To learn mybatis, you can follow the official website to learn http://www.mybatis.org/mybatis-3/configuration.html ා properties
There are two ways to write mybatis? HelloWorld 2.2 Mybatis? Helloworld02 (interface programming) Now, the second way is commonly used: interface programming
Let's look at the first way:
Step:
- Import jar package
- Configure the global configuration file mybatis-config.xml
- Configuration mapping file xxxMapper.xml
1. Directory structure:
RE2A9J%24MP~S%60%60XEV6I4M.png)
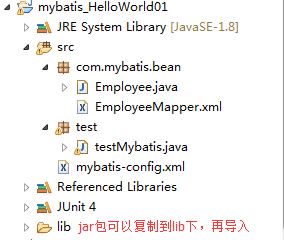
2. Complete code:
Employee.java
package com.mybatis.bean; public class Employee { private Integer id; private String lastName; private String email; private String gender; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } @Override public String toString() { return "Employee [id=" + id + ", lastName=" + lastName + ", email=" + email + ", gender=" + gender + "]"; } }
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!-- Print on console sql Sentence --> <settings> <setting name="logImpl" value="STDOUT_LOGGING"/> </settings> <!-- The following configurations need to correspond to their own database information --> <environments default="development"> <environment id="development"> <transactionManager type="JDBC"/> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver"/> <property name="url" value="jdbc:mysql://localhost:3306/mybatis"/> <property name="username" value="root"/> <property name="password" value="shapolang"/> </dataSource> </environment> </environments> <!-- Written sql The mapping file must be registered in the global configuration file --> <mappers> <mapper resource="com/mybatis/bean/EmployeeMapper.xml"/> </mappers> </configuration>
Configuration mapping file EmployeeMapper.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <! -- namespace: namespace id: unique identifier resultType: object type returned If some fields of the database are different from the member variables of the object, you can alias these fields of the database (for example, the last name of the database field is different from the last name of the object variable) --> <mapper namespace="com.mybatis.bean.EmployeeMapper"> <select id="selectEmployee" resultType="com.mybatis.bean.Employee"> select id,last_name lastName,email,gender from tbl_employee where id = #{id} </select> </mapper>
Test file testMabatis.java
package test; import java.io.IOException; import java.io.InputStream; import java.io.Reader; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import org.junit.Test; import com.mybatis.bean.Employee; /* * 1.Create a SqlSessionFactory object based on the xml configuration file (global configuration file) * There are some running environment information of data source * 2.sql Mapping file: each sql is configured, as well as the encapsulation rules of sql * 3.Register the sql mapping file in the global configuration file * 4.Write code: * 1)Get SqlSessionFactory according to the global configuration file; * 2)Use sqlSession factory to get sqlSession object and use it to add, delete, modify and query * An sqlSession is a conversation between the representative and the database, and it is closed when it is used up * 3)Use the unique identifier of sql to tell MyBatis to execute that sql. sql is saved in the sql mapping file. */ public class testMybatis{ @Test public void test() throws IOException { String resource = "mybatis-config.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); SqlSessionFactory sf =new SqlSessionFactoryBuilder().build(inputStream); //Create a SqlSession instance, which can directly execute the mapped sql statements //Session.selectone (unique identification of sql, parameters to execute sql); SqlSession session = sf.openSession(); try { Employee emp = session.selectOne( "com.mybatis.bean.EmployeeMapper.selectEmployee", 1); System.out.println(emp); } finally { session.close(); } } }
Console print display: (the console print sql statement needs to be set, see MyBatis3 Output SQL in the console with log4j)
==> Preparing: select id,last_name lastName,email,gender from tbl_employee where id = ? ==> Parameters: 1(Integer) <== Columns: id, lastName, email, gender <== Row: 1, aa, aa@qq.com, 1 <== Total: 1 Employee [id=1, lastName=aa, email=aa@qq.com, gender=1]