Linux Lower serial communication mainly includes the following steps

Flow chart of serial communication
I will introduce these steps one by one.
1. Open the serial port
Code (serial port is ttyUSB0)
When opening the serial port, you can add more content, such as judging whether the serial port is blocked, testing whether it is a terminal device, etc. These are necessary. Therefore, more complete and robust code flow is shown below than the basic code of opening the serial port above.
Open a more complete flow chart of serial port
Code:
Key Function Interpretation:
open
Function Description: For opening or creating a file, the file descriptor is returned if successful, otherwise - 1 is returned. The file descriptor returned by open must be the smallest unused descriptor.
Parametric interpretation:
pathname: file path name, serial port in linux It's seen as a document.
oflag: Some file mode choices can be set with the following parameters
- O_RDONLY Read-Only Mode
- O_WRONLY Write-Only Mode
- O_RDWR Read-Write Mode
- O_APPEND Writes to the end of the file each time it writes
- O_CREAT Creates the specified file if it does not exist
- O_EXCL returns - 1 if the file to be created already exists, and modifies the value of errno
- O_TRUNC empties the entire contents of a file if it exists and opens in write-only/read-write mode
- O_NOCTTY If the path name points to the terminal device, do not use the device as a control terminal.
-
O_NONBLOCK If the path name points to FIFO/block file/character file, set the opening and subsequent I/O of the file to non-blocking mode.
mode)
The following three constants are also selected for synchronous input and output
- O_DSYNC waits for physical I/O to finish before writing. Without affecting the reading of newly written data, it does not wait for the update of file attributes.
- O_RSYNC Read waits for all writes written to the same area to complete before proceeding
- O_SYNC waits for physical I/O to finish before writing, including updating I/O of file attributes
O_NDELAY indicates that it does not care about the state of the DCD signal (whether the other end of the port is activated or stopped).
fcntl
Function Description: According to the characteristics of the file descriptor to operate the file, return - 1 represents an error.
Description of parameters:
- fd: File Descriptor
- cmd: Command parameters
1. Copy an existing descriptor (cmd=F_DUPFD).
2. Obtain/set file descriptor tags (cmd=F_GETFD or F_SETFD).
3. Obtain/set the file status tag (cmd=F_GETFL or F_SETFL).
4. Obtain/set asynchronous I/O ownership (cmd=F_GETOWN or F_SETOWN).
5. Get/set record locks (cmd = F_GETLK, F_SETLK or F_SETLKW).
2. Initialization of Serial Port
- set baud rate
- Setting up data flow control
- Set the format of the frame (i.e. number of data bits, stop bits, check bits)
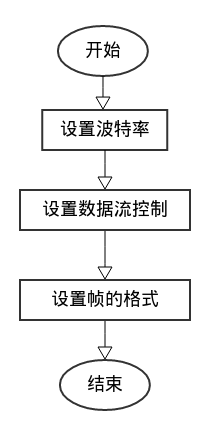
Before explaining this piece of code, we need to study the data structure of termios. Typical definitions of the smallest termios structure are as follows:
The above five structural member names represent:
- c_iflag: Input mode
- c_oflag: Output mode
- c_cflag: Control mode
- c_lflag: Local mode
- c_cc[NCCS]: Special Control Mode
Tcgetattr can initialize a termios structure corresponding to a terminal. The prototype of tcgetattr function is as follows:
This function call writes the value of the low-cost front-end interface variable to the structure pointed by the termios_p parameter. If these values are later modified, they can be reconfigured by calling the function tcsetattr.
There are three ways to modify the parameters actions, which are as follows:
- TCSANOW: Modify the value immediately
- TCSADRAIN: Modify the value after the current output is complete
- TCSAFLUSH: After the current output is complete, the value is modified, but any currently available input that has not been returned from the read call is discarded.
When queue_selector controls the operation of tcflush, the value can be one of the following parameters: TCIFLUSH clearly receives the data and does not read it out; TCOFLUSH clearly writes the data and does not send it to the terminal; TCIOFLUSH clears all I/O data being sent.
c_cflag represents control mode
- CLOCAL means ignoring the status lines of all modems. This is to ensure that the program does not occupy the serial port.
- CREAD stands for enabling character receivers to read and read input data from serial ports.
- CS5/6/7/8 denotes the use of 5/6/7/8 bits for sending or receiving characters.
- CSTOPB means that each character uses two stop bits.
- HUPCL means to suspend the modem when it is turned off.
- PARENB: Enables parity code generation and detection.
- PARODD: Use odd checks instead of even checks.
- BRKINT: An interrupt occurs when a termination state is detected in the input line.
- TGNBRK: Ignores the termination state in the input line.
- TCRNL: Converts the accepted carriage return character to a new line character.
- TGNCR: Ignore new lines accepted.
- INLCR: Converts the new line character received to a carriage return character.
- IGNPAR: Characters that ignore parity checking errors.
- INPCK: Perform parity checks on received characters.
- PARMRK: Mark parity checking errors.
- ISTRIP: Reduce all received characters to 7 bits.
- IXOFF: Enable software flow control for input.
- IXON: Enable software flow control for output.
In standard mode and non-standard mode, the subscripts of c_cc arrays have different values:
Standard mode:
- VEOF:EOF character
- VEOL:EOF Character
- VERASE:ERASE character
- VINTR:INTR character
- VKILL:KILL character
- VQUIT:QUIT character
- VSTART:START character
- VSTOP:STOP character
Non-standard mode:
- VINTR:INTR character
- VMIN:MIN value
- VQUIT:QUIT character
- VSUSP:SUSP character
- VTIME:TIME value
- VSTART:START character
- VSTOP:STOP character
cfsetispeed and cfsetospeed are used to set the baud rate of input and output. The function model is as follows:
Description of parameters:
- struct termios *termptr: pointer to termios structure
- speed_t speed: baud rate to be set
- Return value: Return 0 successfully or - 1 otherwise
In this way, all the initialization operations are completed.