GateWay gateway
An important component in the Cloud component bucket is the gateway. Zuul gateway is adopted in version 1.x;
However, in version 2.x, Zuul's upgrade has been skipped. Spring cloud finally developed a gateway to replace Zuul
GateWay provides a simple and effective way to route API s and provides some powerful filter functions, such as fusing, current limiting, Retry, etc
Reason for choosing GateWay
Zuul 1's model
Three concepts
Route: the basic module for building a gateway. It consists of ID, target URI and a series of assertions
And filter. If the assertion is true, the route will be matched
Predicate: the developer can match all the contents of the HTTP request (such as the request header or request parameters), and route the request if it matches the assertion
Filter: it is an instance of GateWay in the spring cloud framework. Using the filter, you can modify the request before or after it is routed
Build gateway 9527
- Create a new module cloud gateway gateway 9527
- pom
The pom file for the gateway does not require web and auactor dependencies
<dependencies> <!--gateway--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-gateway</artifactId> </dependency> <!-- Reference self defined api General package, you can use Payment payment Entity --> <dependency> <groupId>com.atguigu.springcloud</groupId> <artifactId>cloud-api-commons</artifactId> <version>${project.version}</version> </dependency> <!--eureka client(Dynamic routing through microservice name)--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <!--Hot deployment--> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
- yml
server: port: 9527 spring: application: name: cloud-gateway eureka: instance: hostname: cloud-gateway-service client: fetch-registry: true register-with-eureka: true service-url: defaultZone: http://eureka7001.com:7001/eureka/
- Main startup class
@SpringBootApplication @EnableEurekaClient public class GatewayMain9527 { public static void main(String[] args) { SpringApplication.run(GatewayMain9527.class,args); } }
- Modify yml file
server: port: 9527 spring: application: name: cloud-gateway cloud: gateway: routes: - id: payment_route # The id of the route. There are no rules, but it is required to be unique. It is recommended to cooperate with the service name #The routing address of the service provided after matching uri: http://localhost:8001 predicates: - Path=/payment/get/** # Assert that the path matches the route - id: payment_route2 uri: http://localhost:8001 predicates: - Path=/payment/lb/** #Assert that the path matches the route eureka: instance: hostname: cloud-gateway-service client: fetch-registry: true register-with-eureka: true service-url: defaultZone: http://eureka7001.com:7001/eureka/
- Test start 7001 gateway 9527 8001
Access 8001 via 9527
Predict is true, access succeeded, false access failed
Two ways to configure routing
- Configure in configuration file yml
- Configure in configuration class
Inject the Bean of RouteLocator into the code
Configuration class configuration
@Configuration public class GateWayConfig { @Bean public RouteLocator customRouteLocator(RouteLocatorBuilder routeLocatorBuilder){ RouteLocatorBuilder.Builder routes = routeLocatorBuilder.routes(); routes.route("path_route", r -> r.path("/guonei") // Equivalent to localhost:9527/guonei go to Baidu below .uri("http://news.baidu.com/guonei")).build(); return routes.build(); } }
Test: http://localhost:9527/guonei
Dynamic routing
- Modify yml file
spring: application: name: cloud-gateway cloud: gateway: discovery: locator: enabled: true #Enable the function of dynamically creating routes from the registry, and route using the microservice name (false by default) routes: - id: payment_routh #The id of the route. There are no rules, but it is required to be unique. It is recommended to cooperate with the service name # uri: http://localhost:8001 # matches the routing address of the service uri: lb://CLOUD-PAYMENT-SERVICE predicates: - Path=/payment/get/** #Assert that the path matches the route - id: payment_routh2 # uri: http://localhost:8001 uri: lb://CLOUD-PAYMENT-SERVICE predicates: - Path=/payment/lb/** #Assert that the path matches the route
Test, start 700180029527
http://localhost:9527/payment/lb
Use of common Predicate
Official website: https://cloud.spring.io/spring-cloud-static/spring-cloud-gateway/2.2.1.RELEASE/reference/html/#gateway-request-predicates-factories
After/Before/Between
First use a test class to get the current time
import java.time.ZonedDateTime; public class Test2 { public static void main(String[] args) { //Get current time string ZonedDateTime now = ZonedDateTime.now();//Default time zone System.out.println(now); //2021-12-04T14:32:44.032+08:00[Asia/Shanghai] } } } 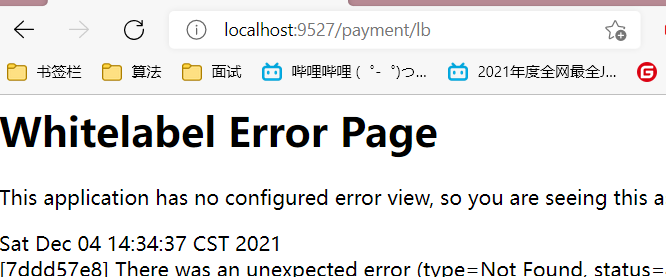
Add in yml file
predicates: - Path=/payment/lb/** #Assert that the path matches the route # The time is one hour later than the current time - After=2021-12-04T15:32:44.032+08:00[Asia/Shanghai]
Test, start 700180029527
http://localhost:9527/payment/lb
#Cannot access Before the specified time (Before) - Before=2021-12-04T15:32:44.032+08:00[Asia/Shanghai] #Can only be accessed within a specified time (Between) - Between=2021-12-04T15:32:44.032+08:00[Asia/Shanghai],2021-12-04T16:32:44.032+08:00[Asia/Shanghai]
Cookie Route Predicate
Two parameters are required, one is Cookie name and the other is regular expression
The routing rule will obtain the corresponding Cookie name value and regular expression. If there is a match, the routing will be executed. If there is no match, the routing will not be executed
predicates: - Path=/payment/lb/** #Assert that the path matches the route - After=2021-12-04T15:32:44.032+08:00[Asia/Shanghai] - Cookie=username,ssyy
Header Route Predicate Factroy
One of the two parameters is the attribute name and a regular expression. The attribute value matches the regular expression for execution
predicates: - Path=/payment/lb/** #Assert that the path matches the route # - After=2021-12-04T15:32:44.032+08:00[Asia/Shanghai] # - Cookie=username,ssyy - Header=X-Request-Id, \d+ #The request header must have an X-Request-Id attribute and a regular expression with an integer value
predicates: - Path=/payment/lb/** #Assert that the path matches the route # - After=2021-12-04T15:32:44.032+08:00[Asia/Shanghai] # - Cookie=username,ssyy - Header=X-Request-Id, \d+ #The request header must have an X-Request-Id attribute and a regular expression with an integer value - Host=**.angenin.com #Host: xxx.angelin.com request is that the host must have * *.Angelin.com - Method=GET #Only get request access is allowed - Path=/payment/lb/** #The url address to be accessed must be / payment/lb / - Query=username, \d+ #The url request address must take the username parameter and the value must be an integer
Filter
Routing filters can be used to modify incoming HTTP requests and returned HTTP responses. Routing filters can only be used by specifying routes
Many built-in filters are generated by the GateWayFilter factory
GatewayFilter (31 kinds)
Global Filter (10 kinds)
Custom filter
Create a new filter.MyLogGateWayFilter
@Component @Slf4j public class MyLogGateWayFilter implements GlobalFilter, Ordered { @Override public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) { log.info("***************come in MyLogGateWayFilter"+new Date()); String name = exchange.getRequest().getQueryParams().getFirst("name"); if (name == null){ log.info("********User name is null,Illegal user, T T"); exchange.getResponse().setStatusCode(HttpStatus.NOT_ACCEPTABLE); return exchange.getResponse().setComplete(); } return chain.filter(exchange); } @Override public int getOrder() { //The return value is the priority of the filter. The lower the priority, the higher the priority (minimum - 2147483648, maximum 2147483648) return 0; } }
Start 700180029527
http://localhost:9527/payment/lb?name=111
Take name and you'll succeed