Today, we do an activity of graph rotation. When we get the dom element, we find the value of $(obj).css('transform'); we find several kinds of situations:
(1) Code first
<!DOCTYPE HTML> <html> <head> <meta charset=UTF-8> <title>recursion</title> <style> #div { margin-top: 90px; -webkit-transform: rotate(10deg); transform: rotate(10deg); } </style> </head> <body> <button>rotate</button> <div id="div" style="width: 300px; height: 300px; border: 1px solid black; ">ddd</div> </body> <!-- <script type="text/javascript" src="http://apps.bdimg.com/libs/jquery/2.1.4/jquery.min.js"></script> --> <script src="https://cdn.bootcss.com/zepto/1.2.0/zepto.js"></script> <script src="transform.js"></script> </html>
//transform.js $(function() { var test = function() { var reg = /(rotate\([\-\+]?((\d+)(deg))\))/i; var wt = $('#div').css('transform'), wts = wt.match(reg); var $2 = RegExp.$2; console.log($2); div.style['-webkit-transform'] = wt.replace($2, parseFloat(RegExp.$3) + 10 + RegExp.$4); } $('button').on('click', function() { test() }); });
Note: In this case, without setting the interline style, both zepto and jquery get a matrix
(2) Code first and set the inter-line style
1. When zepto is used, the results are shown in the figure.<!DOCTYPE HTML> <html> <head> <meta charset=UTF-8> <title>recursion</title> <style> #div { margin-top: 90px; -webkit-transform: rotate(10deg); transform: rotate(10deg); } </style> </head> <body> <button>rotate</button> <div id="div" style="width: 300px; height: 300px; border: 1px solid black; transform: rotate(10deg) scale(1.1);">ddd</div> </body> <!-- <script type="text/javascript" src="http://apps.bdimg.com/libs/jquery/2.1.4/jquery.min.js"></script> --> <script src="https://cdn.bootcss.com/zepto/1.2.0/zepto.js"></script> <script src="transform.js"></script> </html>
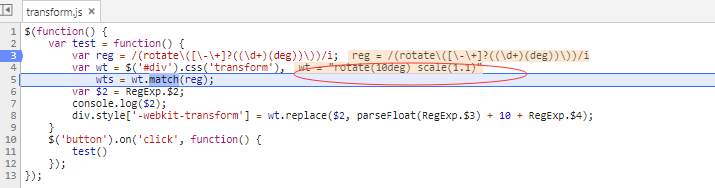
Obviously, what we get is the value of the transform we set.
But when we use jquery, the result is still a matrix
So, if you want to get the value of transform, you can replace it with zepto.
Or it can be obtained directly in the following way
$(function() { var supportCssArr = ["transform", "webkitTransform", "WebkitTransform"], styles = $('div')[0].style, supportCss; $.each(supportCssArr, function(i, item) { if (styles[item] != undefined) { supportCss = item; return false; } }); var wt = $('#div')[0].style[supportCss]; });
Note: Set to interline style.