This blog shares with you the knowledge about the operation of C language files, exchanges with each other and makes common progress
What is a file?
The code we wrote earlier uses "variables", or memory
Memory characteristics
Small capacity, speed block, data loss after power failure, relatively expensive
But memory can't meet all our usage scenarios. Sometimes we also want to have a larger storage capacity. It doesn't matter if the speed is a little slower. We hope that the data can be stored permanently. At this time, we use external memory, such as stored in the hard disk
External memory characteristics
Large capacity, slow speed, data still exists after power failure, which is cheaper than memory
So how do we store data in external memory? The key is through documents
Path to file
There are many files on our computer, so how does the operating system manage files? The key here is the path
eg.
E: \ embedded development learning \ development environment configuration 2021.docx
This is the path of the development environment configuration 2021.docx file on my computer
Note: the path of each file must be different on the same machine
File name suffix
File name suffixes are also called extensions
Some people may find that the files on their computer can't see the extension. This is because your computer hides the suffix. You can display the extension in the following ways
So you can see your file extension
Extension function:
- Describe the data format in this file
- This file should be opened using that program
Document classification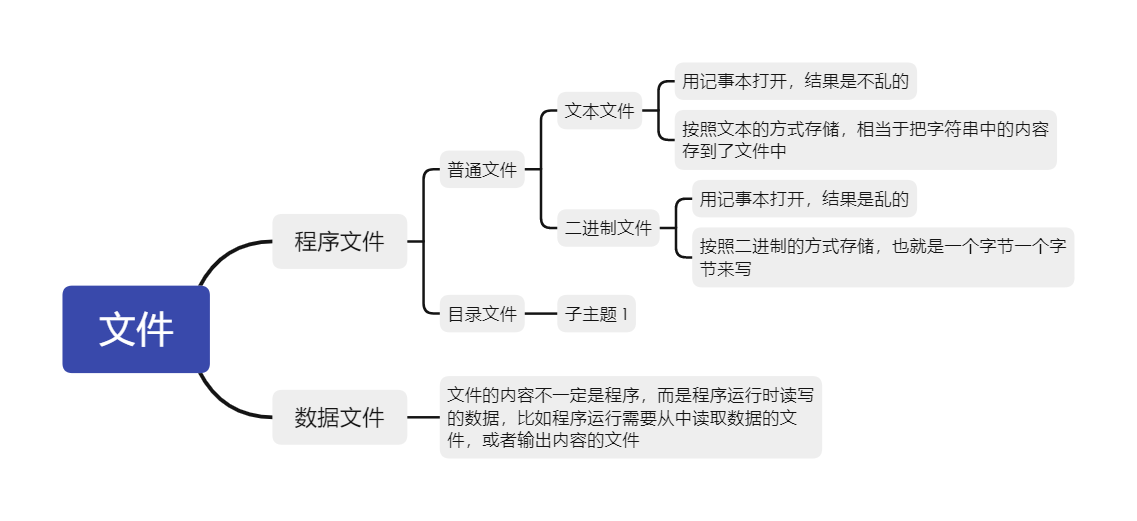
What we're talking about here is data files
How to operate files in C language?
The standard library also provides a FILE structure to describe a FILE
Files are stored on disk. It is not easy to directly operate the disk, so the operating system is encapsulated. When you open a FILE, you actually create a variable (FILE structure variable) in memory, which is associated with the FILE on the disk. Therefore, reading / writing this variable is equivalent to reading and writing the FILE.
C language standard library provides a set of operation functions (header files are < stdio. H >)
Here we learn some of the main functions
- fopen
- fclose
- fwrite
- fread
fopen
Used to open files
(in fact, a FILE structure is created in memory)
Function declaration
FILE* fopen (const char* filename, const char* mode);
Return value:
FILE* : field name pointer
If the file fails to be opened (the file does not exist or has no read-write permission), NULL is returned
Parameters:
filename: this parameter indicates a file path
mode: how to open the file
There are several basic ways to open
For text files:
- "r" read
- "w" Write Clear the original file data and write from the beginning of the file
- "a" Add Write the data to the end of the original file
Open for binary: "rb", "wb", "ab"
code
#include <stdio.h> int main() { FILE* fp = fopen("e:/test.txt", "r"); printf("%p\n", fp); return 0; }
Use this code to open the test.c file of disk d
Operation results:
You can see that the print result is 0, which is because the opening failed and the return value is NULL (because there is no such file on e disk of my computer)
We should judge the operation of opening files as follows:
#include <stdio.h> int main() { FILE* fp = fopen("e:/test.txt", "r"); if (fp == NULL) { perror("fopen"); return 0; } printf("File opened successfully\n"); return 0; }
Operation results:
There is an error code (errno) in the standard library to indicate the failure reason
There will be a corresponding error code when there is a problem in the operation of the program
You can use the strerror function to translate error codes
Using perror this function can easily print error codes
Let me create a text.txt file on disk e to have a look
fclose
This function closes the file
Why close the file?
This is similar to memory leakage. If we do not release the dynamically developed memory, it will cause memory leakage
If fclose is not performed after fopen, file resource leakage may be triggered (the number of files that a program can open at the same time is also limited). If the file is opened all the time without closing, it cannot be opened again to a certain extent
Function declaration
int fclose (FILE* stream);
Return value:
If the file is closed successfully, 0 is returned; otherwise, EOF (- 1) is returned
Parameters:
Stream: result returned by fopen
The following code:
#include <stdio.h> int main() { FILE* fp = fopen("e:/test.txt", "r"); if (fp == NULL) { perror("fopen"); return 0; } printf("File opened successfully\n"); fclose(fp); return 0; }
Operation results:
fread
read file
(it is actually copying the data in the disk to memory)
Function declaration
size_t fread (void* ptr, size_t size, size_t count, FILE* stream);
Return value:
Number of elements actually read successfully
Parameters:
ptr: to read a file is to copy the data from the disk to memory, and ptr points to this memory space
size: how big is each element
count: how many elements to copy
stream: pass in the result returned by fopen and find the specific location of the corresponding file on the disk
The following code:
#include <stdio.h> int main() { FILE* fp = fopen("e:/test.txt", "r"); if (fp == NULL) { perror("fopen"); return 0; } printf("File opened successfully\n"); char buffer[1024] = { 0 }; size_t count = fread(buffer, 1, 100, fp); printf("%d\n", count); printf("%s\n", buffer); fclose(fp); return 0; }
The data "hello" has been saved in the test.txt file
Operation results:
Open the file through this code, then read 100 elements from the file, one byte for each element, print the number of successfully read elements, and print the contents in the buffer
fwrite
Write file
(copy data in memory to disk)
Function declaration
size_t fwrite (const void* ptr, size_t size, size_t count, FILE* stream);
Return value:
size_t type, indicating the number of elements successfully written
Parameters:
ptr: copy the data in memory to disk. ptr points to this memory space
Size: the size of an element is size
Count: a total of count elements
stream: Pass in the result returned by fopen and find the specific location of the corresponding file on the disk
Code demonstration:
int main() { FILE* fp = fopen("e:/test.txt", "w"); if (fp == NULL) { perror("fopen"); return 0; } printf("File opened successfully\n"); char buffer[1024] = "success"; size_t count = fwrite(buffer, 1, strlen(buffer), fp); printf("%d\n", count); return 0; }
Operation results:
At this point, you can see that success has been saved in the test.txt file
Then let's run the following program again:
#include <stdio.h> int main() { FILE* fp = fopen("e:/test.txt", "w"); if (fp == NULL) { perror("fopen"); return 0; } printf("File opened successfully\n"); return 0; }
Open test.txt
It was found that the file was emptied after the code that only opened the file was executed
be careful
If you don't want to empty the original data, be sure to use "w" carefully
fprintf
Write data to file (format write)
See the following code:
#include <stdio.h> int main() { FILE* fp = fopen("e:/test.txt", "w"); if (fp == NULL) { perror("fopen"); return 0; } printf("File opened successfully\n"); fprintf(fp, "ret = %d", 2); return 0; }
Operation results:
You can see that the data is written to the file
fscanf
Format read
Then follow the operation of fprintf above
Modify the code as follows:
int main() { FILE* fp = fopen("e:/test.txt", "r"); if (fp == NULL) { perror("fopen"); return 0; } printf("File opened successfully\n"); int ret = 0; fscanf(fp, "ret = %d", &ret); printf("%d\n", ret); return 0; }
Operation results:
Successfully read data from file
sscanf
Parse the content from a string
The following code:
#include <stdio.h> int main() { char ch[] = "ret = 2"; int ret = 0; sscanf(ch, "ret = %d", &ret); printf("%d\n", ret); return 0; }
Operation results:
The result was successfully read from this string
sprintf
Output a formatted result to a string
The following code:
#include <stdio.h> int main() { char ch[50] = { 0 }; sprintf(ch, "ret = %d", 2); printf("%s\n", ch); return 0; }
Operation results:
The formatted result was successfully output to the string
Application scenario
For conversion between strings and numbers (integers / floating point numbers)
1. Convert string to integer
#include <stdio.h> int main() { char ch[] = "11"; char ch2[] = "12"; int num1 = 0; int num2 = 0; sscanf(ch, "%d", &num1); sscanf(ch2, "%d", &num2); int ret = num1 + num2; printf("%d\n", ret); return 0; }
Here, the contents of ch and ch2 are converted into integers through sscanf and added
Operation results:
2. Convert integer to string
#include <stdio.h> int main() { char ch[50] = { 0 }; int ret = 10; sprintf(ch, "%d", ret); printf("%s\n", ch); return 0; }
Operation results:
-----------------------------------------------------------------
-----------C language file operation completed---------
Welcome to pay attention!!!
Learn and communicate together!!!
Let's finish the programming!!!
--------------It's not easy to organize. Please support the third company------------------