7-7 modelform Submitted to me for Learning Consultation 1
Corresponding table userask
form validates the fields first and then saves them to the database.
You can see that many of our forms and our model s are the same. How do we reuse code?
Use modelform to solve this problem.
# encoding: utf-8 from django import forms from operation.models import UserAsk __author__ = 'mtianyan' __date__ = '2018/1/12 0012 03:20' # Ordinary version of form # class UserAskForm(forms.Form): # name = forms.CharField(required=True, min_length=2, max_length=20) # phone = forms.CharField(required=True, max_length=11, min_length=11) # course_name = forms.CharField(required=True, min_length=5, max_length=50) # Advanced version of modelform: It can save like model class AnotherUserForm(forms.ModelForm): # In addition to inheritance, new fields can be added. # Which model is converted by class Meta: model = UserAsk # Fields I need to validate fields = ['name','mobile','course_name']
include's mechanism configuration applies its own url
Django 1.9.8 creates organization/urls.py:
# encoding: utf-8 __author__ = 'mtianyan' __date__ = '2018/1/12 0012 06:20' # encoding: utf-8 from organization.views import OrgView from django.conf.urls import url urlpatterns = [ # Curriculum organization list url url('list/&', OrgView.as_view(), name="org_list"),
django1.9.8 Mxonline2/urls.py:
Delete orglist and add the following
# url configuration of app in curriculum organization url(r"^org/", include('organization.urls',namespace="org")),
Django 2.0.1: New organization/urls.py
# encoding: utf-8 from organization.views import OrgView __author__ = 'mtianyan' __date__ = '2018/1/12 0012 03:28' from django.urls import path, re_path urlpatterns = [ # Curriculum organization list url path('list/', OrgView.as_view(), name="org_list"), ]
Django 2.0.1: urls.py:
Delete org_list and add include
# url configuration of app in curriculum organization path("org/", include('organization.urls',namespace="org")),
Use namespaces to prevent duplication

Resolve Django 2.0.1 error reporting:
'Specifying a namespace in include() without providing an app_name ' django.core.exceptions.ImproperlyConfigured: Specifying a namespace in include() without providing an app_name is not supported. Set the app_name attribute in the included module, or pass a 2-tuple containing the list of patterns and app_name instead.
Write appname in urls under your app
# encoding: utf-8 from organization.views import OrgView __author__ = 'mtianyan' __date__ = '2018/1/12 0012 03:28' from django.urls import path, re_path app_name = "organization" urlpatterns = [ # Curriculum organization list url path('list/', OrgView.as_view(), name="org_list"), ]
Namespace is used in html:

Submit using modelform
Reasonable operations are asynchronous and do not refresh the entire page.
If there are errors, display them. An asynchronous operation of ajax.
So we can't render a page directly at this time.
It should be to return json data to the front end, not pages
The HttpResponse class specifies what type of data is returned to the user
from django.http import HttpResponse
Configure a view of modelform
# User Addition I Want to Learn class AddUserAskView(View): # Processing form submission post of course def post(self,request): userask_form = UserAskForm(request.POST) # Determine whether the form is valid if userask_form.is_valid(): # Here's the difference between modelform and form # It has the properties of model # When commit really saves true user_ask = userask_form.save(commit=True) # This eliminates the need to take out fields one by one and save them after they are stored in the object of the model. # If saved successfully, the json string is returned, and the content type is later told to the browser. return HttpResponse("{'status': 'success'}", content_type='application/json') else: # If the save fails, return the json string and pass the form's error message through msg to the front end return HttpResponse("{'status': 'fail', 'msg':{0}}".format(userask_form.errors), content_type='application/json')
Configure the corresponding url
# Add I want to learn path('add_ask/', AddUserAskView.as_view(), name="add_ask")
7-8 modelform submits to me for study
Analysis of ajax requests
<script> $(function(){ $('#jsStayBtn').on('click', function(){ $.ajax({ cache: false, type: "POST", url:"{% url "org:add_ask" %}", data:$('#jsStayForm').serialize(), async: true, success: function(data) { if(data.status == 'success'){ $('#jsStayForm')[0].reset(); alert("Submit successfully") }else if(data.status == 'fail'){ $('#jsCompanyTips').html(data.msg) } }, }); }); }) </script>
Listen for this button if the user clicks it. Let's make a post request to this url.
Serialize our form.
Form form adds crsf_token
If the status value returned in the background is success, then we will pop up the submission successfully.
If it fails, it will be written in the error prompt box.
Mobile phone number regular expression validation:
organization/forms.py
# Verification of Regular Expressions for Mobile Phone Numbers def clean_mobile(self): mobile = self.cleaned_data['mobile'] REGEX_MOBILE = "^1[358]\d{9}$|^147\d{8}$|^176\d{8}$" p = re.compile(REGEX_MOBILE) if p.match(mobile): return mobile else: raise forms.ValidationError(u"Illegal mobile phone number", code="mobile_invalid")
At the end of this section, commit:
7-7-8 Use modelform to complete the form asynchronous submission I want to learn
7-9 Institutional Details
- Home page of organization
- Institutional Curriculum
- Introduction of mechanism
- Institutional lecturer
Log in xadmin to add the basic necessary data. Adding courses and lecturers.
There should be a foreign key pointing to which institution it is in the course.
courses/models.py
In Django 1.9.8:
from organization.models import CourseOrg course_org = models.ForeignKey(CourseOrg, verbose_name=u"Affiliate",null=True,blank=True)
Django 2.0.1;
course_org = models.ForeignKey(CourseOrg,on_delete=models.CASCADE, verbose_name=u"Affiliate",null=True,blank=True)
New foreign key fields should be null=true,blank=true.
Because there is no foreign key field in the historical data
makemigration course migrate course
Copy the four pages related to our org from the front end into the template
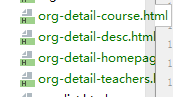
New org_base page
Take over the content of the org_home page.

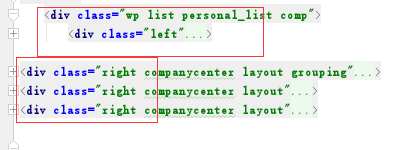
- Load static files - > change css file path - > change js file path - > change picture path
- Change the url that has been implemented. - > After inheriting the sub-page, you need to change the place to use the block package.
Clear the home page
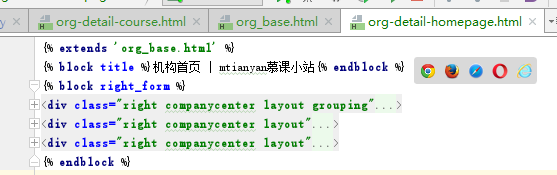
Add access view and URl after replacement
organization/views.py:
class OrgHomeView(View): """ //Home page of organization """ def get(self, request, org_id): # Get to the course organization according to id course_org = CourseOrg.objects.get(id= int(org_id)) # Find the course through the course organization. Built-in variables to find foreign key references to this field all_courses = course_org.course_set.all()[:4] all_teacher = course_org.teacher_set.all()[:2] return render(request, 'org-detail-homepage.html',{ 'all_courses':all_courses, 'all_teacher':all_teacher, })
url under Django 2.0.1
# home page, take pure numbers re_path('home/(?P<org_id>\d+)/', OrgHomeView.as_view(), name="org_home"),
Under Django 1.9.8 url:
# home page, take pure numbers url(r'home/(?P<org_id>\d+)/$', OrgHomeView.as_view(), name="org_home")
Use for loop traversal in html:
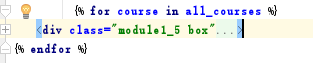

As shown in the figure above, you can find the field of the foreign key object directly through the foreign key field.
templates/org-list.html
The url that jumps to the details page in the configuration

return render(request, 'org-detail-homepage.html',{ 'all_courses':all_courses, 'all_teacher':all_teacher, 'course_org': course_org, })
By return ing course_org back, you can replace this part of the value in the web page.
templates/org_base.html
The values are passed up the inheritance chain.

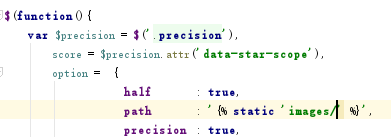
Adding Head Image Fields for Lecturers
organization/models.py
image = models.ImageField( default= '', upload_to="teacher/%Y/%m", verbose_name=u"Head portrait", max_length=100)
Then
makemgration organization migrate organization
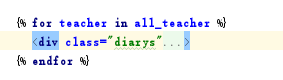
Fill in data with a for loop
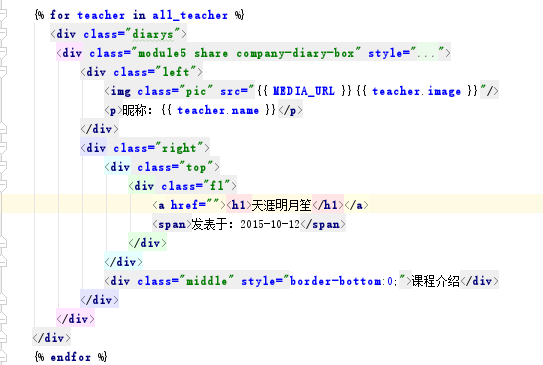
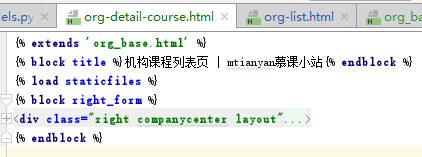
Similarly, we can take out the different parts of the institutional curriculum page.
Configure the view and url for access
organization/urls.py:
Django 2.0.1:
# Visiting courses re_path('course/(?P<org_id>\d+)/', OrgCourseView.as_view(), name="org_course"),
Django 1.9.8:
# Visiting courses url(r'^course/(?P<org_id>\d+)/$', OrgCourseView.as_view(), name="org_course"),
organization/views.py
class OrgCourseView(View): """ //Institutional Course List Page """ def get(self, request, org_id): # Get to the course organization according to id course_org = CourseOrg.objects.get(id= int(org_id)) # Find the course through the course organization. Built-in variables to find foreign key references to this field all_courses = course_org.course_set.all() return render(request, 'org-detail-course.html',{ 'all_courses':all_courses, 'course_org': course_org, })
left Link Modification of base Page
You can use course_org.id directly here. Because the course_org object is passed up when the sub-page render

Fill in the content using the for loop.
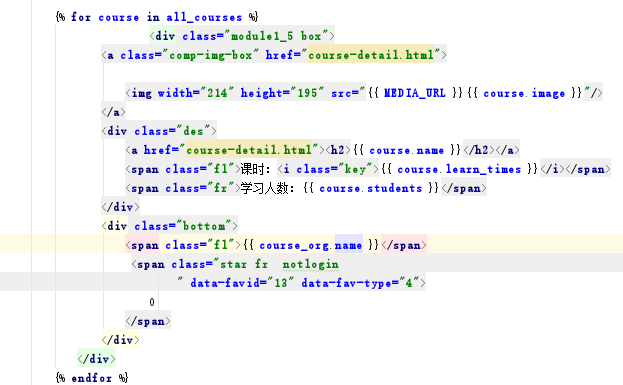
Left active modification
Because there is no value to determine which page is currently. So pass the value back to the current page in orghomeview
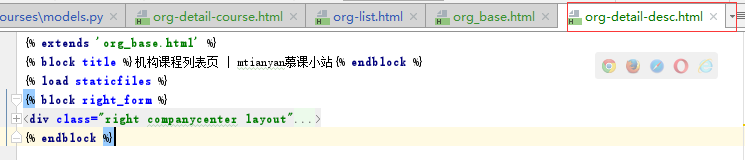
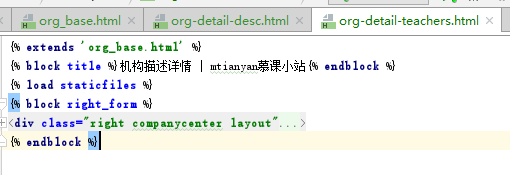
Write two view s
class OrgDescView(View): """ //Institutional Description Details Page """ def get(self, request, org_id): # Pass a value to the front end to indicate that it is now on the home page current_page = "desc" # Get to the course organization according to id course_org = CourseOrg.objects.get(id= int(org_id)) # Find the course through the course organization. Built-in variables to find foreign key references to this field # Passing a value to the front indicates whether the user has a collection or not has_fav = False # It must be that the user has logged in before we need to judge. if request.user.is_authenticated: if UserFavorite.objects.filter(user=request.user, fav_id=course_org.id, fav_type=2): has_fav = True return render(request, 'org-detail-desc.html',{ 'course_org': course_org, "current_page":current_page, "has_fav":has_fav, }) class OrgTeacherView(View): """ //Institutional Lecturer List Page """ def get(self, request, org_id): # Pass a value to the front end to indicate that it is now on the home page current_page = "teacher" # Get to the course organization according to id course_org = CourseOrg.objects.get(id= int(org_id)) # Find the course through the course organization. Built-in variables to find foreign key references to this field all_teachers = course_org.teacher_set.all() # Passing a value to the front indicates whether the user has a collection or not has_fav = False # It must be that the user has logged in before we need to judge. if request.user.is_authenticated: if UserFavorite.objects.filter(user=request.user, fav_id=course_org.id, fav_type=2): has_fav = True return render(request, 'org-detail-teachers.html',{ 'all_teachers':all_teachers, 'course_org': course_org, "current_page":current_page, "has_fav":has_fav })
Add two url
Under Django 2.0.1:
# Visitor description re_path('desc/(?P<org_id>\d+)/', OrgDescView.as_view(), name="org_desc"), # Visiting Institution Lecturer re_path('teacher/(?P<org_id>\d+)/', OrgTeacherView.as_view(), name="org_teacher"),
Under Django 1.9.8:
# Visitor description url(r'^desc/(?P<org_id>\d+)/$', OrgDescView.as_view(), name="org_desc"), # Visiting Institution Lecturer url(r'^teacher/(?P<org_id>\d+)/$', OrgTeacherView.as_view(), name="org_teacher"),
Modify the jump link on the base page. Note: Add course_org.id
Overload our pagepath to make breadcrumbs dynamically display


7-10 Course Institution Collection Function
Write the background logic of the collection:
url configuration
Under Django 2.0.1:
# Institutional collection path('add_fav/', AddFavView.as_view(), name="add_fav"),
Django 1.9.8;
# Institutional collection url(r'^add_fav/$', AddFavView.as_view(), name="add_fav"),
Supporting view;
class AddFavView(View): """ //User Collection and Cancellation of Collection Function """ def post(self, request): # Show that you have a collection of courses, lecturers, and institutions. Their id # The default value of 0 is due to an empty tandem int error. id = request.POST.get('fav_id', 0) # Get to your collection category, from the ajax request submitted by the front desk type = request.POST.get('fav_type', 0) # Collection and Cancellation of Collection # Determine whether a user is logged in: Even if it is not logged in, there will be an anonymous user if not request.user.is_authenticated: # When you are not logged in, you return the json prompt that you are not logged in and jump to the login page is done in ajax return HttpResponse('{"status":"fail", "msg":"User not logged in"}', content_type='application/json') exist_records = UserFavorite.objects.filter(user=request.user, fav_id=int(id), fav_type=int(type)) if exist_records: # If the record already exists, the user cancels the collection exist_records.delete() return HttpResponse('{"status":"success", "msg":"Collection"}', content_type='application/json') else: user_fav = UserFavorite() # Filter out defaults that are not fetched to fav_id type if int(type) >0 and int(id) >0: user_fav.fav_id = int(id) user_fav.fav_type = int(type) user_fav.user = request.user user_fav.save() return HttpResponse('{"status":"success", "msg":"Have been collected"}', content_type='application/json') else: return HttpResponse('{"status":"fail", "msg":"Collection error"}', content_type='application/json')
The jQuery code for dealing with collections is written at org base Html

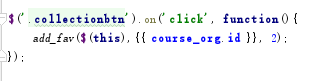
Add the collection value of the return page.
# Passing a value to the front indicates whether the user has a collection or not has_fav = False # It must be that the user has logged in before we need to judge. if request.user.is_authenticated: if UserFavorite.objects.filter(user=request.user, fav_id=course_org.id, fav_type=2): has_fav = True # return redener plus value "has_fav": has_fav
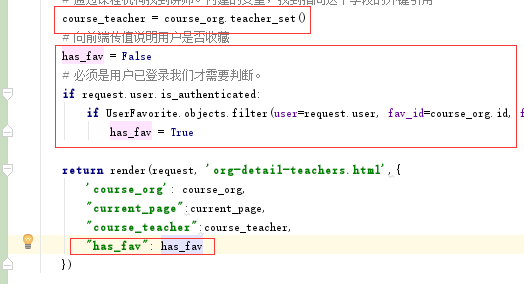
In the Front Desk org_base.html
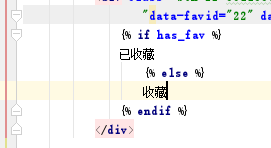
Similarly, add the remaining pages.
Chapter VII Ends Spraying Flowers. Corresponding to commit:
7-9-10-11-12 shows the details of the institution and adds a collection function to the curriculum institution. Fixed the bug of index exploding when it was not logged in