I. Asp.net Project
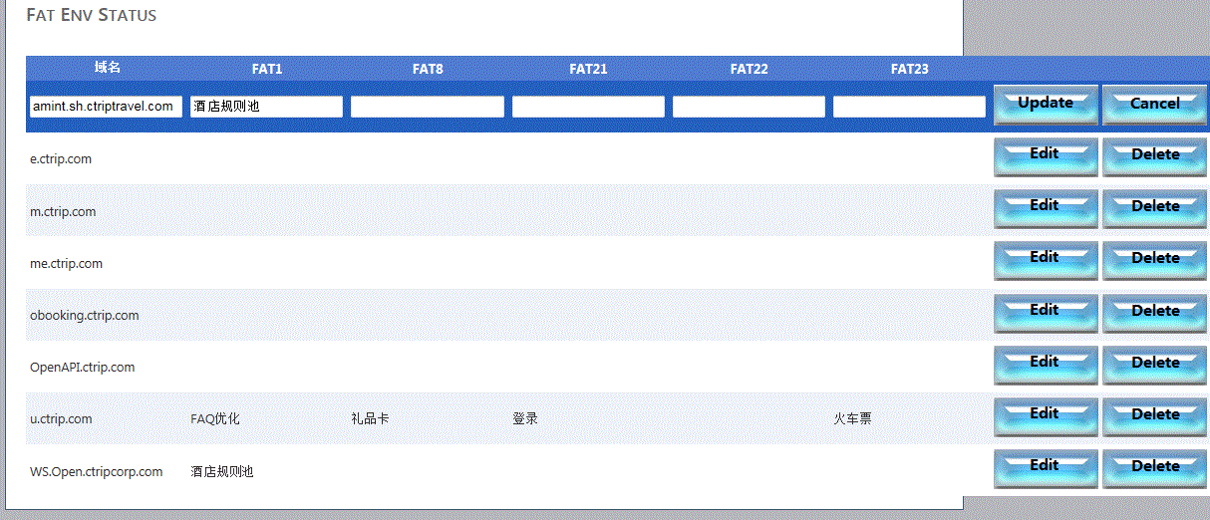
Use asp.net data control gridview to display data
The data source uses xml (the advantage is that the structure is standardized, it is convenient for rapid development, bypassing authority control, etc.)
Button uses imgbutton instead of using the text link button that comes with gridview. It is beautiful and easy to beautify.
Open the Tool bar Editor with VS2010, as follows:
1. Adding new pages
2. Drag GridView onto the page
3. Automatic Generation of Front Section Code
Open VS2010 and expand Solution to view the file structure:
Major resources:
1. Pictures
2. Data
3. Pages
4. Class files
Common Codes
HTML code for GridView control:
asp:GridView ID="GridView1" runat="server">
</asp:GridView>
Gridview control events are added and background code is generated as follows:
The front-end code is as follows:
<asp:GridView ID="gv_xml" runat="server" AutoGenerateColumns="False" OnRowCancelingEdit="gv_xml_RowCancelingEdit" OnRowUpdating="gv_xml_RowUpdating" OnRowEditing="gv_xml_RowEditing" OnRowDeleting="gv_xml_RowDeleting" CellPadding="4" ForeColor="#333333" GridLines="None" > <Columns> <asp:TemplateField HeaderText="domain name"> <ItemTemplate> <%# DataBinder.Eval(Container.DataItem,"Domain") %> </ItemTemplate> <EditItemTemplate> <asp:TextBox ID="Txtbox0" runat="server" Text='<%# DataBinder.Eval(Container.DataItem,"Domain") %>'></asp:TextBox> </EditItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="FAT1"> <ItemTemplate> <%# DataBinder.Eval(Container.DataItem, "FAT1")%> </ItemTemplate> <EditItemTemplate> <asp:TextBox ID="Txtbox1" runat="server" Text='<%#DataBinder.Eval(Container.DataItem,"FAT1") %>'></asp:TextBox> </EditItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="FAT8"> <ItemTemplate> <%# DataBinder.Eval(Container.DataItem, "FAT8")%> </ItemTemplate> <EditItemTemplate> <asp:TextBox ID="Txtbox2" runat="server" Text='<%#DataBinder.Eval(Container.DataItem,"FAT8") %>'></asp:TextBox> </EditItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="FAT21"> <ItemTemplate> <%# DataBinder.Eval(Container.DataItem, "FAT21")%> </ItemTemplate> <EditItemTemplate> <asp:TextBox ID="Txtbox3" runat="server" Text='<%#DataBinder.Eval(Container.DataItem,"FAT21") %>'></asp:TextBox> </EditItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="FAT22"> <ItemTemplate> <%# DataBinder.Eval(Container.DataItem, "FAT22")%> </ItemTemplate> <EditItemTemplate> <asp:TextBox ID="Txtbox4" runat="server" Text='<%#DataBinder.Eval(Container.DataItem,"FAT22") %>'></asp:TextBox> </EditItemTemplate> </asp:TemplateField> <asp:TemplateField HeaderText="FAT23"> <ItemTemplate> <%# DataBinder.Eval(Container.DataItem, "FAT23")%> </ItemTemplate> <EditItemTemplate> <asp:TextBox ID="Txtbox5" runat="server" Text='<%#DataBinder.Eval(Container.DataItem,"FAT23") %>'></asp:TextBox> </EditItemTemplate> </asp:TemplateField> <asp:TemplateField> <ItemTemplate> <asp:ImageButton ID="Button2" runat="server" Text="Edit" ImageUrl="~/Imgs/btnEdit.png" CommandName="Edit" /> <asp:ImageButton ID="ImageButton1" runat="server" ImageUrl="~/Imgs/btnDelete.png" CommandName="Delete" /> </ItemTemplate> <EditItemTemplate> <asp:ImageButton ID="Button1" runat="server" Text="Update" ImageUrl="~/Imgs/btnUpdate.png" CommandName="Update" /> <asp:ImageButton ID="Button3" runat="server" Text="Cancel" ImageUrl="~/Imgs/btnCancel.png" CommandName="Cancel" /> </EditItemTemplate> </asp:TemplateField> </Columns> <AlternatingRowStyle Wrap="False" BackColor="White" /> <EditRowStyle BackColor="#2461BF" /> <FooterStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" /> <HeaderStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" /> <PagerStyle BackColor="#2461BF" ForeColor="White" HorizontalAlign="Center" /> <RowStyle BackColor="#EFF3FB" /> <SelectedRowStyle BackColor="#D1DDF1" Font-Bold="True" ForeColor="#333333" /> <SortedAscendingCellStyle BackColor="#F5F7FB" /> <SortedAscendingHeaderStyle BackColor="#6D95E1" /> <SortedDescendingCellStyle BackColor="#E9EBEF" /> <SortedDescendingHeaderStyle BackColor="#4870BE" /> </asp:GridView>
The code corresponding to the background is as follows:
protected void gv_xml_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e) { gv_xml.EditIndex = -1; Databind(); } protected void gv_xml_RowEditing(object sender, GridViewEditEventArgs e) { gv_xml.EditIndex = e.NewEditIndex; Databind(); } protected void gv_xml_RowUpdating(object sender, GridViewUpdateEventArgs e) { string Xmlpath = String.Format("{0}\\APIData\\FatEnvData.xml", RootPath); GridViewRow row = gv_xml.Rows[e.RowIndex]; //Get the current row int numCell = row.Cells.Count; //Several columns of cells(Contain Edit and Delete 2 column) int currentRow = row.DataItemIndex; //Corresponding DataSet Corresponding row index DataSet ds = new DataSet(); ds.ReadXml(Xmlpath); DataRow dr; dr = ds.Tables[0].Rows[row.DataItemIndex]; string[] str = null; //This array defines the column name of the table str = new string[]{ "Domain", "FAT1", "FAT8", "FAT21", "FAT22", "FAT23"}; int j = 0; TextBox myTextBox = null; //Start with column 1, The last two columns are Edit and Delete for (int i = 0; i < numCell-1; i++) { myTextBox = row.Cells[i].FindControl("Txtbox"+i) as TextBox; //string cText = ((TextBox)row.Cells[i].Controls[0]).Text; dr[str[j]] = myTextBox.Text; j++; } ds.WriteXml(Xmlpath); //Write changes to Table.xml gv_xml.EditIndex = -1; Databind(); } protected void gv_xml_RowDeleting(object sender, GridViewDeleteEventArgs e) { string Xmlpath = String.Format("{0}\\APIData\\FatEnvData.xml", RootPath); GridViewRow row = this.gv_xml.Rows[e.RowIndex]; int curr = row.RowIndex; DataSet ds = new DataSet(); ds.ReadXml(Xmlpath); DataRow dr = ds.Tables[0].Rows[curr]; dr.Delete(); ds.WriteXml(Xmlpath); Databind(); }
When a page is loaded, data binding:
protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) Databind(); } //xml Data binding public void Databind() { var query = GetXMLData(); gv_xml.DataSource = query; gv_xml.DataBind(); } //query xml data private List<LinqXmlGridViewControl> GetXMLData() { var xDoc = XDocument.Load(Server.MapPath("~/APIData/FatEnvData.xml")); var query = (from LinqXmlGridViewControl in xDoc.Descendants("Server") select new LinqXmlGridViewControl() { Domain = LinqXmlGridViewControl.Element("Domain").Value, FAT1 = LinqXmlGridViewControl.Element("FAT1").Value, FAT8 = LinqXmlGridViewControl.Element("FAT8").Value, FAT21 = LinqXmlGridViewControl.Element("FAT21").Value, FAT22 = LinqXmlGridViewControl.Element("FAT22").Value, FAT23 = LinqXmlGridViewControl.Element("FAT23").Value }).ToList(); return query; }
III. Website Deployment
After the code is completed, you need to deploy it in IIS, open the Control Panel - "Control Panel Project -" Management Tool:
On the website, click the right mouse button to choose to add the website, as follows:
The application pool defaults to. Net4.0, otherwise the IIS website cannot start:
Physical path selection, local site file storage address. IP chooses local machine ip, default port 80
Host name is empty if there is a domain name that can be set.
Deployment completed
As shown below, the pool can be replaced here; the path can be reassigned;
Companies currently use tools to automatically configure, if there is a problem, you can login to the server for manual modification (site connection configuration, jump pointing, database connection string, code version view, etc.)