1. What is a persistence class
1. Persistence class: it is a Java class (the JavaBean we wrote), which can be a persistence class if the mapping relationship between the Java class and the table is established.
Persistence class = JavaBean + xxx.hbm.xml
2. The concept of persistent classes exists in the Hibernate framework environment.
2. Rules for writing persistent classes
Provides a constructor with no parameter public access controller. – the underlying layer needs to be reflected.
Provide an identity property to map the primary key field of the data table. – uniquely identify the OID. The database uses the primary key; the Java object uses the address to determine the object; and the persistence class uses the unique ID OID to determine the record.
All properties need to provide get and set methods.
As far as possible, the packaging type of the basic data type should be used for identification properties.
Choose to implement the serialization interface.
3. Persistence class example
1. New User class
/Hibernate5_d02_c01/src/hibernate/domain/User.java
The program code is as follows:
package hibernate.domain;
import java.io.Serializable;
/*
* Choose to implement serialization interface
*/
public class User implements Serializable{
//A unique ID OID
private Integer id;
private String name;
//Type try to use basic type of packaging class
private Integer age;
/**
* Empty construction method for reflecting generated objects
@Title: User
*/
public User() {
// TODO Auto-generated constructor stub
}
//Provide getter and setter methods
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
}
2. New User.hbm.xml
/Hibernate5_d02_c01/src/hibernate/domain/User.hbm.xml
The program code is as follows:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="hibernate.domain.User" table="t_user">
<id name="id" column="id">
<generator class="native"></generator>
</id>
<property name="name" column="name"></property>
<property name="age" column="age"></property>
</class>
</hibernate-mapping>
3. Associate User.hbm.xml in hibernate.cfg.xml
/Hibernate5_d02_c01/src/hibernate.cfg.xml
The program code is as follows:
<?xml version="1.0" encoding="UTF-8"?>
...
<!-- Mapping file -->
<mapping resource="/hibernate/domain/Person.hbm.xml"/>
<mapping resource="/hibernate/domain/User.hbm.xml"/>
</session-factory>
</hibernate-configuration>
4. New TestUser test class
/Hibernate5_d02_c01/src/hibernate/test/TestUser.java
The program code is as follows:
package hibernate.test;
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.junit.Test;
import hibernate.domain.User;
import hibernate.util.HibernateUtils;
public class TestUser {
@Test
public void testSave(){
//Get session
Session session = HibernateUtils.openSession();
Transaction tr = session.beginTransaction();
//New User object
User user = new User();
user.setName("Xiao Zhang");
user.setAge(18);
//Preservation
session.save(user);
tr.commit();
//close resource
session.close();
}
}
5. Running the project
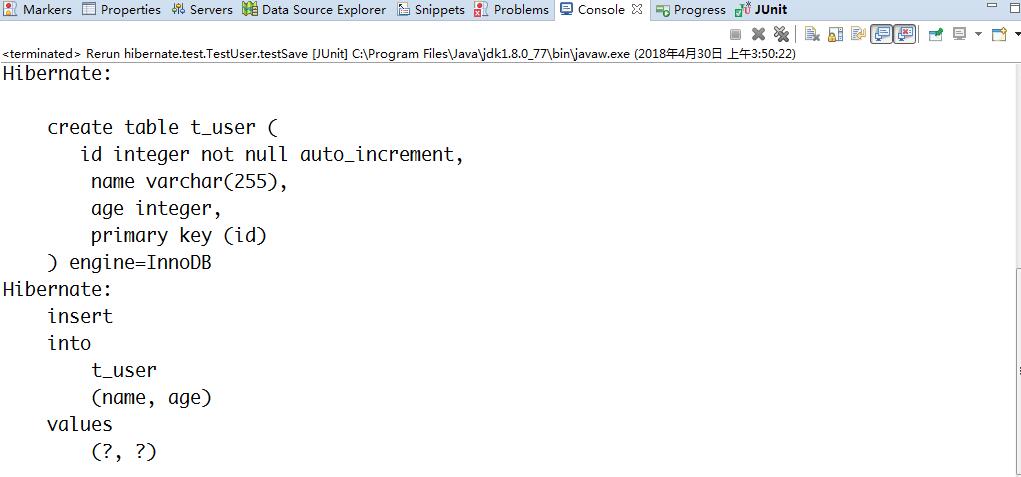
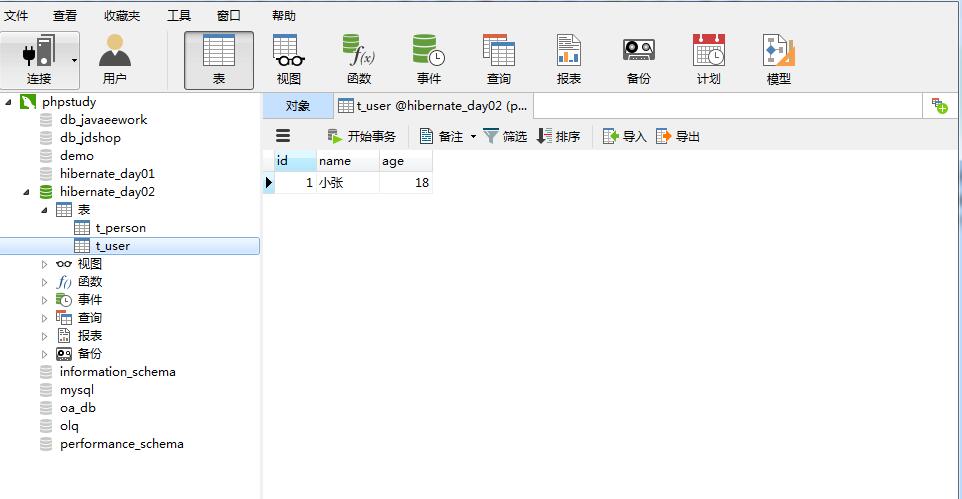