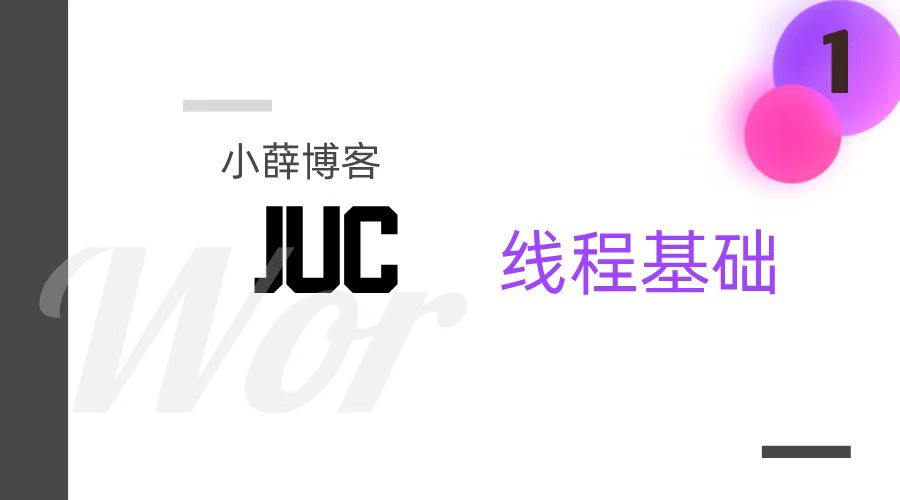
1. Java multithreading related concepts
1. Process
It is the second execution of the program and the unique unit of the system for resource allocation and scheduling. Each process has its own memory space and system resources
2. Thread
Multiple tasks can be executed in the same process. Each task can be regarded as one thread. Each process will have one or more threads
3. Tube side
Monitor, which is what we usually call lock
// Monitor is actually a synchronization mechanism. Its obligation is to ensure that only one thread can access the protected data and code at the same time. // Synchronization in the JVM is based on entering and exiting Monitor objects (Monitor, management objects). Each object instance will have a Monitor object, Object o = new Object(); new Thread(() -> { synchronized (o) { } },"t1").start(); // The Monitor object will be created and destroyed together with the Java object, and its bottom layer is implemented by C + + language.
4. Thread status?
// Thread.State public enum State { NEW,(newly build) RUNNABLE,((ready) BLOCKED,(Blocking) WAITING,((see you or leave) TIMED_WAITING,(Obsolete (not waiting) TERMINATED;(End) }
5. What is the difference between wait/sleep?
The function is to suspend the current thread. What's the difference?
wait, let go to sleep, let go of the lock in your hand
Sleep clenched his hand and went to sleep. When he woke up, he still had a lock in his hand
2. Why is multithreading extremely important???
- Hardware - Moore's law failure
Moore's Law:
It was proposed by Gordon Moore, one of the founders of Intel. The contents are as follows:
When the price remains unchanged, the number of components that can be accommodated on the integrated circuit will double every 18-24 months, and the performance will also double.
In other words, the computer performance that every dollar can buy will more than double every 18-24 months. This law reveals the speed of information technology progress.
However, since 2003, the main frequency of CPU has no longer doubled, but adopts multi-core instead of faster main frequency.
Moore's law fails.
When the dominant frequency is no longer increased and the number of cores is increasing, parallel or concurrent programming is needed to make the program faster.
- Software aspect
High concurrency system, asynchronous + callback and other production requirements
3. start with a thread
// Java thread understanding and implementation in openjdk private native void start0(); // The bottom layer of Java language itself is C + + language
OpenJDK source code website: http://openjdk.java.net/
openjdk8\hotspot\src\share\vm\runtime
Interpretation of more underlying C + + source code
openjdk8\jdk\src\share\native\java\lang thread.c java Thread is through start The main contents are native method start0 In, Openjdk Write JNI Generally, they correspond to each other one by one, Thread.java The corresponding is Thread.c start0 Actually JVM_StartThread. When you view the source code, you can see that jvm.h Statement found in, jvm.cpp It is implemented in.
openjdk8\hotspot\src\share\vm\prims jvm.cpp
openjdk8\hotspot\src\share\vm\runtime thread.cpp
4. User thread and daemon thread
Java threads are divided into user threads and daemon threads. If the daemon attribute of a thread is true, it means daemon threads and false means user threads
Daemon thread
It is a special thread that silently completes some systematic services in the background, such as garbage collection thread
User thread
It is the working thread of the system, which will complete the business operations that the program needs to complete
public class DaemonDemo{ public static void main(String[] args){ Thread t1 = new Thread(() -> { System.out.println(Thread.currentThread().getName()+"\t Start running,"+(Thread.currentThread().isDaemon() ? "Daemon thread":"User thread")); while (true) { } }, "t1"); //The daemon attribute of a thread is true, which means it is a daemon thread, and false means it is a user thread t1.setDaemon(true); t1.start(); //After 3 seconds, the main thread will run again try { TimeUnit.SECONDS.sleep(3); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("----------main The thread has finished running"); } }
a key
When all user threads in the program are executed, the system will automatically exit whether the daemon thread ends or not
If all user threads end, it means that the business operations that the program needs to complete have ended, and the system can exit. So when there are only daemons left in the system, the java virtual opportunity exits automatically
Setting the daemon thread needs to be done before the start() method
5. How many ways to get multithreading?
-
Traditionally
- Inherit thread class
- Implement the runnable interface,
-
After java5
- Implement the callable interface
- java thread pool access
6. Callable interface
1. Compared with runnable
// Create a new class MyThread to implement the runnable interface class MyThread implements Runnable{ @Override public void run() { } } // The new class MyThread2 implements the callable interface class MyThread2 implements Callable<Integer>{ @Override public Integer call() throws Exception { return 200; } } // Interview question: what is the difference between callable interface and runnable interface? // Answer: (1) is there a return value // (2) Throw exception or not // (3) The landing methods are different. One is run and the other is call
2. How
Is it feasible to replace runnable directly?
This is not feasible because the thread class has no Callable constructor at all
Meet different people and find a middleman
FutureTask ft = new FutureTask(new MyThread2()); new Thread(ft, "AA").start();
How to get the return value after running successfully?
FutureTask ft = new FutureTask(new MyThread2()); new Thread(ft, "AA").start(); System.out.println(ft.get());