Experimental task 1
Task 1-1
assume ds:data, cs:code, ss:stack data segment db 16 dup(0) data ends stack segment db 16 dup(0) stack ends code segment start: mov ax, data mov ds, ax mov ax, stack mov ss, ax mov sp, 16 mov ah, 4ch int 21h code ends end start
task1_1 screenshot before the end of line17 and line19
① In debug, execute until the end of line17 and before line19. Record this time: register (DS) =_ 076A_, Register (SS)=__ 076B__, Register (CS)=_ 076C_.
② Assuming that the segment address of the code segment is X after the program is loaded, the segment address of the data segment is X__ X-2__, The segment address of stack is__ X-1__ .
Task 1-2
Task task1_2.asm source code
assume ds:data, cs:code, ss:stack data segment db 4 dup(0) ; Four byte units are reserved, and the initial value is 0 data ends stack segment db 8 dup(0) ; 8 byte units are reserved, and the initial values are 0 stack ends code segment start: mov ax, data mov ds, ax mov ax, stack mov ss, ax mov sp, 8 ; Set stack top mov ah, 4ch int 21h code ends end start
task1_2. After debugging to the end of line17 and before line19, observe the screenshot of register DS, CS and SS values
① In debug, execute until the end of line17 and before line19. Record this time: register (DS) =__ 076A__, Register (SS)=_ 076B__, Register (CS) =__ 076C__
② Assuming that the segment address of the code segment is X after the program is loaded, the segment address of the data segment is X__ X-2__, The segment address of stack is__ X-1__
Task 1-3
Task task1_3.asm source code
assume ds:data, cs:code, ss:stack data segment db 20 dup(0) ; 20 byte units are reserved, and the initial values are 0 data ends stack segment db 20 dup(0) ; 20 byte units are reserved, and the initial values are 0 stack ends code segment start: mov ax, data mov ds, ax mov ax, stack mov ss, ax mov sp, 20 ; Set initial stack top mov ah, 4ch int 21h code ends end start
task1_3. Screenshot of the values of registers DS, CS and SS before the end of debugging to line17 and line19
① In debug, execute until the end of line17 and before line19. Record this time: register (DS) =__ 076A_, Register (SS)=__ 076C__, Register (CS) =__ 076E__
② Assuming that the segment address of the code segment is X after the program is loaded, the segment address of the data segment is X__ X-4__, The segment address of stack is__ X-2__.
Tasks 1-4
Source code:
assume ds:data, cs:code, ss:stack code segment start: mov ax, data mov ds, ax mov ax, stack mov ss, ax mov sp, 20 mov ah, 4ch int 21h code ends data segment db 20 dup(0) data ends stack segment db 20 dup(0) stack ends end start
① In debug, execute until the end of line9 and before line11. Record this time: register (DS) =__ 076C__, Register (SS)=__ 076E__, Register (CS) =_ 076A_
② Assuming that the segment address of the code segment is X after the program is loaded, the segment address of the data segment is X__ X+2__, The segment address of stack is_ X+4__.
Tasks 1-5
① For the segment defined below, after the program is loaded, the actual memory space allocated to the segment is 16*ceil(N/16) .
② task1_4.asm can still be executed correctly, because the entry of the original program is described after end. If it is empty, the program will be executed from scratch, only Task1_ The beginning of 4 is the content of the code segment.
Experimental task 2
Source code:
assume cs:code code segment mov ax,0b800h mov ds,ax mov bx,0f00h mov cx,160 s: mov ax,0304h mov [bx],al inc bx loop s mov ax,4c00h int 21h code ends end
Screenshot of operation results
Experimental task 3
assume cs:code data1 segment db 50, 48, 50, 50, 0, 48, 49, 0, 48, 49 ; ten numbers data1 ends data2 segment db 0, 0, 0, 0, 47, 0, 0, 47, 0, 0 ; ten numbers data2 ends data3 segment db 16 dup(0) data3 ends code segment start: mov ax,data3 mov ds,ax mov ax,data1 mov es,ax mov cx,10 mov bx,0 s1: mov al,es:[bx] add [bx],al inc bx loop s1 mov ax,data2 mov es,ax mov cx,10 mov bx,0 s2: mov al,es:[bx] add [bx],al inc bx loop s2 mov ah,41h int 21h code ends end start
Screenshot of loading, disassembling and debugging in debug:
After the data items are added in turn, the debug command and screenshot of the original value of memory space data corresponding to logical segments data1, data2 and data3:
Experiment task 4:
Complete source code
assume cs:code data1 segment dw 2, 0, 4, 9, 2, 0, 1, 9 data1 ends data2 segment dw 8 dup(?) data2 ends code segment start: mov ax,data1 mov ds,ax mov ax,data2 mov ss,ax mov sp,16 mov cx,8 mov bx,0 s: push [bx] add bx,2 loop s mov ah, 4ch int 21h code ends end start
Before and after execution
Experimental task 5
Source code:
assume cs:code, ds:data data segment db 'Nuist' db 5 dup(5) data ends code segment start: mov ax, data mov ds, ax mov ax, 0b800H mov es, ax mov cx, 5 mov si, 0 mov di, 0f00h s: mov al, [si] and al, 0dfh mov es:[di], al mov al, [5+si] mov es:[di+1], al inc si add di, 2 loop s mov ah, 4ch int 21h code ends end start
Screenshot of operation results:
Use the debug tool to debug the program, and use the g command to execute it once before the program returns (i.e. after ine25 and before line27):
What is the function of line19 in the source code?
A: if the lower address is a lowercase letter, turn it into an uppercase letter. By bitwise and, turn the fifth bit of the binary Ascii code corresponding to the lowercase letter into 0, that is, subtract 32 from the Ascii value to become an uppercase letter.
What is the purpose of the byte data in the data segment line4 in the source code?
A: set the color of the word.
Experimental task 6
assume cs:code, ds:data data segment db 'Pink Floyd ' db 'JOAN Baez ' db 'NEIL Young ' db 'Joan Lennon ' data ends code segment start: mov ax,data mov ds,ax mov cx,4 mov bx,0 s: mov dx,[bx] mov ax,dx add al,32 mov [bx],ax add bx,16 loop s mov ah, 4ch int 21h code ends end start
Load, disassemble and debug screenshots in debug
Experimental task 7
assume cs:code, ds:data, es:table data segment db '1975', '1976', '1977', '1978', '1979' ;20 bytes per line dw 16, 22, 382, 1356, 2390 dw 3, 7, 9, 13, 28 data ends table segment db 5 dup( 16 dup(' ') ) ; table ends code segment start: mov ax,data mov ds,ax mov ax,table mov es,ax mov bx,0 mov si,0 mov bp,0 mov di,0 mov cx,5 s: mov ax,[bx+si] ;1975 mov es:[bp+di],ax add si,2 add di,2 mov ax,[bx+si] mov es:[bp+di],ax mov ax,0 ;[sp]0016[sp] add ax,cx add ax,cx add ax,8 add si,ax ;Here to implement y=8+2n To track this specific si How much,among n by cx Value of add di,2 mov ax,[bx+si] ;ax Store 16 bit divisor mov dl,32 mov dh,00h mov es:[bp+di],dx add di,2 mov dl,00h mov dh,al mov es:[bp+di],dx add di,2 mov dl,ah mov dh,32 mov es:[bp+di],dx add si,10 add di,2 mov dx,[bx+si] ;dl Stored divisor mov es:[bp+di],dx add di,2 div dl ;al Depositors, ah Deposit remainder mov dl,32 mov dh,al mov es:[bp+di],dx add di,2 mov dl,00h mov dh,32 mov es:[bp+di],dx add bx,4 ;Next cycle initialization add bp,16 mov si,0 mov di,0 loop s mov ah, 4ch int 21h code ends end start
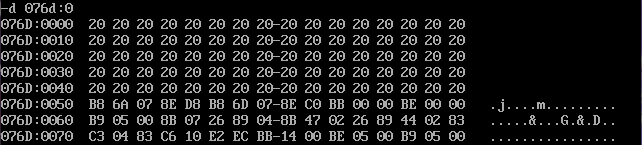
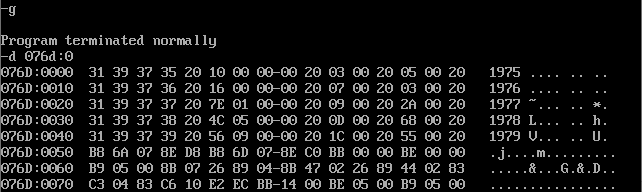