Write a mystruts framework
Mvc mode: Model Model View tries to Control the controller
Control, Controller
Servlet acts as a controller!
Getting request data encapsulation [BeanUtils can be optimized, (call method? ]
Call Service to process business logic.
Skip (Forwarding/Redirecting) [Skip Code Write Death]
Summary of traditional mvc development:
1. Flexible jump code
2. Write servlet every time, configure servlet in web.xml!
(Configuration Purpose: Request, Servlet Processing Class)
package com.xp.entity;
public class User {
private String name;
private String pwd;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
}
package com.xp.dao;
import com.xp.entity.User;
/**
* User login and registration
*/
public class UserDao {
// Simulated landing
public User login(User user){
if ("tom".equals(user.getName()) && "888".equals(user.getPwd()) ){
// Landing successfully
return user;
}
// Landing failed
return null;
}
// Simulated registration
public void register(User user) {
System.out.println("Successful registration: Users," + user.getName());
}
}
package com.xp.service;
import com.xp.dao.UserDao;
import com.xp.entity.User;
public class UserService {
private UserDao ud = new UserDao();
// Simulated landing
public User login(User user){
return ud.login(user);
}
// Simulated registration
public void register(User user) {
ud.register(user);
}
}
package com.xp.framework.action;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.xp.entity.User;
import com.xp.service.UserService;
/**
* Action Represents action class 1. A servlet corresponds to an action 2. action is responsible for handling specific requests
*
*/
public class LoginAction {
public Object execute(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
return null;
}
/**
* Processing landing requests
*/
public Object login(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
Object uri = null;
// 1. Get request data and encapsulate it
String name = request.getParameter("name");
String pwd = request.getParameter("pwd");
User user = new User();
user.setName(name);
user.setPwd(pwd);
// 2. Call Service
UserService userService = new UserService();
User userInfo = userService.login(user);
// 3. jump
if (userInfo == null) {
// Landing failed
// request.getRequestDispatcher("/login.jsp").forward(request,
// response);
// uri = request.getRequestDispatcher("/login.jsp");
uri = "loginFaild"; // loginFaild = /login.jsp
} else {
// Landing successfully
request.getSession().setAttribute("userInfo", userInfo);
// home page
// response.sendRedirect(request.getContextPath() + "/index.jsp");
// uri = "/index.jsp";
uri = "loginSuccess"; // loginSuccess = /index.jsp
}
return uri;
}
}
package com.xp.framework.action;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.xp.entity.User;
import com.xp.service.UserService;
public class RegisterAction {
public Object register(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
Object uri;
// 1. Get request data and encapsulate it
String name = request.getParameter("name");
String pwd = request.getParameter("pwd");
User user = new User();
user.setName(name);
user.setPwd(pwd);
// 2. Call Service
UserService userService = new UserService();
userService.register(user);
// 3. jump
// request.getRequestDispatcher("/login.jsp").forward(request, response);
//uri = request.getRequestDispatcher("/login.jsp");
return "registerSuccess"; //Returns the registered tag; registerSuccess = login. JSP
}
}
package com.xp.framework.bean;
public class Result {
// Result markers for jumps
private String name;
// Jump type, default forwarding; "redirect" is redirect
private String type;
// Jump pages
private String page;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getPage() {
return page;
}
public void setPage(String page) {
this.page = page;
}
}
package com.xp.framework.bean;
import java.util.Map;
/**
* Encapsulating action nodes
* <action name="login" class="com.xp.framework.action.LoginAction" method="login">
<result name="success" type="redirect">/index.jsp</result>
<result name="loginFaild">/login.jsp</result>
</action>
*
*/
public class ActionMapping {
// Request path name
private String name;
// Processing the full name of the aciton class
private String className;
// processing method
private String method;
// Result View Collection
private Map<String,Result> results;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className;
}
public String getMethod() {
return method;
}
public void setMethod(String method) {
this.method = method;
}
public Map<String, Result> getResults() {
return results;
}
public void setResults(Map<String, Result> results) {
this.results = results;
}
}
package com.xp.framework.bean;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.dom4j.Document;
import org.dom4j.Element;
import org.dom4j.io.SAXReader;
/**
* Load the configuration file and encapsulate all the real mystruts.xml
*
*/
public class ActionMappingManager {
// Save the collection of action s
private Map<String,ActionMapping> allActions ;
public ActionMappingManager(){
allActions = new HashMap<String,ActionMapping>();
// Initialization
this.init();
}
/**
* Returns the mapping object of Action based on the request path name
* @param actionName Current request path
* @return Returns the AcitonMapping object representing the action node in the configuration file
*/
public ActionMapping getActionMapping(String actionName) {
if (actionName == null) {
throw new RuntimeException("There is a mistake in the incoming parameter. Please check it. struts.xml Configuration path.");
}
ActionMapping actionMapping = allActions.get(actionName);
if (actionMapping == null) {
throw new RuntimeException("Path in struts.xml I can't find it. Please check it.");
}
return actionMapping;
}
// Initialize the allActions collection
private void init() {
/********DOM4J Read configuration file ***************/
try {
// 1. Get the parser
SAXReader reader = new SAXReader();
// Get the src/mystruts.xml file stream
InputStream inStream = this.getClass().getResourceAsStream("/mystruts.xml");
// 2. Loading files
Document doc = reader.read(inStream);
// 3. get roots
Element root = doc.getRootElement();
// 4. Get the package node
Element ele_package = root.element("package");
// 5. Get all action subnodes under the package node
List<Element> listAction = ele_package.elements("action");
// 6. Traversal, encapsulation
for (Element ele_action : listAction) {
// 6.1 Encapsulates an Action Mapping object
ActionMapping actionMapping = new ActionMapping();
actionMapping.setName(ele_action.attributeValue("name"));
actionMapping.setClassName(ele_action.attributeValue("class"));
actionMapping.setMethod(ele_action.attributeValue("method"));
// 6.2 Encapsulates all results views under the current aciton node
Map<String,Result> results = new HashMap<String, Result>();
// Get all result subnodes under the current action node
Iterator<Element> it = ele_action.elementIterator("result");
while (it.hasNext()) {
// Each element of the current iteration is <result...>.
Element ele_result = it.next();
// Encapsulated object
Result res = new Result();
res.setName(ele_result.attributeValue("name"));
res.setType(ele_result.attributeValue("type"));
res.setPage(ele_result.getTextTrim());
// Add to Collection
results.put(res.getName(), res);
}
// Set it to action Mapping
actionMapping.setResults(results);
// 6.x action Mapping added to map collection
allActions.put(actionMapping.getName(), actionMapping);
}
} catch (Exception e) {
throw new RuntimeException("Initialization error at startup",e);
}
}
}
package com.xp.framework;
import java.io.IOException;
import java.lang.reflect.Method;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.xp.framework.bean.ActionMapping;
import com.xp.framework.bean.ActionMappingManager;
import com.xp.framework.bean.Result;
/**
* Core controller, this project has only one servlet
* 1. Intercept all *. action suffix requests
* 2. Request: http://localhost:8080/mystruts/login.action
* http://localhost:8080/mystruts/register.action
*/
public class ActionServlet extends HttpServlet{
private ActionMappingManager actionMappingManager;
// Execute only once (when you want to start)
@Override
public void init() throws ServletException {
System.out.println("1111111111111111ActionServlet.init()");
actionMappingManager = new ActionMappingManager();
}
// http://localhost:8080/mystruts/login.action
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
try {
// 1. Get the request uri, get the request path name [login]
String uri = request.getRequestURI();
// Get login
String actionName=uri.substring(uri.lastIndexOf("/")+1, uri.indexOf(".action"));
// 2. Read the configuration file according to the path name and get the full name of the class [cn. action. LoginAction]
ActionMapping actionMapping = actionMappingManager.getActionMapping(actionName);
String className = actionMapping.getClassName();
// Processing method of current request [method="login"]
String method = actionMapping.getMethod();
// 3. Reflection: Create objects and call methods; Get tags returned by methods
Class<?> clazz = Class.forName(className);
Object obj = clazz.newInstance(); //LoginAction loginAction = new LoginAction();
Method m = clazz.getDeclaredMethod(method, HttpServletRequest.class,HttpServletResponse.class );
// The tag returned by the calling method
String returnFlag = (String) m.invoke(obj, request, response);
// 4. Get the tag, read the configuration file to get the page and jump type corresponding to the tag.
Result result = actionMapping.getResults().get(returnFlag);
// type
String type = result.getType();
// page
String page = result.getPage();
// Jump
if ("redirect".equals(type)) {
response.sendRedirect(request.getContextPath() + page);
} else {
request.getRequestDispatcher(page).forward(request, response);
}
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doGet(req, resp);
}
}
package com.xp.servlet;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.xp.framework.action.LoginAction;
// Controller
public class LoginServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Create an Action object and call the login method
LoginAction loginAction = new LoginAction();
Object uri = loginAction.login(request, response);
// Jump
if (uri instanceof String) {
response.sendRedirect(request.getContextPath() + uri.toString());
} else {
((RequestDispatcher) uri).forward(request, response);
}
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
package com.xp.servlet;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.xp.framework.action.RegisterAction;
public class RegisterServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
RegisterAction registerAction = new RegisterAction();
Object uri = registerAction.register(request, response);
// Configuration File - "jsp"
// Jump
if (uri instanceof String) {
response.sendRedirect(request.getContextPath() + uri.toString());
} else {
((RequestDispatcher)uri).forward(request, response);
}
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
<?xml version="1.0" encoding="UTF-8"?>
<mystruts>
<package>
<!-- Configure request path, and process action Class relations -->
<!--
1. Request Path and Processing Action Relationship
/login = LoginAction login
success = /index.jsp Landing successfully(redirect)
loginFaild = /login.jsp Landing failed
-->
<action name="login" class="com.xp.framework.action.LoginAction" method="login">
<result name="loginSuccess" type="redirect">/index.jsp</result>
<result name="loginFaild">/login.jsp</result>
</action>
<action name="register" class="com.xp.framework.action.RegisterAction" method="register">
<result name="registerSuccess">/login</result>
</action>
</package>
</mystruts>
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>index</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
//Welcome, ${sessionScope.userInfo.name}
</body>
</html>
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>login</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<form action="${pageContext.request.contextPath }/login.action" name="frmLogin" method="post">
//User name: <input type="text" name="name"> <br/>
//Password: <input type="text" name="pwd"> <br/>
<input type="submit" value="Land"> <br/>
</form>
</body>
</html>
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>login</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<form action="${pageContext.request.contextPath }/register.action" name="frmRegister" method="post">
//User name: <input type="text" name="name"> <br/>
//Password: <input type="text" name="pwd"> <br/>
<input type="submit" value="register"> <br/>
</form>
</body>
</html>
Web.Xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>mystruts</display-name>
<!-- Core Controller -->
<servlet>
<servlet-name>ActionServlet</servlet-name>
<servlet-class>com.xp.framework.ActionServlet</servlet-class>
<!-- Start-up execution servlet Initialization method -->
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>ActionServlet</servlet-name>
<url-pattern>*.action</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
2.struts First Casestruts of application framework based on mvc mode
Struts is a framework based on mvc mode!
(struts is also a servlet package to improve development efficiency! )
Struts development steps:
1. web project, introducing struts - jar package
2. Introducing the core functions of struts in web.xml
Configure filters
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>struts20150313</display-name>
<!-- Introduce struts Core filter -->
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter.class</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
3. Developing action
package com.xp.action;
import com.opensymphony.xwork2.ActionSupport;
//Developing action: Processing requests
public class HelloAction extends ActionSupport{
// Processing request
public String execute() throws Exception {
System.out.println("Visited. action,Requests are being processed");
System.out.println("call service");
return "success";
}
}
4. To configure action
src/struts.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts SYSTEM "http://struts.apache.org/dtds/struts-2.0.dtd" PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN">
<struts>
<package extends="struts-default" name="xxxx">
<action name="hello" method="execute" class="com.xp.action.HelloAction">
<result name="success">/success.jsp</result>
</action>
</package>
</struts>
3. Structures Explanation
Positional role of SSH framework in mvc mode:
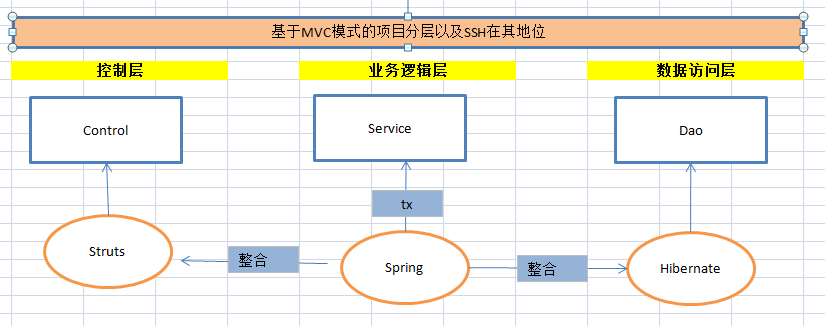
Framework:
The framework in software is a semi-finished product; our project development needs to be based on the framework!
Because the framework has implemented some functions, so it can improve the efficiency of development!
B. Struts 2 Framework
Struts 1 is the earliest framework based on mvc mode.
Struts 2 is based on Struts 1 and integrates the functions of xwork.
In other words, Struts 2 = struts1 + xwork
The Struts2 framework implements some functions in advance:
1. Automatic encapsulation of request data
2. File upload function
3. Simplification of internationalization function
4. Data validation function
4. Struts 2 development process
Introduction of jar files
commons-fileupload-1.2.2.jar
commons-io-2.0.1.jar
Strts2-core-2.3.4.1.jar [Strts2 Core Function Package]
xwork-core-2.3.4.1.jar
ognl-3.0.5.jar [Ognl expression function support table]
commons-lang3-3.1.jar [struts extension to java.lang package]
freemarker-2.3.19.jar [struts tag template library jar file]
javassist-3.11.0.GA.jar
Configuring web.xml
Tomcat starts - - loads its own web.xml - - loads web.xml for all projects
By introducing filters into the web.xml of the project,
- Initialization of the core functions of Struts is accomplished through filters
filter [
init / Start execution
doFilter / Access Execution
destroy
]
<!-- Introduce struts Core filter -->
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
struts2-core-2.3.4.1.jarStruts PrepareAndExecute Filter is the core filter
Be careful:
The versions of struts used are different, and the core filter classes are different!
Developing Action
Be careful:
1. action classes, also known as action classes; ActionSupport classes are generally inherited
That is, the class that handles the request (the action class in struts replaces the previous servlet)
2. Business methods in action to handle specific requests
- Must return to String
The method cannot have parameters
public class HelloAction extends ActionSupport {
// Processing request
public String execute() throws Exception {}
}
//Configure struts.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts SYSTEM "http://struts.apache.org/dtds/struts-2.0.dtd" PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN">
<struts>
<package extends="struts-default" name="xxxx">
<action name="hello" method="execute" class="com.xp.action.HelloAction">
<result name="success">/success.jsp</result>
</action>
</package>
</struts>
5. Struts 2 execution process
c.Struts2 execution process
Server startup:
Loading project web.xml
Create Struts core filter object and execute filter_init()
struts-default.xml, Initialization of Core Functions
Strts-plugin.xml, Strts-related plug-ins
struts.xml Configuration Files Written by Users
Visit:
3. User accesses Action, server finds the corresponding aciton configuration according to the access path name, and creates action object.
4. Execute 18 interceptors defined in the default interceptor stack
5. Business Processing Method for Executing action
6.struts-default.xml, detailed
Directory: struts 2-core-2.3.4.1.jar/struts-default.xml
Content:
1. The bean node specifies the type of object struts create at run time
2. Specify struts-default packages [user-written packages (struts.xml) inherit the packages as well]
The package struts-default package defines:
a. Types of jump results
Disatcher Forwarding, not Default Forwarding
redirect
redirectAction redirect to action resource
stream
b. Define all interceptors
Thirty-two interceptors are defined!
To facilitate interceptor reference, interceptors can be referenced by defining stacks.
At this point, if the stack is referenced, the interceptor in the stack will be referenced!
defaultStack
Default stack, which defines 18 interceptors to be executed by default!
c. Interceptor stack executed by default, action executed by default
<default-interceptor-ref name="defaultStack"/>
<default-class-ref class="com.opensymphony.xwork2.ActionSupport" />
<interceptor
name="prepare" class="com.opensymphony.xwork2.interceptor.PrepareInterceptor"/>
<interceptor
name="params" class="com.opensymphony.xwork2.interceptor.ParametersInterceptor"/>
Interceptors (see first):
The interceptor function is similar to the filter function.
Difference:
Common: intercept resources!
Difference:
Filter, interceptor all resources can; (/ index.jsp/servlet/img/css/js)
Interceptor, intercepts only action requests.
Interceptor is the concept of struts and can only be used in struts.
Filter is the concept of servlet, which can be used in struts project and servlet project.
// Interview Question: When does the interceptor execute? (Access/Start) Execute action class creation first, and interceptor first?
// 18 interceptors are executed sequentially when users visit.
// ---> 2. Execute the creation of Action class first, then the interceptor; finally, the interceptor executes, and then the business method.
package com.xp.b_action;
public class User {
private String userName;
private String pwd;
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
}
package com.xp.b_action;
import java.util.Map;
import com.opensymphony.xwork2.ActionContext;
import com.opensymphony.xwork2.ActionSupport;
public class UserAction extends ActionSupport{
// Interview Question: When does the interceptor execute? (Access/Start) Execute action class creation first, and interceptor first?
// 18 interceptors are executed sequentially when users visit.
//---> 2. Execute the creation of Action class first, then the interceptor; finally, the interceptor executes, and then the business method.
public UserAction() {
System.out.println("UserAction.enclosing_method()");
}
/**
*
private String userName;
private String pwd;
public void setUserName(String userName) {
this.userName = userName;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
*/
private User user = new User();
public void setUser(User user) {
this.user = user;
}
public User getUser() {
return user;
}
public String login() {
// Get the username password
System.out.println(user.getUserName());
System.out.println(user.getPwd());
// Save the data to the domain
ActionContext ac = ActionContext.getContext();
// Get the map representing the request
Map<String, Object> request = ac.getContextMap();
// Get a map representing session
Map<String, Object> session = ac.getSession();
// Get a map representing servletContext
Map<String, Object> application = ac.getApplication();
// Preservation
request.put("request_data", "request_data");
session.put("session_data", "session_data");
application.put("application_data", "application_data");
return "login";
}
}
<action name="login" class="com.xp.b_action.UserAction" method="login">
<result name="login">/index.jsp</result>
</action>
d. Common problemsQuestion 1: Struts.xml configuration file is not prompted
Solve a:
Find the struts-2.0.dtd file and copy it to a directory: d:/dtd/. (Do not use Chinese directory)
Let MyEclipse be associated with the dtd file above.
windows preferences - - Search xml catalog
To configure:
Location: The dtd directory configured above
Key: -//Apache Software Foundation//DTD Struts Configuration 2.0//EN
Solve b:
Or,
Let the machine connect to the Internet, the tool will automatically download dtd files, cached to MyEclipse!
7. Detailed configuration
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <!-- A package defines a package. Package function, manage action. (Usually, a business template uses a package) The name of the name package; the name of the package cannot be repeated; Which package extends the current package inherits from In struts, packages must inherit struts-default Packages defined by struts-default in struts-default.xml abstract Represents that the current package is an abstract package; there can be no action definition in the abstract package, otherwise runtime errors will be reported abstract=true is only used when the current package is inherited by other packages! Such as: <package name="basePackage" extends="struts-default" abstract="true"></package> <package name="user" extends="basePackage"> Namespace namespace, default "/" As part of the path Access path = http://localhost:8080/project/namespace/ActionName Mapping relationship between Action configuration request path and Action class name Request Path name Full name of aciton class for class request processing method Request Processing method result Name action processing method return value type Jump Result type Pages that specify jumps in the tag body --> <package name="user" extends="struts-default" namespace="/"> <action name="login" class="cn.itcast.b_execute.UserAction" method="login"> <result name="login">/index.jsp</result> </action> </package> </struts> Or introduce other configuration files <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <! -- Struts loads this general configuration file at runtime: src/struts.xml - >. <! - Introduce all other configuration files into the master configuration file - >. <include file="com/xp/a_action/hello.xml"></include> <include file="com/xp/b_execute/config.xml"></include> </struts>