1: Basic knowledge
Note that the MessageBox is asynchronous. Unlike a regular JavaScript alert (which
will halt browser execution), showing a MessageBox will not cause the code to stop. For this reason, if you have code that should only run after some user feedback from the MessageBox, you must use a callback function (see the functionparameter
for show for
more details).
Note that MessageBox is asynchronous, unlike general alert, general alert will stop browser execution, but MessageBox will not stop the code running. For this reason, if some code needs feedback after user operation is completed, then use callback function (callback function).
2: Ext.MessageBox.alert()
alert.jsp
-
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
-
<%
-
String path = request.getContextPath();
-
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
-
%>
-
-
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
-
<html>
-
<head>
-
<base href="<%=basePath%>">
-
-
<title>My JSP 'messagebox.jsp' starting page</title>
-
-
<meta http-equiv="pragma" content="no-cache">
-
<meta http-equiv="cache-control" content="no-cache">
-
<meta http-equiv="expires" content="0">
-
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
-
<meta http-equiv="description" content="This is my page">
-
-
<link rel="stylesheet" type="text/css" href="ext3.2/resources/css/ext-all.css"></link>
-
<script type="text/javascript" src="ext3.2/adapter/ext/ext-base.js"></script>
-
<script type="text/javascript" src="ext3.2/ext-all.js"></script>
-
<script type="text/javascript" src="ext3.2/src/local/ext-lang-zh_CN.js"></script>
-
-
<script type="text/javascript">
-
Ext.onReady(function(){
-
Ext.get('test').on('click', function() {
-
Ext.MessageBox.alert("System hint", "Do you want to click on me, please choose! ", function(but) {
-
Ext.MessageBox.alert("Selection prompt", "You have chosen." + but);
-
});
-
});
-
});
-
</script>
-
</head>
-
-
<body>
-
<div id="test">Click on me to test Ext.MessageBox</div>
-
</body>
-
</html>
In the above code, MessageBox.alert (title, content, callback function) provides three parameters. The title is used to modify the title information of the prompt box. The content is used to display the information content of the prompt to the user. The callback function is used to accept the user's choice when the prompt box disappears. If the user clicks the "OK" button, the OK selected by the user is returned. If the user clicks the close button, cancel information is returned.
Demonstration effect:
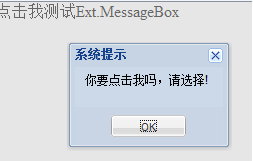
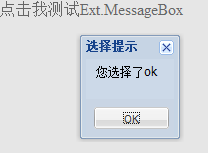
3: Ext.MessageBox.confirm() function
-
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
-
<%
-
String path = request.getContextPath();
-
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
-
%>
-
-
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
-
<html>
-
<head>
-
<base href="<%=basePath%>">
-
-
<title>My JSP 'messagebox.jsp' starting page</title>
-
-
<meta http-equiv="pragma" content="no-cache">
-
<meta http-equiv="cache-control" content="no-cache">
-
<meta http-equiv="expires" content="0">
-
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
-
<meta http-equiv="description" content="This is my page">
-
-
<link rel="stylesheet" type="text/css" href="ext3.2/resources/css/ext-all.css"></link>
-
<script type="text/javascript" src="ext3.2/adapter/ext/ext-base.js"></script>
-
<script type="text/javascript" src="ext3.2/ext-all.js"></script>
-
<script type="text/javascript" src="ext3.2/src/local/ext-lang-zh_CN.js"></script>
-
-
<script type="text/javascript">
-
Ext.onReady(function(){
-
Ext.get('test').on('click', function() {
-
Ext.MessageBox.confirm("System hint", "Do you want to hit me, please choose", function(but) {
-
Ext.MessageBox.alert("Selection prompt", "You have chosen." + but);
-
});
-
});
-
});
-
</script>
-
</head>
-
-
<body>
-
<div id="test">Click on me to test Ext.MessageBox.confirm</div>
-
</body>
-
</html>
Procedural effect:
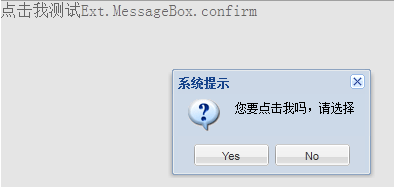
Picture: The effect of clicking on the test div
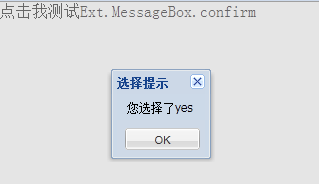
Picture: Click Yes to see the effect.
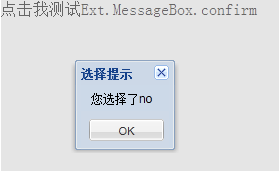
Picture: The effect of clicking No
4: Ext.MessageBox.prompt
-
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
-
<%
-
String path = request.getContextPath();
-
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
-
%>
-
-
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
-
<html>
-
<head>
-
<base href="<%=basePath%>">
-
-
<title>My JSP 'messagebox.jsp' starting page</title>
-
-
<meta http-equiv="pragma" content="no-cache">
-
<meta http-equiv="cache-control" content="no-cache">
-
<meta http-equiv="expires" content="0">
-
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
-
<meta http-equiv="description" content="This is my page">
-
-
<link rel="stylesheet" type="text/css" href="ext3.2/resources/css/ext-all.css"></link>
-
<script type="text/javascript" src="ext3.2/adapter/ext/ext-base.js"></script>
-
<script type="text/javascript" src="ext3.2/ext-all.js"></script>
-
<script type="text/javascript" src="ext3.2/src/local/ext-lang-zh_CN.js"></script>
-
-
<script type="text/javascript">
-
Ext.onReady(function(){
-
Ext.get('test').on('click', function() {
-
Ext.MessageBox.prompt("System hint", "Please enter your QQ Number and password! Hey",
-
function(but, text){
-
alert("You have chosen." + but + ":Your QQ Information is" + text);
-
});
-
});
-
});
-
</script>
-
</head>
-
-
<body>
-
<div id="test">Click on me to test Ext.MessageBox.prompt</div>
-
</body>
-
</html>
Procedural effect maps:
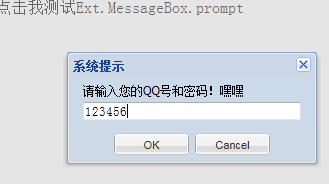
Figure: Procedural effects
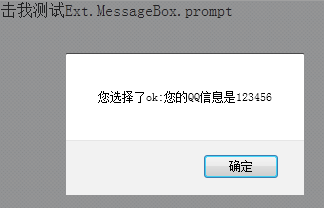
Figure: Procedural effects
5:prompt displays multiple lines
-
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
-
<%
-
String path = request.getContextPath();
-
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
-
%>
-
-
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
-
<html>
-
<head>
-
<base href="<%=basePath%>">
-
-
<title>My JSP 'messagebox.jsp' starting page</title>
-
-
<meta http-equiv="pragma" content="no-cache">
-
<meta http-equiv="cache-control" content="no-cache">
-
<meta http-equiv="expires" content="0">
-
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
-
<meta http-equiv="description" content="This is my page">
-
-
<link rel="stylesheet" type="text/css" href="ext3.2/resources/css/ext-all.css"></link>
-
<script type="text/javascript" src="ext3.2/adapter/ext/ext-base.js"></script>
-
<script type="text/javascript" src="ext3.2/ext-all.js"></script>
-
<script type="text/javascript" src="ext3.2/src/local/ext-lang-zh_CN.js"></script>
-
-
<script type="text/javascript">
-
Ext.onReady(function(){
-
Ext.get('test').on('click', function() {
-
Ext.MessageBox.prompt('Tips', 'Enter some content to see: ', callBack, this, true);
-
function callBack(id, msg) {
-
alert('The button you clicked id yes' + id + '\n' + 'The input is: ' + msg);
-
}
-
});
-
});
-
</script>
-
</head>
-
-
<body>
-
<div id="test">Click on me to test Ext.MessageBox.prompt</div>
-
</body>
-
</html>
Procedural effect:
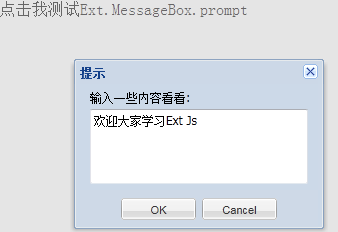
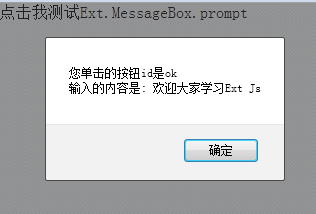
6:Ext.MessageBox.wait
-
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
-
<%
-
String path = request.getContextPath();
-
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
-
%>
-
-
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
-
<html>
-
<head>
-
<base href="<%=basePath%>">
-
-
<title>My JSP 'messagebox.jsp' starting page</title>
-
-
<meta http-equiv="pragma" content="no-cache">
-
<meta http-equiv="cache-control" content="no-cache">
-
<meta http-equiv="expires" content="0">
-
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
-
<meta http-equiv="description" content="This is my page">
-
-
<link rel="stylesheet" type="text/css" href="ext3.2/resources/css/ext-all.css"></link>
-
<script type="text/javascript" src="ext3.2/adapter/ext/ext-base.js"></script>
-
<script type="text/javascript" src="ext3.2/ext-all.js"></script>
-
<script type="text/javascript" src="ext3.2/src/local/ext-lang-zh_CN.js"></script>
-
-
<script type="text/javascript">
-
Ext.onReady(function(){
-
Ext.get('test').on('click', function(){
-
Ext.MessageBox.wait('Added to deleted data, please wait a moment.....', "Add to delete"); //A waiting bar appears.
-
});
-
});
-
</script>
-
</head>
-
-
<body>
-
<div id="test">Click on me to test Ext.MessageBox.prompt</div>
-
</body>
-
</html>
Procedural effect:
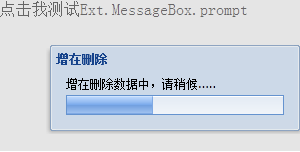
7: Ext.MessageBox.show
Its function is very powerful. It uses config configuration and has many parameters. Some commonly used parameters are listed.
animal: Animation effects when dialog boxes pop up and close, for example, when set to "id1", they pop up from Id1 and produce animation, while shrinking is the opposite.
buttons: The settings of pop-up dialog boxes are as follows: Ext.Msg.OK, Ext.Msg.OKCANCEL, Ext.Msg.CANCAL, Ext.Msg.YESNO, Ext.Msg.YESNOCANCEL,
You can also customize the text on the button: {"ok", "I was originally ok"}. If set to false, no buttons are displayed
* closable: If false, the fork in the upper right corner is not displayed, and the default value is true
* msg: Text content
* title: title
* fn: Functions executed after closing the pop-up box
* con: The icon in front of the pop-up box content takes the values Ext.MessageBox.INFO, Ext.MessageBox.ERROR, Ext.MessageBox.WARNING, Ext.Message.QUESTION
* width: The width of the pop-up box, without units
* prompt: Set to true, then pop-up box with input box
* progress: Set to true to display the progress bar
* progressText: Displays the text on the progress bar
* wait: true, dynamic display progress
* waitConfig: Configure parameters to display control progress
The procedure is as follows:
-
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
-
<%
-
String path = request.getContextPath();
-
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
-
%>
-
-
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
-
<html>
-
<head>
-
<base href="<%=basePath%>">
-
-
<title>My JSP 'messagebox.jsp' starting page</title>
-
-
<meta http-equiv="pragma" content="no-cache">
-
<meta http-equiv="cache-control" content="no-cache">
-
<meta http-equiv="expires" content="0">
-
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
-
<meta http-equiv="description" content="This is my page">
-
-
<link rel="stylesheet" type="text/css" href="ext3.2/resources/css/ext-all.css"></link>
-
<script type="text/javascript" src="ext3.2/adapter/ext/ext-base.js"></script>
-
<script type="text/javascript" src="ext3.2/ext-all.js"></script>
-
<script type="text/javascript" src="ext3.2/src/local/ext-lang-zh_CN.js"></script>
-
-
<script type="text/javascript">
-
Ext.onReady(function(){
-
Ext.get('test').on('click', function() {
-
Ext.MessageBox.show({
-
title: 'Title',
-
buttons: Ext.Msg.YESNO,
-
width: 200,
-
height: 400,
-
closable: false,
-
msg: 'Content displayed',
-
fn: function(e, text) {
-
Ext.MessageBox.alert('What you click on is' + e + "The input is: " + text);
-
},
-
icon: Ext.MessageBox.INFO,
-
prompt: true,
-
multiline: true
-
});
-
});
-
});
-
</script>
-
</head>
-
-
<body>
-
<div id="test">Click on me to test Ext.MessageBox.show</div>
-
</body>
-
</html>
Procedural effect:
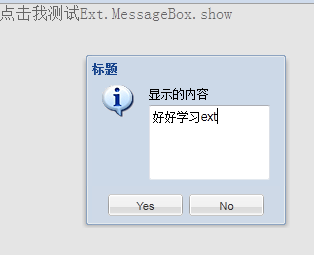
Figure: Procedure Diagram
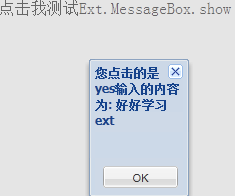
Figure: The prompt box displayed after clicking Yes