3. Timer (Master)
* Timer class:timer public class Demo5_Timer { /** * @param args * timer * @throws InterruptedException */ public static void main(String[] args) throws InterruptedException { Timer t = new Timer(); t.schedule(new MyTimerTask(), new Date(114,9,15,10,54,20),3000); while(true) { System.out.println(new Date()); Thread.sleep(1000); } } } class MyTimerTask extends TimerTask { @Override public void run() { System.out.println("Get up and recite English words"); } }
4. Communication between two threads (Master)
-
1. When do I need to communicate
-
When multiple threads execute concurrently, the CPU switches threads randomly by default
-
If we want them to execute regularly, we can use communication, such as printing once per thread
-
-
2. How to communicate
-
If you want the thread to wait, call wait()
-
If you want to wake up the waiting thread, call notify();
-
These two methods must be executed in the synchronization code and called using the synchronization lock object
-
5. Communication between three or more threads
-
The problem of multi thread communication
-
The notify() method wakes up a thread at random
-
The notifyAll() method wakes up all threads
-
Unable to wake up a specified thread before JDK5
-
If multiple threads communicate, you need to use notifyAll() to notify all threads, and use while to repeatedly judge the conditions
-
6. Mutex, a new feature of JDK1.5 (Master)
-
1. Synchronization
- Use the lock() and unlock() methods of the ReentrantLock class for synchronization
-
2. Communication
-
Use the newCondition() method of the ReentrantLock class to obtain the Condition object
-
When you need to wait, use the await() method of Condition, and when you wake up, use the signal() method
-
Different threads use different conditions, so that you can distinguish which thread to find when waking up
-
7. Overview and use of thread group (understand)
-
A: Thread group overview
-
In Java, ThreadGroup is used to represent thread groups. It can classify and manage a batch of threads. Java allows programs to directly control thread groups.
-
By default, all threads belong to the main thread group.
-
public final ThreadGroup getThreadGroup() / / get the group it belongs to through the thread object
-
public final String getName() / / get the name of other groups through the thread group object
-
-
We can also group threads
-
1. ThreadGroup (string name) creates a thread group object and assigns a name to it
-
2. Create thread object
-
3,Thread(ThreadGroup?group, Runnable?target, String?name)
-
4. Set the priority or daemon thread of the whole group
-
-
8. Five thread states (Master)
-
Look at the picture and talk
-
New, ready, running, blocking, dead
9. Overview and use of thread pool (understand)
-
A: Thread pool overview
-
The cost of starting a new thread is relatively high because it involves interacting with the operating system. Using thread pool can improve performance, especially when a large number of threads with short lifetime are to be created in the program, thread pool should be considered. After the end of each thread code in the thread pool, it will not die, but return to the thread pool again to become idle and wait for the next object to use. Before JDK5, we must manually implement our own thread pool. Starting from JDK5, Java built-in supports thread pool
Function: when there are many short-lived threads in the program, in order to create threads infrequently, you can use thread pool,
Because the threads in the thread pool do not die after the code ends, but return to the thread pool again and become idle,
Wait for the next object to use (be sure to close it at last).
-
-
B: Overview of the use of built-in thread pool
-
JDK5 adds an Executors factory class to generate thread pool. There are the following methods
-
public static ExecutorService newFixedThreadPool(int nThreads)
-
public static ExecutorService newSingleThreadExecutor()
-
The return value of these methods is the ExecutorService object, which represents a thread pool that can execute the threads represented by the Runnable object or Callable object. It provides the following methods
-
Future<?> submit(Runnable task)
-
Future submit(Callable task)
-
-
Use steps:
-
Create thread pool object
-
Create Runnable instance
-
Submit Runnable instance
-
Close thread pool
-
-
10. Implementation mode of multithreaded program 3 (understand)
* Submitted is Callable
Advantages and disadvantages of mode 3 of multithreaded program implementation
*Benefits:
*Can have return value
*Exceptions can be thrown
* Disadvantages: * The code is complex, so it is generally not used
11. Overview and use of simple plant model (understand)
-
A: Simple factory model overview
- Also known as static factory method pattern, it defines a specific factory class, which is responsible for creating some class instances
-
B: Advantages
- The client does not need to be responsible for the creation of objects, so as to clarify the responsibilities of each class
-
C: Shortcomings
- This static factory class is responsible for the creation of all objects. If new objects are added or some objects are created in different ways, the factory class needs to be constantly modified, which is not conducive to later maintenance
-
D: Case demonstration
-
Animal abstract class: public abstract animal {public abstract void eat();}
-
Specific dogs: public class dog extensions animal {}
-
Specific cat category: public class cat extensions animal {}
-
At first, each specific content in the test class creates its own object. However, if it is troublesome to create an object, someone needs to do it specially, so we know a special class to create an object.
-
12. Overview and use of plant method model (understand)
-
A: Overview of factory method mode
- In the factory method pattern, the abstract factory class is responsible for defining the interface for creating objects, and the creation of concrete objects is implemented by the concrete class that inherits the abstract factory.
-
B: Advantages
- The client does not need to be responsible for the creation of objects, so as to clarify the responsibilities of each class. If a new object is added, only a specific class and a specific factory class need to be added, which does not affect the existing code. It is easy to maintain in the later stage and enhances the scalability of the system
-
C: Shortcomings
- Additional coding is required, which increases the workload
13. GUI (how to create a window and display it)
-
Graphical user interface.
Frame f = new Frame("my window"); f.setLayout(new FlowLayout());//Set up layout manager f.setSize(500,400);//Set form size f.setLocation(300,200);//Sets where the form appears on the screen f.setIconImage(Toolkit.getDefaultToolkit().createImage("qq.png")); f.setVisible(true);
14. GUI (layout manager)
-
FlowLayout (flow layout manager)
-
From left to right.
-
Panel the default layout manager.
-
-
BorderLayout (boundary layout manager)
-
East, South, West, north, middle
-
Frame is the default layout manager.
-
-
GridLayout (grid layout manager)
- Regular matrix
-
CardLayout (card layout manager)
- tab
-
GridBagLayout (grid package layout manager)
- Irregular matrix
15. GUI (form listening)
Frame f = new Frame("My form"); //The event source is a form that registers the listener to the event source //The event object is passed to the listener f.addWindowListener(new WindowAdapter() { # Learn, share and encourage **Android Advanced path of Senior Architect** **[CodeChina Open source projects:< Android Summary of study notes+Mobile architecture video+Real interview questions for large factories+Project practice source code](https://codechina.csdn.net/m0_60958482/android_p7)** Aside, I have worked in Ali for many years and know the direction of technological reform and innovation, Android With its beautiful, fast, efficient and open advantages, development has quickly captured the hearts of the people, but there are many problems Android The advanced learning materials required by interest lovers are really not systematic and complete. Today, I share the learning materials I collected and sorted out with people in need * **Android Advanced knowledge system learning brain map** 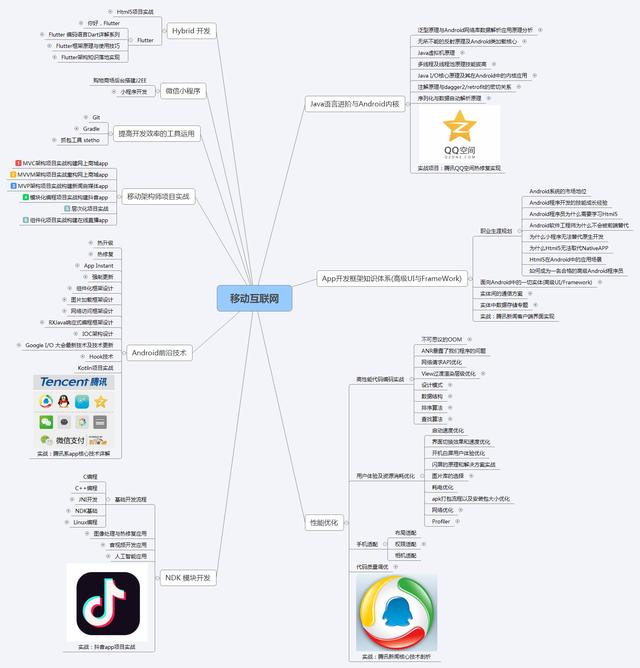 * **Android Advanced senior engineers learn a full set of manuals** 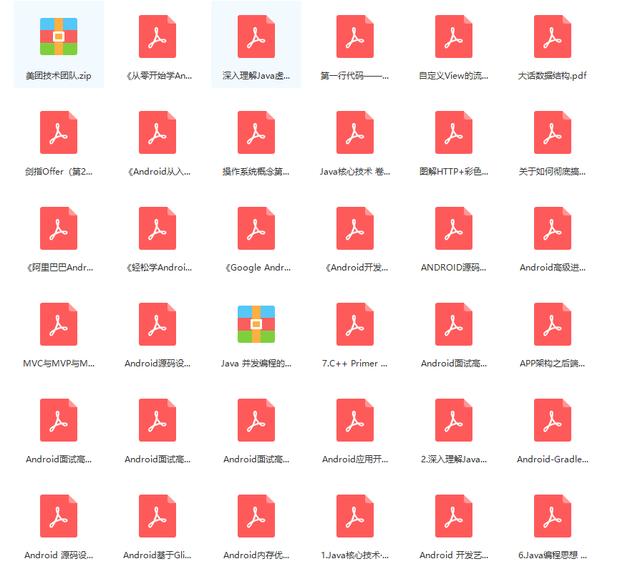 * **benchmarking Android Ali P7,Annual salary 50 w+Learning video** 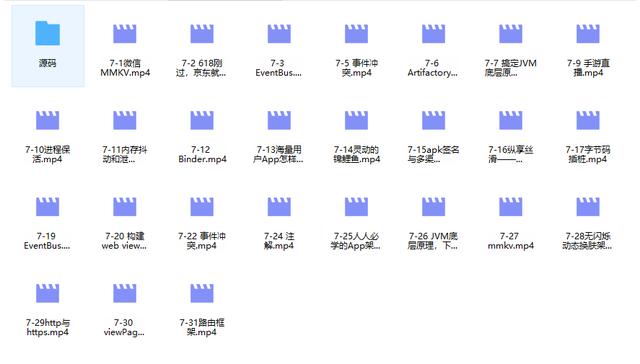 * **Inside the big factory Android High frequency interview questions and interview experience** He has worked for many years and knows the direction of technological reform and innovation, Android With its beautiful, fast, efficient and open advantages, development has quickly captured the hearts of the people, but there are many problems Android The advanced learning materials required by interest lovers are really not systematic and complete. Today, I share the learning materials I collected and sorted out with people in need * **Android Advanced knowledge system learning brain map** [External chain picture transfer...(img-quHZlcnf-1630931786786)] * **Android Advanced senior engineers learn a full set of manuals** [External chain picture transfer...(img-HbkVr2Kr-1630931786787)] * **benchmarking Android Ali P7,Annual salary 50 w+Learning video** [External chain picture transfer...(img-a1ztINvh-1630931786788)] * **Inside the big factory Android High frequency interview questions and interview experience** 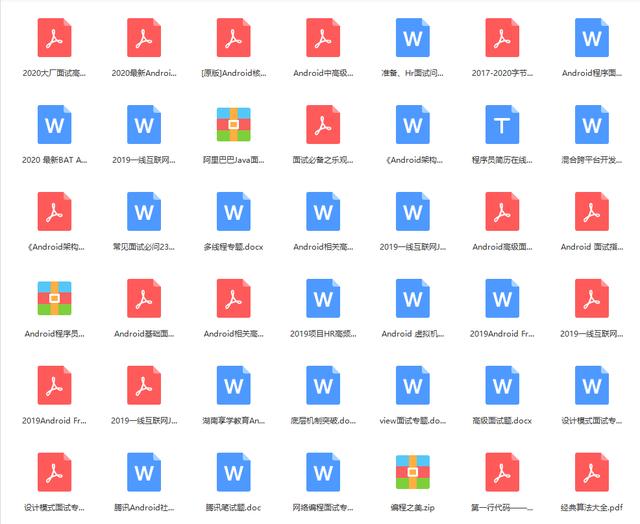