4-5 user modesl.py design
Circular reference:
When designing apps, each app has a model
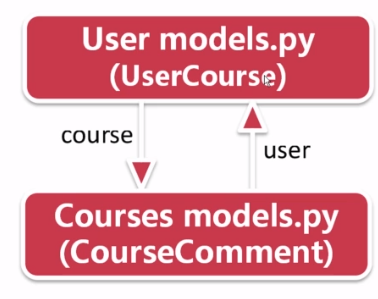
Figure: We define usercourse in user to record user learning courses. There will be two foreign keys: user and course.
We'll import Courses.models.
If users comment on the course, it will be placed in Courses.models. Comments need to be saved to the appropriate users.
We'll import User.models
Loop import will fail. a and b call each other, causing waiting.
Solving circular references: hierarchical design
app: users courses organization is available
Another app is higher than the hierarchy of these apps. operation. The upper app can import the lower app.
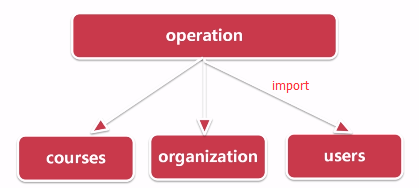
In the previous section: Customize userprofile to override the default user table
Additional features are required in user (provided they are relatively independent):
- Email VerifyRecord - Mail Verification Code
- PageBanner - Rotary Graph
Watch the rotation chart:
- Picture 2. Click on the picture address 3. Rotary map number (before and after control)
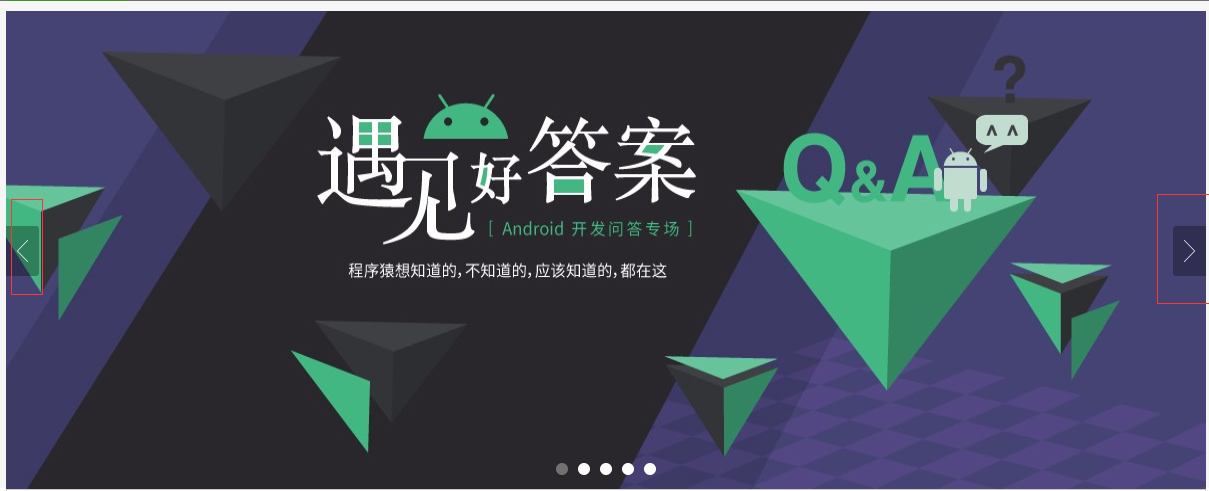
Add code to users/models.py:
from datetime import datetime # Mailbox Verification Code model class EmailVerifyRecord(models.Model): SEND_CHOICES = ( ("register", u"register"), ("forget", u"Retrieve the password") ) code = models.CharField(max_length=20, verbose_name=u"Verification Code") # null = true blank = true is not set. The default is not empty. email = models.EmailField(max_length=50, verbose_name=u"mailbox") send_type = models.CharField(choices=SEND_CHOICES, max_length=10) # Here now has to be removed (), not removed will be based on compilation time. Instead of instantiating time. send_time = models.DateTimeField(default=datetime.now) class Meta: verbose_name = "Mailbox Verification Code" verbose_name_plural = verbose_name # Rotary Planting model class Banner(models.Model): title = models.CharField(max_length=100, verbose_name=u"Title") image = models.ImageField( upload_to="banner/%Y/%m", verbose_name=u"Rotary Planting Map", max_length=100) url = models.URLField(max_length=200, verbose_name=u"Access Address") # The default index is far behind. You want to change the index value ahead. index = models.IntegerField(default=100, verbose_name=u"order") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"Rotary Planting Map" verbose_name_plural = verbose_name
From top to bottom: the first area import official package and the second import third party. (PEP8)
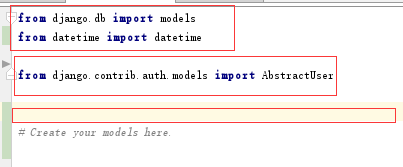
Here's how we created three tables: Structure Viewable
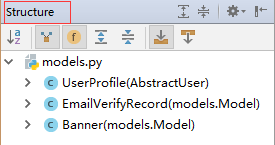
Comments related to users, please. The courses of study are not included because they are not independent.
It is easy to generate circular references. Let's put those in operation.
This section is completed and corresponds to commit:
Usermodel adds mailbox validation code and home page rotation chart. Correspondence 4-5
4-6 course models.py
Click Run management.py Task under the Tools menu
startapp courses
Which tables are needed in course r:
- The course itself needs a table.
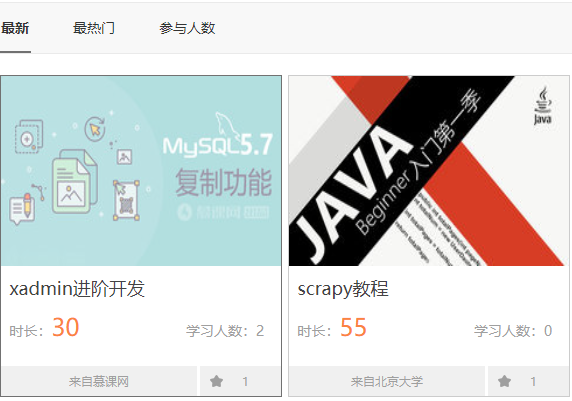
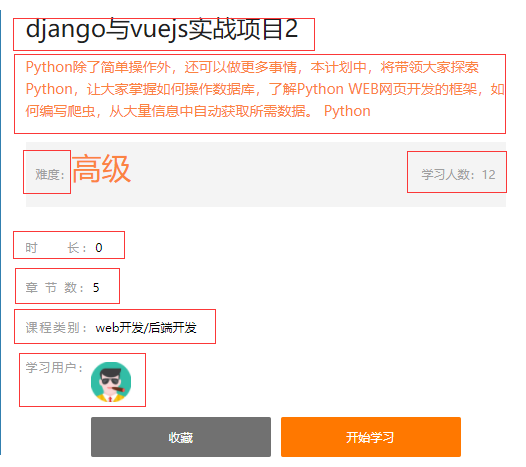
Click in and start learning.
The basic information of the course needs a table, and there are chapters and schedules (one course corresponds to multiple chapters).
Chapter list: Chapter name. Chapters and videos (one chapter corresponds to multiple videos)
Structure: Course itself - (one-to-many) > Chapter - (one-to-many) - > Video information
Resources are downloaded in the course. A course corresponds to multiple resources
There are four tables: course itself - (one-to-many) > chapters - (one-to-many) - > video information - resource table
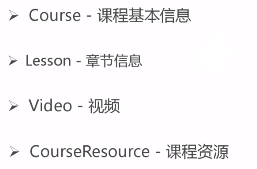
courses/models.py:
from datetime import datetime # Course information sheet class Course(models.Model): DEGREE_CHOICES = ( ("cj", u"primary"), ("zj", u"intermediate"), ("gj", u"senior") ) name = models.CharField(max_length=50, verbose_name=u"Course Name") desc = models.CharField(max_length=300, verbose_name=u"Course Description") # TextField allows us not to enter length. It can be input to infinity. Temporarily defined as TextFiled, then updated to Rich Text detail = models.TextField(verbose_name=u"Course Details") degree = models.CharField(choices=DEGREE_CHOICES, max_length=2) # Use Minutes for Background Recording (Minimum Storage Unit) Front-end Conversion learn_times = models.IntegerField(default=0, verbose_name=u"Learning time(Minutes)") # Save the number of learners: Start learning by clicking students = models.IntegerField(default=0, verbose_name=u"Number of students") fav_nums = models.IntegerField(default=0, verbose_name=u"Number of Collectors") image = models.ImageField( upload_to="courses/%Y/%m", verbose_name=u"Cover Chart", max_length=100) # Save the clicks and click on the page. click_nums = models.IntegerField(default=0, verbose_name=u"Number of clicks") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"curriculum" verbose_name_plural = verbose_name
Let's write a chapter - Video & Course Resources: courses/models.py
One-to-many, many-to-one can be accomplished using the foreign key of django.
# chapter class Lesson(models.Model): # Because a course corresponds to many chapters. Therefore, the curriculum is set as a foreign key in the chapter table. # As a field, let's know that this chapter corresponds to that course. course = models.ForeignKey(Course, verbose_name=u"curriculum") name = models.CharField(max_length=100, verbose_name=u"Chapter title") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"chapter" verbose_name_plural = verbose_name # Each Chapter Video class Video(models.Model): # Because a chapter corresponds to many videos. So set the chapters as foreign keys in the video table. # Storing it as a field lets us know which chapter the video corresponds to. lesson = models.ForeignKey(Lesson, verbose_name=u"chapter") name = models.CharField(max_length=100, verbose_name=u"Video Name") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"video" verbose_name_plural = verbose_name # Course resources class CourseResource(models.Model): # Because a course corresponds to many resources. Therefore, the curriculum is set as a foreign key in the curriculum resource table. # As a field, let's know which resource corresponds to which course. course = models.ForeignKey(Course, verbose_name=u"curriculum") name = models.CharField(max_length=100, verbose_name=u"Name") # This field is defined as a file type, and there will be a button to upload directly in the background management system. # FileField is also a string type, specifying the maximum length. download = models.FileField( upload_to="course/resource/%Y/%m", verbose_name=u"resource file", max_length=100) add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"Course resources" verbose_name_plural = verbose_name
From Structure, you can see the four tables we just designed.
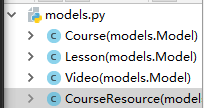
At the end of this section, it corresponds to commit:
Design and complete four data tables in the course app: courses, chapters, videos, resources. Correspondence 4-6
4-7 Organizationmodesl.py Design
app:
Click Run management.py Task under the Tools menu
startapp organization
Courses belong to institutions, institutions have institutional categories, cities and other fields. Lecturer entity.
The submission form I want to learn will be associated with the user and stored in the organization.
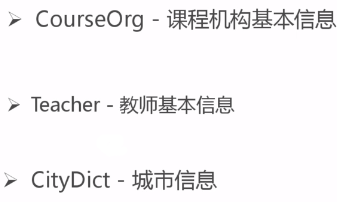
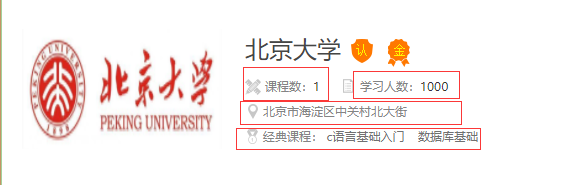
Among them, the number of courses and the number of students can be dynamically counted. Institutional Address, Institutional Classic Course.
Institutional lecturers, institutional courses can be obtained through foreign keys, not saved to the organization.
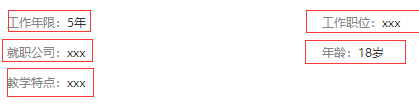
The fields required by the instructor are shown in the figure.
The organization/models.py code is as follows:
# encoding : utf-8 from datetime import datetime from django.db import models # Create your models here. # City Dictionary class CityDict(models.Model): name = models.CharField(max_length=20, verbose_name=u"City") # City Description: Standby may not be displayed desc = models.CharField(max_length=200, verbose_name=u"describe") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"City" verbose_name_plural = verbose_name # Course Institutions class CourseOrg(models.Model): name = models.CharField(max_length=50, verbose_name=u"Name of organization") # Institutional description, later replaced by rich text presentation desc = models.TextField(verbose_name=u"Mechanism description") click_nums = models.IntegerField(default=0, verbose_name=u"Number of clicks") fav_nums = models.IntegerField(default=0, verbose_name=u"Collection Number") image = models.ImageField( upload_to="org/%Y/%m", verbose_name=u"Cover Chart", max_length=100) address = models.CharField(max_length=150, verbose_name=u"Institutional address") # A city can have many curricula. By setting the foreign key of city, it can become a field of curricula. # We can find cities through institutions. city = models.ForeignKey(CityDict, verbose_name=u"City") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"Course Institutions" verbose_name_plural = verbose_name # lecturer class Teacher(models.Model): # There are many teachers in an organization, so we add foreign keys to the lecture table and save the name of the course organization. # It enables us to find corresponding institutions through lecturers. org = models.ForeignKey(CourseOrg, verbose_name=u"Subordinate institutions") name = models.CharField(max_length=50, verbose_name=u"Name of Teacher") work_years = models.IntegerField(default=0, verbose_name=u"Working life") work_company = models.CharField(max_length=50, verbose_name=u"Inaugural company") work_position = models.CharField(max_length=50, verbose_name=u"Company position") points = models.CharField(max_length=50, verbose_name=u"Teaching Characteristics") click_nums = models.IntegerField(default=0, verbose_name=u"Number of clicks") fav_nums = models.IntegerField(default=0, verbose_name=u"Collection Number") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"Teacher" verbose_name_plural = verbose_name
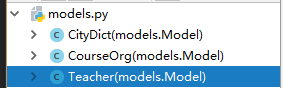
We can see that we have created three tables: city, course organization and lecturer.
This section corresponds to commit:
Course Institution app: City, Institution, Lecturer Form Written. Correspondence 4-7
4-8 operation models.py design
What tables are needed for analysis:
- Users can submit personal needs that I want to learn.
- Course review information for trainees
- Collection: You can collect public lectures, lecturers, teaching institutions, user message reminders.
- Personal Center: My course shows that the learning relationship between users and courses also needs to be preserved.
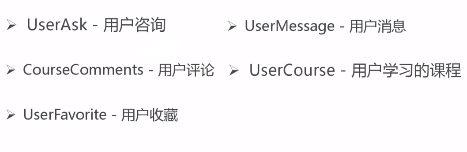
New operation app:
Click Run management.py Task under the Tools menu
startapp operation
operation/models.py adds code:
# encoding: utf-8 from datetime import datetime # Foreign key models needed to introduce our CourseComments from users.models import UserProfile from courses.models import Course # Users I want to learn form class UserAsk(models.Model): name = models.CharField(max_length=20, verbose_name=u"Full name") mobile = models.CharField(max_length=11, verbose_name=u"Mobile phone") course_name = models.CharField(max_length=50, verbose_name=u"Course Name") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Add time") class Meta: verbose_name = u"User consultation" verbose_name_plural = verbose_name # User comments on courses class CourseComments(models.Model): # There are two foreign keys involved: 1. Users, 2. Courses. import in course = models.ForeignKey(Course, verbose_name=u"curriculum") user = models.ForeignKey(UserProfile, verbose_name=u"user") comments = models.CharField(max_length=250, verbose_name=u"comment") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Comment time") class Meta: verbose_name = u"Course Review" verbose_name_plural = verbose_name # User Collection of Courses, Institutions, Lecturers class UserFavorite(models.Model): # It involves four foreign keys. Users, Courses, Institutions, Lecturers import TYPE_CHOICES = ( (1, u"curriculum"), (2, u"Course Institutions"), (3, u"lecturer") ) user = models.ForeignKey(UserProfile, verbose_name=u"user") # course = models.ForeignKey(Course, verbose_name=u "course") # teacher = models.ForeignKey() # org = models.ForeignKey() # fav_type = # Wise Edition # Save the user's id directly. fav_id = models.IntegerField(default=0) # Indicate the type of collection. fav_type = models.IntegerField( choices=TYPE_CHOICES, default=1, verbose_name=u"Collection type") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Commentary time") class Meta: verbose_name = u"User collection" verbose_name_plural = verbose_name # User Message Table class UserMessage(models.Model): # Because we have two kinds of messages: to the whole staff and to a certain user. # So if you use foreign keys, each message will have a user. It's hard to get full information. # The smart version is 0 for all users, not 0 is the id for users. user = models.IntegerField(default=0, verbose_name=u"Receiving user") message = models.CharField(max_length=500, verbose_name=u"Message content") # Read or not: Boolean Field False of Boolean type is not read, True means read has_read = models.BooleanField(default=False, verbose_name=u"Is it read?") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Adding time") class Meta: verbose_name = u"User message" verbose_name_plural = verbose_name # User Course Schedule class UserCourse(models.Model): # There are two foreign keys involved: 1. Users, 2. Courses. import come in. course = models.ForeignKey(Course, verbose_name=u"curriculum") user = models.ForeignKey(UserProfile, verbose_name=u"user") add_time = models.DateTimeField(default=datetime.now, verbose_name=u"Adding time") class Meta: verbose_name = u"User course" verbose_name_plural = verbose_name
So far: the design of data table models under our five operation s has been completed
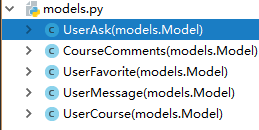
Configuration Add app in setting
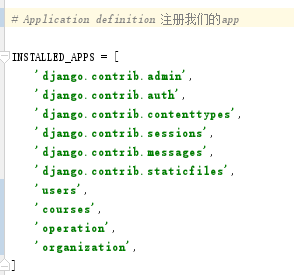
This section corresponds to commit:
models design under operation. User: Course & Message & Collection & Comment - I want to learn. Registered in setting s. Corresponding 4-8
4-9 Data Table Generation and apps Directory Establishment
Learn how to generate tables corresponding to a database through the models just designed
Click Run management.py Task under the Tools menu:
Python 2 is different from Python 3:
Some noASCII errors may be reported under Python 2:
Just add on the first line that corresponds to your Chinese writing:
# encoding: utf-8
Python 3 (django 2.0.1) will report errors:
org = models.ForeignKey(CourseOrg, verbose_name=u"Affiliate") TypeError: __init__() missing 1 required positional argument: 'on_delete'
This is because in 2.0.1, the foreign key relationship must specify the operation at the time of deletion.
For example, taxis belong to taxi companies. If the taxi company goes bankrupt, what should be done with these cars?
It must be pointed out by myself: I think cascade deletion can be done directly.
django provides:
- CASCADE
- PROTECT
- SET_NULL
- SET_DEFAULT
And other options. I chose CASCADE to delete.
Modify all foreign keys in the (dajngo 2.0.1) project to the following code:
That is to say, on_delete=models.CASCADE is added to make it cascade delete.
org = models.ForeignKey(CourseOrg, on_delete=models.CASCADE, verbose_name=u"Affiliate")
Makemirgration & migrate generation table
makemirgration migrate
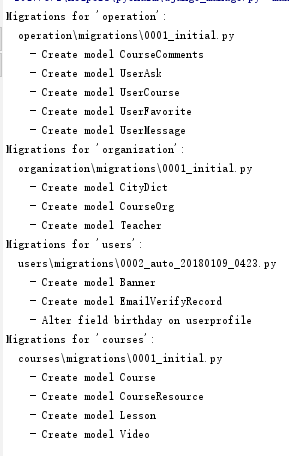
The figure above shows the information output in the makemirgration process. You can see the changes we've made.
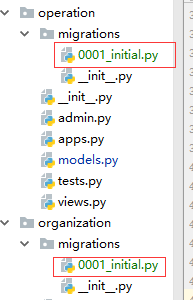
At this point, when we look at the migrations folder in the app directory, we can see the new files generated.
operation/migrations/0001_initial.py:
You can see that it's also Python's grammar. He'll help us generate data tables.
A new initial file will be generated each time migrations occur in the future. This is a very important change file and cannot be deleted at will.
Open Navicat to see that django's database has its default django_migrations table

Double-click the django_migrations table to see our migration record.
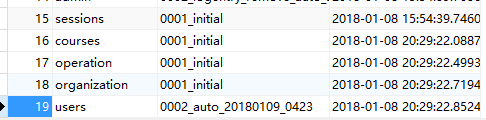
It records which initial.py is running under which app.
Enter Navicat to verify the success:
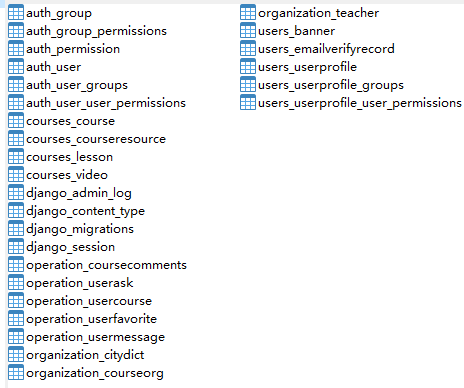
You can see that our table has been successfully generated, and the naming rule is: app name + our class name becomes lowercase
Put our four app s in a folder.
- New Python package: apps

- Drag all four apps into apps.
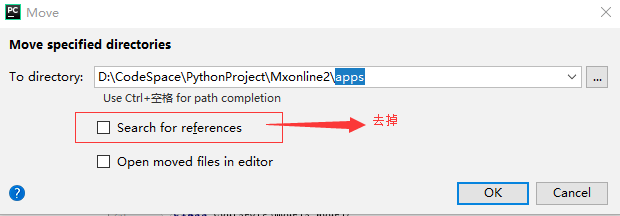
Remove the search for check. After dragging in, you will report an error and say you can't find the import modules.
Solution: Right-click Mark for sourceRoot. If you can't find it in the root directory, you will search under apps.
But at this time, there will still be errors under cmd.
Solution (Figure from my Django GetStarted tutorial):
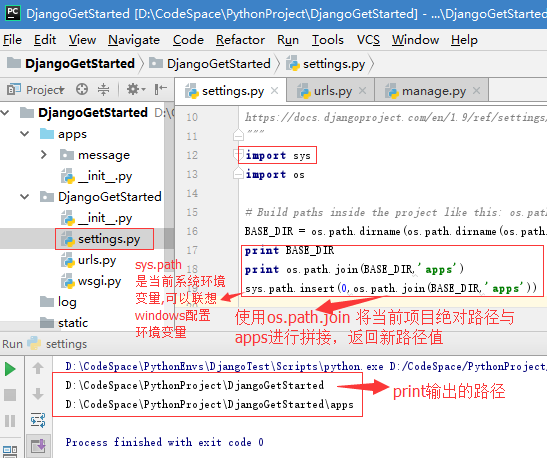
Similarly, inserting 0 is to hope that it first searches for something under our app:
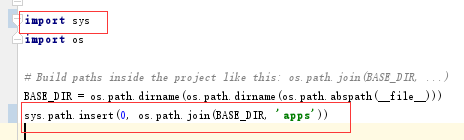
Success Verification
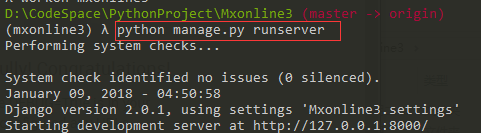
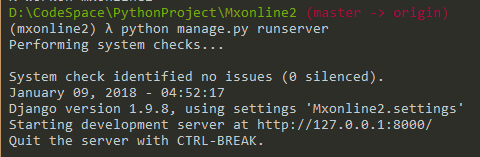
You can see that Django has been able to run properly.
Chapter IV Summary
- We designed app
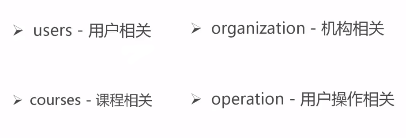
- Usemodels.py is designed.
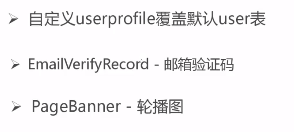
- Circular reference
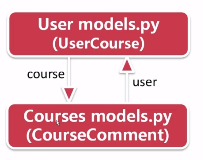
We need to create a higher level app. Layered design, operation at a higher level.
- Courses models.py
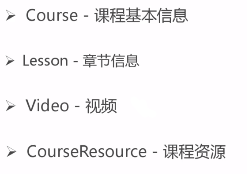
- organization models.py
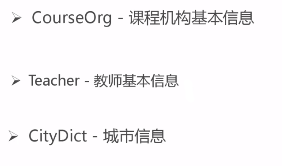
- operation models.py
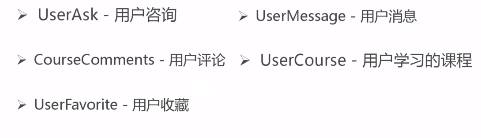
Generating table changes through makemigrations & migrate
The purpose of the migration directory under each app, and django_migration in the database
Place all app s in the same directory.
The end of this chapter corresponds to commit:
All data tables are generated, migration Directory & table django_migration. Place apps in the apps directory. Corresponding 4-9.
Chapter IV concludes! Scatter flowers.