Blog content is used for learning and sharing. If you have any questions, please leave a comment.
About the author:
Programmer: ellende
blog: http://blog.csdn.NET/ellende
email: yangh.personal@qq.com
Reprinted please indicate the source, cite some online blogs, if there is infringement, please contact me.
In this paper, the intelligent driving platform H5 data provided by Baidu Open Source is read, and the H5 format file is parsed by python.
Baidu Data Download Address:
http://roadhackers.baidu.com/#downloads
The download contains three files: training set data (4.xG), training set results (several megabytes), test set data (5.xG), and more data after decompression. The following are the data analysis instructions.
1. Training set results
#!usr/bin/python # -*- coding: utf-8 -*- import cv2 import numpy as np import h5py import xlwt from datetime import datetime def write_data_to_excel(name,result): # Instantiate a Workbook() object (excel file) wbk = xlwt.Workbook() # Create an excel sheet called Sheet1. The cell_overwrite_ok =True here is to be able to repeat operations on the same cell. sheet = wbk.add_sheet('Sheet1',cell_overwrite_ok=True) # Get the current date and get a datetime object such as: (2016, 8, 9, 23, 12, 23, 424000) today = datetime.today() # The datetime object to be retrieved is taken only by date such as 2016-8-9 today_date = datetime.date(today) # Traverse through each element in result. for i in xrange(len(result)): #Traversing through each child element of result, for j in xrange(len(result[i])): #Write each element of each row into excel by line number i and column number j. sheet.write(i,j,result[i][j]) # Save the passed name + current date as excel name. wbk.save(name+str(today_date)+'.xls') f = h5py.File('D:\\AllDownloadFiles\\train-result-119\\119.h5', 'r') dset = f[f.keys()[0]] #f.keys() has only one key value: u'attrs' data = np.array(dset[:,:]) # Data writing to excel table write_data_to_excel('D:\\AllDownloadFiles\\train-result-119\\', data);
The data format is as follows:
2. Training set data
max_num limits the maximum number and can be resized.#!usr/bin/python # -*- coding: utf-8 -*- import cv2 import numpy as np import h5py import xlwt def saveOnePic(fh, data_type, save_dir): #data_type = "%.3f" % float(index_num) dset = fh[data_type] data = np.array(dset[:,:,:]) # Store a picture filePath = save_dir+'\\'+data_type+'.jpg' cv2.imwrite(filePath, data) if __name__=="__main__": f = h5py.File('D:\\AllDownloadFiles\\train-data-119\\119.h5', 'r') max_num = 100 file_len = len(f.keys()) for index in range(0,max_num-1): if index < file_len: index_type = f.keys()[index] saveOnePic(f, index_type, 'D:\\AllDownloadFiles\\train-data-119')
Here are the parsed pictures:
3. Test set data
#!usr/bin/python # -*- coding: utf-8 -*- import cv2 import numpy as np import h5py import xlwt def saveOnePic(fh, data_type, save_dir): #data_type = "%.3f" % float(index_num) dset = fh[data_type] data = np.array(dset[:,:,:]) # Store a picture filePath = save_dir+'\\'+data_type+'.jpg' cv2.imwrite(filePath, data) if __name__=="__main__": f = h5py.File('D:\\AllDownloadFiles\\test-part01.h5\\testfile_part01.h5', 'r') max_num = 100 file_len = len(f.keys()) for index in range(0,max_num-1): if index < file_len: index_type = f.keys()[index] saveOnePic(f, index_type, 'D:\\AllDownloadFiles\\test-part01.h5')
max_num limits the maximum number and can be resized.
Here are the parsed pictures:
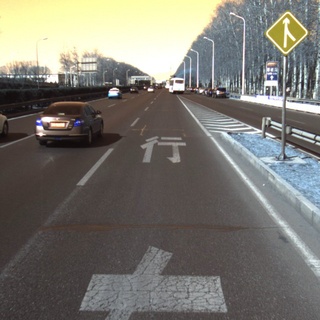
After parsing, we can use these image data to develop the algorithm. Come on.~