(7)spring expression
Note: Read the properties of bean s
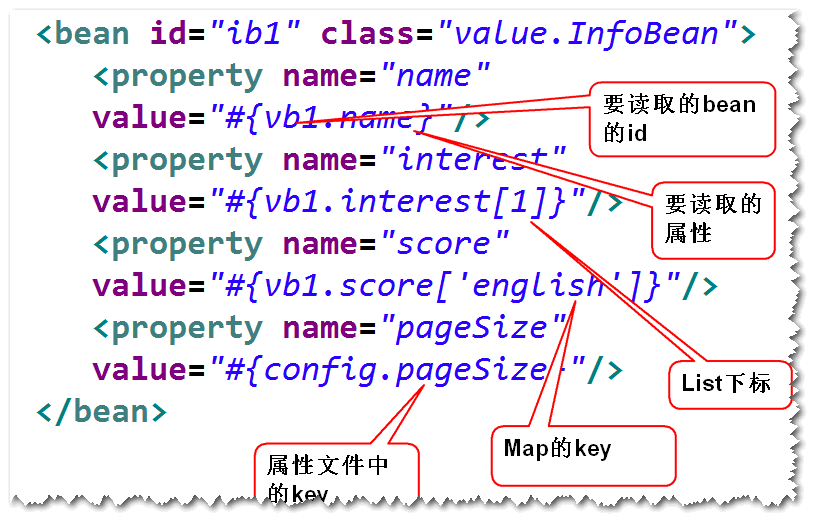
1. Use annotations to simplify configuration files
(1) What is component scanning?
The container scans the specified package and all of its subpackages
Class, if there are specific annotations in front of it, such as @Component,
Then the container will incorporate it into the container management (equivalent to in the configuration file).
There is a bean element.
(2) How to scan components?
Step 1. Add specific annotations before the class, such as @Component
Note: The default id is the class name after lowercase.
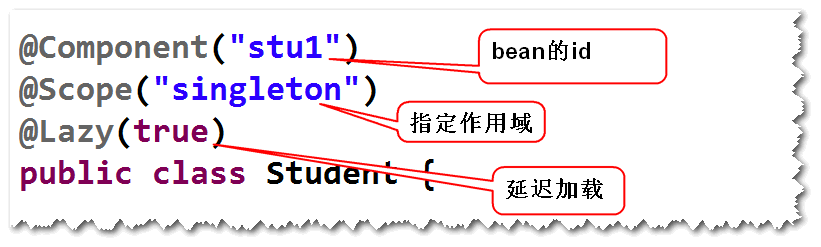
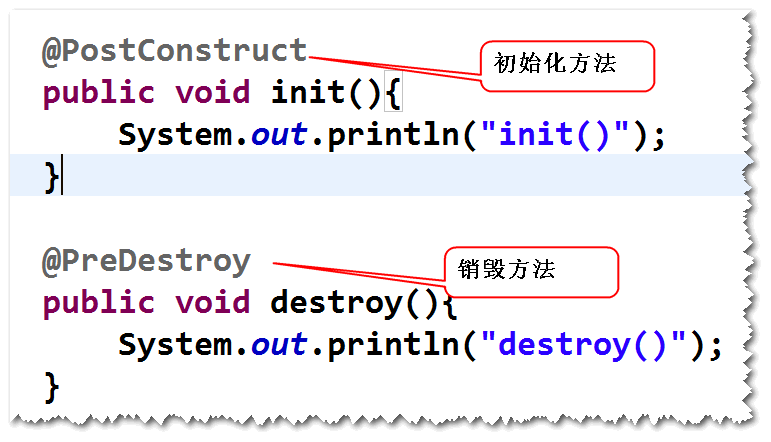
Step 2. In the configuration file, the configuration component scans.
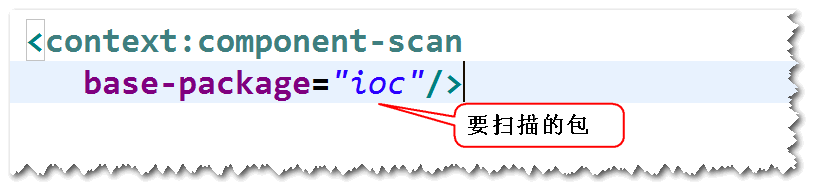
(3) Dependency Injection-related annotations
1)@Autowired @Qualifier
Note: This annotation supports set method and constructor injection.
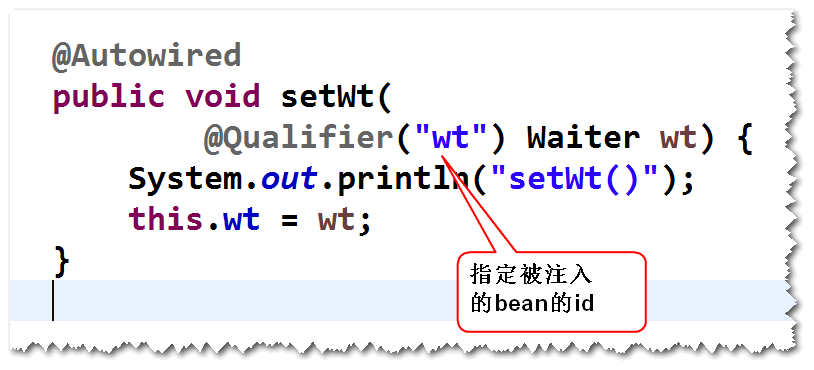
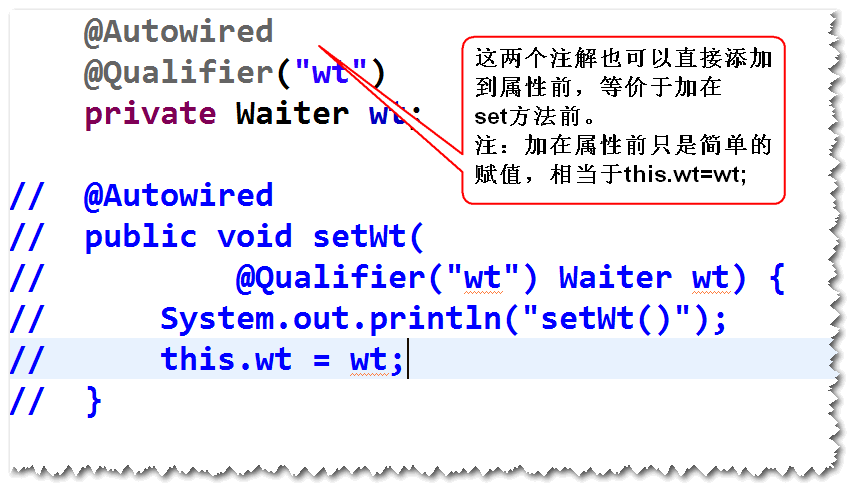
2)@Resource
Note: This annotation only supports set method injection.
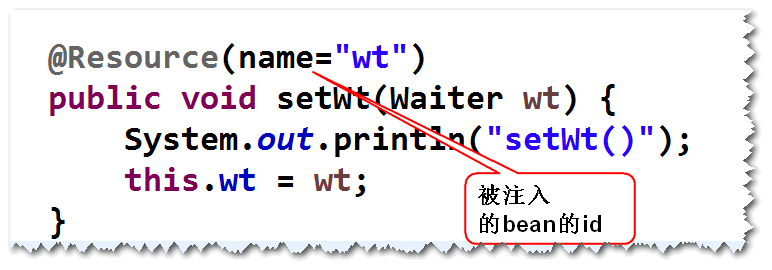
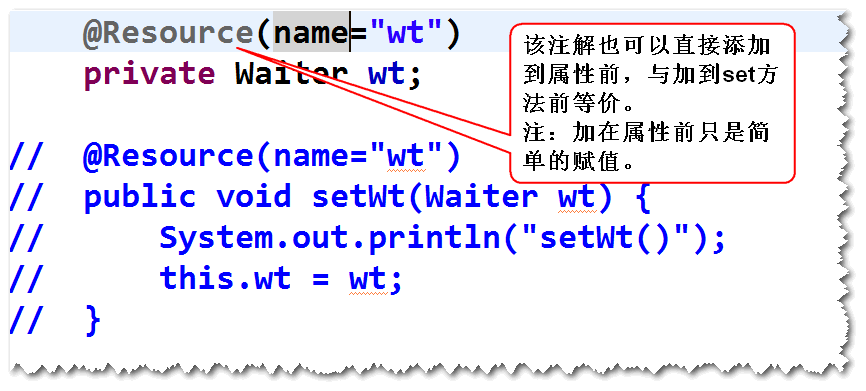
(4) Injecting values of basic types and values of spring expressions
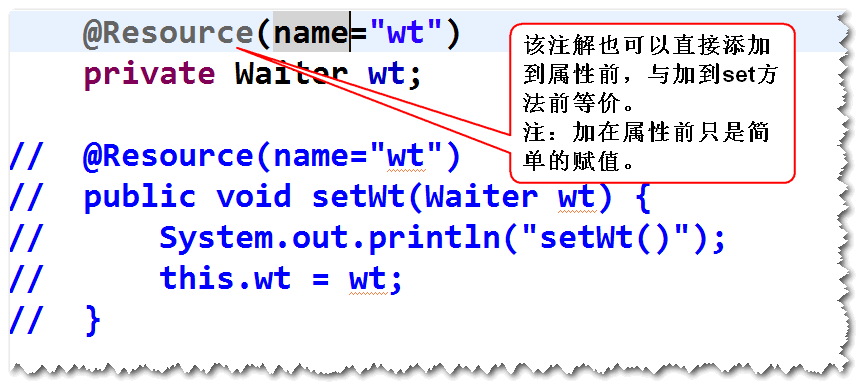
SpringMVC
(1) What is Spring MVC?
It is used to simplify the framework of web application development based on MVC architecture.
Note: Spring MVC is a module in spring.
(2) Five components
1) What are the five components?
Dispatcher Servlet Front-end Controller
Handler Mapping Mapping Processor
Controller processor
ModelAndView
ViewResolver View Parser
2) The relationship between them
a. Requests are sent to Dispatcher Servlet for processing, Dispatcher Servlet
The corresponding Controller is invoked based on the configuration of the Handler Mapping.
b.Controller encapsulates the processing results as ModelAndView and returns them to
DispatcherServlet.
C. Dispatcher Servlet calls the corresponding one based on ViewResolver's parsing
View objects (such as jsp) are used to generate corresponding pages.
Note: The view section can use jsp or other view technologies, such as
freemarker,velocity, etc.
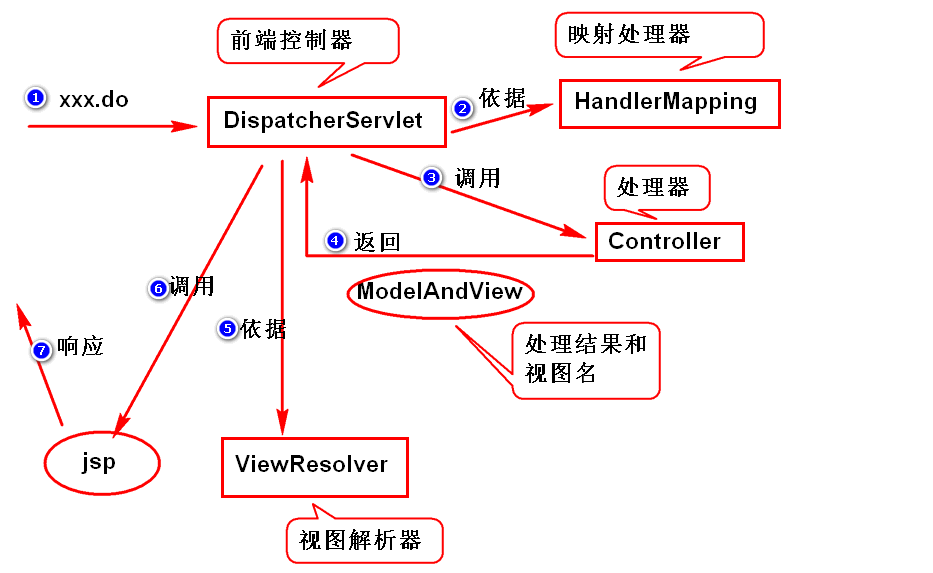
(3) Programming steps
step1. Guide bag
spring-webmvc
step2. Add configuration files.
step3. To configure DispatcherServlet.
step4. write Controller.
step5. write jsp.
step6.In the configuration file, add HandlerMapping,
ViewResolver Configuration.
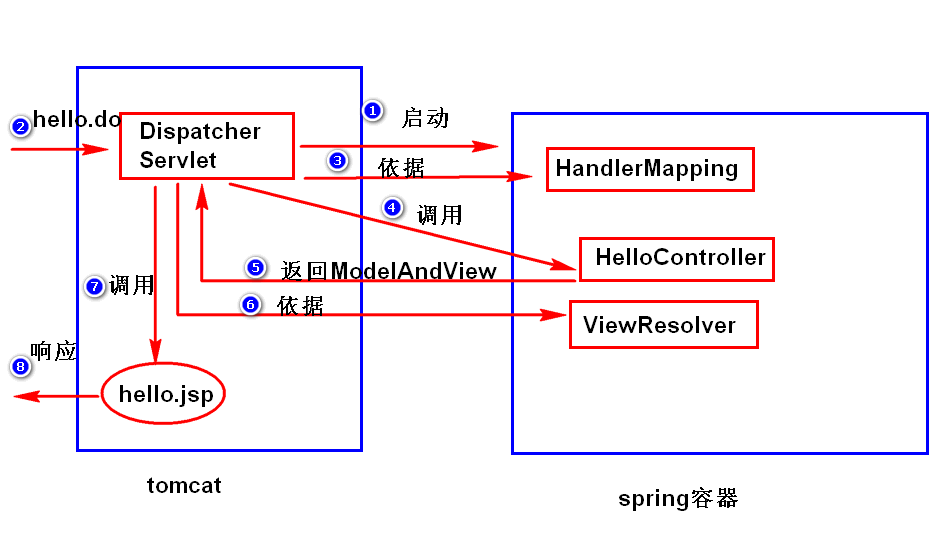
Code demo:
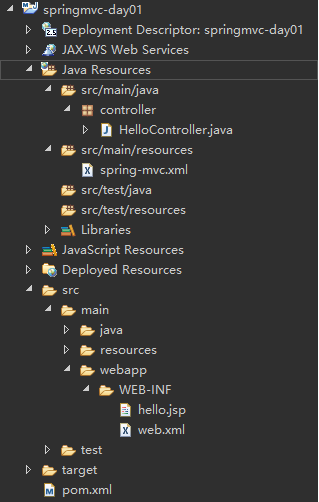
/springmvc-day01/src/main/java/controller/HelloController.java
package controller;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.Controller;
/**
* processor
*
*/
public class HelloController implements Controller {
public ModelAndView handleRequest(HttpServletRequest arg0, HttpServletResponse arg1) throws Exception {
System.out.println("handleRequest()");
return new ModelAndView("hello");
}
}
/springmvc-day01/src/main/resources/spring-mvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:jdbc="http://www.springframework.org/schema/jdbc" xmlns:jee="http://www.springframework.org/schema/jee"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:util="http://www.springframework.org/schema/util"
xmlns:jpa="http://www.springframework.org/schema/data/jpa"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-3.2.xsd
http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-3.2.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd
http://www.springframework.org/schema/data/jpa http://www.springframework.org/schema/data/jpa/spring-jpa-1.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.2.xsd">
<bean class="org.springframework.web.servlet.handler.SimpleUrlHandlerMapping">
<property name="mappings">
<props>
<prop key="/hello.do">helloController</prop>
</props>
</property>
</bean>
<bean id="helloController" class="controller.HelloController" />
<bean
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/" />
<property name="suffix" value=".jsp" />
</bean>
</beans>
/springmvc-day01/src/main/webapp/WEB-INF/hello.jsp
<h1>Hello Kitty</h1>
/springmvc-day01/src/main/webapp/WEB-INF/web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5">
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>
org.springframework.web.servlet.DispatcherServlet
</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring-mvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
/springmvc-day01/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>welkin</groupId>
<artifactId>springmvc-day01</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>3.2.8.RELEASE</version>
</dependency>
</dependencies>
</project>