*Student numbers are represented as strings and entered in a random order in the Map collection.
* The elements in the Map collection are then printed in order of the number from largest to smallest.
*You need to customize the Comparator of the Map collection because the size comparison of string objects is in dictionary order, not the corresponding numeric value.
Requirements: You must sort using the internal sorting mechanism of the Map collection, not externally.
import java.util.*; public class Test { public static void main(String[] args) { //Create map object TreeMap tm =new TreeMap(new MyComparator()); //Store keys and values tm.put("1006", "Zhenghuan"); tm.put("1003", "Sub-ink"); tm.put("1002", "Boyi"); tm.put("1004", "In Van"); tm.put("1005", "Yanbin"); tm.put("1015", "a fine piece of jade"); tm.put("1001", "Enchang"); tm.put("1009", "Zhenxi"); tm.put("1010", "Ji-Hee"); tm.put("1008", "Sheng En"); tm.put("1014", "Young Forest"); tm.put("1011", "Zhenxian"); tm.put("1007", "Smart"); tm.put("1012", "Xiaolin"); tm.put("1013", "Sensitivity"); //Get a collection of keys Set keySet=tm.keySet(); //Get Iterator Object Iterator it=keySet.iterator(); while(it.hasNext()) { //Get Key Value Object key=it.next(); //Get the value for the key Object value=tm.get(key); //Output keys and values System.out.println(key +":"+value); } /* Or access keys and values through foreach for(Object key: tm.keySet()){ System.out.println(key+" "+tm.get(key)); } */ } } //Custom Comparator MyComparator class MyComparator implements Comparator{ public int compare(Object b1, Object b2) { String s1=(String)b1; String s2=(String)b2; return s2.compareTo(s1); } }
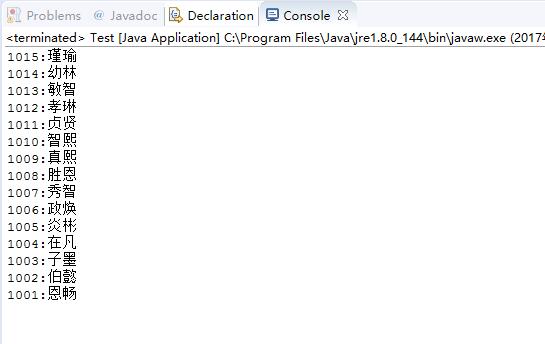