1, Briefly answer the following questions
1. Briefly describe the provisions of identifiers in C language; What should you pay attention to when naming variables, arrays, and functions?
A: in the computer high-level language, the effective character sequences used to name variables, symbols, constant names, functions, arrays, types, etc. are collectively referred to as identifiers. C language stipulates that identifiers can only be composed of letters, numbers and underscores, and the first character must be letters or underscores.
2. What is an expression? If int x is defined in a program, is x=5 an expression? If yes, what are the values and types?
A: the formula connecting operands with C language operators is called expression. x=5 is an expression. Its type is int and its value is 5.
3. Defined statement char ch; What type of variable is ch? How many bytes does it occupy in memory? What function can be used to find the number of bytes? What data does this type of variable usually store? Give me an example.
A: ch is a character variable, which occupies 1 byte in memory. You can find its corresponding number of bytes through sizeof function. This type of variable is usually used to store characters. For example, char ch = 'A' defines a character variable ch, whose initial value is a. because it is essentially an integer variable of one byte, you can assign an integer between 0 and 127 to a character variable.
4. Write out the general form of if...else statement and draw the flow chart.
A: the general form is as follows:
if(expression){ Statement 1 }else{ Statement 2 }
The flow chart is as follows:
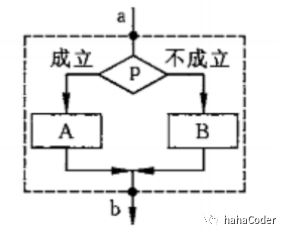
In the above figure, p represents the judgment condition. When the judgment condition is true, execute the program in box A. if the judgment condition is not true, execute the program in box B.
5. Write out the general form of the for statement and draw the flow chart.
A: the general form is as follows:
for(Expression 1;Expression 2;Expression 3){ sentence }
Among them, expression 1 is used to set the initial conditions and is executed only once; Expression 2 is a loop condition expression used to determine whether to continue the loop; As a loop adjustment, expression 3 is generally executed after the loop body.
The flow chart is as follows:
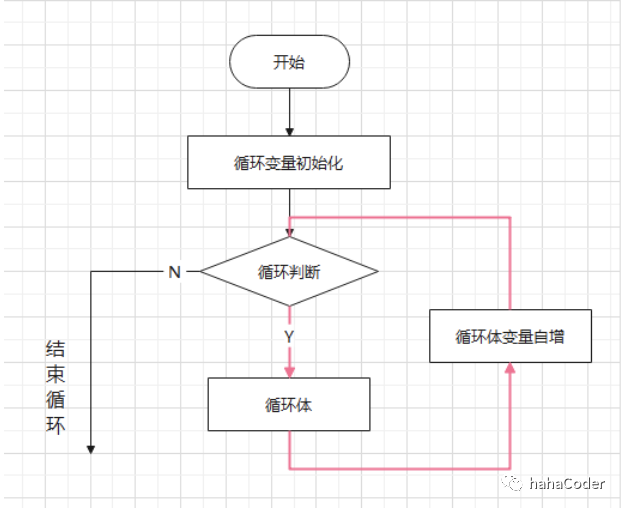
6. If the following two-dimensional array is defined, float x[3][4]: Please list each element of X one by one according to the storage order in memory; Generally speaking, if an array has m columns, how many elements are there before the elements in row i and column j of the array?
A: the arrangement order of two-dimensional array in memory is stored by row, that is, the first row of elements are stored in memory first, and then the second row of elements. And so on. For two-dimensional array float x[3][4], the storage order in memory is: x[0][0],x[0][1],x[0][2],x[0][3],x[1][0],x[1][1],x[1][2],x[1][3],x[2][0],x[2][1],x[2][2],x[2][3], For an array with m columns, there are i*m+j elements before array element a[i][j].
7. A program can have at most one main function? Who calls the main function? What is the return value commonly used for?
A: a program can have at most one main function. The main function is called by the operating system. Its return value is used to describe the exit status of the program. If 0 is returned, it represents the normal exit of the program, and the meaning of other numbers is determined by the system. Generally, the return of non-zero represents the abnormal exit of the program.
8. If a pointer P points to an integer variable a, what is stored in variable p? What does the expression (char *)p point to? What does the expression (char *)p+1 point to?
A: the address of integer variable a is stored in variable p, (char *)p points to a character pointer, (char *)p+1 also points to a character pointer, and the position difference between (char *)p+1 and (char *)p is one byte.
Please see the demonstration case:
#include<stdio.h> #include<stdlib.h> int main(){ int a = 3; int *p = &a; printf("%d\n",(char *)(p+1)-(char *)p); // 4 printf("%d\n",(char *)p+1-(char *)p); // 1 return 0; }
9. There is struct pos2d{int x,int y;} in a program; What does this statement define? What is pos2d? Does the system allocate memory to it? After that, there is struct pos2d point; What is the definition? Is memory allocated to point? If allocated, how do I get the number of bytes allocated?
A: this statement defines a structure named pos2d, which contains two members: integer variable x and integer variable y; Pos2d is the structure name; Since the above code only establishes a structure model and does not define variables, there is no specific data, and the system does not allocate storage units to them; struct pos2d point; A structure variable named point is defined, and the system will allocate storage units to it, occupying a total of 8 (4 + 4) bytes.
10. a program called the file open function is as follows: fp=fopen("file.dat","rb"); Please describe its function, and tell the difference between text file and binary file.
A: this line of code opens the file.dat FILE in binary reading mode through fopen function. If the FILE is successfully opened, it returns a pointer of FILE type. If the FILE fails to be opened, it returns NULL, and its return value is saved in fp.
Difference between binary file and text file:
Binary file refers to the data stored in binary form in memory and output to external memory without conversion. It can be considered as the data image stored in memory, that is, image file; If it is required to store in the form of ASCII code on external memory, it needs to be converted before storage. ASCII file is also called text file, and each byte stores the ASCII code of one character. Generally, all characters are stored in ASCII form, and numerical data can be stored in ASCII form or binary form.
2, Program reading questions
1. Character pointer variable
#include<stdio.h> void f1(char *t,char *s); int main() { char *p="a1b2c3d4e5", st[20]; printf("%s\n",p+2); f1(st,p); printf("%s\n",st); return 0; } void f1(char *t,char *s) { int i; for(i=0;*(s+i)!='\0';i++) { *(t++)=*(s+2*i); if(*(s+2*i)=='\0'|| *(s+2*i+1)=='\0'){ break; } } *t='\0'; return ; }
Answer: the program output results are as follows:
b2c3d4e5 abcde
The program first declares a function named f1, which receives two parameters, namely, pointer variable t pointing to char type and pointer variable s pointing to char type. In f1 function, there is a for loop to copy the characters at even positions in the string indicated by pointer variable s into the string indicated by pointer variable t, That is, the string indicated by the pointer variable t stores the characters corresponding to the even position in the string indicated by the pointer variable S. after completing the above operations, manually add \ 0 as the end flag of the valid string at the end of the string indicated by the pointer variable T. in the main program, first define a character pointer variable P, By default, the pointer variable p stores the address of the first character of the string a1b2c3d4e5, followed by printf("%s\n",p+2); For output, the pointer variable p+2 should point to the position of the character B, so the final output result is: b2c3d4e5, then the f1 function is invoked in the main program and output, the output of the character in the even position of the original string, that is, abcde.
2. Switch case statement
#include<stdio.h> int main() { float score; char grade; int i,k; for(i=0;i<5;i++) { scanf("%f",&score); k=(int)(score/10); if(score>100){ k++; } switch(k) { case 0: case 1: case 2: case 3: case 4: case 5:grade='E';break; case 6:grade='D';break; case 7:grade='C';break; case 8:grade='B';break; case 9: case 10:grade='A';break; default: grade='n'; } if(grade=='n') { printf("input data wrong!\n"); } else { printf("score=%6.1f-->grade=%c\n",score,grade); } } return 0; }
Answer: the program output results are as follows:
33.5 score= 33.5-->grade=E 89.2 score= 89.2-->grade=B 93.6 score= 93.6-->grade=A 100 score= 100.0-->grade=A 101 input data wrong!
The program first declares a float type variable score and a char type variable grade, and then declares two integer variables i and k. in the for loop, first receives the score value entered by the user from the keyboard, then divides the score value by 10 and forcibly rounds it, and assigns its calculation result to the integer variable k. if the score value is greater than 100, execute the k + + operation, Then execute the switch case statement, return different grade values according to different k values, and finally judge according to the if..else selection statement. If the corresponding grade value is n, output input data wrong! Otherwise, the corresponding score value and its corresponding grade value are output, and the cycle is executed for 5 times.
For example, when the input score value is 33.5, k=3 and the grade value is e, so the final output result is: score = 33.5 -- > grade = E; When the input score value is 89.2, k=8 and grade value is B, so the final output result is: score = 89.2 -- > grade = B; When the input score value is 93.6, k=9 and grade value is A, so the final output result is: score = 93.6 -- > grade = A; When the input value score is 100, k=10 and the grade value is A, so the final output result is: score = 100.0 -- > grade = A; When the input score value is 101, k=11 and the grade value is n, so the final output result is: input data wrong!.
3. Character subtraction
#include<stdio.h> int MyStrCmp(char *s,char *t); int main() { char *str1="ab",*str2="ac",*str3="AB"; char *str4="abc",*str5="ab",*str6="a"; printf("%d\n",MyStrCmp(str1,str2)); printf("%d\n",MyStrCmp(str1,str3)); printf("%d\n",MyStrCmp(str1,str4)); printf("%d\n",MyStrCmp(str1,str5)); printf("%d\n",MyStrCmp(str1,str6)); return 0; } int MyStrCmp(char *s,char *t) { int r; while(*s !='\0' && *t !='\0') { if(*s!=*t){ break; } s++; t++; } r=*s - *t; return r; }
Answer: the output results of program operation are as follows:
-1 32 -99 0 98
The program first declares a function named MyStrCmp, which receives two parameters for comparing two string parameters. When the first unequal character is encountered in the comparison process, it returns the difference of the subtraction of its corresponding character. If the two strings are equal, the return value is 0. In the main program, six character pointer variables are defined, and then MyStrCmp function is called five times to return its corresponding results.
For example, when the parameters of MyStrCmpy function are character pointer variables str1 and str2, the final return result is - 1, that is, 98-99 = - 1; When the parameters of MyStrCmpy function are character pointer variables str1 and str3, the final return result is 32, that is, 97-65 = 32; When the parameters of MyStrCmpy function are character pointer variables str1 and str4, the final return result is - 99, that is, 0-99 = - 99; When the parameters of MyStrCmpy function are character pointer variables str1 and str5, the final return result is 0, that is, 0-0 = 0; When the parameters of MyStrCmpy function are character pointer variables str1 and str6, the final return result is 98, that is, 98-0 = 98.
4. static local variable
#include<stdio.h> int fun(void); int main() { int i; for(i=0;i<5;i++){ printf("%d\n",fun()); } return 0; } int fun(void) { static int f1=1,f2=1; int r; r=f1+f2; f1=f2; f2=r; return r; }
Answer: the output results of program operation are as follows:
2 3 5 8 13
The program first declares a nonparametric function named fun, and then calls fun function five times through the for loop in the main program. In the definition of fun function, integer variables f1 and f2 are declared as static local variables, so their values do not disappear after the function call is completed. The value corresponding to this variable is the value at the end of the last function call when the function is called next time.
For example, when the first cycle ends, the corresponding values of each variable are: r= f1+f2=1+1=2,f1=1,f2=2, so the return result of the first program is 2; When the second cycle ends, the corresponding values of each variable are: r=f1+f2=1+2=3,f1=2,f2=3, so the return result of the second program is 3; When the third cycle ends, the corresponding values of each variable are: r=f1+f2=2+3=5,f1=3,f2=5, so the return result of the third program is 5; When the fourth cycle ends, the corresponding values of each variable are: r=f1+f2=3+5=8,f1=5,f2=8, so the return result of the fourth program is 8; When the fifth cycle ends, the corresponding values of each variable are: r=f1+f2=5+8=13,f1=8,f2=13, so the return result of the fifth program is 13. To sum up, the return result of the program at the end of the above five cycles is the final output result of the program.
5. Structure + file operation + sorting
#include<stdio.h> struct person { char name[40]; int age; }; void MyFunc (struct person *ps,int n); int main() { struct person team[5]; FILE *fp; int i; fp=fopen("input.txt","r"); if(fp==NULL) return 1; for(i=0;i<5;i++){ fscanf(fp,"%s%d",team[i].name,&(team[i].age)); } fclose(fp); MyFunc (team,5); for(i=0;i<5;i++){ printf("%s %d\n",team[i].name,team[i].age); } return 0; } void MyFunc (struct person *ps,int n){ int i,j,k; struct person t; for(i=0;i<n-1;i++) { k=i; for(j=i+1;j<n;j++){ if(ps[j].age>ps[k].age)k=j; } t=ps[i]; ps[i]=ps[k]; ps[k]=t; } return; }
Answer: the output results of program operation are as follows:
zh3 88 li4 55 ma6 35 zhao7 28 wang5 10
This program first defines a structure named person, which contains two members: name and age. Then, open the input.txt data file in read-only mode in the main program. After successful opening, read the contents corresponding to name and age one by one. After reading, close the file and continue to execute MyFunc function, The function of this function is to sort the data in the structure according to age, so the results are shown above.
3, Programming problem
1. Write a program to output all prime numbers between 100 and 200 (prime numbers refer to integers that can only be divided by 1 and themselves, but not by other numbers).
A: the procedure is as follows:
#include<stdio.h> int main(){ int i, j; for (i = 100; i <=200; i++){ for (j = 2; j < i; j++){ if (i%j == 0){ break; } } if (j==i){ printf("%d\n", i); } } return 0; }
2. Write a function to complete the exchange of the contents of two integer variables, and pointer parameters are required; And write a main function calling the function, read in the 10 integers entered by the keyboard, sort them from small to large, and output the sorting results.
A: the procedure for exchanging the contents of two integer variables is as follows:
#include<stdio.h> int main(){ void swap(int *p1,int *p2); int a,b; int *pointer_1,*pointer_2; printf("Please enter two integers:"); scanf("%d %d",&a,&b); pointer_1 = &a; pointer_2 = &b; swap(pointer_1, pointer_2); printf("a = %d,b = %d\n",a,b); return 0; } // Swap what two pointers point to void swap(int *p1,int *p2){ int temp; temp = *p1; *p1 = *p2; *p2 = temp; }
The sorting code is as follows: (prompt: select sorting or bubble sorting)
#include<stdio.h> int main(){ void sort(int arr[],int n); int *p,arr[10]; p = arr; printf("Please enter an array element:"); for (int i = 0; i < 10; i++) { scanf("%d",p++); } p = arr; sort(p, 10); printf("The order from small to large is:\n"); for (p = arr; p < (arr+10); p++) { printf("%3d",*p); } printf("\n"); return 0; } // Select sort void sort(int *arr,int n){ void swap(int *p1,int *p2); int i,j,k; for (i = 0; i < n-1; i++) { k = i; for (j = k+1; j < n; j++) { if(*(arr+j)<*(arr+k)){ k = j; } } if(k!=i){ swap(arr+i,arr+k); } } } // Swap what two pointers point to void swap(int *p1,int *p2){ int temp; temp = *p1; *p1 = *p2; *p2 = temp; }
3. Write a function to count the number of occurrences of a character in a given string, and write a main function that calls it to count @ and # occurrences of the string entered on the keyboard.
A: the procedure is as follows:
#include<stdio.h> int main(){ int chCount(char str[],char ch); char str[100]; printf("Please enter a string:\n"); scanf("%s",str); printf("@The number of occurrences is:%d,#The number of occurrences is:%d\n",chCount(str,'@'),chCount(str,'#')); return 0; } // Counts the number of occurrences of a specific character int chCount(char str[],char ch){ int count = 0; for (int i = 0; str[i]!='\0'; i++) { if(str[i]==ch){ count++; } } return count; }
4. Input the data of 40 students from the keyboard. The data of each student includes name (assuming there is no space in the middle) and three course scores. Calculate the average score of each student's three courses, and write each student's name, three course scores and average scores into a text file ouput.txt.
A: the procedure is as follows:
#include<stdio.h> #include<stdlib.h> #define NUM 40 struct Student{ char name[20]; float score[3]; float aver; }; int main(){ void input(struct Student student[]); void writeToText(struct Student student[]); void read(struct Student student[]); struct Student student[NUM],*p = student; input(p); writeToText(p); /* Write the file in binary form. In order to verify the correctness of the written file, redefine a read function and read it in binary form. If the content can be read correctly, then Indicates that the program is correct. During the exam, you can not write the read function for verification. */ read(p); return 0; } // Read in the data and calculate the aver value void input(struct Student student[]){ for (int i = 0; i < NUM; i++) { printf("Please enter page%d Names of students and grades of three courses:\n",i+1); scanf("%s %f %f %f",student[i].name,&student[i].score[0],&student[i].score[1],&student[i].score[2]); student[i].aver = (student[i].score[0]+student[i].score[1]+student[i].score[2])/3.0; } } // write file void writeToText(struct Student student[]){ FILE *fp; if((fp=fopen("output.txt", "wb"))==NULL){ printf("File opening failed!\n"); exit(0); } for (int i = 0; i < NUM; i++) { if(fwrite(&student[i], sizeof(struct Student), 1, fp)!=1){ printf("Error writing file!\n"); } } fclose(fp); printf("File written successfully!\n"); } // Verify whether the data written in the file is correct. It can be written or not during the exam. void read(struct Student student[]){ FILE *fp; if((fp = fopen("output.txt", "rb"))==NULL){ printf("Cannot open file!\n"); exit(0); } for (int i = 0; i < NUM; i++) { fread(&student[i], sizeof(struct Student), 1, fp); printf("full name:%s\t Results of three courses:%.2f,%.2f,%.2f\t Average score:%.2f\n",student[i].name,student[i].score[0],student[i].score[1],student[i].score[2],student[i].aver); } fclose(fp); }
5. Write a one-way linked list processing program. The node definition is as follows:
struct student { char name[40]; int score; struct student *next; };
According to the names and scores of 5 people entered on the keyboard, establish a one-way linked list, sort according to the scores (the ascending and descending order is determined by yourself), and finally output the names and scores of each node of the linked list (sorted).
A: the procedure is as follows:
#include<stdio.h> #include<stdlib.h> #include<string.h> #define NUM 5 struct student{ char name[40]; int score; struct student *next; }; struct student *createList(); struct student *bubbleSort(struct student *head); void displayList(struct student *head); struct student *head = NULL; struct student *bubble_head = NULL; int main(){ head = createList(); bubble_head = bubbleSort(head); displayList(bubble_head); return 0; } // 1. Establish student information struct student *createList(){ struct student *head;//Head node struct student *p1;//Open up new nodes struct student *p2;//Connect to p1 char name[40]; int score; head = NULL; for (int count = 1; count <= NUM; count++){ printf("Please enter page%d Names and scores of students(Separated by spaces):",count); scanf("%s %d",name,&score); p1 = (struct student*)malloc(sizeof(struct student)); strcpy(p1->name,name); p1->score = score; p1->next = NULL; if(head == NULL){ head = p1; }else{ p2->next = p1; } p2 = p1; } return head; } // 2. Bubble sorting struct student *bubbleSort(struct student *head){ struct student *p,*q; int temp1,i; char temp2[40]; for (p = head,i=1;i < NUM; i++,p=p->next) { for (q = p->next; q!=NULL; q = q->next) { if(p->score < q->score){ // Exchange score temp1 = p->score; p->score = q->score; q->score = temp1; // Swap name strcpy(temp2, p->name); strcpy(p->name, q->name); strcpy(q->name, temp2); } } } return head; } // 3. Output student information in the same order as the establishment void displayList(struct student *head){ struct student *p; int n = 0; if(head!=NULL){ printf("Output the information in the linked list in sequence as follows:\n"); for(p=head;p!=NULL;p=p->next){ printf("full name:%20s fraction:%6d\n",p->name,p->score); n++; } printf("Total number of students:%d\n",n); }else{ printf("Empty linked list!\n"); } }