catalogue
IO stream overview and classification
Byte stream abstract base class
FileOutputStream: the File output stream is used to write data to File
To write data using a byte output stream
Three ways to write data in byte stream
Byte stream write data to realize line feed and additional write
Byte stream write data plus exception handling
To read data using a byte input stream
Read one byte of data at a time
Read one byte array data at a time
Case: byte stream copy picture
IO stream overview and classification
Overview of IO streams
- IO: input / output
- Stream: it is an abstract concept, which is the general name of data transmission. In other words, the transmission of data between devices is called stream. The essence of stream is data transmission. IO stream is used to deal with the problem of data transmission between devices
- Common applications: file replication; File upload; File download
IO stream classification
- According to the flow direction of data
- Input stream: reading data
- Output stream: write data
- By data type
- Byte stream
- Byte input stream; Byte output stream
- Character stream
- Character input stream; Character output stream
- Byte stream
Generally speaking, we say that IO streams are classified according to data types
So when are both streams used?
- If the data is opened through the Notepad software provided by Window, we can also read the contents inside, and use the character stream, otherwise use the byte stream. If you don't know which type of stream to use, use byte stream
Byte stream
Byte stream write data
Byte stream abstract base class
- InputStream: this abstract class is a superclass that represents all classes of byte input stream
- OutputStream: this abstract class is a superclass representing all classes of byte output stream
- Subclass name features: subclass names are suffixed with their parent class name
FileOutputStream: the File output stream is used to write data to File
- FileOutputStream(String name): create a file output stream and write to the file with the specified name
- FileOutputStream(File file): creates a File output stream to write to the File represented by the specified File object
To write data using a byte output stream
- Create a byte output stream object (call the system function to create a file, create a byte output stream object, and let the byte output stream object point to the file)
- Call the write data method of byte output stream object
- Write character data, such as write 97, and display a in txt file; 57 is 9; 55 is 7
- The console has no output and writes directly to the file.
- byte[] getBytes(); : Return the byte array corresponding to the string, as follows: output result in fos.txt: abcde
FileOutputStream fos = new FileOutputStream("myByteStream\\fos.txt"); byte[] bys = "zbcde".getBytes(); fos.write(bys);
- Free resources (close this file output stream and free any system resources associated with this stream)
- colse();
import java.io.File; import java.io.FileOutputStream; import java.io.IOException; public class File0utputStreamDemo01{ public static void main(String[]args) throws IOException { //Create byte output stream object //FileOutputStream(String name): creates a file output stream and writes it to the file with the specified name FileOutputStream fos = new FileOutputStream( "myByteStream\\fos,txt"); /*Three things were done: A:The system function is called to create the file B:Created byte output stream object C: Let the byte output stream object point to the created file */ //void write(int b): writes the specified bytes to this file output stream fos.write(97); //fos.write(57); //fos.write(55); //Finally, we should release resources //void close(): closes the file output stream and frees any system resources associated with the stream. fos.close(); } }
Three ways to write data in byte stream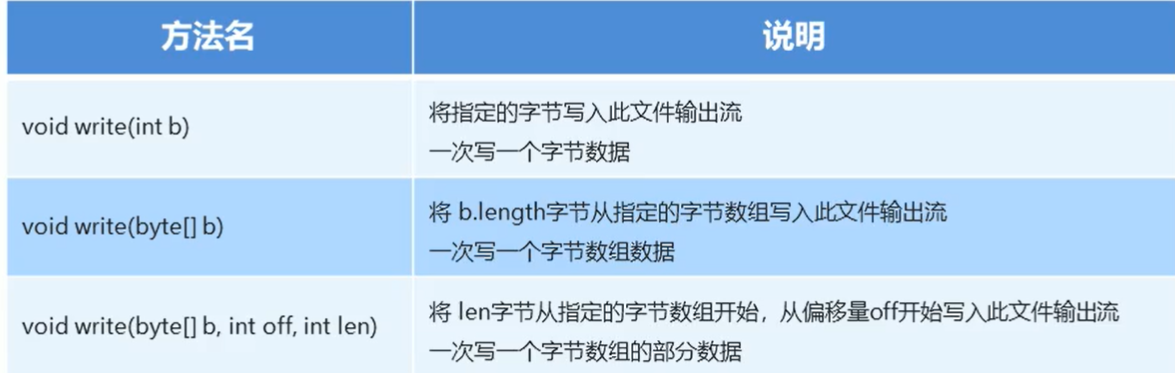
Byte stream write data to realize line feed and additional write
How to wrap byte stream write data?
fos.write("\n".getBytes());
window:\r\n
linux:\n
mac:\r
How to realize additional writing of byte stream write data?
- public FileOutputStream (String name,boolean append)
- Creates a file output stream and writes to the file with the specified name. If the second parameter is true, the bytes are written to the end of the file instead of the beginning
Byte stream write data plus exception handling
import java.io.File; import java.io.FileOutputStream; import java.io.IOException; public class File0utputStreamDemo01{ public static void main(String[]args){ //Join finalLy to release resources FileOutputStream fos = null; try { fos = new FileOutputStream("myByteStream\\fos.txt"); fos.write("hello".getBytes()); }catch (IOException e) { e.printStackTrace(); }finally { //Program robustness if(fos != null) { try { fos.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
Byte stream read data
Requirements: read the contents of the file fos.txt and output it on the console
FilelnputStream: get input bytes from files in the file system
- FilelnputStream(String name): create a filelnputstream by opening the connection with the actual file, which is named by the pathname name in the file system
To read data using a byte input stream
① Create byte input stream object
② Call the read data method of byte input stream object
③ Release resources
Read one byte of data at a time
- Method: int read();
- Read one byte of data from the input stream.
- If the end of the file is reached, the next byte of the data is - 1.
FileInputStream fis = new FileInputStream("myByteStream\\fos.txt"); int by; /* fis.read(): Read data by=fis.read(): Assign the read data to by by != -1: Judge whether the read data is - 1 */ while((by=fis.read())!=-1){ System.out.print((char)by); } //Release resources fis.close();
Read one byte array data at a time
-
-
String(byte[] bytes, int offset, int length) Construct a new String by decoding the specified byte subarray using the platform's default character set.
-
package lianxi; import java.io.FileInputStream; import java.io.IOException; public class Main { public static void main(String[] args) throws IOException { //Create byte input stream object FileInputStream fis = new FileInputStream("C:\\Users\\86183\\eclipse-workspace\\lianxi\\fis.txt"); byte[] bys = new byte[1024];//Generally, it is set to 1024 and its integer multiples int len; while((len=fis.read(bys)) != -1) { System.out.print(new String(bys,0,len)); } } }
Byte stream copy text file
Requirements:
Copy "E:\itcast \ window inside and window txt" to "window inside and window txt" under the module directory
analysis:
① Copying a text file actually reads the contents of the text file from one file (data source) and then writes them to another file (destination)
② Data source: E:litcast \ window txt --- read data --- InputStream--- FilelnputStream
③ Destination: myByteStream \ window. txt --- write data --- OutputStream--- FileOutputStream
Idea:
① Create byte input stream object from data source
② Create byte output stream object based on destination
③ Read and write data, copy text files (read one byte at a time, write one byte at a time)
④ Release resources
Code implementation:
Case: byte stream copy picture
package lianxi; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; public class Main { public static void main(String[] args) throws IOException { //Create byte input stream object FileInputStream fis = new FileInputStream("C:\\Users\\86183\\Desktop\\exo\\Bian Boxian\\b2.png"); //Create byte output stream object FileOutputStream fos = new FileOutputStream("C:\\Users\\86183\\Desktop\\exo\\b2.png"); //Read and write data, copy pictures byte[] bys = new byte[1024];//Generally, it is set to 1024 and its integer multiples int len; while((len=fis.read(bys)) != -1) { System.out.print(new String(bys,0,len)); } //Release resources fos.close(); fis.close(); } }
Note the format of the picture png or jpg
Byte buffer stream
Byte buffer stream:
- BufferOutputStream: this class implements a buffered output stream. By setting such an output stream, an application can write bytes to the underlying output stream without causing a call to the underlying system for each byte written
- BufferedInputStream: creating BufferedlnputStream will create an internal buffer array. When bytes are read or skipped from the stream, the internal buffer will be refilled from the included input stream as needed, many bytes at a time
Construction method:
- Byte buffered output stream: BufferedOutputStream(OutputStream) out)
- Input stream in byte buffer: BufferedInputStream(InputStream in)
Why does the construction method need a byte stream rather than a specific file or path?
- Byte buffer stream only provides buffer, and the real reading and writing data still depends on the basic byte stream object
package lianxi; import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; public class Main { public static void main(String[] args) throws IOException { //Byte buffered output stream: Buffered0utputStream(OutputStream out) BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("lianxi\\bos.txt")); //Write data bos.write("hello\r\n".getBytes()); bos.write("world\r\n".getBytes()); //Release resources bos.close(); //Byte buffered input stream: BufferedInputStream(InputStream in) BufferedInputStream bis = new BufferedInputStream(new FileInputStream("lianxi\\bos.txt")); //Read one byte array data at a time byte[]bys = new byte[1024]; int len; while((len=bis.read(bys))!=-1) { System.out.print(new String(bys,0,len)); } //Release resources bis.close(); } }
Case: byte stream copy video
The basic byte stream reads and writes one byte at a time Total time: 64565 MS
The basic byte stream reads and writes one byte array at a time Total time: 107 MS
The byte buffer stream reads and writes one byte at a time Total time: 405 MS
The byte buffer stream reads and writes one byte array at a time Total time: 60 ms
The byte buffer stream is the most efficient to read and write one byte array at a time.