Django 2.0.2 (Django-rest-framework) and the front-end and back-end separated mall website developed by front-end vue
Online demo address: http://vueshop.mtianyan.cn/
github source code address: https://github.com/mtianyan/VueDjangoFrameWorkShop
This section: Realization of Rotary Graph Interface and vue Debugging
Realization of Rotary Graph Interface and vue Debugging
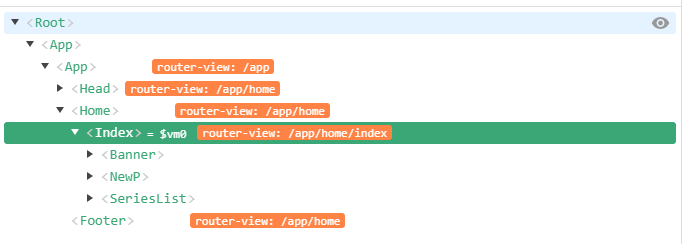
vue home page component structure.
index is the content area of the home page, banner is the rotation map, NewP is the display part of new products, SeriesList is a series of products.
- Rotary Graph Interface-New Product Interface-The following Series of Product Interfaces
The payment function has been developed. We need to enter the local debugging environment.
Run to see if the local debugging environment works. Change the address in the vue project to our local address.
banner has a foreign key pointing to our goods.
Build a new matching Banner Serializer:
goods/serializers.py:
class BannerSerializer(serializers.ModelSerializer): class Meta: model = Banner fields = "__all__"
goods/views.py:
class BannerViewset(mixins.ListModelMixin, viewsets.GenericViewSet): """ Get a list of rotation maps """ queryset = Banner.objects.all().order_by("index") serializer_class = BannerSerializer
Setting up our url
# Home page banner rotation map url router.register(r'banners', BannerViewset, base_name="banners")
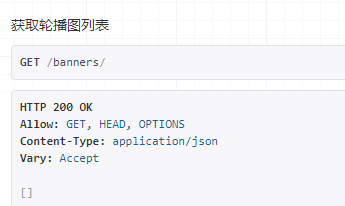
There are no errors but no data. We come directly to our background management system to add three rotation maps.
The interface for obtaining the rotation graph is
//Getting the Rotary Planting Map export const bannerGoods = params => { return axios.get(`${host}/banners/`) }
Change to localhost
src/views/index/index.vue:
Three components are referenced in the index component on the home page
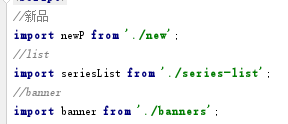
<div> <banner></banner> <newP></newP> <seriesList></seriesList> </div>
The banner component calls getBanner when it creates
created(){ this.getBanner(); }
getBanner calls bannerGoods
getBanner(){ bannerGoods() .then((response)=> { console.log(response) //Jump to the response.body surface on the home page this.banners = response.data }) .catch(function (error) { console.log(error); }); }
Fill in the response data into banners

Data rendering by swipe component
import { swiper, swiperSlide } from 'vue-awesome-swiper'
Development of New Functional Interface
The new product is an is_new option in goods.
Direct use of Goods interface, a filter, filter out which is new products.
goods/filters.py:
fields = ['pricemin', 'pricemax', 'name', 'is_hot','is_new']
Add is_new field to goods filter

Using filters, you can see is_new =ture in url
At this point, the correct status code of 200 is returned, but the value is empty. Because we didn't set it is_new.
Two new products are added in the xadmin background.
Then go to vue to see how the component is completed.
src/views/index/new.vue
created(){ this.getOpro(); }
getOpro calls the method getGoods
getOpro(){ getGoods({ "is_new":"true" }) .then((response)=> { //Jump to the response.body surface on the home page this.newopro = response.data.results }) .catch(function (error) { console.log(error); }); }
Calling interface
//Get a list of items export const getGoods = params => { return axios.get(`${local_host}/goods/`, { params: params }) }
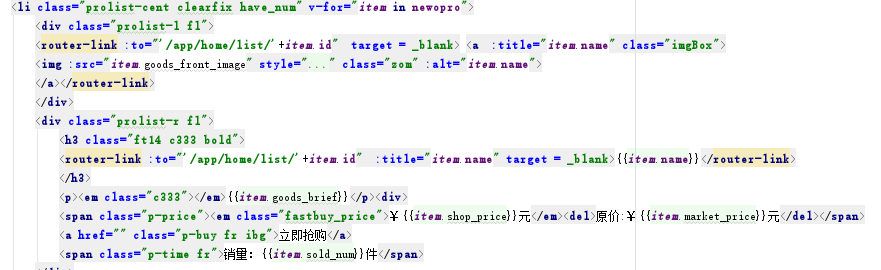
The response data is used to traverse and fill in the commodity information value.
Home page commodity classification display function-1
Development of large classified commodities.
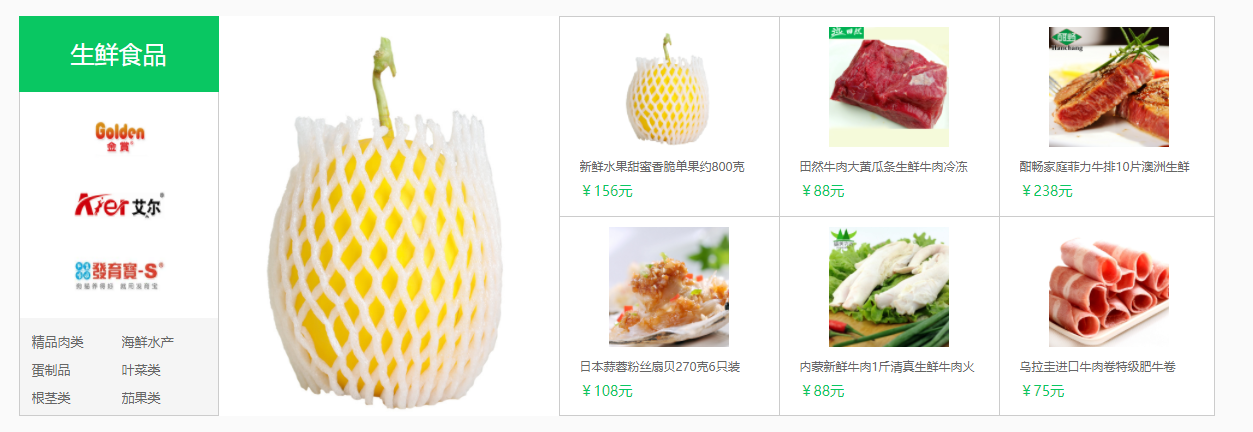
The relationship between element data in this div is more complex.
- There are many brand trademarks in a large category.
- Big class and small class, still one-to-many
- Commodities in a large category.
- Plus it has a big picture.
Let's start by writing a Serilizer for this series of merchandise displays.
class IndexCategorySerializer(serializers.ModelSerializer): class Meta: model = GoodsCategory fields = "__all__"
Find out one-to-many relationships.
- First, we need to get brand. Goods Category Brand has a foreign key pointing to category, and we add a related_name to it. It's more convenient to make it retrieve.
category = models.ForeignKey(GoodsCategory, on_delete=models.CASCADE, related_name='brands', null=True, blank=True, verbose_name="Commodity category")
We can retrieve brand by goods_category.
First write a BrandSerializer
class BrandSerializer(serializers.ModelSerializer): class Meta: model = GoodsCategoryBrand fields = "__all__"
Put brand in Index Category Serializer
# A category has multiple brand s. brands = BrandSerializer(many=True)
Why can't you use Google's Serilizer directly?
There is indeed a foreign key in goods that points to our category. Why can't we take it backwards?
Because when choosing which category of goods, the smallest category is often chosen. (third kinds)
It is not directly subordinate to the first category.
- Foreign keys point to the third category. And we're going to get the first kind of data. The Serilizer that uses goods directly does not come out.
goods = serializers.SerializerMethodField() def get_goods(self, obj): # Match this item with parent, child, etc. all_goods = Goods.objects.filter(Q(category_id=obj.id)|Q(category__parent_category_id=obj.id)|Q(category__parent_category__parent_category_id=obj.id)) goods_serializer = GoodsSerializer(all_goods, many=True, context={'request': self.context['request']}) return goods_serializer.data
Categorization of secondary commodities
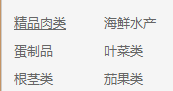
sub_cat = CategorySerializer2(many=True)
First of all, this is a commodity, which belongs to the first class.
Design IndexAd model to store advertising space under large categories.
class IndexAd(models.Model): """ //Seven Advertisements on the Right of Home Category Label """ category = models.ForeignKey(GoodsCategory, on_delete=models.CASCADE, related_name='category',verbose_name="Commodity category") goods =models.ForeignKey(Goods, on_delete=models.CASCADE, related_name='goods') class Meta: verbose_name = 'Homepage ads' verbose_name_plural = verbose_name def __str__(self): return self.goods.name
ad_goods = serializers.SerializerMethodField() def get_ad_goods(self, obj): goods_json = {} ad_goods = IndexAd.objects.filter(category_id=obj.id, ) if ad_goods: good_ins = ad_goods[0].goods goods_json = GoodsSerializer(good_ins, many=False, context={'request': self.context['request']}).data return goods_json
In fact, ad_goods only needs its id and a picture. It doesn't need to use the one that returns all the commodity information.
Goods Serializer, take the fields in it and return them.
Or write a Serializer
Write the corresponding viewset
class IndexCategoryViewset(mixins.ListModelMixin, viewsets.GenericViewSet): """ Home page commodity classification data """ # Get the data that we have set for the top navigation Queryset = GoodsCategory. objects. filter (is_tab = True, name_u in=["fresh food", "drinks"]) serializer_class = IndexCategorySerializer
Drinks, drinks and fresh foods are available here because brand and ad_goods can be added later.
Configure the corresponding url for the interface
# Home Page Series merchandise display url router.register(r'indexgoods', IndexCategoryViewset, base_name="indexgoods")
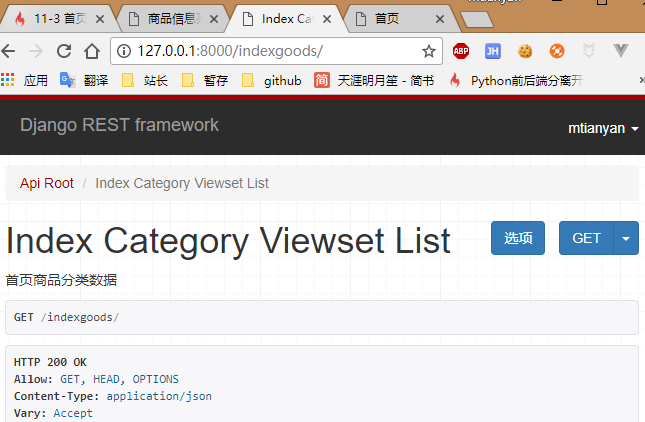
You can see that we successfully accessed the commodity classification data on the home page.