Foreword: in this article, we learn the definition and use of arrays. We have learned some basic contents of Java before, and then today we will learn arrays in Java.
Each picture:
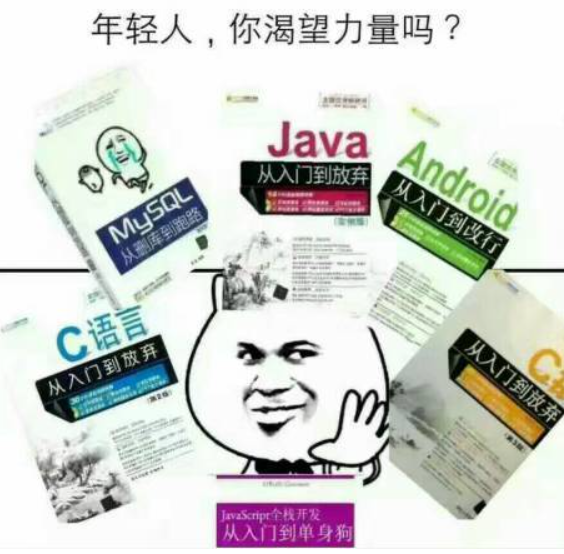
Let's go into the study of arrays ba!
1, Basic usage of array
To understand the basic usage of arrays, first understand: what is an array? In fact, array essentially allows us to "batch" create variables of the same type, and then combine them as a combination.
For example:
When we need to represent two data, we can create two variables int a; int b when we need to represent five data, we can create five variables int a1;int a2; int a3; int a4; int a5;
But if you need to represent 10000 data, you can't create 10000 variables (of course, if you have enough liver). At this time, we need to use arrays to help us create data in batches.
So array is to make it more convenient for us to create a large amount of the same data, and we can first some continuity functions. Here is another note: in Java, the variables contained in the array must be of the same type!
Let's go to the basic usage of arrays >
1. Create an array
First, let's learn the basic syntax for creating arrays:
Basic syntax:
dynamic initialization
Data type [] array name = new data type [] {initialization data};
initiate static
Data type [] array name = {initialization data};
New here is actually a keyword that instantiates an object, which means that the array in Java is also an object. Although there is no new in static initialization, it is also an object.
Code example:
public static void main(String[] args) { int [] array1 = {1,2,3,4,5};//initiate static int [] array2 = new int[5];//Uninitialized array int [] array3 = new int[]{1,2,3,4,5};//dynamic initialization }
matters needing attention:
1. When defining an array, you cannot write a specific array, such as int [10] array = {};
2. During static initialization, the number of array elements is consistent with the format of initialization data. For example, we define int [] array1, and the type of data in initialization should be integer. Floating point type and other types cannot appear
For array initialization, this is actually the case:
When we create an array and initialize it, we will get an array like this. Five elements are stored in the array. Each element has a corresponding subscript. The subscript starts from 0. For example, array[0] is 1 here. For array definitions that are not initialized, the default value of the elements in them is 0.
This is the creation of the array.
2. Use of arrays
1. Get length & access element
As we said above, for the created element, each element has its corresponding subscript, so that we can better access this element. At the same time, after creating the array, we can obtain the length of the array through array +. Length, that is, how many elements there are.
Let's go directly to the code:
public static void main(String[] args) { int [] array1 = {1,2,3,4,5}; int [] array2 = new int[5]; // Get array length System.out.println("length: " + array1.length); // Execution result: 5 // Accessing elements in an array System.out.println(array1[0]); // Execution result: 1 System.out.println(array1[1]); // Execution result: 2 array2[0] = 100; System.out.println(array2[0]); // Execution result: 100 } }
As in the above code, we can use array1.length to obtain the length of array array1. Then, when we want to know the value of which element, we can access this element through its subscript. For example, array1[0] is the first element in array1.
Then we create an uninitialized array array2, and we can assign a value to it, such as array2[0] = 100; The value is then stored in the first element corresponding to the 0 subscript of array2. Similarly, if we add array2[0] = 10;, The 0 subscript of array2 corresponds to 10, because 100 has been overwritten.
Finally, when accessing our array subscript, if we define 5 elements, the subscript should be 0-4, so we can only access the elements with 0-4 subscript, not those with 5 subscript, such as array1[5]=0 //err. It's equivalent to buying only the four floors of the house. We don't have the key to the fifth floor.
So here we summarize some precautions:
1. The length of the array can be obtained by using arr.length. This operation is a member access operator, which will be often used in object-oriented later.
2. Press [] to mark the array elements. Note that the subscript counts from 0!
3. Use [] to read and modify data.
4. The subscript access operation cannot exceed the valid range [0, length - 1]. If it exceeds the valid range, the subscript out of range exception will occur.
Here are some examples:
1. Subscript out of bounds (breaking into other people's houses without keys)
public static void main(String[] args) { int[] arr = {1, 2, 3}; System.out.println(arr[100]); // results of enforcement //Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 100 //at Test.main(Test.java:4) }
Good guy, I bought three floors and wanted to go to the 100th floor? That certainly won't work, so our operation result is an error. We often encounter errors when writing code. We need to understand the errors in order to learn better. Here is actually ArrayIndexOut, that is, the array is out of bounds.
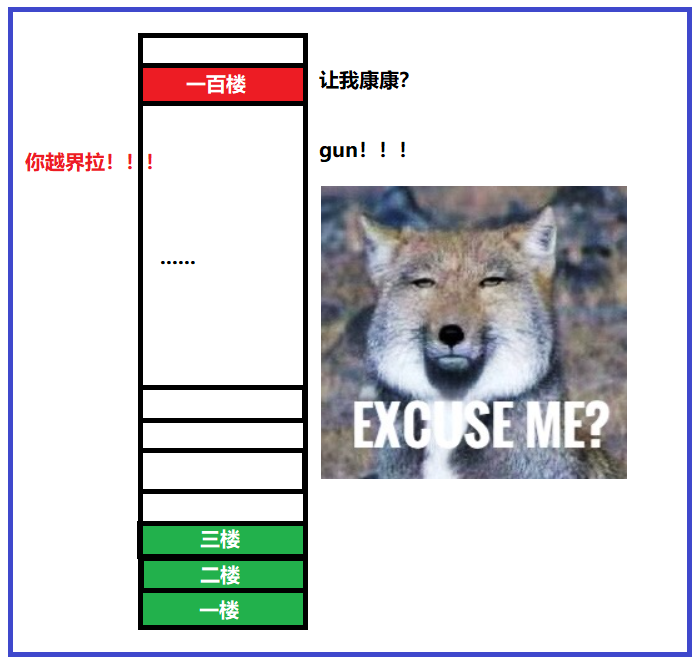
2. Traverse the array (see all my houses)
In fact, the so-called "traversal" refers to accessing all elements in the array without repetition or leakage. We usually need to match loop statements. Here we will match a wave of for loops.
public static void main(String[] args) { int[] arr = {1, 2, 3, 4, 5}; for (int i = 0; i < arr.length; i++) { System.out.println(arr[i]); } //arr.length indicates the length of the array. Print one at a time and print as many times as you want }
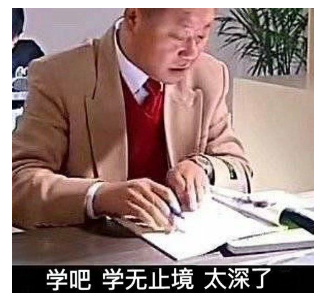
3. Use for each to traverse the array
For the for loop, there is also for each, which is written here because it is closely related to the array. For each is another way to use the for loop, which can more conveniently complete the traversal of the array. You can avoid writing errors in loop conditions and update statements.
public static void main(String[] args) { int[] arr = {1, 2, 3}; for (int x : arr) { System.out.println(x); } //Traverse the array and print all //Advantages: no need to write cyclic update statements disadvantages: full printing, no subscript // }
This is the basic syntax of arrays.
2, Array as an argument to the method
In our last article, we learned methods and knew the benefits of methods. What kind of sparks will be generated when methods and arrays are together? Let's take a look:
1. Basic usage
The basic syntax is still traversal printing, but it is wrapped in the form of a method, so that we can call the method directly when we want to print, and we don't need to write it again.
public static void printArray(int[] a) { for (int x : a) { System.out.println(x);//Traversal printing } } public static void main(String[] args) { int[] arr = {1, 2, 3}; printArray(arr);//Call the method to print the array }
In this code, we need to pay attention to:
int[] a is the formal parameter of the function, and int[] arr is the function argument. The ARR of the passed parameter represents a reference to the array type. If you need to get the array length, you can also use a.length.
What is a reference? We'll see next.

2. Understand reference types (key / difficult points)
For reference types, we start from the code:
Look at the following code first:
public static void main(String[] args) { int num = 0; func(num); System.out.println("num = " + num); } public static void func(int x) { x = 10; System.out.println("x = " + x); } // Execution result x = 10 num = 0
We found that modifying the value of parameter x does not affect the num value of the argument.
Let's look at the version of the array:
public static void main(String[] args) { int[] arr = {1, 2, 3}; func(arr); System.out.println("arr[0] = " + arr[0]); } public static void func(int[] a) { a[0] = 10; System.out.println("a[0] = " + a[0]); } // results of enforcement // a[0] = 10 // arr[0] = 10
We found that when we modify the contents of the array inside the function, such as a[0], the arr[0] outside the function also changes. This is because the array name arr is a "reference". When parameters are passed, they are passed by reference!
For references:
Let's start with memory. We know that computers, mobile phones and other devices have memory. The memory here refers to the familiar "memory".
So how to understand memory?
Memory can be intuitively understood as a dormitory building with a long corridor and many rooms on it. The size of each room is 1 Byte (if the computer has 8G memory, it is equivalent to 8 billion such rooms). Each room has a house number, which is called the address.
So what is a quote?
A reference is equivalent to an "alias" and can also be understood as a pointer (a pointer to this address). Creating a reference is equivalent to creating a small variable that holds an integer representing an address in memory.
Let's use specific diagrams to demonstrate:
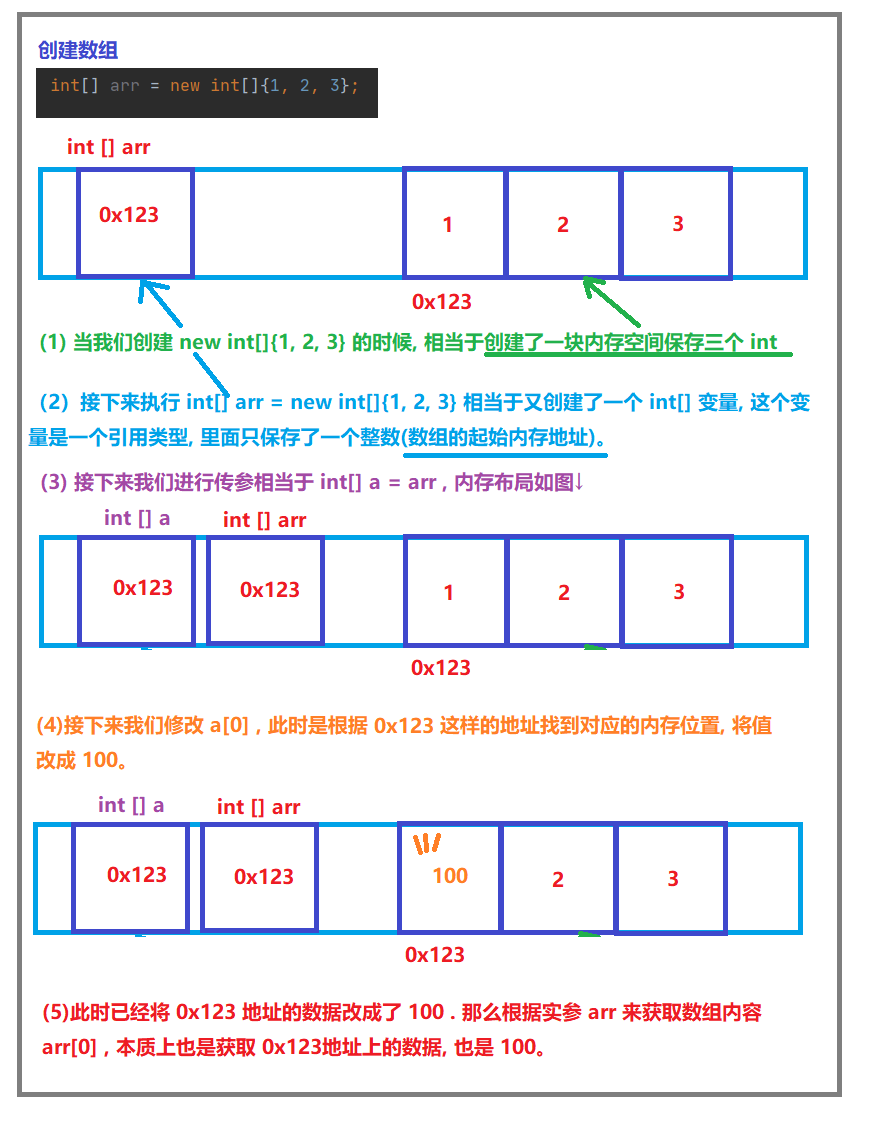
In fact, it is still an example of a house. Our quotation is that we can find the key that can enter our house, so that we can change our house. One day, we are too lazy to change, so we ask others to get it and give others a spare key. If others change the things of our house, our house must really change.
Let's write another code:
public static void print(int [] a){ for (int i = 0; i < a.length; i++) { System.out.print(a[i]); } System.out.println(); } public static void main(String[] args) { int[] arr = new int[]{1, 2, 3}; print(arr); }
In fact, there are roughly these things in the bottom layer of Java:
Let's take the stack heap as an example about references:
When creating an array, we will open up space in the stack to create an array name and its reference, and then create an array object in the heap, that is, the data stored in the array, and the reference points to the location of these data. Then pass the reference to a method, which is equivalent to copying the reference to him.
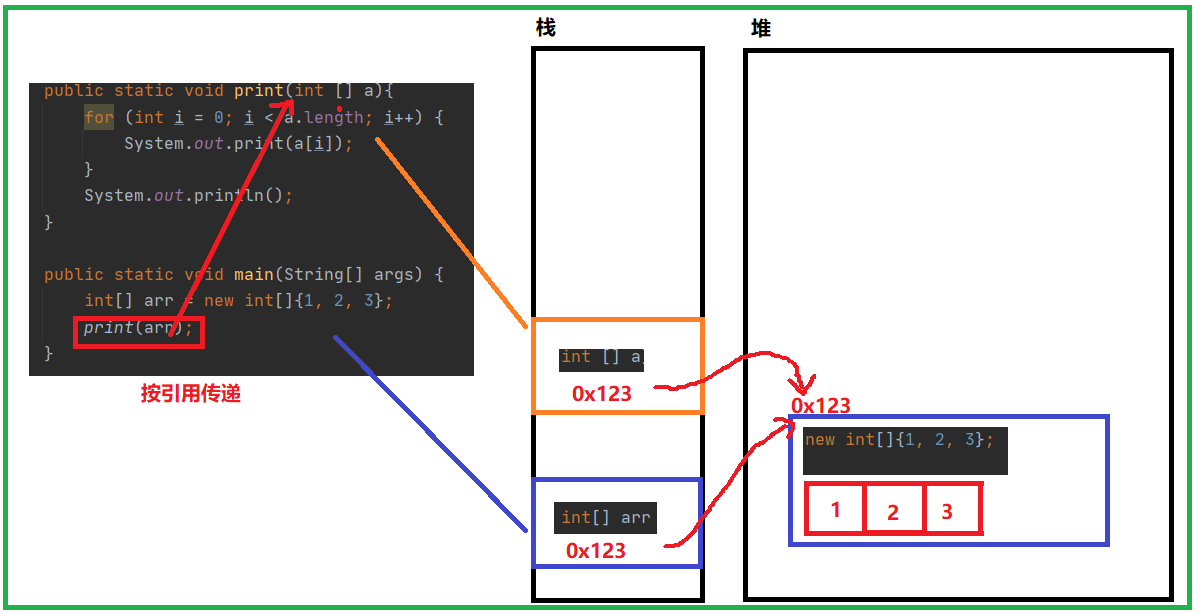
Summary:
The so-called "reference" is essentially just an address.
Java sets the array as the reference type. In this case, the subsequent array parameter transfer is actually just to pass the address of the array into the function parameter. This can avoid copying the entire array (the array may be long, so the copying cost will be great).
3. Recognition
Null means "null reference" in Java, that is, an invalid reference.
For example, we access an array with null reference:
public static void main(String[] args) { int[] arr = null; System.out.println(arr[0]); // Execution result: null error // Exception in thread "main" java.lang.NullPointerException // at Test.main(Test.java:6) }
The function of NULL is similar to NULL (NULL pointer) in C language. They all represent an invalid memory location. Therefore, no read and write operations can be performed on this memory. A NullPointerException is thrown once a read-write attempt is made.
4. Get familiar with JVM memory area division
JVM is the abbreviation of Java virtual machine. JVM is a specification for computing devices. It is a fictitious computer, which is realized by simulating various computer functions on an actual computer.

We found that in the above figure, the program counter, virtual machine stack and local method stack are surrounded by many forgive colored boxes called thread, and there are many copies. The heap, method area, and runtime constant pool have only one copy.
Here, we only need to understand the general structure, and then use it to understand references. For the learning of the whole JVM, let's say (bushi).
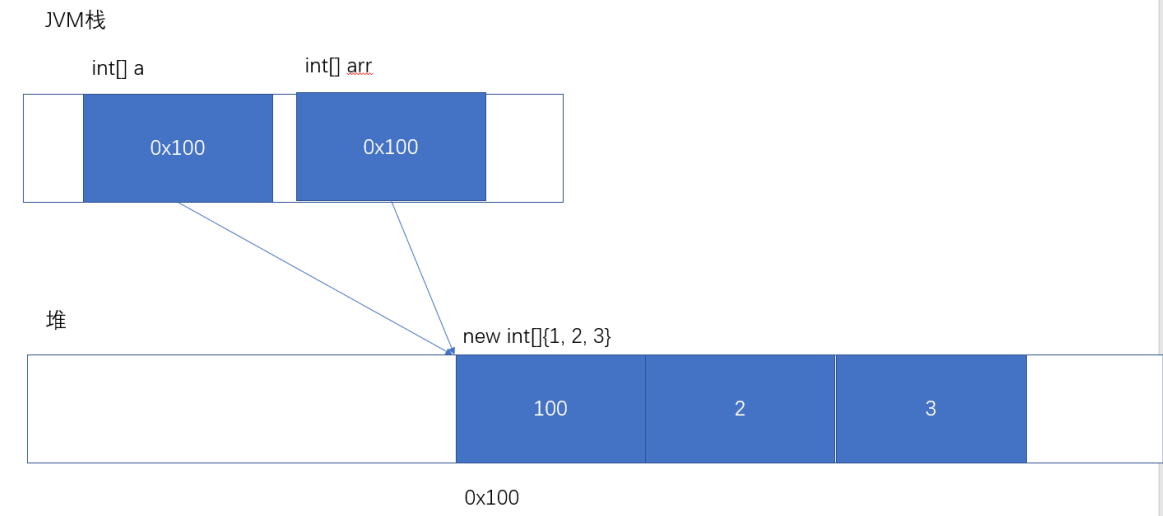
1. Local variables and references are saved on the stack, and new objects are saved on the heap.
2. The heap space is very large and the stack space is relatively small.
3. The heap is a stack shared by the whole JVM, and each thread has a copy (there may be multiple stacks in a Java program).
3, Array as the return value of the method
If I want every element of the array to double, how should I write it?
Let's look at this Code:
// Modify the original array directly public static void main(String[] args) { int[] arr = {1, 2, 3}; transform(arr); printArray(arr); } public static void printArray(int[] arr) { for (int i = 0; i < arr.length; i++) { System.out.println(arr[i]); } } public static void transform(int[] arr) { for (int i = 0; i < arr.length; i++) { arr[i] = arr[i] * 2; } }
Here, our code can achieve the desired effect, but it destroys the original array. Sometimes we don't want to destroy the original array, we need to create a new array inside the method and return it by the method.
So we need to receive the return value code:
// Returns a new array public static void main(String[] args) { int[] arr = {1, 2, 3}; int[] output = transform(arr); printArray(output); } public static void printArray(int[] arr) { for (int i = 0; i < arr.length; i++) { System.out.println(arr[i]); } } public static int[] transform(int[] arr) { int[] ret = new int[arr.length]; for (int i = 0; i < arr.length; i++) { ret[i] = arr[i] * 2; } return ret; }
In this way, the original array will not be destroyed. Because the array is a reference type, the first address of the array is only returned to the function caller when returning, and the array content is not copied, so it is relatively high
Effective.
4, Two dimensional array
We have introduced so many. In fact, what we just introduced are all one-dimensional arrays, as well as two-dimensional and three-dimensional arrays, but they all have the same advantages, so let's introduce two-dimensional arrays first.
A two-dimensional array is essentially a one-dimensional array, but each element is a one-dimensional array.
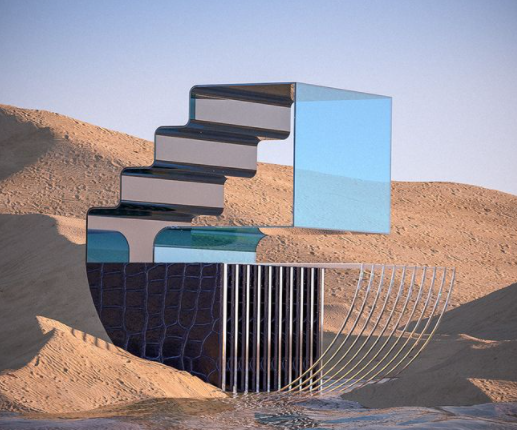
Basic syntax:
Data type [] [] array name = new data type [number of rows] [number of columns] {initialization data};
Code demonstration:
//Similar to a one-dimensional array public static void main(String[] args) { int[][] arr1 = {{1,2,3},{4,5}}; int[][] arr2 = new int[][]{{1,2,3},{4,5}}; int[][] arr3 = new int[2][3]; }
Why does the above say that a two-dimensional array is essentially a one-dimensional array? In fact, we can understand that in a two-dimensional array, each layer is regarded as an element, and there are some elements in the element of each layer.
Therefore, the first layer of a two-dimensional array represents how many rows there are, and then each row represents the column as a one-dimensional array to show the elements inside.
//Print 2D array public static void main(String[] args) { int[][] arr= new int[][]{{1,2,3},{4,5,6}}; for (int i = 0; i < arr.length; i++) { //Indicates how many lines there are for (int j = 0; j < arr[i].length; j++) { //Print all elements of each line System.out.printf("%d\t", arr[i][j]); } System.out.println(""); } }
This is a two-dimensional array.
There is no obvious difference between the usage of two-dimensional array and one-dimensional array, so we won't repeat it. Similarly, there are more complex arrays such as "three-dimensional array" and "four-dimensional array", but the frequency is very low.
This is all about the definition and use of arrays in Java. If you think it's good or helpful to you, you might as well like it and pay attention to one key three links. I will write an exercise on arrays later to learn together. Study together and work together! You can also look forward to the next blog of this series.
Link: It's all here! Java SE takes you from zero to a series
There is another thing: