In our last article A Brief Introduction to Shader and the Use of Bitmap Shader Bitmap Shader Bitmap Render This paper introduces the use of Shader, the base class of renderer, and BitmapShader bitmap renderer in its five subclasses. Today we will continue to talk about Linear Gradient linear renderer, Radial Gradient ring renderer and Radial Gradient ring renderer. Sweep Gradient Gradient Gradient Render
Linear Gradient Linear Render
Linear Gradient is a renderer that creates a linear gradient along a straight line. Let's look at its constructor:
LinearGradient (float x0, // X-axis coordinates of the starting point of color gradient float y0, // Y-axis coordinates of the starting point of color gradient float x1, // Endpoint X-axis coordinates of color gradient float y1, // Endpoint Y-axis coordinates of color gradient int[] colors, // Gradient distribution of color array, that is, gradient from the beginning to the end of the color transition array, array length is unlimited, but must not be empty float[] positions, //The position of the color array corresponding to the gradient distribution is a floating-point value array in the range of [0-1]. The length of the array should be the same as that of colors. It can also be empty. If it is empty, it means that the color distribution is uniform. Shader.TileMode tile) // Rendering tiling mode
Example:
public class MyView extends View { public MyView(Context context) { this(context, null, 0); } public MyView(Context context, AttributeSet attrs) { this(context, attrs, 0); } public MyView(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); int width = getWidth(); int height = 500; int[] colors = { Color.RED, Color.GREEN, Color.BLUE, Color.YELLOW}; float[] positions = { 0f, 0.5f, 0.8f, 1f }; LinearGradient linearGradient = new LinearGradient(0, 0, width, 0, colors,positions, Shader.TileMode.CLAMP); Paint paint = new Paint(Paint.ANTI_ALIAS_FLAG); paint.setShader(linearGradient); canvas.drawRect(0, 0, width, height, paint); } }
In the code above, a Linear Gradient is defined. The first four parameters specify two coordinates of the color gradient. The fifth parameter is an array of colors, where red, green, blue and yellow, and the sixth parameter is the proportion of the color distribution.
Operation effect:
Radial Gradient Ring Render
Radial Gradient is a renderer that creates a radial gradient of the rendering center and radius. Let's look at its constructor:
RadialGradient (float centerX, // X-coordinates of the center of a circle float centerY, // Y-coordinates of the center of a circle float radius, // Radius of circle int[] colors, // Gradient distribution of color arrays (as described earlier in Linear Gradient colors) float[] stops, // The position of the color array corresponding to the gradient distribution (the same as the position parameter introduced earlier in LinearGradient) Shader.TileMode tileMode) // Rendering tiling mode
Example:
public class MyView extends View { public MyView(Context context) { this(context, null, 0); } public MyView(Context context, AttributeSetattrs) { this(context, attrs, 0); } public MyView(Context context, AttributeSetattrs, int defStyleAttr) { super(context, attrs, defStyleAttr); } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); int width = getWidth(); int height = getHeight(); int radius = 200; int[] colors = { Color.RED, Color.GREEN, Color.BLUE, Color.YELLOW}; float[] positions = { 0f, 0.9f, 0.95f, 1f }; Paint paint = new Paint(Paint.ANTI_ALIAS_FLAG); RadialGradient radialGradientA = new RadialGradient(width / 2, height / 4, radius,colors, positions, Shader.TileMode.CLAMP); paint.setShader(radialGradientA); canvas.drawCircle(width / 2, height / 4, radius,paint); RadialGradient radialGradientB = new RadialGradient(width / 2, height / 4 * 3 + 50, radius, colors, positions,Shader.TileMode.CLAMP); paint.setShader(radialGradientB); canvas.drawCircle(width / 2, height / 4 * 3, radius, paint); } }
In the code above, two Radial Gradients are defined, in which Radial Gradient A specifies the coordinates and radius of the center of the circle that are consistent with the circle drawn, while Radial Gradient B adds 50 pixels to the radius.
Operation effect:
SweepGradient Gradient Render
SweepGradient is a renderer that draws the scan gradient at the center point. Let's look at its constructor:
SweepGradient (float cx, // X-coordinates of the center of a circle float cy, // Y-coordinates of the center of a circle int[] colors, // Gradient distribution of color arrays (as described earlier in Linear Gradient colors) float[] positions) // The position of the color array corresponding to the gradient distribution (the same as the position parameter introduced earlier in LinearGradient)
Example
public class MyView extends View { public MyView(Context context) { this(context, null, 0); } public MyView(Context context, AttributeSet attrs) { this(context, attrs, 0); } public MyView(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); int width = getWidth(); int height = getHeight(); int radius = 200; int[] colors = { Color.RED, Color.GREEN, Color.BLUE, Color.YELLOW}; float[] positions = { 0f, 0.9f, 0.95f, 1f }; Paint paint = new Paint(Paint.ANTI_ALIAS_FLAG); SweepGradient sweepGradient = new SweepGradient(width / 2, height / 2, colors, positions); paint.setShader(sweepGradient); canvas.drawCircle(width / 2, height / 2, radius, paint); } }
In the above code, a SweepGradient is defined. The parameters are very simple. They are the center of the circle that keeps one to the drawing, the color array and the color distribution array.
Operation effect:
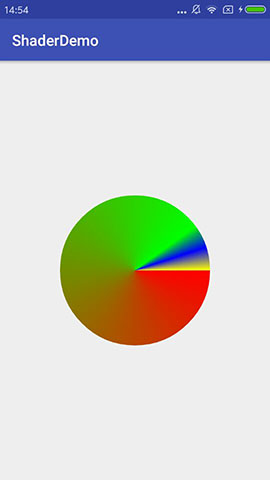