-
Create a new jquery.min.js file in vs (any name here, as long as it is a JS file)
-
Import in html file
<script src = "jquery.min.js"></script>
// The first method is recommended $(function(){ ... // This is the entrance to the completion of page DOM loading }) // The second method $(document).ready(function(){ ... //This is the entrance to the completion of page DOM loading })
-
After the DOM structure is rendered, we can execute the internal code. We don't have to wait until all external resources are loaded. jQuery helps us complete the encapsulation
-
Equivalent to DOMContentLoaded in native js
-
Unlike the load event in native js, the internal code is executed only after the page document, external js file, css file and picture are loaded
-
The first method is more recommended
1.3 top level object of jQuery$ 🔥
-
$is a nickname of jQuery, which can be replaced by jQuery in code$
-
$is the top-level object of jQuery, which is equivalent to window in native JavaScript.
-
By wrapping the element into a jQuery object with $, you can call the method of jQuery.
<body> <div></div> <script> // 1. $is another name of jQuery (another name) // $(function() { // alert(11) // }); jQuery(function() { // alert(11) // $('div').hide(); jQuery('div').hide(); }); // 2. $is also the top-level object of jQuery </script> </body>
1.4. jQuery object and DOM object 🔥
-
DOM object: the object obtained with native js
-
JQuery object: the object obtained by jQuery is a jQuery object.
Essence: DOM elements are wrapped by $(stored in pseudo array form)
-
JQuery objects can only use jQuery methods, while DOM objects use native JavaScript properties and methods
<body> <div></div> <span></span> <script> // 1. DOM object: the object obtained with native js is the DOM object var myDiv = document.querySelector('div'); // myDiv is a DOM object var mySpan = document.querySelector('span'); // mySpan is a DOM object console.dir(myDiv); // 2. jQuery object: the object obtained by jQuery is a jQuery object. // Essence: DOM elements are wrapped by $ $('div'); // $('div ') is a jQuery object $('span'); // $('span ') is a jQuery object console.dir($('div')); // 3. jQuery objects can only use jQuery methods, while DOM objects use native JavaScirpt attributes and methods // myDiv.style.display = 'none'; // myDiv.hide(); myDiv is a dom object. You cannot use the hide method in jquery // $('div').style.display = 'none'; This $('div ') is a jQuery object and cannot use the properties and methods of native js </script> </body>
1.5. jQuery object and DOM object conversion 🔥
-
DOM objects and jQuery objects can be converted to each other.
-
Because the native js is larger than jQuery, some of the native properties and methods of jQuery are not encapsulated. To use these properties and methods, you need to convert the jQuery object into a DOM object.
1.5.1 converting DOM objects to jQuery objects 🔥
- Syntax: $(DOM object)
$('div')
1.5.2 converting jQuery objects to DOM objects 🔥
- Syntax:
// The first method $('div')[index] index Is the index number // The second method $('div').get(index) index Is the index number
<video src="mov.mp4" muted></video> <script> // 1. Convert DOM object to jQuery object // (1) We get the video directly and get the jQuery object // $('video'); // (2) We have used native js to get DOM objects var myvideo = document.querySelector('video'); // $(myvideo).play(); There is no play method in jQuery // 2. Convert jQuery object to DOM object // myvideo.play(); $('video')[0].play() $('video').get(0).play() </script>
[]( )2,jQuery Commonly used API🔥 ================================================================================= []( )2.1,jQuery selector🔥 --------------------------------------------------------------------------------- ### [] () 2.1.0, jQuery basic selector 🔥 Primordial JS There are many ways to obtain elements, which are very complex, and the compatibility is inconsistent, so jQuery We have made encapsulation to make the acquisition elements unified. Syntax:`$("selector")` Write directly inside CSS Selectors are OK, but use quotation marks
$(".nav");
| name | usage | describe | | --- | --- | --- | | ID selector | `$("#ID ") ` | get the element with the specified ID| | Select all selector | `$('*')` | Match all elements | | Class selector | `$(".class")` | Get the same class class Element of | | tag chooser | `$(".div")` | Gets all elements of the same class of labels | | Union selector | `$("div,p,li")` | Select multiple elements | | Intersection selector | `$("li.current")` | Intersection element | ### [] () 2.1.1. jQuery level selector 🔥 | name | usage | describe | | --- | --- | --- | | Progeny selector | `$("ul>li")` | use>Number, get the selector of parent-child level; Note that elements at the grandchild level are not obtained | | Descendant Selectors | `$("ul li")` | Using a space to represent the descendant selector, get ul All under li Elements, including grandchildren, etc |
$("ul li");
<body> <div>I am div</div> <div class="nav">I am nav div</div> <p>I am p</p> <ol> <li>I am ol of</li> <li>I am ol of</li> <li>I am ol of</li> <li>I am ol of</li> </ol> <ul> <li>I am ul of</li> <li>I am ul of</li> <li>I am ul of</li> <li>I am ul of</li> </ul> <script> $(function() { console.log($(".nav")); console.log($("ul li")); }) </script> </body> ``` ### [] () 2.1.2. Knowledge foreshadowing jQuery Set style ``` $('div').css('attribute','value') ``` ### [] () 2.1.3. jQuery implicit iteration 🔥 * Traverse inside DOM element(Pseudo array storage)The process is called **Implicit iteration** * Simple understanding: Loop through all the matched elements and execute the corresponding methods instead of looping, which simplifies our operation and facilitates our call ``` <body> <div>Surprise no, surprise no</div> <div>Surprise no, surprise no</div> <div>Surprise no, surprise no</div> <div>Surprise no, surprise no</div> <ul> <li>Same operation</li> <li>Same operation</li> <li>Same operation</li> </ul> <script> // 1. Get four div elements console.log($("div")); // 2. Set the background color of four div s to pink. jquery objects cannot use style $("div").css("background", "pink"); // 3. Implicit iteration is to loop through all matched elements and add css to each element $("ul li").css("color", "red"); </script> </body> ``` ### [] () 2.1.3. jQuery filter selector 🔥 ``` // Select the first li in ul $("ul li:first").css("color","red"); ``` | grammar | usage | describe | | --- | --- | --- | | : first | `$('li:first')` | Get first li element | | : last | `$('li:last')` | Get last li element | | : eq(index) | `$("li:eq(2)")` | Obtained li Element, select the element with index number 2 index Start from 0 | | : odd | `$("li:odd")` | Obtained li Element, select an element with an odd index number | | : even | `$("li:even")` | Obtained li Element, select the element with an even index number | ``` <body> <ul> <li>Select several from multiple</li> <li>Select several from multiple</li> <li>Select several from multiple</li> <li>Select several from multiple</li> <li>Select several from multiple</li> <li>Select several from multiple</li> </ul> <!-- Ordered list --> <ol> <li>Select several from multiple</li> <li>Select several from multiple</li> <li>Select several from multiple</li> <li>Select several from multiple</li> <li>Select several from multiple</li> <li>Select several from multiple</li> </ol> <script> $(function() { // Get the first li element $("ul li:first").css("color", "red"); // Gets li with index number 2 $("ul li:eq(2)").css("color", "blue"); // Gets li with odd index numbers $("ol li:odd").css("color", "skyblue"); // Gets li with an even index number $("ol li:even").css("color", "pink"); }) </script> </body> ``` ### [] () 2.1.4 jQuery filtering method 🔥 | grammar | usage | explain | | --- | --- | --- | | 🔥parent() | `$("li").parent();` | Find the parent, the nearest parent element | | 🔥children(selector) | `$("ul").children("li")` | amount to`$("ul>li")`,Nearest level(Own son) | | 🔥find(selector) | `$("ul").find("li")` | amount to`$("ul li")` Descendant Selectors | | 🔥siblings(selector) | `$(".first").siblings("li")` | Find sibling nodes,**Not myself** | | nextAll(\[expr\]) | `$(".first").nextAll()` | Find current element**after**All peer elements | | prevtAll(\[expr\]) | `$(".last").prevAll()` | Find current element**before**All peer elements | | hasClass(class) | `$('div').hasClass("protected")` | Check whether the current element contains a specific class. If so, return true | | 🔥eq(index) | `$("li").eq(2);` | amount to`$("li:eq(2),index")`index Start from 0 | ``` <body> <div class="yeye"> <div class="father"> <div class="son">Son</div> </div> </div> <div class="nav"> <p>I'm fart</p> <div> <p>I am p</p> </div> </div> <script> // Note that all methods are parenthesized $(function() { // 1. parent() returns the parent of the nearest parent element console.log($(".son").parent()); // 2. Sub // (1) The parent children() is similar to the offspring selector UL > Li // $(".nav").children("p").css("color", "red"); // (2) You can select all the children, including sons and grandchildren. find() is similar to the offspring selector $(".nav").find("p").css("color", "red"); }); </script> </body> ``` ### [] () 2.1.5 jQuery exclusivity 🔥 * If you want to choose one more effect, exclusive thought: set the style for the current element and clear the style for the other sibling elements. ``` <body> <button>fast</button> <button>fast</button> <button>fast</button> <button>fast</button> <button>fast</button> <button>fast</button> <button>fast</button> <script> $(function() { // 1. Implicit iteration binds click events to all buttons $("button").click(function() { // 2. The current element changes the background color $(this).css("background", "pink"); // 3. The other brothers remove the background color and iterate implicitly $(this).siblings("button").css("background", ""); }); }) </script> </body> ``` ### [] () 2.1.6 jQuery chain programming 🔥 Chain programming is to save code and look more elegant. When using chained programming, be sure to pay attention to which object executes the style. ``` $(this).css("color","red").siblings().css("color","");
<button>fast</button> <button>fast</button> <button>fast</button> <button>fast</button> <button>fast</button> <button>fast</button> <button>fast</button> <script> $(function() { // 1. Implicit iteration binds click events to all buttons $("button").click(function() { // 2. Change the current element color to red (click which to change to red) // $(this).css("color", "red"); // 3. Keep the other sibling elements from changing color // $(this).siblings().css("color", ""); // Chain programming completes the above functions $(this).css("color", "red").siblings().css("color", ""); }); }) </script>
[]( )2.2,jQuery Style operation🔥 ---------------------------------------------------------------------------------- ### [] () 2.2.1. jQuery modify style css method jQuery have access to css Method to modify the simple element style; You can also manipulate classes to modify multiple styles 1. Parameter writes only the property name,Property value is returned
$(this).css("color");
2. The parameter is the property name,Attribute value,Comma separated,Is to set a set of styles. Attribute needs quotation marks,The value is a number without units and quotation marks
$(this).css("color",300);
3. Parameters can be in the form of objects,It is convenient to set multiple sets of styles. Property names and property values are separated by colons,Attributes can be used without quotes
$(this).css({
"color":"red", "width": 400, "height": 400, // If it is a composite attribute, the hump naming method must be adopted. If the value is not a number, quotation marks are required backgroundColor: "red"
})
### [] () 2.2.2. jQuery setting class style method The effect is equivalent to the previous one classList,You can manipulate the class style. Pay attention not to add points to the parameters in the operation class.
//1. Add class
$("div").addClass("current");
//2. Remove class
$("div").removeClass("current");
//3. Switching class
$("div").toggleClass("current");
<head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <style> div { width: 150px; height: 150px; background-color: pink; margin: 100px auto; transition: all 0.5s; } .current { background-color: red; transform: rotate(360deg); } </style> <script src="jquery.min.js"></script> </head> <body> <div class="current"></div> <script> $(function() { // 1. Add class addClass() // $("div").click(function() { // // $(this).addClass("current"); // }); // 2. Delete class removeClass() // $("div").click(function() { // $(this).removeClass("current"); // }); // 3. Toggle class () $("div").click(function() { $(this).toggleClass("current"); }); }) </script> </body> ``` ### [] () 2.2.3. Differences between jQuery class operation and className * Primordial JS Medium className It will overwrite the original class name of the element * jQuery The inner class operation only operates on the specified class and does not affect the original class name ``` <body> <div class="one two"></div> <script> // var one = document.querySelector(".one"); // one.className = "two"; // $(".one").addClass("two"); This addclass is equivalent to appending the class name without affecting the previous class name $(".one").removeClass("two"); </script> </body> ``` []( )2.3,jQuery effect🔥 -------------------------------------------------------------------------------- ### [] () 2.3.1. jQuery display and hiding effect jQuery Many animation effects are encapsulated for us. The most common are as follows: 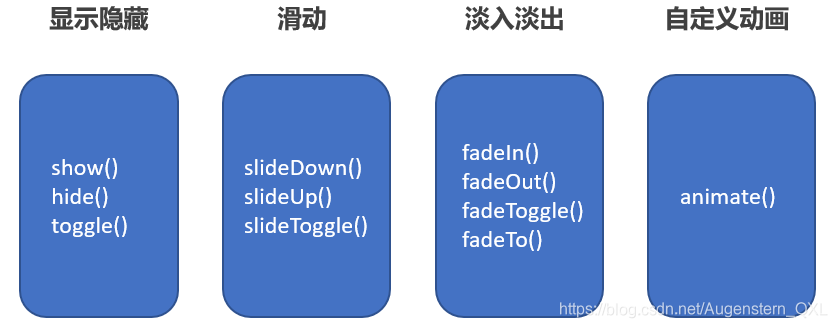 #### [] () ① display effect Syntax: ``` // Display syntax specification show([speed,[easing],[fn]]) //Brackets indicate that all parameters can be omitted $("div").show(); ``` Parameters: * Parameters can be omitted and displayed directly without animation. * speed: A string of three predetermined speeds("slow","normal", or "fast")Or a number in milliseconds that represents the duration of the animation(For example: 1000). * easing: (Optional) Used to specify the switching effect. The default is“ swing",Available parameters“ linear". * fn: Callback function, which is executed when the animation is completed. Each element is executed once. #### [] () ② hidden effect Syntax: ``` // Hide syntax specification hide([speed,[easing],[fn]]) $("div").hide(); ``` Parameters: * Parameters can be omitted and displayed directly without animation. * speed: A string of three predetermined speeds("slow","normal", or "fast")Or a number in milliseconds that represents the duration of the animation(For example: 1000). * easing: (Optional) Used to specify the switching effect. The default is“ swing",Available parameters“ linear". * fn: Callback function, which is executed when the animation is completed. Each element is executed once. #### [] () ③ switching effect Syntax: ``` // Switch syntax specification toggle([speed,[easing],[fn]]) $("div").toggle(); ``` Parameters: * Parameters can be omitted and displayed directly without animation. * speed: A string of three predetermined speeds("slow","normal", or "fast")Or a number in milliseconds that represents the duration of the animation(For example: 1000). * easing: (Optional) Used to specify the switching effect. The default is“ swing",Available parameters“ linear". * fn: Callback function, which is executed when the animation is completed. Each element is executed once. Suggestion: generally, there are no parameters, and you can directly display or hide them ``` <body> <button>display</button> <button>hide</button> <button>switch</button> <div></div> <script> $(function() { $("button").eq(0).click(function() { $("div").show(1000, function() { alert(1); }); }) $("button").eq(1).click(function() { $("div").hide(1000, function() { alert(1); }); }) $("button").eq(2).click(function() { $("div").toggle(1000); }) // In general, we can directly display and hide without adding parameters }); </script> </body> ``` ### [] () 2.3.2. jQuery sliding effect and event switching #### [] () ① sliding down Syntax: ``` // Sliding down slideDown([speed,[easing],[fn]]) $("div").slideDown(); ``` #### [] () ② sliding up Syntax: ``` // Slide up slideUp([speed,[easing],[fn]]) $("div").slideUp(); ``` #### [] () ③ sliding switching Syntax: ``` // Sliding switching effect slideToggle([speed,[easing],[fn]]) $("div").slideToggle();
<button>Pull down sliding</button> <button>Pull up sliding</button> <button>Switching sliding</button> <div></div> <script> $(function() { $("button").eq(0).click(function() { // Slide down () $("div").slideDown(); }) $("button").eq(1).click(function() { // Slide up $("div").slideUp(500); }) $("button").eq(2).click(function() { // Slide toggle () $("div").slideToggle(500); }); }); </script>
#### [] () ④ event switching Syntax:
hover([over,]out)
* over: Move the mouse over the element to trigger the function(amount to mouseenter) * out: Move the mouse out of the function to be triggered by the element(amount to mouseleave) * If you write only one function, it will be triggered when the mouse passes and leaves
$("div").hover(function(){},function(){});
//The first function is the function passed by the mouse
//The second function is the function that the mouse leaves
//If hover only writes one function, this function will be triggered when the mouse passes and when the mouse leaves
$("div").hover(function(){
$(this).slideToggle();
})
### [] () 2.3.3. jQuery animation queue and its stopping method Once the animation or effect is triggered, it will be executed. If it is triggered multiple times, multiple animations or effects will be queued for execution #### [] () ① stop queuing Syntax:
stop()
* stop()Method to stop an animation or effect * be careful: stop() Writing in front of the animation or effect is equivalent to stopping and ending the last animation
$(".nav>li").hover(function(){
// The stop method must be written before the animation $(this).children("ul").stop().slideToggle();
})
### [] () 2.3.4. jQuery fade in and out and highlight effect #### [] () ① fade in and fade out switching Syntax:
//Fade in
fadeIn([speed,[easing],[fn]])
$("div").fadeIn();
//Fade out
fadeOut([speed,[easing],[fn]])
$("div").fadeOut;
//Fade in and fade out switch
fadeToggle([speed,[easing],[fn]])
#### [] () ② adjust gradually to the specified opacity Syntax:
//Modify transparency. This speed and transparency must be written
fadeTo(speed,opacity,[easing],[fn])
Parameters: * opacity : Transparency must be written, and the value is 0~1 between * speed: A string of three predetermined speeds("slow","normal", or "fast")Or a number in milliseconds that represents the duration of the animation(For example: 1000). Must write
$("div").fadeTo(1000,0.5)
<body> <button>Fade in effect</button> <button>Fade out effect</button> <button>Fade in and fade out switch</button> <button>Modify transparency</button> <div></div> <script> $(function() { $("button").eq(0).click(function() { // Fade fadeIn() $("div").fadeIn(1000); }) $("button").eq(1).click(function() { // Fade fadeOut() $("div").fadeOut(1000); }) $("button").eq(2).click(function() { // Fade in and fade out switch fadeToggle() $("div").fadeToggle(1000); }); $("button").eq(3).click(function() { // Modify the transparency fadeTo(), the speed and transparency must be written $("div").fadeTo(1000, 0.5); }); }); </script> </body> ``` ### [] () 2.3.5. jQuery custom animation method Syntax: ``` animate(params,[speed],[easing],[fn]) ``` Parameters: * params: If the style attribute you want to change is passed as an object, it must be written. The attribute name can be without quotation marks. If it is a composite attribute, the hump naming method needs to be adopted borderLeft. Other parameters can be omitted. ``` <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <script src="jquery.min.js"></script> <style> div { position: absolute; width: 200px; height: 200px; background-color: pink; } </style> </head> <body> <button>Move</button> <div></div> <script> $(function() { $("button").click(function() { $("div").animate({ left: 500, top: 300, opacity: .4, width: 500 }, 500); }) }) </script> </body> ``` []( )2.4,jQuery Attribute operation🔥 ---------------------------------------------------------------------------------- ### [] () 2.4.1. Get the element intrinsic attribute value prop() The so-called inherent attribute of an element is the attribute of the element itself, such as `<a>` Inside the element href ,such as `<input>` Inside the element type. Syntax:`prop("attribute")` ``` prop("attribute") $("a").prop("href"); ``` ### [] () 2.4.2. Set intrinsic attribute value of element Syntax:`prop("attribute","Attribute value")` ``` prop("attribute","Attribute value") $("a").prop("title","We're all fine~"); ``` ### [] () 2.4.3. Get element custom attribute value The attributes added by the user to the element are called custom attributes. For example, give div add to index ="1". Syntax:`attr("attribute")` ``` attr("attribute") // Similar to native getAttribute() $("div").attr("index"); ``` ### [] () 2.4.4. Set element custom attribute value Syntax:`attr("attribute","Attribute value")` # summary We always like to visit the great gods of big factories, but in fact, the great gods are just mortals. Compared with rookie programmers, they spend a little more time. If you don't work hard, the gap will only become bigger and bigger. The interview questions are more or less helpful for what you have to do next, but I hope you can summarize your shortcomings through the interview questions to improve your core technical competitiveness. Every interview experience is a literacy of your technology. The effect of re examination and summary after the interview is excellent! If you need this full version**Interview notes**,As long as you do more**support**I this article. **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** quiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <script src="jquery.min.js"></script> <style> div { position: absolute; width: 200px; height: 200px; background-color: pink; } </style> </head> <body> <button>Move</button> <div></div> <script> $(function() { $("button").click(function() { $("div").animate({ left: 500, top: 300, opacity: .4, width: 500 }, 500); }) }) </script> </body> ``` []( )2.4,jQuery Attribute operation🔥 ---------------------------------------------------------------------------------- ### [] () 2.4.1. Get the element intrinsic attribute value prop() The so-called inherent attribute of an element is the attribute of the element itself, such as `<a>` Inside the element href ,such as `<input>` Inside the element type. Syntax:`prop("attribute")` ``` prop("attribute") $("a").prop("href"); ``` ### [] () 2.4.2. Set intrinsic attribute value of element Syntax:`prop("attribute","Attribute value")` ``` prop("attribute","Attribute value") $("a").prop("title","We're all fine~"); ``` ### [] () 2.4.3. Get element custom attribute value The attributes added by the user to the element are called custom attributes. For example, give div add to index ="1". Syntax:`attr("attribute")` ``` attr("attribute") // Similar to native getAttribute() $("div").attr("index"); ``` ### [] () 2.4.4. Set element custom attribute value Syntax:`attr("attribute","Attribute value")` # summary We always like to visit the great gods of big factories, but in fact, the great gods are just mortals. Compared with rookie programmers, they spend a little more time. If you don't work hard, the gap will only become bigger and bigger. The interview questions are more or less helpful for what you have to do next, but I hope you can summarize your shortcomings through the interview questions to improve your core technical competitiveness. Every interview experience is a literacy of your technology. The effect of re examination and summary after the interview is excellent! If you need this full version**Interview notes**,As long as you do more**support**I this article. **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** 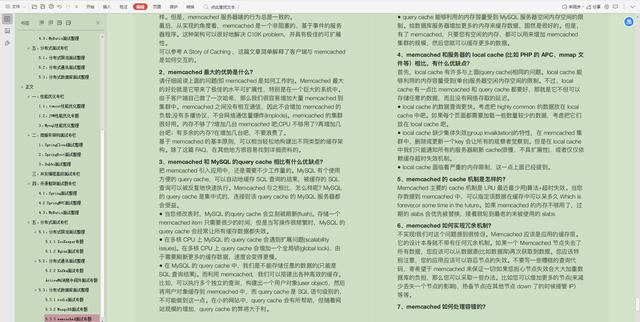