Template Matching - Matching a single or multiple object in an image that is similar to a template
(1) Target matching function:
cvMatchTemplate( const CvArr* image, constCvArr* templ,
CvArr* result,int method );
Image
Templ template image
Result Matching Result is used to store the similarity between sliding window and template calculated by the following method
Method
With respect to matching methods, the results produced by different methods may have different meanings. Some of the returned values indicate that the greater the matching degree is, while some of the returned values indicate that the smaller the matching degree is, the better the matching degree is.
With regard to parameters, method:
CV_TM_SQDIFF square difference matching method: This method uses square difference to match; the best matching value is 0; the worse the matching, the bigger the matching value.
CV_TM_CCORR correlation matching method: This method uses multiplication operation; the larger the value, the better the matching degree.
CV_TM_CCOEFF correlation coefficient matching method: 1 represents perfect matching; 1 represents worst matching.
CV_TM_SQDIFF_NORMED Normalized Square Difference Matching Method
CV_TM_CCORR_NORMED normalized correlation matching method
CV_TM_CCOEFF_NORMED normalized correlation coefficient matching method
(2): The next step is to find the coordinates corresponding to the maximum value and the maximum value.
cvMinMaxLoc() Finds the Maximum and Minimum of a Matrix and the Corresponding Coordinates
cvMinMaxLoc( constCvArr* arr, double* min_val, double* max_val,
CvPoint* min_locCV_DEFAULT(NULL),
CvPoint* max_locCV_DEFAULT(NULL),
const CvArr* mask CV_DEFAULT(NULL) );
Single target matching results:
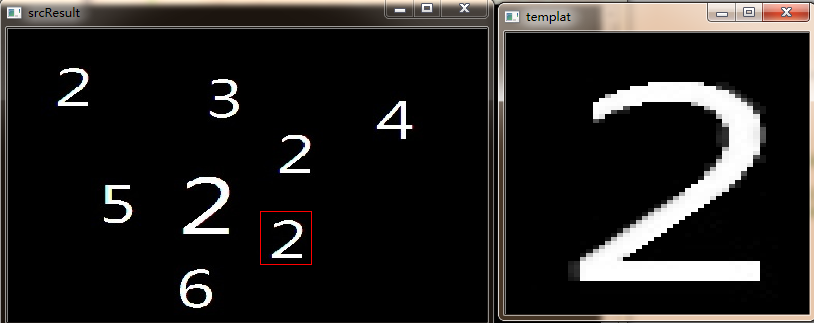
Code:
-
#include <iostream>
-
#include "cv.h"
-
#include "cxcore.h"
-
#include "highgui.h"
-
using namespace std;
-
int main()
-
{
-
IplImage *src = cvLoadImage("E:\\study_opencv_video\\lesson16_1\\images\\src.jpg", 0);
-
IplImage *srcResult = cvLoadImage("E:\\study_opencv_video\\lesson16_1\\images\\src.jpg", 3);
-
IplImage *templat = cvLoadImage("E:\\study_opencv_video\\lesson16_1\\images\\template.png", 0);
-
IplImage *result;
-
if(!src || !templat)
-
{
-
cout << "Failed to open the image"<< endl;
-
return 0;
-
}
-
int srcW, srcH, templatW, templatH, resultH, resultW;
-
srcW = src->width;
-
srcH = src->height;
-
templatW = templat->width;
-
templatH = templat->height;
-
if(srcW < templatW || srcH < templatH)
-
{
-
cout <<"Template cannot be smaller than original image" << endl;
-
return 0;
-
}
-
resultW = srcW - templatW + 1;
-
resultH = srcH - templatH + 1;
-
result = cvCreateImage(cvSize(resultW, resultH), 32, 1);
-
cvMatchTemplate(src, templat, result, CV_TM_SQDIFF);
-
double minValue, maxValue;
-
CvPoint minLoc, maxLoc;
-
cvMinMaxLoc(result, &minValue, &maxValue, &minLoc, &maxLoc);
-
cvRectangle(srcResult, minLoc, cvPoint(minLoc.x + templatW, minLoc.y+ templatH), cvScalar(0,0,255));
-
cvNamedWindow("srcResult", 0);
-
cvNamedWindow("templat", 0);
-
cvShowImage("srcResult", srcResult);
-
cvShowImage("templat", templat);
-
cvWaitKey(0);
-
cvReleaseImage(&result);
-
cvReleaseImage(&templat);
-
cvReleaseImage(&srcResult);
-
cvReleaseImage(&src);
-
return 0;
-
}
(3) Multi-objective matching:
Result:
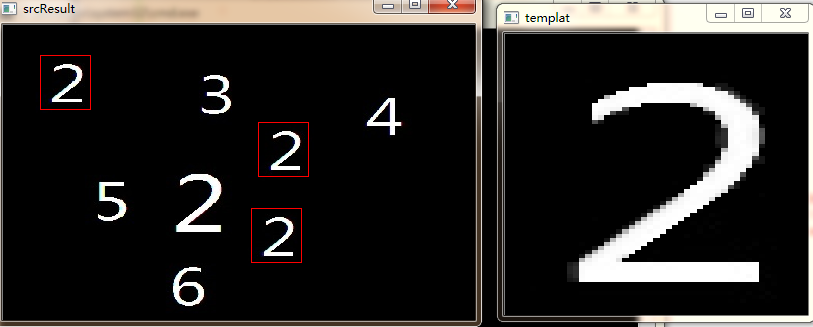
Code:
-
#include <iostream>
-
#include "cv.h"
-
#include "cxcore.h"
-
#include "highgui.h"
-
using namespace std;
-
-
CvPoint getNextMinLoc(IplImage *result, CvPoint minLoc, int maxVaule, int templatW, int templatH)
-
{
-
-
-
int startX = minLoc.x - templatW;
-
int startY = minLoc.y - templatH;
-
int endX = minLoc.x + templatW;
-
int endY = minLoc.y + templatH;
-
if(startX < 0 || startY < 0)
-
{
-
startX = 0;
-
startY = 0;
-
}
-
if(endX > result->width - 1 || endY > result->height - 1)
-
{
-
endX = result->width - 1;
-
endY = result->height - 1;
-
}
-
int y, x;
-
for(y = startY; y < endY; y++)
-
{
-
for(x = startX; x < endX; x++)
-
{
-
cvSetReal2D(result, y, x, maxVaule);
-
}
-
}
-
-
double new_minVaule, new_maxValue;
-
CvPoint new_minLoc, new_maxLoc;
-
cvMinMaxLoc(result, &new_minVaule, &new_maxValue, &new_minLoc, &new_maxLoc);
-
return new_minLoc;
-
-
}
-
int main()
-
{
-
IplImage *src = cvLoadImage("E:\\study_opencv_video\\lesson16_1\\images\\src.jpg", 0);
-
IplImage *srcResult = cvLoadImage("E:\\study_opencv_video\\lesson16_1\\images\\src.jpg", 3);
-
IplImage *templat = cvLoadImage("E:\\study_opencv_video\\lesson16_1\\images\\template.png", 0);
-
IplImage *result;
-
if(!src || !templat)
-
{
-
cout << "Failed to open the picture" << endl;
-
return 0;
-
}
-
int srcW, srcH, templatW, templatH, resultH, resultW;
-
srcW = src->width;
-
srcH = src->height;
-
templatW = templat->width;
-
templatH = templat->height;
-
if(srcW < templatW || srcH < templatH)
-
{
-
cout << "Template can't be smaller than original" << endl;
-
return 0;
-
}
-
resultW = srcW - templatW + 1;
-
resultH = srcH - templatH + 1;
-
result = cvCreateImage(cvSize(resultW, resultH), 32, 1);
-
cvMatchTemplate(src, templat, result, CV_TM_SQDIFF);
-
double minValue, maxValue;
-
CvPoint minLoc, maxLoc;
-
cvMinMaxLoc(result, &minValue, &maxValue, &minLoc, &maxLoc);
-
cvRectangle(srcResult, minLoc, cvPoint(minLoc.x + templatW, minLoc.y+ templatH), cvScalar(0,0,255));
-
CvPoint new_minLoc;
-
-
-
new_minLoc = getNextMinLoc(result, minLoc, maxValue, templatW, templatH);
-
cvRectangle(srcResult, new_minLoc, cvPoint(new_minLoc.x + templatW, new_minLoc.y+ templatH), cvScalar(0,0,255));
-
-
new_minLoc = getNextMinLoc(result, new_minLoc, maxValue, templatW, templatH);
-
cvRectangle(srcResult, new_minLoc, cvPoint(new_minLoc.x + templatW, new_minLoc.y+ templatH), cvScalar(0,0,255));
-
cvNamedWindow("srcResult", 0);
-
cvNamedWindow("templat", 0);
-
cvShowImage("srcResult", srcResult);
-
cvShowImage("templat", templat);
-
cvWaitKey(0);
-
cvReleaseImage(&result);
-
cvReleaseImage(&templat);
-
cvReleaseImage(&srcResult);
-
cvReleaseImage(&src);
-
return 0;
-
}
Author: Villager from: http://blog.csdn.net/lu597203933 Reprint or share are welcome, but be sure to state the source of the article. (Sina Weibo: Xiaocun Mayor zack, welcome to exchange