Vue+Django REST framework
Set up a website of fresh supermarket with front and back ends separated
Django rtf completes the commodity category page
vue Displays the List of Commodities Page Data
- Click on a navigation level class to display the data. Or click on the search to find the results
These two functions need to be completed together: the same pattern, left navigation, right commodity list.
Clicking on a navigation category will show how many items it has at the bottom of the secondary and tertiary categories.
Searches only show first-and second-level categories.
The merchandise list page has his pagination and sorting.
When we click on fresh food and search, we use the same left nav component. But it's different.
Click Search, which asks for all categories to be displayed in the secondary directory, and click Fresh Food. It only requests secondary and tertiary directories under the current category.
How to distinguish the world navigation bar from the search page in a component?
The difference between the two Vue routers can be found in the head.

You can see that either pop-up by clicking or navigation jumps to the vue router shown above.

Click on the hot search list to jump to search router
- Look at jump logic in vue router
src/router/index.js
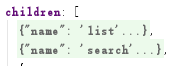
list is for commodity classification, search is for search
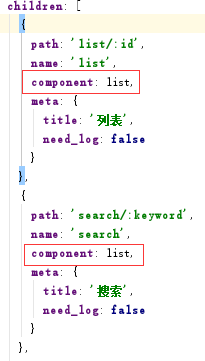
You can see that component points to the same component. It's just that the url is different. Of course, the parameters attached to the url are different.
One is id and the other is keyword
In src/views/list/list.vue
getalldata is called in the create method of page life cycle:
created () { this.getAllData (); },
In getAllData, we can get that this.$route.params share a component, but the parameters passed in the path are different.
getAllData () { console.log(this.$route.params) if(this.$route.params.id){ this.top_category = this.$route.params.id; this.getMenu(this.top_category); // Get the list of menus on the left }else{ this.getMenu(null); // Get the list of menus on the left this.pageType = 'search' this.searchWord = this.$route.params.keyword; } this.getCurLoc(); // Get the current location this.getListData(); //Get the product list this.getPriceRange(); // Get the price range },
If you pass in an id, it will be getMenu in the code above, passing in the ID of the current category as a parameter.
The second-level and third-level classification of a category is obtained.
getMenu(id) { if(id != null){ getCategory({ id:this.$route.params.id }).then((response)=> { this.cateMenu = response.data.sub_cat; this.currentCategoryName = response.data.name }).catch(function (error) { console.log(error); }); }else { getCategory({}).then((response)=> { this.cateMenu = response.data; this.isObject = false }).catch(function (error) { console.log(error); }); } },
If the id is not empty, that is, the second level and third level of classification query, then call getCategory to fill in the current cateMenu and current Category Name using response.data.
src/views/list/list-nav/listNav.vue:
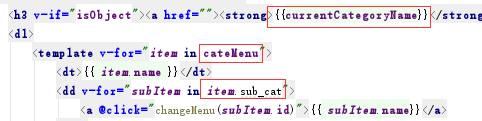
You can see the parameters passed in. The data we get in the list is to be passed to the list nav.
<list-nav :currentCategoryName="currentCategoryName" :cateMenu="cateMenu" :proNum="proNum" :isObject="isObject" @on-change="changeMenu"></list-nav>
Add variables and parameter names after colons. Pass the current in-page value to list_nav. Because you need to get not only the category, but also the total number of items.
The list on the left calls the getCategory function, which we have previously configured to localize data.
List page of goods
Getting a list of products will tell us that we're only search ing for a few days. Or get the goods getGoods
getListData() { if(this.pageType=='search'){ getGoods({ search: this.searchWord, //Search keywords }).then((response)=> { this.listData = response.data.results; this.proNum = response.data.count; }).catch(function (error) { console.log(error); }); }else { getGoods({ page: this.curPage, //CurrentPage top_category: this.top_category, //Commodity type ordering: this.ordering, //Sort type pricemin: this.pricemin, //The lowest price defaults to', i.e. no price range. pricemax: this.pricemax // The highest default price is'' }).then((response)=> { this.listData = response.data.results; this.proNum = response.data.count; }).catch(function (error) { console.log(error); }); } },
You don't need to know the current page number and sorting rules when searching. If page_type is not a search (page_type is set in getalldata based on request parameters), we can call getGoods.
getGoods is located in api.js
//Get a list of items export const getGoods = params => { return axios.get(`${local_host}/goods/`, { params: params }) }
getgoods in getListData can see the parameter names used in the front end.
We can go to view and change the paging parameters to page to be consistent with the front end.
page_query_param = "page"
The parameter top_category is our first level. It passes in the id value of our parameter.
So we also need to make a category filter in the background.
How to obtain all the goods under the first-level classification.
goods/filters.py: class GoodsFilter
top_category = filters.NumberFilter(name="category",method='top_category_filter') def top_category_filter(self, queryset, name, value): # Whether the current click is a first-level directory, a second-level directory or a third-level directory. return queryset.filter(Q(category_id=value)|Q(category__parent_category_id=value)|Q(category__parent_category__parent_category_id=value))
Then return the queryset back.
pricemin and pricemax are inconsistent with our front-end code.
So modify goods/filters.py to be consistent with the front end.
Paging data comes with a count value.
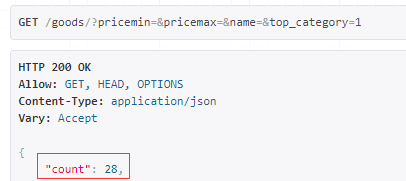
Turn up a little; we get response.data.count in getGoods in getListdata;
Then it's stored in proNum. ProNum is a variable in our current page data, passed to the list NAV page through list nav:
<list-sort @on-sort="changeSort" :proNum="proNum"></list-sort>
Listsort also passes the variable past.
- Setting paging size is consistent with the front end
class GoodsPagination(PageNumberPagination): page_size = 12
- The order field in the GoodsListViewSet is consistent with the front end
ordering_fields = ('sold_num', 'shop_price')
vue's commodity search function
search_fields = ('name', 'goods_brief', 'goods_desc')
There is no interface to configure, because search still calls the back-end goods interface.
Price range
<price-range :priceRange="priceRange" @on-change="changePrice"></price-range>
priceRange parameters are passed for price-range components
getPriceRange () { this.$http.post('/priceRange', { params: { proType: this.type, //Commodity type } }).then((response)=> { this.priceRange = response.data; }).catch(function (error) { console.log(error); }); },
getPriceRange is called in getAllData, or crate life cycle.
http.post is the request to the data interface in the mock.
Mock.mock('/priceRange',
It is recommended that these data be set in the background. Price intervals are calculated according to different commodity categories.