service and set SMS template
Sign in AppGallery Connect Website, click "my project".
1. Click your item in the item list.
2. Select "growth > SMS service", enter the SMS service page, and complete the following operations:
a. Open SMS service
b. Configure SMS signature
c. Configure SMS template
d. Enable API calls
The cloud function calls the SMS service Rest Api interface to send SMS
1. Obtain the user's mobile phone number and user information through the trigger
var phoneNumber = event.phone.slice(4); var userID = event.uid; var userName = "Authenticated user ID" + phoneNumber.slice(11);
2. Call SMS service Rest Api to send SMS
var requestData = { "account": "AGC199", "password":"Huawei1234567890!", "requestLists": [ { "mobiles":["" + phoneNumber], "templateId":"SMS02_21090100001", "messageId":"12345", "signature":"[PhotoPlaza]" } ], "requestId": "" + curTime }; logger.info("requestData: " + JSON.stringify(requestData)); var options = { hostname: '121.37.23.38', port: 18312, path: '/common/sms/sendTemplateMessage', method: 'POST', headers: { 'Content-Type' : 'application/json' }, rejectUnauthorized: false, requestCert: false }; var req = https.request(options, function(res) { res.on('data', function(data) { var response = JSON.parse(data.toString()); logger.info('All resultList: ' + JSON.stringify(response.resultLists)); }); res.on('end', function(){ logger.info('RequestResult: success'); let result = {"message":"Send Message Success"}; callback(result); }); res.on('error', function(e) { logger.info('request error, ' + e.message); let result = {"message":"error: " + e.message} callback(result); }); }); req.on('error', function(error) { logger.info('request error, ' + error.message); let result = {"message":"error: " + e.message} callback(result); }); req.write(JSON.stringify(requestData)); req.end();