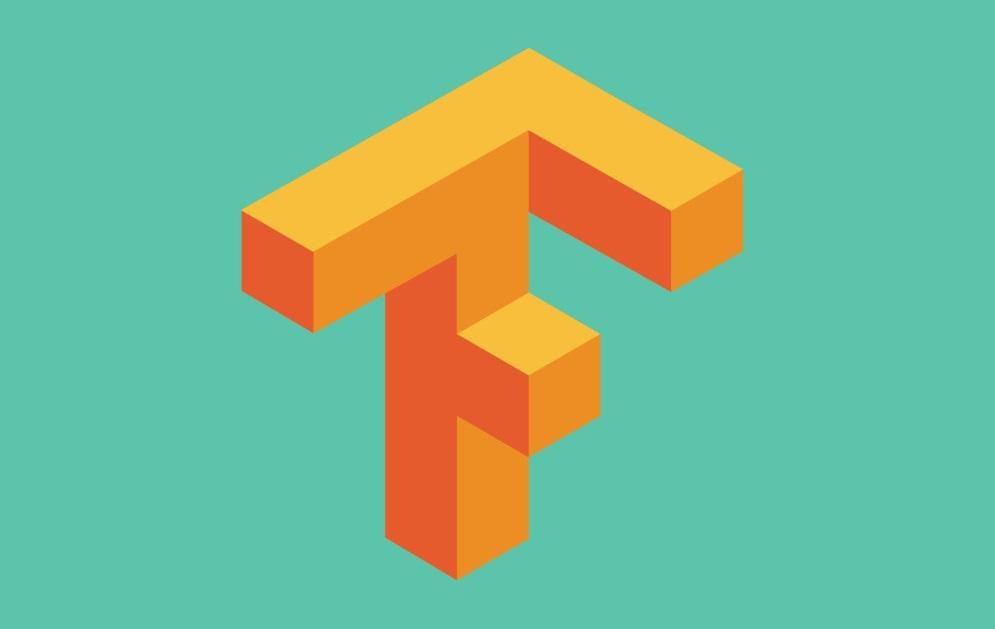
TensorFlow
This article is about how to build TensorFlow under Aliyun Student Server
OS Ubuntu 16.04 64-bit python 3.5
1. Updating Software Sources
$ apt-get update
2. Install Python 3.5
$ apt-get install python3.5 $ cp /usr/bin/python /usr/bin/python_bak #backups $ rm /usr/bin/python #delete $ ln -s /usr/bin/python3.5 /usr/bin/python #Set default to Python 3.5
Enter the python command to view the current default Python version

Python 3.5
3. Install TensorFlow
$ apt-get install python3-pip $ pip3 install tensorflow # Python 3.n; CPU support (no GPU support)
locale.Error: unsupported locale setting problem (language environment configuration problem) may be encountered when installing TensorFlow with pip3
Solution
Step 1
$ locale locale: Cannot set LC_ALL to default locale: No such file or directory LANG=en_US.UTF-8 LANGUAGE= LC_CTYPE="en_US.UTF-8" LC_NUMERIC=zh_CN.UTF-8 LC_TIME=zh_CN.UTF-8 LC_COLLATE="en_US.UTF-8" LC_MONETARY=zh_CN.UTF-8 LC_MESSAGES="en_US.UTF-8" LC_PAPER=zh_CN.UTF-8 LC_NAME=zh_CN.UTF-8 LC_ADDRESS=zh_CN.UTF-8 LC_TELEPHONE=zh_CN.UTF-8 LC_MEASUREMENT=zh_CN.UTF-8 LC_IDENTIFICATION=zh_CN.UTF-8 LC_ALL=
Step 2
$ export LC_ALL=C root@ubuntu:~# locale LANG=en_US.UTF-8 LANGUAGE= LC_CTYPE="C" LC_NUMERIC="C" LC_TIME="C" LC_COLLATE="C" LC_MONETARY="C" LC_MESSAGES="C" LC_PAPER="C" LC_NAME="C" LC_ADDRESS="C" LC_TELEPHONE="C" LC_MEASUREMENT="C" LC_IDENTIFICATION="C" LC_ALL=C
Step 4 Verifies TensorFlow Installation
$ python #Enter python import tensorflow as tf hello = tf.constant('Hello TensorFlow!') sess = tf.Session() print(sess.run(hello)) exit() #Sign out
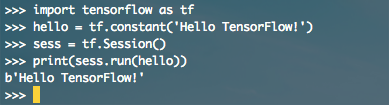
Hello TensorFlow!
Using Tensorboard Visualization Tool
1. Install graphical interface for Aliyun server
$ apt-get install x-window-system-core $ apt-get install gnome-core $ apt-get install gdm $ startx #Aliyun console remote connection can see the graphical interface
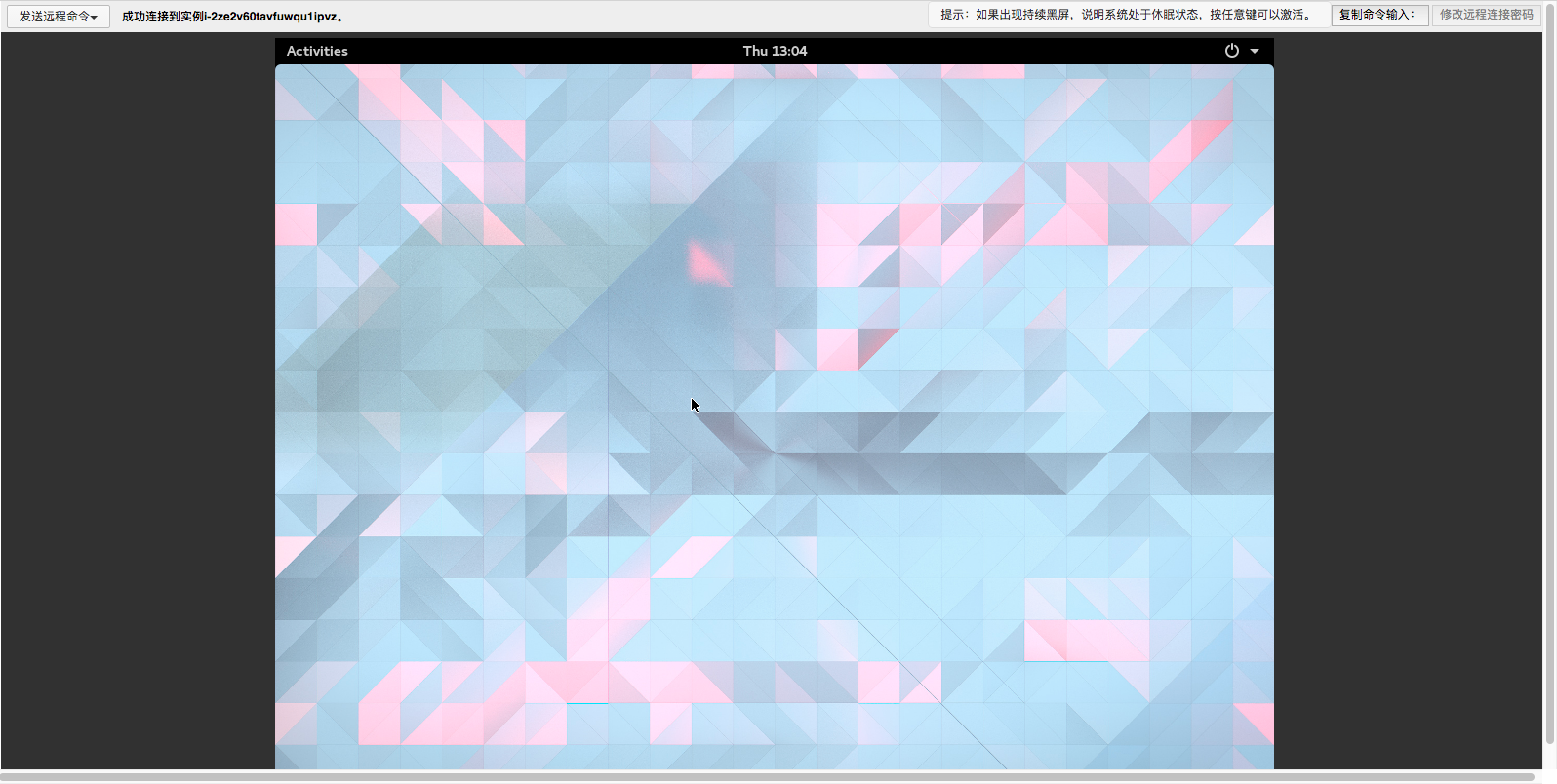
Graphical Interface
Install vim to edit documents
$ apt-get install vim
If E: Sub-process/usr/bin/dpkg returned an error code appears when apt-get is installed
Solution
sudo mv /var/lib/dpkg/info /var/lib/dpkg/info.bak //Now rename the info folder sudo mkdir /var/lib/dpkg/info //Create a new info folder sudo apt-get update
2. Create a new TensorFlow folder under the home directory and put it into the tensorboard.py file.
$ mkdir TensorFlow $ cd TensorFlow $ vim tensorboard.py
tensorboard.py file
""" Please note, this code is only for python 3+. If you are using python 2+, please modify the code accordingly. """ from __future__ import print_function import tensorflow as tf import numpy as np def add_layer(inputs, in_size, out_size, n_layer, activation_function=None): # add one more layer and return the output of this layer layer_name = 'layer%s' % n_layer with tf.name_scope(layer_name): with tf.name_scope('weights'): Weights = tf.Variable(tf.random_normal([in_size, out_size]), name='W') tf.summary.histogram(layer_name + '/weights', Weights) with tf.name_scope('biases'): biases = tf.Variable(tf.zeros([1, out_size]) + 0.1, name='b') tf.summary.histogram(layer_name + '/biases', biases) with tf.name_scope('Wx_plus_b'): Wx_plus_b = tf.add(tf.matmul(inputs, Weights), biases) if activation_function is None: outputs = Wx_plus_b else: outputs = activation_function(Wx_plus_b, ) tf.summary.histogram(layer_name + '/outputs', outputs) return outputs # Make up some real data x_data = np.linspace(-1, 1, 300)[:, np.newaxis] noise = np.random.normal(0, 0.05, x_data.shape) y_data = np.square(x_data) - 0.5 + noise # define placeholder for inputs to network with tf.name_scope('inputs'): xs = tf.placeholder(tf.float32, [None, 1], name='x_input') ys = tf.placeholder(tf.float32, [None, 1], name='y_input') # add hidden layer l1 = add_layer(xs, 1, 10, n_layer=1, activation_function=tf.nn.relu) # add output layer prediction = add_layer(l1, 10, 1, n_layer=2, activation_function=None) # the error between prediciton and real data with tf.name_scope('loss'): loss = tf.reduce_mean(tf.reduce_sum(tf.square(ys - prediction), reduction_indices=[1])) tf.summary.scalar('loss', loss) with tf.name_scope('train'): train_step = tf.train.GradientDescentOptimizer(0.1).minimize(loss) sess = tf.Session() merged = tf.summary.merge_all() writer = tf.summary.FileWriter("logs/", sess.graph) init = tf.global_variables_initializer() sess.run(init) for i in range(1000): sess.run(train_step, feed_dict={xs: x_data, ys: y_data}) if i % 50 == 0: result = sess.run(merged, feed_dict={xs: x_data, ys: y_data}) writer.add_summary(result, i) # direct to the local dir and run this in terminal: # $ tensorboard --logdir logs
Run command
$ python tensorboard.py #Generate a logs folder and enter the tensorboard command to view the tensorboard web site $ tensorboard --logdir logs
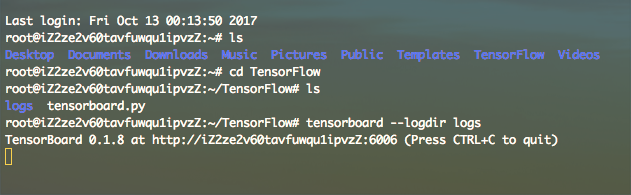
Tensorboard
Enter 127.0.0.1:6006 in the browser to visit Tensorboard Visual Page of tensorflow.py
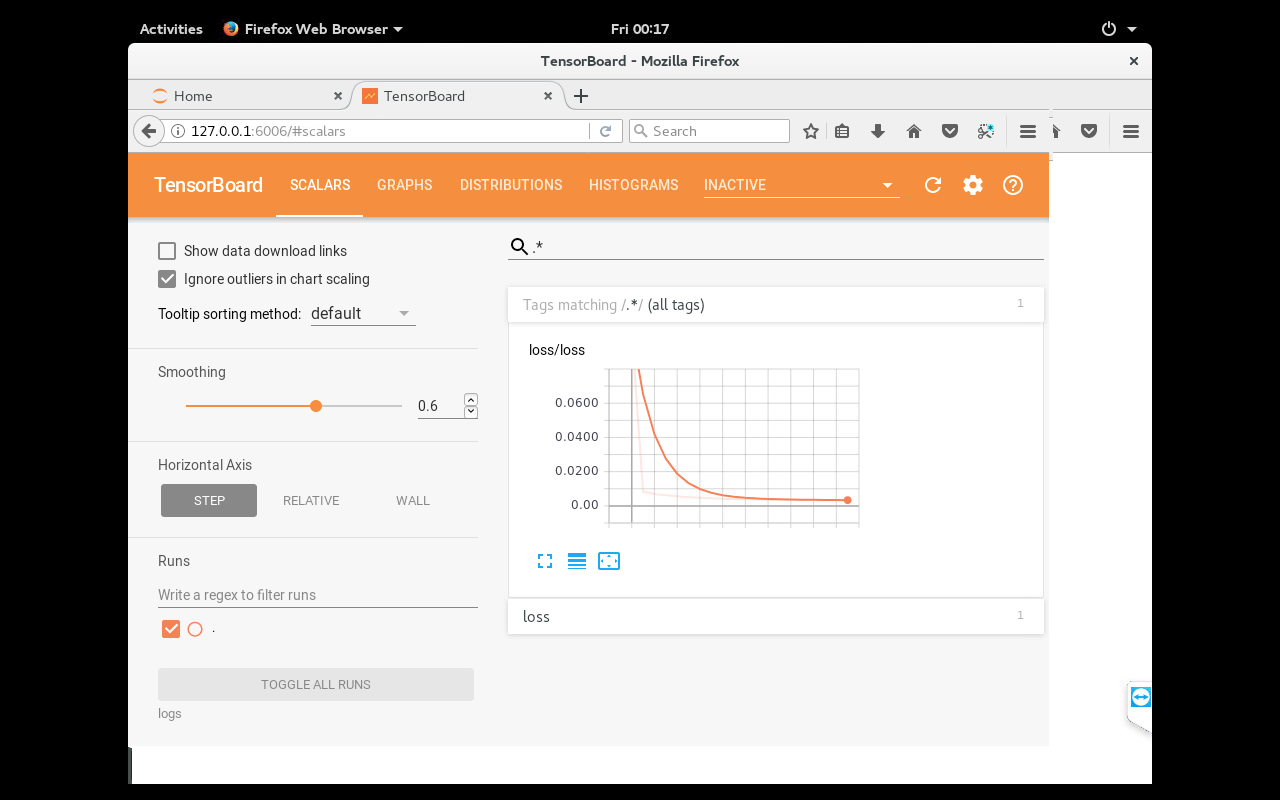
Tensorboard
Tensorboard error TensorBoard attempted to bind to port 6006, but it was already in use solution
$ lsof -i:6006 root@iZ2ze2v60tavfuwqu1ipvzZ:~/TensorFlow# lsof -i:6006 COMMAND PID USER FD TYPE DEVICE SIZE/OFF NODE NAME tensorboa 1635 root 3u IPv4 19343 0t0 TCP *:x11-6 (LISTEN)
Kill process
root@iZ2ze2v60tavfuwqu1ipvzZ:~/TensorFlow# kill -9 1635 [1]+ Killed tensorboard --logdir logs
Run again
$ tensorboard --logdir logs
jupyter notebook installation configuration
$ pip install jupyter
$ jupyter notebook –generate-config –allow-root $ ipython Python 3.5.2 (default, Aug 4 2017, 02:13:48) Type 'copyright', 'credits' or 'license' for more information IPython 6.1.0 -- An enhanced Interactive Python. Type '?' for help. In [1]: from notebook.auth import passwd In [2]: passwd() Enter password: Verify password: Out[2]: 'token' //token refers to a string of generated text that needs to be used later In [3]: exit()
Modify the jupyter notebook configuration file
$ vim ~/.jupyter/jupyter_notebook_config.py c.NotebookApp.ip='*' c.NotebookApp.password = u'token' c.NotebookApp.open_browser = False c.NotebookApp.port =8888 #Specify a port at will, or use the default 8888
Visit jupyter notebook
$ jupyter notebook --ip=0.0.0.0 --no-browser --allow-root
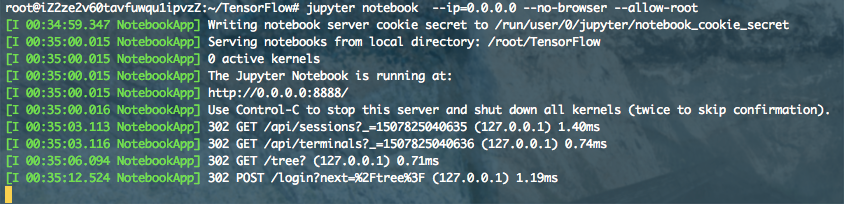
jupyter notebook
Browser input 0.0.0.0:8888 access
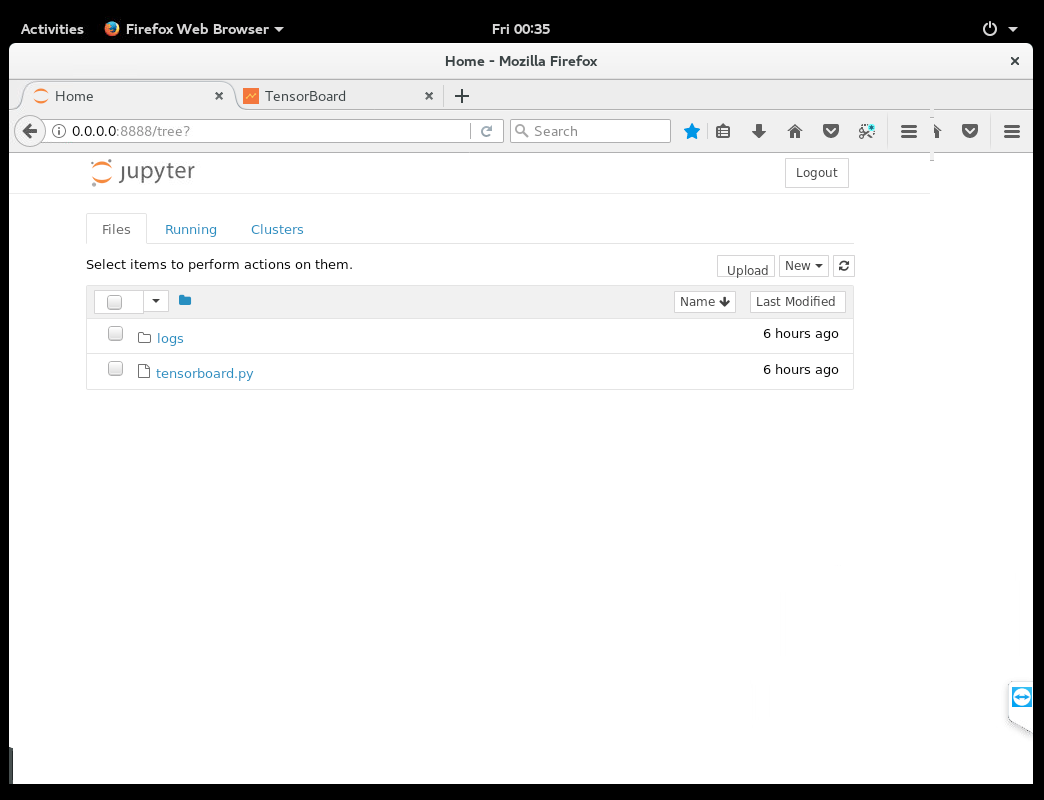
jupyter notebook