Now use Spring 4 to integrate Struts 2 and Hibernate 4.
Struts 2.3.16, Spring 4.0.6, Hibernate 4.3.5 integrate the required jar packages:
The jar package required for Struts 2.3.6:
Spring 4.0.6 requires jar packages:
The jar package required for Hibernate 4.3.5:
The MySQL database is installed locally, and MySQL driver package is added: mysql-connector-java-3.1.12-bin.jar
1. New dynamic web project project project: S2SH
2. Paste the required jar packages into the lib directory:
3. Configure the web.xml file as follows:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5"> <display-name>S2SH</display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <!-- Add pair spring Support --> <context-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:applicationContext.xml</param-value> </context-param> <!-- Definition Spring Monitor, load Spring --> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <!-- Add pair struts2 Support --> <filter> <filter-name>struts2</filter-name> <filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!-- Session Delayed loading to pages --> <filter> <filter-name>openSessionInViewFilter</filter-name> <filter-class>org.springframework.orm.hibernate4.support.OpenSessionInViewFilter</filter-class> <init-param> <param-name>singleSession</param-name> <param-value>true</param-value> </init-param> </filter> <filter-mapping> <filter-name>openSessionInViewFilter</filter-name> <url-pattern>*.action</url-pattern> </filter-mapping> </web-app>
In particular, there is a session delayed loading to the page configuration. If this configuration is not added, the session is closed when the associated data is retrieved in the background, and the page can not retrieve the cascaded data. When openSessionInViewFilter is added, session can be delayed loading to the page. The following <url-pattern>*. action </url-pattern> indicates that all *. action requests are lazily loaded, that is, all struts2 requests are lazily loaded.
4. Configure struts 2 and create a new struts.xml file under the src folder:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.3//EN" "http://struts.apache.org/dtds/struts-2.3.dtd"> <struts> <constant name="struts.action.extension" value="action" /> </struts>
This represents the use of the request suffix for struts.
New hibernate.cfg.xml file configuration hibernate 4 under src:
<?xml version='1.0' encoding='UTF-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!--dialect--> <property name="dialect">org.hibernate.dialect.MySQL5Dialect</property> <!-- display sql Sentence --> <property name="show_sql">true</property> <!-- Auto update --> <property name="hbm2ddl.auto">update</property> </session-factory> </hibernate-configuration>
These configurations were mentioned here when we talked about hibernate.
6. You can see that the configuration files of struts 2 and Hibernate 4 above save a lot of things compared with talking about these two technologies alone. This is because what is omitted is to configure in the spring configuration file. Spring takes over some of the things of struts 2 and hibernate 4. Create a new file applicationContext.xml under the src directory to configure spring:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context" xmlns:jee="http://www.springframework.org/schema/jee" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation=" http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-4.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd"> <!-- Define data sources --> <bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name="driverClassName" value="com.mysql.jdbc.Driver"> </property> <property name="url" value="jdbc:mysql://localhost:3306/test"> </property> <property name="username" value="root"></property> <property name="password" value="123456"></property> </bean> <!-- session factory --> <bean id="sessionFactory" class="org.springframework.orm.hibernate4.LocalSessionFactoryBean"> <property name="dataSource"> <ref bean="dataSource" /> </property> <property name="configLocation" value="classpath:hibernate.cfg.xml"/> <!-- Automatic Scanning Annotation Configuration hibernate Class files --> <property name="packagesToScan"> <list> <value>com.test.entity</value> </list> </property> </bean> <!-- Configuring Transaction Manager --> <bean id="transactionManager" class="org.springframework.orm.hibernate4.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory" /> </bean> <!-- Configure transaction notification properties --> <tx:advice id="txAdvice" transaction-manager="transactionManager"> <!-- Define transaction propagation properties --> <tx:attributes> <tx:method name="insert*" propagation="REQUIRED" /> <tx:method name="update*" propagation="REQUIRED" /> <tx:method name="edit*" propagation="REQUIRED" /> <tx:method name="save*" propagation="REQUIRED" /> <tx:method name="add*" propagation="REQUIRED" /> <tx:method name="new*" propagation="REQUIRED" /> <tx:method name="set*" propagation="REQUIRED" /> <tx:method name="remove*" propagation="REQUIRED" /> <tx:method name="delete*" propagation="REQUIRED" /> <tx:method name="change*" propagation="REQUIRED" /> <tx:method name="get*" propagation="REQUIRED" read-only="true" /> <tx:method name="find*" propagation="REQUIRED" read-only="true" /> <tx:method name="load*" propagation="REQUIRED" read-only="true" /> <tx:method name="*" propagation="REQUIRED" read-only="true" /> </tx:attributes> </tx:advice> <!-- Configuring Transaction Aspects --> <aop:config> <aop:pointcut id="serviceOperation" expression="execution(* com.test.service..*.*(..))" /> <aop:advisor advice-ref="txAdvice" pointcut-ref="serviceOperation" /> </aop:config> <!-- Automatic Loading Construction bean --> <context:component-scan base-package="com.test" /> </beans>
Here are a few points to emphasize:
1) The hibernate class file is configured by automatically scanning annotations. If we use the packagesToScan attribute, all the classes under the com.test.entity package will be saved one by one.
2) Auto-loading build beans: All the classes are loaded by annotations later, so configure the auto-loading build beans and scan the classes under package com.test.
6. Build a package layer, there are more transaction layers than the traditional one. Build a package layer as follows:
7. Create a new index.jps page to test the configuration effect:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> S2SH Hello! </body> </html>
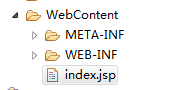
8. Add the project to the tomcat server, run the program, and find that there is no error in the program. Open the browser:
The program runs successfully, which indicates that Spring integrates struts 2 and Hibernate 4 successfully.